23. DRL usage example
In this notebook example, Stable Baselines 3 has been used to train and to load an agent. However, Sinergym is completely agnostic to any DRL algorithm (although there are custom callbacks for SB3 specifically) and can be used with any DRL library that works with gymnasium interface.
23.1. Training a model
We are going to rely on the script available in the repository root called train_agent.py
. This script applies all the possibilities that Sinergym has to work with deep reinforcement learning algorithms and set parameters to everything so that we can define the training options from the execution of the script easily by a JSON file.
For more information about how run train_agent.py
, please, see Train a model.
[1]:
import sys
from datetime import datetime
import gymnasium as gym
import numpy as np
import wandb
from stable_baselines3 import *
from stable_baselines3.common.callbacks import CallbackList
from stable_baselines3.common.logger import HumanOutputFormat
from stable_baselines3.common.logger import Logger as SB3Logger
from stable_baselines3.common.monitor import Monitor
import sinergym
import sinergym.utils.gcloud as gcloud
from sinergym.utils.callbacks import *
from sinergym.utils.constants import *
from sinergym.utils.logger import CSVLogger, WandBOutputFormat
from sinergym.utils.rewards import *
from sinergym.utils.wrappers import *
First let’s define some variables for the execution.
[2]:
# Environment ID
environment = "Eplus-5zone-mixed-discrete-stochastic-v1"
# Training episodes
episodes = 5
#Name of the experiment
experiment_date = datetime.today().strftime('%Y-%m-%d_%H:%M')
experiment_name = 'SB3_DQN-' + environment + \
'-episodes-' + str(episodes)
experiment_name += '_' + experiment_date
We can combine this experiment executions with Weights&Biases in order to host all information extracted. With wandb, it’s possible to track and visualize all DRL training process in real time, register hyperparameters and details of each experiment, save artifacts such as models and sinergym output, and compare between different executions.
[3]:
# Create wandb.config object in order to log all experiment params
experiment_params = {
'sinergym-version': sinergym.__version__,
'python-version': sys.version
}
experiment_params.update({'environment':environment,
'episodes':episodes,
'algorithm':'SB3_DQN'})
# Get wandb init params (you have to specify your own project and entity)
wandb_params = {"project": 'sinergym',
"entity": 'alex_ugr'}
# Init wandb entry
run = wandb.init(
name=experiment_name + '_' + wandb.util.generate_id(),
config=experiment_params,
** wandb_params
)
Failed to detect the name of this notebook, you can set it manually with the WANDB_NOTEBOOK_NAME environment variable to enable code saving.
wandb: Currently logged in as: alex_ugr. Use `wandb login --relogin` to force relogin
/workspaces/sinergym/examples/wandb/run-20231117_094304-pf27dlou
Now we are ready to create the Gymnasium Environment. Here we use the environment name defined, remember that you can change default environment configuration. We will create a eval_env too in order to interact in the evaluation episodes. We can overwrite the env name with experiment name if we want.
[4]:
env = gym.make(environment, env_name=experiment_name)
eval_env = gym.make(environment, env_name=experiment_name+'_EVALUATION')
#==============================================================================================#
[ENVIRONMENT] (INFO) : Creating Gymnasium environment... [SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43]
#==============================================================================================#
[MODELING] (INFO) : Experiment working directory created [/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43-res1]
[MODELING] (INFO) : runperiod established: {'start_day': 1, 'start_month': 1, 'start_year': 1991, 'end_day': 31, 'end_month': 12, 'end_year': 1991, 'start_weekday': 1, 'n_steps_per_hour': 4}
[MODELING] (INFO) : Episode length (seconds): 31536000.0
[MODELING] (INFO) : timestep size (seconds): 900.0
[MODELING] (INFO) : timesteps per episode: 35040
[MODELING] (INFO) : Model Config is correct.
[REWARD] (INFO) : Reward function initialized.
[ENVIRONMENT] (INFO) : Environment SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43 created successfully.
[WRAPPER DiscretizeEnv] (INFO) : New Discrete Space and mapping: Discrete(10)
[WRAPPER DiscretizeEnv] (INFO) : Make sure that the action space is compatible and contained in the original environment.
[WRAPPER DiscretizeEnv] (INFO) : Wrapper initialized
#==============================================================================================#
[ENVIRONMENT] (INFO) : Creating Gymnasium environment... [SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43_EVALUATION]
#==============================================================================================#
[MODELING] (INFO) : Experiment working directory created [/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43_EVALUATION-res1]
[MODELING] (INFO) : runperiod established: {'start_day': 1, 'start_month': 1, 'start_year': 1991, 'end_day': 31, 'end_month': 12, 'end_year': 1991, 'start_weekday': 1, 'n_steps_per_hour': 4}
[MODELING] (INFO) : Episode length (seconds): 31536000.0
[MODELING] (INFO) : timestep size (seconds): 900.0
[MODELING] (INFO) : timesteps per episode: 35040
[MODELING] (INFO) : Model Config is correct.
[REWARD] (INFO) : Reward function initialized.
[ENVIRONMENT] (INFO) : Environment SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43_EVALUATION created successfully.
[WRAPPER DiscretizeEnv] (INFO) : New Discrete Space and mapping: Discrete(10)
[WRAPPER DiscretizeEnv] (INFO) : Make sure that the action space is compatible and contained in the original environment.
[WRAPPER DiscretizeEnv] (INFO) : Wrapper initialized
We can also add a Wrapper to the environment, we are going to use an action normalization wrapper and a logger (extensions of gym.Wrapper
). Normalization is very recommended in DRL algorithms with continuous action space and logger is used to monitor and log the interactions with the environment and save the data into a CSV. Files generated will be stored as artifact in wandb too.
[6]:
env = LoggerWrapper(env)
eval_env = LoggerWrapper(eval_env)
[WRAPPER LoggerWrapper] (INFO) : Wrapper initialized.
[WRAPPER LoggerWrapper] (INFO) : Wrapper initialized.
At this point, we have the environment set up and ready to be used. We are going to create our learning model (Stable Baselines 3 DQN), but we can use any other algorithm.
[7]:
model = DQN('MlpPolicy', env, verbose=1)
Using cpu device
Wrapping the env with a `Monitor` wrapper
Wrapping the env in a DummyVecEnv.
Evaluation will execute the current model during a number of episodes determined to decide if it is the best current version of the model at that point of the training. Output generated will be stored in wandb server too. We are going to use the LoggerEval callback to print and save the best model evaluated during training.
[8]:
callbacks = []
# Set up Evaluation and saving best model
eval_callback = LoggerEvalCallback(
eval_env,
best_model_save_path=eval_env.get_wrapper_attr('workspace_path') +
'/best_model/',
log_path=eval_env.get_wrapper_attr('workspace_path') +
'/best_model/',
eval_freq=(eval_env.get_wrapper_attr('timestep_per_episode') - 1) * 2 - 1,
deterministic=True,
render=False,
n_eval_episodes=1)
callbacks.append(eval_callback)
In order to track all the training process in wandb, it is necessary to create a callback with a compatible wandb output format (which call wandb log method in the learning algorithm process).
[9]:
# wandb logger and setting in SB3
logger = SB3Logger(
folder=None,
output_formats=[
HumanOutputFormat(
sys.stdout,
max_length=120),
WandBOutputFormat()])
model.set_logger(logger)
# Append callback
log_callback = LoggerCallback()
callbacks.append(log_callback)
callback = CallbackList(callbacks)
This is the number of total time steps for the training.
[10]:
timesteps = episodes * (env.get_wrapper_attr('timestep_per_episode') - 1)
Now, is time to train the model with the callbacks defined earlier. This may take a few minutes, depending on your computer.
- warning:
The warning messages that appear in
model.learn()
output is due to Stable Baselines 3 is not adapted to new standar to get environment attributes yet.
[11]:
model.learn(
total_timesteps=timesteps,
callback=callback,
log_interval=1)
#----------------------------------------------------------------------------------------------#
[ENVIRONMENT] (INFO) : Starting a new episode... [SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43] [Episode 1]
#----------------------------------------------------------------------------------------------#
[MODELING] (INFO) : Episode directory created [/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43-res1/Eplus-env-sub_run1]
[MODELING] (INFO) : Weather file USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3.epw used.
[MODELING] (INFO) : Updated building model with whole Output:Variable available names
[MODELING] (INFO) : Updated building model with whole Output:Meter available names
[MODELING] (INFO) : Adapting weather to building model. [USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3.epw]
[ENVIRONMENT] (INFO) : Saving episode output path... [/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43-res1/Eplus-env-sub_run1/output]
/usr/local/lib/python3.10/dist-packages/opyplus/weather_data/weather_data.py:493: FutureWarning: the 'line_terminator'' keyword is deprecated, use 'lineterminator' instead.
epw_content = self._headers_to_epw(use_datetimes=use_datetimes) + df.to_csv(
[SIMULATOR] (INFO) : Running EnergyPlus with args: ['-w', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43-res1/Eplus-env-sub_run1/USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3_Random_1.0_0.0_0.001.epw', '-d', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43-res1/Eplus-env-sub_run1/output', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43-res1/Eplus-env-sub_run1/5ZoneAutoDXVAV.epJSON']
[ENVIRONMENT] (INFO) : Episode 1 started.
[SIMULATOR] (INFO) : handlers initialized.
[SIMULATOR] (INFO) : handlers are ready.
[SIMULATOR] (INFO) : System is ready.
[WRAPPER LoggerWrapper] (INFO) : Creating monitor.csv for current episode (episode 1) if logger is active
--------------------------------------------------------------------------------------------------------------| 1%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 3296.2168 |
| air_humidity | 14.309143 |
| air_temperature | 19.127739 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 22.0 |
| month | 1.0 |
| outdoor_humidity | 39.0 |
| outdoor_temperature | -9.724175 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2966595.2 |
| wind_direction | 320.0 |
| wind_speed | 7.7 |
| rollout/ | |
| exploration_rate | 0.995 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 1%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1932.6711 |
| air_humidity | 15.199731 |
| air_temperature | 20.344173 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 22.0 |
| month | 1.0 |
| outdoor_humidity | 62.0 |
| outdoor_temperature | -2.906447 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1739404.0 |
| wind_direction | 60.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.989 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 1%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 1637.6606 |
| air_humidity | 34.85248 |
| air_temperature | 20.126642 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 21.0 |
| month | 1.0 |
| outdoor_humidity | 62.0 |
| outdoor_temperature | -1.4313949 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1473894.6 |
| wind_direction | 270.0 |
| wind_speed | 11.3 |
| rollout/ | |
| exploration_rate | 0.984 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 1%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 2696.1394 |
| air_humidity | 17.76754 |
| air_temperature | 18.343163 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 17.0 |
| month | 1.0 |
| outdoor_humidity | 50.0 |
| outdoor_temperature | -6.7237883 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2426525.5 |
| wind_direction | 270.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.978 |
------------------------------------------------
/workspaces/sinergym/sinergym/utils/callbacks.py:279: UserWarning: Training and eval env are not of the same type<stable_baselines3.common.vec_env.dummy_vec_env.DummyVecEnv object at 0x7f6e4589ee90> != <LoggerWrapper<DiscretizeEnv<OrderEnforcing<PassiveEnvChecker<EplusEnv<Eplus-5zone-mixed-discrete-stochastic-v1>>>>>>
warnings.warn(
/usr/local/lib/python3.10/dist-packages/gymnasium/spaces/box.py:240: UserWarning: WARN: Casting input x to numpy array.
gym.logger.warn("Casting input x to numpy array.")
/usr/local/lib/python3.10/dist-packages/gymnasium/core.py:311: UserWarning: WARN: env.observation_variables to get variables from other wrappers is deprecated and will be removed in v1.0, to get this variable you can do `env.unwrapped.observation_variables` for environment variables or `env.get_wrapper_attr('observation_variables')` that will search the reminding wrappers.
logger.warn(
/usr/local/lib/python3.10/dist-packages/gymnasium/core.py:311: UserWarning: WARN: env.action_variables to get variables from other wrappers is deprecated and will be removed in v1.0, to get this variable you can do `env.unwrapped.action_variables` for environment variables or `env.get_wrapper_attr('action_variables')` that will search the reminding wrappers.
logger.warn(
/usr/local/lib/python3.10/dist-packages/gymnasium/core.py:311: UserWarning: WARN: env.is_discrete to get variables from other wrappers is deprecated and will be removed in v1.0, to get this variable you can do `env.unwrapped.is_discrete` for environment variables or `env.get_wrapper_attr('is_discrete')` that will search the reminding wrappers.
logger.warn(
--------------------------------------------------------------------------------------------------------------| 2%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 2682.915 |
| air_humidity | 12.916248 |
| air_temperature | 19.004652 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 19.0 |
| month | 1.0 |
| outdoor_humidity | 67.0 |
| outdoor_temperature | -6.6576667 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2414623.5 |
| wind_direction | 240.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.973 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 2%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 1243.6388 |
| air_humidity | 13.383576 |
| air_temperature | 18.792883 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 15.0 |
| month | 1.0 |
| outdoor_humidity | 60.0 |
| outdoor_temperature | 0.53871423 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1119275.0 |
| wind_direction | 240.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.968 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 2%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 1664.6848 |
| air_humidity | 13.20179 |
| air_temperature | 18.895155 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 6.0 |
| htg_setpoint | 19.0 |
| month | 1.0 |
| outdoor_humidity | 41.0 |
| outdoor_temperature | -5.1032705 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1498216.4 |
| wind_direction | 50.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.962 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 2%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 244.03078 |
| air_humidity | 33.12003 |
| air_temperature | 19.675444 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 39.5 |
| direct_solar_radiation | 9.0 |
| hour | 7.0 |
| htg_setpoint | 16.0 |
| month | 1.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 3.195037 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 219627.69 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.957 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 3%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 1803.2925 |
| air_humidity | 20.75208 |
| air_temperature | 22.000008 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 124.5 |
| direct_solar_radiation | 318.0 |
| hour | 8.0 |
| htg_setpoint | 22.0 |
| month | 1.0 |
| outdoor_humidity | 72.0 |
| outdoor_temperature | -2.6576498 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1622963.2 |
| wind_direction | 340.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.951 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 3%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 2222.2266 |
| air_humidity | 13.443321 |
| air_temperature | 22.207747 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 158.0 |
| direct_solar_radiation | 228.0 |
| hour | 9.0 |
| htg_setpoint | 18.0 |
| month | 1.0 |
| outdoor_humidity | 37.0 |
| outdoor_temperature | -4.8114486 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 2000003.9 |
| wind_direction | 260.0 |
| wind_speed | 8.8 |
| rollout/ | |
| exploration_rate | 0.946 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 3%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 130.00592 |
| air_humidity | 16.974932 |
| air_temperature | 19.933048 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 202.5 |
| direct_solar_radiation | 79.0 |
| hour | 10.0 |
| htg_setpoint | 19.0 |
| month | 1.0 |
| outdoor_humidity | 61.0 |
| outdoor_temperature | 4.3786383 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 114245.734 |
| wind_direction | 240.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.94 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 4%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 73.03831 |
| air_humidity | 25.305868 |
| air_temperature | 20.497007 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 175.0 |
| direct_solar_radiation | 6.0 |
| hour | 11.0 |
| htg_setpoint | 17.0 |
| month | 1.0 |
| outdoor_humidity | 65.0 |
| outdoor_temperature | 7.873773 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 67578.96 |
| wind_direction | 220.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.935 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 4%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 2316.9001 |
| air_humidity | 15.797696 |
| air_temperature | 21.902811 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 76.0 |
| direct_solar_radiation | 0.0 |
| hour | 12.0 |
| htg_setpoint | 21.0 |
| month | 1.0 |
| outdoor_humidity | 83.0 |
| outdoor_temperature | -7.2039795 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 2085210.1 |
| wind_direction | 50.0 |
| wind_speed | 9.8 |
| rollout/ | |
| exploration_rate | 0.93 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 4%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 755.6465 |
| air_humidity | 38.209152 |
| air_temperature | 22.889372 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 140.5 |
| direct_solar_radiation | 12.0 |
| hour | 13.0 |
| htg_setpoint | 15.0 |
| month | 1.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 3.3975253 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 680081.9 |
| wind_direction | 250.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.924 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 4%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 894.3857 |
| air_humidity | 26.755064 |
| air_temperature | 23.927494 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 71.5 |
| direct_solar_radiation | 590.5 |
| hour | 14.0 |
| htg_setpoint | 18.0 |
| month | 1.0 |
| outdoor_humidity | 70.0 |
| outdoor_temperature | 5.5716357 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 804947.06 |
| wind_direction | 210.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.919 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 5%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 24.887455 |
| air_temperature | 24.93139 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 33.0 |
| direct_solar_radiation | 392.0 |
| hour | 15.0 |
| htg_setpoint | 22.0 |
| month | 1.0 |
| outdoor_humidity | 43.0 |
| outdoor_temperature | 10.7322 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 220.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.913 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 5%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 596.4898 |
| air_humidity | 55.523884 |
| air_temperature | 23.077408 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 16.0 |
| htg_setpoint | 21.0 |
| month | 1.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 11.750119 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 536840.8 |
| wind_direction | 160.0 |
| wind_speed | 11.8 |
| rollout/ | |
| exploration_rate | 0.908 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 5%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 21.506569 |
| air_temperature | 21.110353 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 21.0 |
| month | 1.0 |
| outdoor_humidity | 39.0 |
| outdoor_temperature | 5.498105 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 250.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.903 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 5%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 22.093334 |
| air_temperature | 20.600706 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 17.0 |
| month | 1.0 |
| outdoor_humidity | 82.0 |
| outdoor_temperature | 4.190033 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 200.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.897 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 6%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 479.13632 |
| air_humidity | 43.773174 |
| air_temperature | 20.674847 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 20.0 |
| month | 1.0 |
| outdoor_humidity | 90.0 |
| outdoor_temperature | 7.8929534 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 414870.66 |
| wind_direction | 40.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.892 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 6%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 840.7436 |
| air_humidity | 37.7276 |
| air_temperature | 20.371922 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 21.0 |
| month | 1.0 |
| outdoor_humidity | 97.0 |
| outdoor_temperature | 5.074561 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 785942.25 |
| wind_direction | 60.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.886 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 6%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 401.62372 |
| air_humidity | 58.423054 |
| air_temperature | 19.202482 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 15.0 |
| month | 1.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 10.24485 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 361461.34 |
| wind_direction | 150.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.881 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 7%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 854.80945 |
| air_humidity | 38.847115 |
| air_temperature | 19.064434 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 16.0 |
| month | 1.0 |
| outdoor_humidity | 62.0 |
| outdoor_temperature | 4.9329586 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 716942.4 |
| wind_direction | 310.0 |
| wind_speed | 9.3 |
| rollout/ | |
| exploration_rate | 0.875 |
-----------------------------------------------
Progress: |*******--------------------------------------------------------------------------------------------| 7%-----------------------------------------------
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 35.6719 |
| air_temperature | 20.34233 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 16.0 |
| month | 1.0 |
| outdoor_humidity | 64.0 |
| outdoor_temperature | 2.1815379 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 310.0 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.87 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 7%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1108.0092 |
| air_humidity | 24.518076 |
| air_temperature | 20.095318 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 17.0 |
| month | 1.0 |
| outdoor_humidity | 88.0 |
| outdoor_temperature | 1.2168629 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 997208.2 |
| wind_direction | 250.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.865 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 8%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 27.546406 |
| air_temperature | 19.860954 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 19.0 |
| month | 1.0 |
| outdoor_humidity | 92.0 |
| outdoor_temperature | 3.5815425 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 30.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.859 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 8%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 41.918938 |
| air_temperature | 19.22273 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 18.0 |
| month | 1.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 2.6798549 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 340.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.854 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 8%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 2011.6338 |
| air_humidity | 24.83714 |
| air_temperature | 20.192572 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 21.0 |
| month | 1.0 |
| outdoor_humidity | 65.0 |
| outdoor_temperature | -3.3012602 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1810470.4 |
| wind_direction | 340.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.848 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 8%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 2908.7742 |
| air_humidity | 24.490915 |
| air_temperature | 19.521425 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 22.0 |
| month | 1.0 |
| outdoor_humidity | 33.0 |
| outdoor_temperature | -7.786963 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2617896.8 |
| wind_direction | 20.0 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.843 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 9%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 18.300123 |
| air_temperature | 19.512716 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 22.0 |
| month | 2.0 |
| outdoor_humidity | 70.0 |
| outdoor_temperature | 0.636746 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 250.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.837 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 9%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 245.2585 |
| air_humidity | 27.539667 |
| air_temperature | 19.327127 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 6.0 |
| htg_setpoint | 18.0 |
| month | 2.0 |
| outdoor_humidity | 45.0 |
| outdoor_temperature | 4.9906583 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 211297.28 |
| wind_direction | 320.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.832 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 9%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 2847.735 |
| air_humidity | 13.705198 |
| air_temperature | 17.869244 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 32.0 |
| direct_solar_radiation | 410.0 |
| hour | 7.0 |
| htg_setpoint | 17.0 |
| month | 2.0 |
| outdoor_humidity | 53.0 |
| outdoor_temperature | -7.4817667 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2562961.5 |
| wind_direction | 20.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.827 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 10%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 1402.2615 |
| air_humidity | 24.444305 |
| air_temperature | 20.994953 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 52.5 |
| direct_solar_radiation | 0.0 |
| hour | 8.0 |
| htg_setpoint | 15.0 |
| month | 2.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | -0.7116232 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1262035.2 |
| wind_direction | 60.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.821 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 10%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 2248.6406 |
| air_humidity | 14.282108 |
| air_temperature | 21.999893 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 113.5 |
| direct_solar_radiation | 589.0 |
| hour | 9.0 |
| htg_setpoint | 22.0 |
| month | 2.0 |
| outdoor_humidity | 43.0 |
| outdoor_temperature | -4.8843904 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 2023776.5 |
| wind_direction | 300.0 |
| wind_speed | 11.8 |
| rollout/ | |
| exploration_rate | 0.816 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 10%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 3078.3347 |
| air_humidity | 12.583989 |
| air_temperature | 22.56294 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 143.0 |
| direct_solar_radiation | 538.0 |
| hour | 10.0 |
| htg_setpoint | 18.0 |
| month | 2.0 |
| outdoor_humidity | 52.0 |
| outdoor_temperature | -9.032861 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 2770501.2 |
| wind_direction | 280.0 |
| wind_speed | 7.7 |
| rollout/ | |
| exploration_rate | 0.81 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 10%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 2229.576 |
| air_humidity | 14.426971 |
| air_temperature | 22.87635 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 217.5 |
| direct_solar_radiation | 431.0 |
| hour | 11.0 |
| htg_setpoint | 19.0 |
| month | 2.0 |
| outdoor_humidity | 48.0 |
| outdoor_temperature | -5.2337403 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 2006618.4 |
| wind_direction | 270.0 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.805 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 11%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 1377.8375 |
| air_humidity | 12.806718 |
| air_temperature | 23.000334 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 144.0 |
| direct_solar_radiation | 682.0 |
| hour | 12.0 |
| htg_setpoint | 22.0 |
| month | 2.0 |
| outdoor_humidity | 32.0 |
| outdoor_temperature | -1.3840142 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 1260863.0 |
| wind_direction | 340.0 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.799 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 11%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1800.8235 |
| air_humidity | 12.199289 |
| air_temperature | 21.39844 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 141.0 |
| direct_solar_radiation | 497.5 |
| hour | 13.0 |
| htg_setpoint | 15.0 |
| month | 2.0 |
| outdoor_humidity | 42.0 |
| outdoor_temperature | -2.247209 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1620741.1 |
| wind_direction | 260.0 |
| wind_speed | 7.7 |
| rollout/ | |
| exploration_rate | 0.794 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 11%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 11.153282 |
| air_temperature | 22.06529 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 150.5 |
| direct_solar_radiation | 334.5 |
| hour | 14.0 |
| htg_setpoint | 15.0 |
| month | 2.0 |
| outdoor_humidity | 55.0 |
| outdoor_temperature | 3.2212148 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 190.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.789 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 11%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 917.7593 |
| air_humidity | 26.109697 |
| air_temperature | 23.784363 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 78.0 |
| direct_solar_radiation | 132.5 |
| hour | 15.0 |
| htg_setpoint | 22.0 |
| month | 2.0 |
| outdoor_humidity | 61.0 |
| outdoor_temperature | 5.499552 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 825983.3 |
| wind_direction | 250.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.783 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 12%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 1934.6064 |
| air_humidity | 12.981752 |
| air_temperature | 23.084322 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 29.0 |
| direct_solar_radiation | 92.0 |
| hour | 16.0 |
| htg_setpoint | 17.0 |
| month | 2.0 |
| outdoor_humidity | 23.0 |
| outdoor_temperature | -3.3733482 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1741145.8 |
| wind_direction | 310.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.778 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 12%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1652.0598 |
| air_humidity | 16.321648 |
| air_temperature | 22.911978 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 15.0 |
| month | 2.0 |
| outdoor_humidity | 40.0 |
| outdoor_temperature | -1.960615 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1486853.9 |
| wind_direction | 290.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.772 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 12%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 1822.9177 |
| air_humidity | 17.573734 |
| air_temperature | 24.39188 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 22.0 |
| month | 2.0 |
| outdoor_humidity | 46.0 |
| outdoor_temperature | -2.3576803 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 1640626.0 |
| wind_direction | 270.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.767 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 13%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 32.81119 |
| air_temperature | 22.278952 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 18.0 |
| month | 2.0 |
| outdoor_humidity | 92.0 |
| outdoor_temperature | 3.2496963 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 70.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.762 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 13%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 122.831604 |
| air_humidity | 23.412853 |
| air_temperature | 20.056593 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 15.0 |
| month | 2.0 |
| outdoor_humidity | 53.0 |
| outdoor_temperature | 4.509193 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 107374.445 |
| wind_direction | 50.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.756 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 13%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 18.018625 |
| air_temperature | 20.911348 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 18.0 |
| month | 2.0 |
| outdoor_humidity | 71.0 |
| outdoor_temperature | 2.9650793 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 200.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.751 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 13%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 1572.392 |
| air_humidity | 32.772217 |
| air_temperature | 21.000002 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 21.0 |
| month | 2.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | -1.105051 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1415152.8 |
| wind_direction | 60.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.745 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 14%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 27.115477 |
| air_temperature | 21.000715 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 21.0 |
| month | 2.0 |
| outdoor_humidity | 69.0 |
| outdoor_temperature | 3.6110175 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 220.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.74 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 14%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 32.682682 |
| air_temperature | 21.646172 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 22.0 |
| month | 2.0 |
| outdoor_humidity | 89.0 |
| outdoor_temperature | 2.226016 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 60.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.734 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 14%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 1172.5454 |
| air_humidity | 30.718266 |
| air_temperature | 19.741425 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 17.0 |
| month | 2.0 |
| outdoor_humidity | 56.0 |
| outdoor_temperature | 0.8941815 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1055290.9 |
| wind_direction | 330.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.729 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 15%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 26.496115 |
| air_temperature | 19.798346 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 18.0 |
| month | 2.0 |
| outdoor_humidity | 54.0 |
| outdoor_temperature | 2.6684391 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 220.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.724 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 15%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 145.03104 |
| air_humidity | 30.124374 |
| air_temperature | 21.0 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 21.0 |
| month | 2.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 4.497048 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 131813.45 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.718 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 15%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 2359.907 |
| air_humidity | 15.937994 |
| air_temperature | 19.36234 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 22.0 |
| month | 2.0 |
| outdoor_humidity | 37.0 |
| outdoor_temperature | -5.0426264 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2123916.2 |
| wind_direction | 320.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.713 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 16%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 1996.818 |
| air_humidity | 20.799343 |
| air_temperature | 20.004538 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 20.0 |
| month | 2.0 |
| outdoor_humidity | 79.0 |
| outdoor_temperature | -3.2271817 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1797136.2 |
| wind_direction | 50.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.707 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 16%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 1015.25275 |
| air_humidity | 18.425447 |
| air_temperature | 18.836422 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 17.5 |
| direct_solar_radiation | 0.0 |
| hour | 6.0 |
| htg_setpoint | 16.0 |
| month | 2.0 |
| outdoor_humidity | 80.0 |
| outdoor_temperature | -1.85611 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 913727.5 |
| wind_direction | 70.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.702 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 16%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 244.03078 |
| air_humidity | 32.43741 |
| air_temperature | 19.892696 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 41.0 |
| direct_solar_radiation | 0.0 |
| hour | 7.0 |
| htg_setpoint | 22.0 |
| month | 2.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 2.2410598 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 219627.69 |
| wind_direction | 70.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.696 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 16%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 796.70184 |
| air_humidity | 27.512812 |
| air_temperature | 21.87516 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 122.5 |
| direct_solar_radiation | 655.0 |
| hour | 8.0 |
| htg_setpoint | 20.0 |
| month | 3.0 |
| outdoor_humidity | 37.0 |
| outdoor_temperature | 11.139362 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 730410.0 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.691 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 17%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 103.10883 |
| air_humidity | 35.254738 |
| air_temperature | 20.343086 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 215.5 |
| direct_solar_radiation | 48.5 |
| hour | 9.0 |
| htg_setpoint | 15.0 |
| month | 3.0 |
| outdoor_humidity | 97.0 |
| outdoor_temperature | 5.938736 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 91218.086 |
| wind_direction | 180.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.686 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 17%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 27.49837 |
| air_temperature | 21.649036 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 146.0 |
| direct_solar_radiation | 788.0 |
| hour | 10.0 |
| htg_setpoint | 19.0 |
| month | 3.0 |
| outdoor_humidity | 47.0 |
| outdoor_temperature | 12.220809 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 320.0 |
| wind_speed | 11.3 |
| rollout/ | |
| exploration_rate | 0.68 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 17%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 755.6465 |
| air_humidity | 39.67366 |
| air_temperature | 22.92239 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 114.5 |
| direct_solar_radiation | 0.0 |
| hour | 11.0 |
| htg_setpoint | 22.0 |
| month | 3.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 5.7229357 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 680081.9 |
| wind_direction | 140.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.675 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 17%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 476.10425 |
| air_humidity | 40.264465 |
| air_temperature | 22.832756 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 144.0 |
| direct_solar_radiation | 0.0 |
| hour | 12.0 |
| htg_setpoint | 16.0 |
| month | 3.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 6.998226 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 428493.84 |
| wind_direction | 110.0 |
| wind_speed | 7.7 |
| rollout/ | |
| exploration_rate | 0.669 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 18%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 891.2718 |
| air_humidity | 54.31145 |
| air_temperature | 23.449625 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 95.0 |
| direct_solar_radiation | 0.0 |
| hour | 13.0 |
| htg_setpoint | 22.0 |
| month | 3.0 |
| outdoor_humidity | 97.0 |
| outdoor_temperature | 13.390144 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 829194.06 |
| wind_direction | 240.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.664 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 18%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 25.151756 |
| air_temperature | 24.632774 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 117.5 |
| direct_solar_radiation | 714.5 |
| hour | 14.0 |
| htg_setpoint | 22.0 |
| month | 3.0 |
| outdoor_humidity | 45.0 |
| outdoor_temperature | 10.913635 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 230.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.658 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 18%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 865.85455 |
| air_humidity | 32.74997 |
| air_temperature | 23.198282 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 123.0 |
| direct_solar_radiation | 33.5 |
| hour | 15.0 |
| htg_setpoint | 15.0 |
| month | 3.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 1.9704113 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 779269.06 |
| wind_direction | 70.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.653 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 19%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 964.41034 |
| air_humidity | 33.18654 |
| air_temperature | 20.914831 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 54.0 |
| direct_solar_radiation | 47.5 |
| hour | 16.0 |
| htg_setpoint | 20.0 |
| month | 3.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 1.9348568 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 867969.3 |
| wind_direction | 140.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.648 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 19%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 24.41243 |
| air_temperature | 21.10522 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 18.0 |
| month | 3.0 |
| outdoor_humidity | 56.0 |
| outdoor_temperature | 6.1129913 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 30.0 |
| wind_speed | 9.3 |
| rollout/ | |
| exploration_rate | 0.642 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 19%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 605.5506 |
| air_humidity | 28.835632 |
| air_temperature | 22.536592 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 22.0 |
| month | 3.0 |
| outdoor_humidity | 67.0 |
| outdoor_temperature | 5.9020243 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 562977.0 |
| wind_direction | 180.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.637 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 19%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 20.433092 |
| air_temperature | 22.391197 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 15.0 |
| month | 3.0 |
| outdoor_humidity | 39.0 |
| outdoor_temperature | 2.9986107 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 290.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.631 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 20%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1046.4424 |
| air_humidity | 21.538439 |
| air_temperature | 22.372751 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 22.0 |
| month | 3.0 |
| outdoor_humidity | 27.0 |
| outdoor_temperature | 1.5246968 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 941798.06 |
| wind_direction | 320.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.626 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 20%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 732.6514 |
| air_humidity | 34.63837 |
| air_temperature | 20.51618 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 22.0 |
| month | 3.0 |
| outdoor_humidity | 89.0 |
| outdoor_temperature | 4.440274 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 671326.8 |
| wind_direction | 180.0 |
| wind_speed | 8.2 |
| rollout/ | |
| exploration_rate | 0.621 |
----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 20%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 375.99283 |
| air_humidity | 16.011675 |
| air_temperature | 20.772934 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 22.0 |
| month | 3.0 |
| outdoor_humidity | 35.0 |
| outdoor_temperature | 8.169079 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 348609.22 |
| wind_direction | 20.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.615 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 20%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 1442.9108 |
| air_humidity | 21.037851 |
| air_temperature | 19.690607 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 18.0 |
| month | 3.0 |
| outdoor_humidity | 88.0 |
| outdoor_temperature | -0.4576453 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1298619.6 |
| wind_direction | 30.0 |
| wind_speed | 8.8 |
| rollout/ | |
| exploration_rate | 0.61 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 21%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 1431.4702 |
| air_humidity | 19.788712 |
| air_temperature | 20.099007 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 20.0 |
| month | 3.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | -0.40044233 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1288323.1 |
| wind_direction | 10.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.604 |
-------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 21%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 1195.8418 |
| air_humidity | 35.305504 |
| air_temperature | 20.161875 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 15.0 |
| month | 3.0 |
| outdoor_humidity | 92.0 |
| outdoor_temperature | 0.7776995 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1076257.6 |
| wind_direction | 90.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.599 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 22%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 1452.4503 |
| air_humidity | 32.657394 |
| air_temperature | 20.009953 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 19.0 |
| month | 3.0 |
| outdoor_humidity | 69.0 |
| outdoor_temperature | -0.5053432 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1307205.2 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.593 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 22%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 39.329704 |
| air_temperature | 19.524014 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 22.0 |
| month | 3.0 |
| outdoor_humidity | 97.0 |
| outdoor_temperature | 6.9832764 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 290.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.588 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 22%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1585.7318 |
| air_humidity | 19.347166 |
| air_temperature | 19.60325 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 17.0 |
| month | 3.0 |
| outdoor_humidity | 48.0 |
| outdoor_temperature | -1.1717507 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1427158.6 |
| wind_direction | 310.0 |
| wind_speed | 7.7 |
| rollout/ | |
| exploration_rate | 0.583 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 22%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 2212.4136 |
| air_humidity | 16.154156 |
| air_temperature | 19.999226 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 33.0 |
| direct_solar_radiation | 57.5 |
| hour | 5.0 |
| htg_setpoint | 20.0 |
| month | 3.0 |
| outdoor_humidity | 39.0 |
| outdoor_temperature | -4.3051596 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1991172.2 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.577 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 23%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 17.629131 |
| air_temperature | 20.066673 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 84.5 |
| direct_solar_radiation | 311.0 |
| hour | 6.0 |
| htg_setpoint | 20.0 |
| month | 3.0 |
| outdoor_humidity | 89.0 |
| outdoor_temperature | 2.4818852 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 250.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.572 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 23%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 244.03078 |
| air_humidity | 39.27392 |
| air_temperature | 19.567928 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 119.0 |
| direct_solar_radiation | 87.0 |
| hour | 7.0 |
| htg_setpoint | 16.0 |
| month | 3.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 6.0872054 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 219627.69 |
| wind_direction | 190.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.566 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 23%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 775.9277 |
| air_humidity | 44.55901 |
| air_temperature | 21.999832 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 176.5 |
| direct_solar_radiation | 253.0 |
| hour | 8.0 |
| htg_setpoint | 22.0 |
| month | 3.0 |
| outdoor_humidity | 83.0 |
| outdoor_temperature | 11.008395 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 718974.2 |
| wind_direction | 200.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.561 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 23%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 342.72076 |
| air_humidity | 59.920914 |
| air_temperature | 23.489557 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 135.0 |
| direct_solar_radiation | 0.0 |
| hour | 9.0 |
| htg_setpoint | 20.0 |
| month | 3.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 14.705147 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 312361.38 |
| wind_direction | 250.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.555 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 24%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 329.98727 |
| air_humidity | 34.334488 |
| air_temperature | 24.88112 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 282.0 |
| direct_solar_radiation | 626.5 |
| hour | 10.0 |
| htg_setpoint | 18.0 |
| month | 3.0 |
| outdoor_humidity | 51.0 |
| outdoor_temperature | 14.963529 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 299526.44 |
| wind_direction | 30.0 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.55 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 24%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 669.0633 |
| air_humidity | 28.5489 |
| air_temperature | 24.056192 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 146.5 |
| direct_solar_radiation | 873.5 |
| hour | 11.0 |
| htg_setpoint | 17.0 |
| month | 3.0 |
| outdoor_humidity | 68.0 |
| outdoor_temperature | 7.029778 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 608366.6 |
| wind_direction | 110.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.545 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 24%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 77.871574 |
| air_humidity | 32.33923 |
| air_temperature | 20.417665 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 341.5 |
| direct_solar_radiation | 27.0 |
| hour | 12.0 |
| htg_setpoint | 15.0 |
| month | 3.0 |
| outdoor_humidity | 70.0 |
| outdoor_temperature | 5.2663016 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 72798.83 |
| wind_direction | 120.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.539 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 25%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 39.8185 |
| air_temperature | 20.66404 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 144.0 |
| direct_solar_radiation | 0.0 |
| hour | 13.0 |
| htg_setpoint | 17.0 |
| month | 3.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 8.080436 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 10.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.534 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 25%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 676.7058 |
| air_humidity | 35.900913 |
| air_temperature | 22.70483 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 153.5 |
| direct_solar_radiation | 117.5 |
| hour | 14.0 |
| htg_setpoint | 17.0 |
| month | 4.0 |
| outdoor_humidity | 82.0 |
| outdoor_temperature | 6.9450645 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 609035.25 |
| wind_direction | 170.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.528 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 25%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 493.36768 |
| air_humidity | 20.675724 |
| air_temperature | 23.906479 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 140.0 |
| direct_solar_radiation | 323.0 |
| hour | 15.0 |
| htg_setpoint | 19.0 |
| month | 4.0 |
| outdoor_humidity | 32.0 |
| outdoor_temperature | 7.8594294 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 439279.62 |
| wind_direction | 320.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.523 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 25%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 478.49545 |
| air_humidity | 24.581656 |
| air_temperature | 24.346413 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 51.0 |
| direct_solar_radiation | 528.0 |
| hour | 16.0 |
| htg_setpoint | 15.0 |
| month | 4.0 |
| outdoor_humidity | 53.0 |
| outdoor_temperature | 7.0104027 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 430645.9 |
| wind_direction | 200.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.518 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 26%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 35.376736 |
| air_temperature | 29.603888 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 36.0 |
| direct_solar_radiation | 35.5 |
| hour | 17.0 |
| htg_setpoint | 21.0 |
| month | 4.0 |
| outdoor_humidity | 68.0 |
| outdoor_temperature | 11.504218 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.512 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 26%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 48.570503 |
| air_temperature | 27.450226 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 17.0 |
| month | 4.0 |
| outdoor_humidity | 86.0 |
| outdoor_temperature | 10.577883 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 240.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.507 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 26%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 59.77879 |
| air_temperature | 24.601759 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 16.0 |
| month | 4.0 |
| outdoor_humidity | 80.0 |
| outdoor_temperature | 12.718971 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.501 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 26%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 55.434128 |
| air_temperature | 25.836447 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 21.0 |
| month | 4.0 |
| outdoor_humidity | 54.0 |
| outdoor_temperature | 20.91227 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 270.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.496 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 27%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 78.805756 |
| air_temperature | 24.448067 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 18.0 |
| month | 4.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | 13.798377 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.49 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 27%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 78.469086 |
| air_temperature | 23.550816 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 21.0 |
| month | 4.0 |
| outdoor_humidity | 90.0 |
| outdoor_temperature | 16.486246 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 180.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.485 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 27%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 57.217648 |
| air_temperature | 22.522837 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 19.0 |
| month | 4.0 |
| outdoor_humidity | 43.0 |
| outdoor_temperature | 9.949017 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 290.0 |
| wind_speed | 9.8 |
| rollout/ | |
| exploration_rate | 0.48 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 28%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 46.25956 |
| air_temperature | 21.33544 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 21.0 |
| month | 4.0 |
| outdoor_humidity | 28.0 |
| outdoor_temperature | 7.333998 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 330.0 |
| wind_speed | 8.8 |
| rollout/ | |
| exploration_rate | 0.474 |
----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 28%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 37.304005 |
| air_temperature | 21.064104 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 19.0 |
| month | 4.0 |
| outdoor_humidity | 57.0 |
| outdoor_temperature | 6.7171063 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 250.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.469 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 28%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 43.14323 |
| air_temperature | 18.625456 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 20.0 |
| month | 4.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 3.6882412 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 80.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.463 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 29%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 42.04449 |
| air_temperature | 18.97571 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 22.0 |
| month | 4.0 |
| outdoor_humidity | 89.0 |
| outdoor_temperature | 5.208225 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 110.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.458 |
----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 29%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 81.08231 |
| air_temperature | 19.258926 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 16.0 |
| month | 4.0 |
| outdoor_humidity | 90.0 |
| outdoor_temperature | 9.289529 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 250.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.452 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 29%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 82.286415 |
| air_temperature | 20.703104 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 38.5 |
| direct_solar_radiation | 16.5 |
| hour | 5.0 |
| htg_setpoint | 21.0 |
| month | 4.0 |
| outdoor_humidity | 89.0 |
| outdoor_temperature | 14.200624 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.447 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 29%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 88.664734 |
| air_temperature | 18.851236 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 66.5 |
| direct_solar_radiation | 0.0 |
| hour | 6.0 |
| htg_setpoint | 19.0 |
| month | 4.0 |
| outdoor_humidity | 85.0 |
| outdoor_temperature | 5.579129 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 70.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.442 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 30%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 244.03078 |
| air_humidity | 50.477306 |
| air_temperature | 18.83049 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 201.0 |
| direct_solar_radiation | 329.0 |
| hour | 7.0 |
| htg_setpoint | 21.0 |
| month | 4.0 |
| outdoor_humidity | 54.0 |
| outdoor_temperature | 7.4226103 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 219627.69 |
| wind_direction | 80.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.436 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 30%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 62.191772 |
| air_temperature | 19.665392 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 185.0 |
| direct_solar_radiation | 0.0 |
| hour | 8.0 |
| htg_setpoint | 19.0 |
| month | 4.0 |
| outdoor_humidity | 80.0 |
| outdoor_temperature | 8.042038 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 100.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.431 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 30%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 62.690765 |
| air_temperature | 19.137825 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 201.0 |
| direct_solar_radiation | 0.0 |
| hour | 9.0 |
| htg_setpoint | 17.0 |
| month | 4.0 |
| outdoor_humidity | 97.0 |
| outdoor_temperature | 7.335754 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 30.0 |
| wind_speed | 10.3 |
| rollout/ | |
| exploration_rate | 0.425 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 31%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 840.9814 |
| air_humidity | 37.737446 |
| air_temperature | 22.171951 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 247.0 |
| direct_solar_radiation | 0.0 |
| hour | 10.0 |
| htg_setpoint | 20.0 |
| month | 4.0 |
| outdoor_humidity | 82.0 |
| outdoor_temperature | 6.5257034 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 756883.25 |
| wind_direction | 270.0 |
| wind_speed | 8.8 |
| rollout/ | |
| exploration_rate | 0.42 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 31%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 367.27585 |
| air_humidity | 36.124363 |
| air_temperature | 25.418484 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 137.5 |
| direct_solar_radiation | 828.0 |
| hour | 11.0 |
| htg_setpoint | 18.0 |
| month | 4.0 |
| outdoor_humidity | 55.0 |
| outdoor_temperature | 15.597458 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 330548.9 |
| wind_direction | 180.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.414 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 31%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 232.33136 |
| air_humidity | 44.826347 |
| air_temperature | 23.998606 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 248.5 |
| direct_solar_radiation | 0.0 |
| hour | 12.0 |
| htg_setpoint | 21.0 |
| month | 4.0 |
| outdoor_humidity | 86.0 |
| outdoor_temperature | 12.815099 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 265317.2 |
| wind_direction | 70.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.409 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 31%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 3492.3127 |
| air_humidity | 38.76386 |
| air_temperature | 23.820107 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 212.5 |
| direct_solar_radiation | 553.5 |
| hour | 13.0 |
| htg_setpoint | 15.0 |
| month | 4.0 |
| outdoor_humidity | 33.0 |
| outdoor_temperature | 21.964018 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 3135141.2 |
| wind_direction | 0.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.404 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 32%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 788.53796 |
| air_humidity | 43.409836 |
| air_temperature | 25.469513 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 270.0 |
| direct_solar_radiation | 442.5 |
| hour | 14.0 |
| htg_setpoint | 18.0 |
| month | 4.0 |
| outdoor_humidity | 60.0 |
| outdoor_temperature | 17.625221 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 708291.3 |
| wind_direction | 190.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.398 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 32%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 59.083458 |
| air_temperature | 24.928692 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 155.0 |
| direct_solar_radiation | 542.5 |
| hour | 15.0 |
| htg_setpoint | 16.0 |
| month | 4.0 |
| outdoor_humidity | 78.0 |
| outdoor_temperature | 16.636791 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.393 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 32%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 58.75574 |
| air_temperature | 25.17046 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 99.5 |
| direct_solar_radiation | 80.5 |
| hour | 16.0 |
| htg_setpoint | 21.0 |
| month | 4.0 |
| outdoor_humidity | 59.0 |
| outdoor_temperature | 11.623688 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 110.0 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.387 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 32%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 41.491833 |
| air_temperature | 27.845036 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 29.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 16.0 |
| month | 4.0 |
| outdoor_humidity | 83.0 |
| outdoor_temperature | 11.431208 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 140.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.382 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 33%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 54.379395 |
| air_temperature | 27.763325 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 21.0 |
| month | 4.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | 13.161691 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 60.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.377 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 33%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 36.681477 |
| air_temperature | 27.59846 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 18.0 |
| month | 5.0 |
| outdoor_humidity | 52.0 |
| outdoor_temperature | 11.398606 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 80.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.371 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 33%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 44.83205 |
| air_temperature | 26.145975 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 20.0 |
| month | 5.0 |
| outdoor_humidity | 63.0 |
| outdoor_temperature | 12.721945 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 0.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.366 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 34%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 57.085587 |
| air_temperature | 23.336391 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 19.0 |
| month | 5.0 |
| outdoor_humidity | 60.0 |
| outdoor_temperature | 12.094041 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 20.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.36 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 34%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 64.2879 |
| air_temperature | 21.366455 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 22.0 |
| month | 5.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | 13.429308 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 40.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.355 |
-----------------------------------------------
Progress: |**********************************-----------------------------------------------------------------| 34%-----------------------------------------------
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 60.754135 |
| air_temperature | 22.324484 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 17.0 |
| month | 5.0 |
| outdoor_humidity | 89.0 |
| outdoor_temperature | 13.399098 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 120.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.349 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 35%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 80.84467 |
| air_temperature | 21.444046 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 21.0 |
| month | 5.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 11.571168 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 90.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.344 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 35%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 94.38619 |
| air_temperature | 21.133598 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 22.0 |
| month | 5.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 14.183125 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 110.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.339 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 35%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 100.0 |
| air_temperature | 20.961252 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 18.0 |
| month | 5.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 12.913214 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 260.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.333 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 35%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 82.48104 |
| air_temperature | 21.436998 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 20.0 |
| month | 5.0 |
| outdoor_humidity | 64.0 |
| outdoor_temperature | 12.122469 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 320.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.328 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 36%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 68.75677 |
| air_temperature | 21.790524 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 33.0 |
| direct_solar_radiation | 23.5 |
| hour | 4.0 |
| htg_setpoint | 17.0 |
| month | 5.0 |
| outdoor_humidity | 71.0 |
| outdoor_temperature | 8.485458 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 30.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.322 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 36%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 72.56549 |
| air_temperature | 20.831415 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 95.0 |
| direct_solar_radiation | 255.5 |
| hour | 5.0 |
| htg_setpoint | 15.0 |
| month | 5.0 |
| outdoor_humidity | 83.0 |
| outdoor_temperature | 10.341748 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 20.0 |
| wind_speed | 1.5 |
| rollout/ | |
| exploration_rate | 0.317 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 36%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 74.24994 |
| air_temperature | 20.37937 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 153.0 |
| direct_solar_radiation | 3.0 |
| hour | 6.0 |
| htg_setpoint | 16.0 |
| month | 5.0 |
| outdoor_humidity | 50.0 |
| outdoor_temperature | 10.105156 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 40.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.311 |
-----------------------------------------------
-----------------------------------------------*--------------------------------------------------------------| 37%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 103.51785 |
| air_humidity | 53.202087 |
| air_temperature | 20.09997 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 178.0 |
| direct_solar_radiation | 588.0 |
| hour | 7.0 |
| htg_setpoint | 19.0 |
| month | 5.0 |
| outdoor_humidity | 52.0 |
| outdoor_temperature | 14.155286 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 93643.08 |
| wind_direction | 70.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.306 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 37%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 1136.2172 |
| air_humidity | 42.1363 |
| air_temperature | 23.410343 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 200.0 |
| direct_solar_radiation | 670.0 |
| hour | 8.0 |
| htg_setpoint | 21.0 |
| month | 5.0 |
| outdoor_humidity | 37.0 |
| outdoor_temperature | 18.402655 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1022595.44 |
| wind_direction | 70.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.301 |
------------------------------------------------
-----------------------------------------------*--------------------------------------------------------------| 37%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 3130.67 |
| air_humidity | 48.369793 |
| air_temperature | 23.556591 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 374.0 |
| direct_solar_radiation | 341.0 |
| hour | 9.0 |
| htg_setpoint | 20.0 |
| month | 5.0 |
| outdoor_humidity | 48.0 |
| outdoor_temperature | 19.423044 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 2817603.0 |
| wind_direction | 120.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.295 |
-----------------------------------------------
-----------------------------------------------*--------------------------------------------------------------| 37%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 3276.524 |
| air_humidity | 53.28928 |
| air_temperature | 23.850237 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 279.0 |
| direct_solar_radiation | 649.5 |
| hour | 10.0 |
| htg_setpoint | 19.0 |
| month | 5.0 |
| outdoor_humidity | 55.0 |
| outdoor_temperature | 20.656189 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 2948871.5 |
| wind_direction | 100.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.29 |
-----------------------------------------------
-----------------------------------------------**-------------------------------------------------------------| 38%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 69.13058 |
| air_temperature | 24.301405 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 234.0 |
| direct_solar_radiation | 3.0 |
| hour | 11.0 |
| htg_setpoint | 15.0 |
| month | 5.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 18.272463 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 60.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.284 |
-----------------------------------------------
-----------------------------------------------**-------------------------------------------------------------| 38%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 74.40799 |
| air_temperature | 22.965963 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 281.0 |
| direct_solar_radiation | 9.0 |
| hour | 12.0 |
| htg_setpoint | 15.0 |
| month | 5.0 |
| outdoor_humidity | 90.0 |
| outdoor_temperature | 18.917852 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 140.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.279 |
-----------------------------------------------
-----------------------------------------------**-------------------------------------------------------------| 38%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 3669.714 |
| air_humidity | 39.210167 |
| air_temperature | 23.827646 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 208.5 |
| direct_solar_radiation | 706.5 |
| hour | 13.0 |
| htg_setpoint | 18.0 |
| month | 5.0 |
| outdoor_humidity | 34.0 |
| outdoor_temperature | 23.476068 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 3295098.5 |
| wind_direction | 330.0 |
| wind_speed | 7.7 |
| rollout/ | |
| exploration_rate | 0.273 |
-----------------------------------------------
-----------------------------------------------**-------------------------------------------------------------| 38%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 4127.629 |
| air_humidity | 40.88415 |
| air_temperature | 24.211084 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 239.0 |
| direct_solar_radiation | 528.5 |
| hour | 14.0 |
| htg_setpoint | 21.0 |
| month | 5.0 |
| outdoor_humidity | 44.0 |
| outdoor_temperature | 20.875473 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 3716403.5 |
| wind_direction | 210.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.268 |
-----------------------------------------------
-----------------------------------------------***------------------------------------------------------------| 39%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 3420.548 |
| air_humidity | 45.426456 |
| air_temperature | 23.956959 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 266.0 |
| direct_solar_radiation | 88.5 |
| hour | 15.0 |
| htg_setpoint | 17.0 |
| month | 5.0 |
| outdoor_humidity | 50.0 |
| outdoor_temperature | 19.384127 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 3078493.5 |
| wind_direction | 150.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.263 |
-----------------------------------------------
-----------------------------------------------***------------------------------------------------------------| 39%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 1579.4819 |
| air_humidity | 59.7493 |
| air_temperature | 23.366064 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 188.5 |
| direct_solar_radiation | 101.0 |
| hour | 16.0 |
| htg_setpoint | 22.0 |
| month | 5.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 15.36043 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1421501.6 |
| wind_direction | 120.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.257 |
-----------------------------------------------
-----------------------------------------------***------------------------------------------------------------| 39%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 55.233074 |
| air_temperature | 28.15829 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 39.5 |
| direct_solar_radiation | 34.5 |
| hour | 17.0 |
| htg_setpoint | 17.0 |
| month | 5.0 |
| outdoor_humidity | 97.0 |
| outdoor_temperature | 16.08662 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 230.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.252 |
-----------------------------------------------
-----------------------------------------------****-----------------------------------------------------------| 40%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 73.489456 |
| air_temperature | 25.69325 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 18.0 |
| direct_solar_radiation | 88.5 |
| hour | 18.0 |
| htg_setpoint | 19.0 |
| month | 5.0 |
| outdoor_humidity | 34.0 |
| outdoor_temperature | 20.846014 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 280.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.246 |
-----------------------------------------------
-----------------------------------------------****-----------------------------------------------------------| 40%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 76.32439 |
| air_temperature | 25.073156 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 20.0 |
| month | 5.0 |
| outdoor_humidity | 48.0 |
| outdoor_temperature | 17.44568 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 320.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.241 |
-----------------------------------------------
-----------------------------------------------****-----------------------------------------------------------| 40%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 47.648335 |
| air_temperature | 26.774912 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 17.0 |
| month | 5.0 |
| outdoor_humidity | 32.0 |
| outdoor_temperature | 19.110273 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 320.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.236 |
-----------------------------------------------
-----------------------------------------------****-----------------------------------------------------------| 40%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 69.04204 |
| air_temperature | 25.350838 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 22.0 |
| month | 5.0 |
| outdoor_humidity | 78.0 |
| outdoor_temperature | 18.403831 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.23 |
-----------------------------------------------
-----------------------------------------------*****----------------------------------------------------------| 41%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 73.97231 |
| air_temperature | 24.641607 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 18.0 |
| month | 5.0 |
| outdoor_humidity | 63.0 |
| outdoor_temperature | 22.064882 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.225 |
-----------------------------------------------
Progress: |*****************************************----------------------------------------------------------| 41%-----------------------------------------------
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 69.824036 |
| air_temperature | 23.922873 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 15.0 |
| month | 5.0 |
| outdoor_humidity | 78.0 |
| outdoor_temperature | 18.419962 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.219 |
-----------------------------------------------
-----------------------------------------------*****----------------------------------------------------------| 41%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 75.50254 |
| air_temperature | 23.970839 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 20.0 |
| month | 6.0 |
| outdoor_humidity | 83.0 |
| outdoor_temperature | 18.211943 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 210.0 |
| wind_speed | 3.3 |
| rollout/ | |
| exploration_rate | 0.214 |
-----------------------------------------------
-----------------------------------------------******---------------------------------------------------------| 42%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 76.16311 |
| air_temperature | 23.825941 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 21.0 |
| month | 6.0 |
| outdoor_humidity | 48.0 |
| outdoor_temperature | 14.515478 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 350.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.208 |
-----------------------------------------------
-----------------------------------------------******---------------------------------------------------------| 42%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 88.36912 |
| air_temperature | 21.474377 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 20.0 |
| month | 6.0 |
| outdoor_humidity | 60.0 |
| outdoor_temperature | 12.512131 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 320.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.203 |
-----------------------------------------------
-----------------------------------------------******---------------------------------------------------------| 42%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 82.39349 |
| air_temperature | 21.8717 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 21.0 |
| month | 6.0 |
| outdoor_humidity | 44.0 |
| outdoor_temperature | 16.210348 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 290.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.198 |
-----------------------------------------------
-----------------------------------------------*******--------------------------------------------------------| 43%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 75.15875 |
| air_temperature | 21.211725 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 30.5 |
| direct_solar_radiation | 127.0 |
| hour | 4.0 |
| htg_setpoint | 22.0 |
| month | 6.0 |
| outdoor_humidity | 70.0 |
| outdoor_temperature | 14.304934 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 170.0 |
| wind_speed | 1.5 |
| rollout/ | |
| exploration_rate | 0.192 |
-----------------------------------------------
-----------------------------------------------*******--------------------------------------------------------| 43%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 91.285484 |
| air_temperature | 21.456501 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 131.0 |
| direct_solar_radiation | 120.5 |
| hour | 5.0 |
| htg_setpoint | 22.0 |
| month | 6.0 |
| outdoor_humidity | 84.0 |
| outdoor_temperature | 18.877153 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 180.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.187 |
-----------------------------------------------
-----------------------------------------------*******--------------------------------------------------------| 43%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 97.047325 |
| air_temperature | 20.787859 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 153.0 |
| direct_solar_radiation | 12.0 |
| hour | 6.0 |
| htg_setpoint | 20.0 |
| month | 6.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 16.614325 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 210.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.181 |
-----------------------------------------------
-----------------------------------------------*******--------------------------------------------------------| 43%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 83.41702 |
| air_temperature | 23.228634 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 185.5 |
| direct_solar_radiation | 0.0 |
| hour | 7.0 |
| htg_setpoint | 20.0 |
| month | 6.0 |
| outdoor_humidity | 59.0 |
| outdoor_temperature | 23.758158 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 350.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.176 |
-----------------------------------------------
-----------------------------------------------********-------------------------------------------------------| 44%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 79.8534 |
| air_temperature | 24.084793 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 215.0 |
| direct_solar_radiation | 632.5 |
| hour | 8.0 |
| htg_setpoint | 22.0 |
| month | 6.0 |
| outdoor_humidity | 42.0 |
| outdoor_temperature | 20.098276 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 340.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.17 |
-----------------------------------------------
-----------------------------------------------********-------------------------------------------------------| 44%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 3718.872 |
| air_humidity | 53.877106 |
| air_temperature | 23.519888 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 368.0 |
| direct_solar_radiation | 470.0 |
| hour | 9.0 |
| htg_setpoint | 15.0 |
| month | 6.0 |
| outdoor_humidity | 37.0 |
| outdoor_temperature | 24.126413 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 3319559.5 |
| wind_direction | 70.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.165 |
-----------------------------------------------
-----------------------------------------------********-------------------------------------------------------| 44%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 3689.9016 |
| air_humidity | 59.44847 |
| air_temperature | 23.61409 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 324.0 |
| direct_solar_radiation | 150.5 |
| hour | 10.0 |
| htg_setpoint | 16.0 |
| month | 6.0 |
| outdoor_humidity | 63.0 |
| outdoor_temperature | 21.86438 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 3320911.5 |
| wind_direction | 140.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.16 |
-----------------------------------------------
-----------------------------------------------********-------------------------------------------------------| 44%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 4656.9316 |
| air_humidity | 60.589455 |
| air_temperature | 23.196474 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 285.5 |
| direct_solar_radiation | 137.5 |
| hour | 11.0 |
| htg_setpoint | 22.0 |
| month | 6.0 |
| outdoor_humidity | 79.0 |
| outdoor_temperature | 21.750145 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 4191238.2 |
| wind_direction | 180.0 |
| wind_speed | 8.2 |
| rollout/ | |
| exploration_rate | 0.154 |
-----------------------------------------------
-----------------------------------------------*********------------------------------------------------------| 45%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 3025.9248 |
| air_humidity | 59.557205 |
| air_temperature | 23.235693 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 258.0 |
| direct_solar_radiation | 688.0 |
| hour | 12.0 |
| htg_setpoint | 20.0 |
| month | 6.0 |
| outdoor_humidity | 79.0 |
| outdoor_temperature | 23.093733 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 2717327.2 |
| wind_direction | 180.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.149 |
-----------------------------------------------
-----------------------------------------------*********------------------------------------------------------| 45%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 5699.271 |
| air_humidity | 58.27975 |
| air_temperature | 23.849758 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 248.0 |
| direct_solar_radiation | 632.5 |
| hour | 13.0 |
| htg_setpoint | 21.0 |
| month | 6.0 |
| outdoor_humidity | 79.0 |
| outdoor_temperature | 22.49144 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 5129490.5 |
| wind_direction | 190.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.143 |
-----------------------------------------------
-----------------------------------------------*********------------------------------------------------------| 45%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 63.614998 |
| air_temperature | 28.336887 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 297.0 |
| direct_solar_radiation | 313.0 |
| hour | 14.0 |
| htg_setpoint | 21.0 |
| month | 6.0 |
| outdoor_humidity | 69.0 |
| outdoor_temperature | 25.138285 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 180.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.138 |
-----------------------------------------------
-----------------------------------------------**********-----------------------------------------------------| 46%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 71.799965 |
| air_temperature | 26.287437 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 130.0 |
| direct_solar_radiation | 629.5 |
| hour | 15.0 |
| htg_setpoint | 21.0 |
| month | 6.0 |
| outdoor_humidity | 69.0 |
| outdoor_temperature | 25.576597 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 160.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.133 |
-----------------------------------------------
-----------------------------------------------**********-----------------------------------------------------| 46%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 6111.2476 |
| air_humidity | 59.461708 |
| air_temperature | 23.92439 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 137.5 |
| direct_solar_radiation | 411.0 |
| hour | 16.0 |
| htg_setpoint | 20.0 |
| month | 6.0 |
| outdoor_humidity | 81.0 |
| outdoor_temperature | 23.203123 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 5500122.5 |
| wind_direction | 190.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.127 |
-----------------------------------------------
-----------------------------------------------**********-----------------------------------------------------| 46%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 48.99368 |
| air_temperature | 29.967133 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 77.5 |
| direct_solar_radiation | 232.0 |
| hour | 17.0 |
| htg_setpoint | 21.0 |
| month | 6.0 |
| outdoor_humidity | 85.0 |
| outdoor_temperature | 21.989483 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 210.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.122 |
-----------------------------------------------
-----------------------------------------------**********-----------------------------------------------------| 46%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 54.70692 |
| air_temperature | 31.041197 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 25.5 |
| direct_solar_radiation | 49.0 |
| hour | 18.0 |
| htg_setpoint | 19.0 |
| month | 6.0 |
| outdoor_humidity | 45.0 |
| outdoor_temperature | 33.851494 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 50.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.116 |
-----------------------------------------------
----------------------------------------------************----------------------------------------------------| 47%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 57.80717 |
| air_temperature | 29.11506 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 17.0 |
| month | 6.0 |
| outdoor_humidity | 66.0 |
| outdoor_temperature | 22.44267 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 150.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.111 |
----------------------------------------------
-----------------------------------------------***********----------------------------------------------------| 47%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 69.88379 |
| air_temperature | 26.209824 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 18.0 |
| month | 6.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 22.141724 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 210.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.105 |
-----------------------------------------------
-----------------------------------------------***********----------------------------------------------------| 47%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 67.0384 |
| air_temperature | 26.988644 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 15.0 |
| month | 6.0 |
| outdoor_humidity | 39.0 |
| outdoor_temperature | 25.799631 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 320.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.1 |
-----------------------------------------------
-----------------------------------------------***********----------------------------------------------------| 47%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 71.74893 |
| air_temperature | 25.803793 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 21.0 |
| month | 6.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 22.252745 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 210.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.0945 |
-----------------------------------------------
Progress: |************************************************---------------------------------------------------| 48%-----------------------------------------------
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 82.792534 |
| air_temperature | 23.821617 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 20.0 |
| month | 6.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 23.262617 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 80.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.0891 |
-----------------------------------------------
-----------------------------------------------************---------------------------------------------------| 48%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 79.07156 |
| air_temperature | 24.140497 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 17.0 |
| month | 6.0 |
| outdoor_humidity | 59.0 |
| outdoor_temperature | 23.70243 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 280.0 |
| wind_speed | 7.7 |
| rollout/ | |
| exploration_rate | 0.0837 |
-----------------------------------------------
-----------------------------------------------*************--------------------------------------------------| 49%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 80.9876 |
| air_temperature | 23.416883 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 17.0 |
| month | 6.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 21.679785 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 200.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.0783 |
-----------------------------------------------
-----------------------------------------------*************--------------------------------------------------| 49%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 89.26148 |
| air_temperature | 22.31012 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 18.0 |
| month | 6.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 18.627705 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.0729 |
-----------------------------------------------
-----------------------------------------------*************--------------------------------------------------| 49%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 84.31166 |
| air_temperature | 23.167301 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 22.0 |
| month | 6.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 19.406437 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 120.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.0674 |
-----------------------------------------------
-----------------------------------------------*************--------------------------------------------------| 49%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 86.98578 |
| air_temperature | 22.602865 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 27.5 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 18.0 |
| month | 6.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | 19.922998 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 210.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.062 |
-----------------------------------------------
-----------------------------------------------**************-------------------------------------------------| 50%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 90.4189 |
| air_temperature | 22.064188 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 66.0 |
| direct_solar_radiation | 387.5 |
| hour | 5.0 |
| htg_setpoint | 15.0 |
| month | 7.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 20.899763 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 290.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.0566 |
-----------------------------------------------
-----------------------------------------------**************-------------------------------------------------| 50%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 84.49839 |
| air_temperature | 22.61911 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 89.5 |
| direct_solar_radiation | 616.5 |
| hour | 6.0 |
| htg_setpoint | 21.0 |
| month | 7.0 |
| outdoor_humidity | 81.0 |
| outdoor_temperature | 23.602169 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 1.5 |
| rollout/ | |
| exploration_rate | 0.0512 |
-----------------------------------------------
-----------------------------------------------**************-------------------------------------------------| 50%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 2481.0112 |
| air_humidity | 78.070724 |
| air_temperature | 21.226805 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 281.5 |
| direct_solar_radiation | 33.0 |
| hour | 7.0 |
| htg_setpoint | 18.0 |
| month | 7.0 |
| outdoor_humidity | 64.0 |
| outdoor_temperature | 27.402737 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2232910.0 |
| wind_direction | 0.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------**************-------------------------------------------------| 50%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 5305.9785 |
| air_humidity | 64.83157 |
| air_temperature | 22.350382 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 343.5 |
| direct_solar_radiation | 389.0 |
| hour | 8.0 |
| htg_setpoint | 20.0 |
| month | 7.0 |
| outdoor_humidity | 67.0 |
| outdoor_temperature | 25.031082 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 4764079.0 |
| wind_direction | 70.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------***************------------------------------------------------| 51%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 6145.438 |
| air_humidity | 62.26124 |
| air_temperature | 23.057213 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 316.0 |
| direct_solar_radiation | 322.5 |
| hour | 9.0 |
| htg_setpoint | 21.0 |
| month | 7.0 |
| outdoor_humidity | 77.0 |
| outdoor_temperature | 24.743015 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 5510481.5 |
| wind_direction | 140.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------***************------------------------------------------------| 51%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 68.91196 |
| air_temperature | 26.389618 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 282.5 |
| direct_solar_radiation | 624.5 |
| hour | 10.0 |
| htg_setpoint | 18.0 |
| month | 7.0 |
| outdoor_humidity | 67.0 |
| outdoor_temperature | 30.014492 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 220.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------***************------------------------------------------------| 51%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 65.50601 |
| air_temperature | 27.251705 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 331.0 |
| direct_solar_radiation | 570.0 |
| hour | 11.0 |
| htg_setpoint | 16.0 |
| month | 7.0 |
| outdoor_humidity | 41.0 |
| outdoor_temperature | 35.047768 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 250.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------***************------------------------------------------------| 51%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 9042.422 |
| air_humidity | 57.82255 |
| air_temperature | 24.51498 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 351.5 |
| direct_solar_radiation | 212.0 |
| hour | 12.0 |
| htg_setpoint | 22.0 |
| month | 7.0 |
| outdoor_humidity | 66.0 |
| outdoor_temperature | 30.24039 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 8140713.5 |
| wind_direction | 190.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------****************-----------------------------------------------| 52%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 8990.244 |
| air_humidity | 59.047405 |
| air_temperature | 24.920425 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 298.5 |
| direct_solar_radiation | 578.5 |
| hour | 13.0 |
| htg_setpoint | 15.0 |
| month | 7.0 |
| outdoor_humidity | 57.0 |
| outdoor_temperature | 32.450413 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 8086992.0 |
| wind_direction | 170.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------****************-----------------------------------------------| 52%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 5874.5728 |
| air_humidity | 56.598827 |
| air_temperature | 24.524734 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 267.0 |
| direct_solar_radiation | 392.5 |
| hour | 14.0 |
| htg_setpoint | 20.0 |
| month | 7.0 |
| outdoor_humidity | 33.0 |
| outdoor_temperature | 30.972729 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 5269392.5 |
| wind_direction | 270.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------****************-----------------------------------------------| 52%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 5402.8213 |
| air_humidity | 52.59891 |
| air_temperature | 24.395102 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 202.0 |
| direct_solar_radiation | 505.0 |
| hour | 15.0 |
| htg_setpoint | 21.0 |
| month | 7.0 |
| outdoor_humidity | 32.0 |
| outdoor_temperature | 28.396152 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 4856598.5 |
| wind_direction | 30.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*****************----------------------------------------------| 53%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 5825.845 |
| air_humidity | 58.718395 |
| air_temperature | 24.014736 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 73.5 |
| direct_solar_radiation | 665.0 |
| hour | 16.0 |
| htg_setpoint | 20.0 |
| month | 7.0 |
| outdoor_humidity | 58.0 |
| outdoor_temperature | 25.856668 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 5243260.5 |
| wind_direction | 200.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*****************----------------------------------------------| 53%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 53.448227 |
| air_temperature | 29.858162 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 84.0 |
| direct_solar_radiation | 214.0 |
| hour | 17.0 |
| htg_setpoint | 21.0 |
| month | 7.0 |
| outdoor_humidity | 72.0 |
| outdoor_temperature | 27.553564 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*****************----------------------------------------------| 53%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 64.39038 |
| air_temperature | 26.706186 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 12.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 21.0 |
| month | 7.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 22.227594 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 90.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*****************----------------------------------------------| 53%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 59.30035 |
| air_temperature | 28.517382 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 21.0 |
| month | 7.0 |
| outdoor_humidity | 82.0 |
| outdoor_temperature | 22.560928 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 160.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------******************---------------------------------------------| 54%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 68.19401 |
| air_temperature | 27.295546 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 19.0 |
| month | 7.0 |
| outdoor_humidity | 51.0 |
| outdoor_temperature | 27.625349 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 10.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------******************---------------------------------------------| 54%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 72.78848 |
| air_temperature | 26.51976 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 16.0 |
| month | 7.0 |
| outdoor_humidity | 79.0 |
| outdoor_temperature | 25.196743 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 160.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------******************---------------------------------------------| 54%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 79.561935 |
| air_temperature | 24.249304 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 21.0 |
| month | 7.0 |
| outdoor_humidity | 91.0 |
| outdoor_temperature | 21.584465 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 170.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
Progress: |*******************************************************--------------------------------------------| 55%-----------------------------------------------
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 77.58174 |
| air_temperature | 24.539743 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 18.0 |
| month | 7.0 |
| outdoor_humidity | 94.0 |
| outdoor_temperature | 26.045515 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 200.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*******************--------------------------------------------| 55%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 73.604805 |
| air_temperature | 25.405827 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 19.0 |
| month | 7.0 |
| outdoor_humidity | 85.0 |
| outdoor_temperature | 24.540695 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 230.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*******************--------------------------------------------| 55%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 77.42579 |
| air_temperature | 24.458406 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 21.0 |
| month | 7.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 25.382166 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 9.3 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 56%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 84.15275 |
| air_temperature | 23.566076 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 22.0 |
| month | 7.0 |
| outdoor_humidity | 94.0 |
| outdoor_temperature | 24.34201 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 200.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 56%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 86.7414 |
| air_temperature | 22.618448 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 17.0 |
| month | 7.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 21.542467 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 180.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 56%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 81.86973 |
| air_temperature | 23.105227 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 23.0 |
| direct_solar_radiation | 7.5 |
| hour | 4.0 |
| htg_setpoint | 21.0 |
| month | 7.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 25.863367 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 260.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 56%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 91.777534 |
| air_temperature | 22.225996 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 66.0 |
| direct_solar_radiation | 21.5 |
| hour | 5.0 |
| htg_setpoint | 22.0 |
| month | 7.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | 20.798347 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 200.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 57%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 85.82952 |
| air_temperature | 23.096634 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 102.5 |
| direct_solar_radiation | 235.5 |
| hour | 6.0 |
| htg_setpoint | 17.0 |
| month | 7.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | 21.932587 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 0.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 57%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 80.898224 |
| air_temperature | 24.078562 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 157.0 |
| direct_solar_radiation | 0.0 |
| hour | 7.0 |
| htg_setpoint | 22.0 |
| month | 7.0 |
| outdoor_humidity | 90.0 |
| outdoor_temperature | 23.581535 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 57%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 4853.2866 |
| air_humidity | 64.223564 |
| air_temperature | 22.301798 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 246.0 |
| direct_solar_radiation | 103.5 |
| hour | 8.0 |
| htg_setpoint | 19.0 |
| month | 7.0 |
| outdoor_humidity | 62.0 |
| outdoor_temperature | 25.080772 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 4367958.0 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 57%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 7106.1353 |
| air_humidity | 61.67097 |
| air_temperature | 23.269861 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 352.5 |
| direct_solar_radiation | 310.0 |
| hour | 9.0 |
| htg_setpoint | 18.0 |
| month | 7.0 |
| outdoor_humidity | 82.0 |
| outdoor_temperature | 25.929888 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 6367651.5 |
| wind_direction | 190.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*********************------------------------------------------| 58%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 5841.152 |
| air_humidity | 60.543064 |
| air_temperature | 23.566633 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 416.5 |
| direct_solar_radiation | 66.0 |
| hour | 10.0 |
| htg_setpoint | 20.0 |
| month | 7.0 |
| outdoor_humidity | 62.0 |
| outdoor_temperature | 26.519234 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 5209345.0 |
| wind_direction | 40.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*********************------------------------------------------| 58%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 6789.402 |
| air_humidity | 57.23053 |
| air_temperature | 24.330137 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 197.0 |
| direct_solar_radiation | 784.0 |
| hour | 11.0 |
| htg_setpoint | 19.0 |
| month | 8.0 |
| outdoor_humidity | 44.0 |
| outdoor_temperature | 31.489174 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 6164890.0 |
| wind_direction | 230.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*********************------------------------------------------| 58%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 9290.521 |
| air_humidity | 57.967903 |
| air_temperature | 24.993517 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 184.5 |
| direct_solar_radiation | 711.0 |
| hour | 12.0 |
| htg_setpoint | 20.0 |
| month | 8.0 |
| outdoor_humidity | 72.0 |
| outdoor_temperature | 31.004633 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 8339602.5 |
| wind_direction | 190.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------***********************----------------------------------------| 59%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 68.39203 |
| air_temperature | 28.487068 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 298.0 |
| direct_solar_radiation | 500.0 |
| hour | 13.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 72.0 |
| outdoor_temperature | 30.68283 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------***********************----------------------------------------| 59%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 66.07435 |
| air_temperature | 28.946291 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 244.0 |
| direct_solar_radiation | 481.5 |
| hour | 14.0 |
| htg_setpoint | 18.0 |
| month | 8.0 |
| outdoor_humidity | 63.0 |
| outdoor_temperature | 30.498264 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 210.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------***********************----------------------------------------| 59%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 9002.211 |
| air_humidity | 60.445164 |
| air_temperature | 24.583647 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 151.5 |
| direct_solar_radiation | 0.0 |
| hour | 15.0 |
| htg_setpoint | 22.0 |
| month | 8.0 |
| outdoor_humidity | 88.0 |
| outdoor_temperature | 26.536777 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 8101990.0 |
| wind_direction | 120.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------***********************----------------------------------------| 59%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 4601.3066 |
| air_humidity | 61.324406 |
| air_temperature | 23.162462 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 80.5 |
| direct_solar_radiation | 0.0 |
| hour | 16.0 |
| htg_setpoint | 16.0 |
| month | 8.0 |
| outdoor_humidity | 84.0 |
| outdoor_temperature | 21.187864 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 4141176.0 |
| wind_direction | 30.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------************************---------------------------------------| 60%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 48.871037 |
| air_temperature | 29.925226 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 57.0 |
| direct_solar_radiation | 228.5 |
| hour | 17.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 47.0 |
| outdoor_temperature | 26.071585 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 70.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------************************---------------------------------------| 60%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 53.308235 |
| air_temperature | 29.915752 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 16.0 |
| month | 8.0 |
| outdoor_humidity | 61.0 |
| outdoor_temperature | 21.503227 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 120.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------************************---------------------------------------| 60%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 56.636124 |
| air_temperature | 28.742277 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 20.0 |
| month | 8.0 |
| outdoor_humidity | 66.0 |
| outdoor_temperature | 22.733294 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------************************---------------------------------------| 60%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 63.47045 |
| air_temperature | 27.229761 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 17.0 |
| month | 8.0 |
| outdoor_humidity | 85.0 |
| outdoor_temperature | 23.311094 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 200.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*************************--------------------------------------| 61%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 66.61175 |
| air_temperature | 26.358007 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 20.0 |
| month | 8.0 |
| outdoor_humidity | 94.0 |
| outdoor_temperature | 23.789915 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*************************--------------------------------------| 61%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 73.53897 |
| air_temperature | 25.65634 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 18.0 |
| month | 8.0 |
| outdoor_humidity | 74.0 |
| outdoor_temperature | 27.896782 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 290.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
Progress: |**************************************************************-------------------------------------| 62%-----------------------------------------------
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 75.148575 |
| air_temperature | 24.968826 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 18.0 |
| month | 8.0 |
| outdoor_humidity | 82.0 |
| outdoor_temperature | 22.955194 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 220.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------**************************-------------------------------------| 62%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 83.31977 |
| air_temperature | 24.230255 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 15.0 |
| month | 8.0 |
| outdoor_humidity | 79.0 |
| outdoor_temperature | 24.522755 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 180.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------**************************-------------------------------------| 62%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 78.130356 |
| air_temperature | 23.756025 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 22.0 |
| month | 8.0 |
| outdoor_humidity | 90.0 |
| outdoor_temperature | 22.456839 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 100.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------**************************-------------------------------------| 62%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 83.35365 |
| air_temperature | 24.506315 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 19.0 |
| month | 8.0 |
| outdoor_humidity | 90.0 |
| outdoor_temperature | 23.943144 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 40.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------***************************------------------------------------| 63%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 80.7912 |
| air_temperature | 24.995941 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 20.0 |
| month | 8.0 |
| outdoor_humidity | 58.0 |
| outdoor_temperature | 26.172163 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 20.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------***************************------------------------------------| 63%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 91.53649 |
| air_temperature | 23.048388 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 70.0 |
| outdoor_temperature | 19.684956 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 40.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------***************************------------------------------------| 63%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 87.73716 |
| air_temperature | 21.66965 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 52.5 |
| direct_solar_radiation | 100.0 |
| hour | 5.0 |
| htg_setpoint | 18.0 |
| month | 8.0 |
| outdoor_humidity | 83.0 |
| outdoor_temperature | 15.233419 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 30.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------***************************------------------------------------| 63%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 80.77063 |
| air_temperature | 22.572788 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 71.5 |
| direct_solar_radiation | 425.0 |
| hour | 6.0 |
| htg_setpoint | 17.0 |
| month | 8.0 |
| outdoor_humidity | 66.0 |
| outdoor_temperature | 23.186699 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 250.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------****************************-----------------------------------| 64%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 2988.8997 |
| air_humidity | 73.81425 |
| air_temperature | 21.999039 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 88.0 |
| direct_solar_radiation | 715.0 |
| hour | 7.0 |
| htg_setpoint | 22.0 |
| month | 8.0 |
| outdoor_humidity | 45.0 |
| outdoor_temperature | 26.786617 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2714391.8 |
| wind_direction | 330.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------****************************-----------------------------------| 64%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 3137.0544 |
| air_humidity | 61.19183 |
| air_temperature | 22.177671 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 253.5 |
| direct_solar_radiation | 445.5 |
| hour | 8.0 |
| htg_setpoint | 15.0 |
| month | 8.0 |
| outdoor_humidity | 48.0 |
| outdoor_temperature | 22.343174 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 2801224.0 |
| wind_direction | 20.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------****************************-----------------------------------| 64%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 72.13367 |
| air_temperature | 24.903711 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 234.0 |
| direct_solar_radiation | 632.5 |
| hour | 9.0 |
| htg_setpoint | 17.0 |
| month | 8.0 |
| outdoor_humidity | 51.0 |
| outdoor_temperature | 26.507423 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 260.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*****************************----------------------------------| 65%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 70.41207 |
| air_temperature | 25.408579 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 209.5 |
| direct_solar_radiation | 729.0 |
| hour | 10.0 |
| htg_setpoint | 18.0 |
| month | 8.0 |
| outdoor_humidity | 34.0 |
| outdoor_temperature | 22.097107 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 0.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*****************************----------------------------------| 65%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 5351.1226 |
| air_humidity | 58.171345 |
| air_temperature | 23.91477 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 214.0 |
| direct_solar_radiation | 561.0 |
| hour | 11.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 52.0 |
| outdoor_temperature | 26.362823 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 4806430.0 |
| wind_direction | 190.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*****************************----------------------------------| 65%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 5359.0513 |
| air_humidity | 56.55467 |
| air_temperature | 24.294718 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 246.0 |
| direct_solar_radiation | 455.5 |
| hour | 12.0 |
| htg_setpoint | 17.0 |
| month | 8.0 |
| outdoor_humidity | 65.0 |
| outdoor_temperature | 30.070414 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 4887073.0 |
| wind_direction | 180.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*****************************----------------------------------| 65%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 3752.6602 |
| air_humidity | 52.818874 |
| air_temperature | 24.058065 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 146.5 |
| direct_solar_radiation | 737.5 |
| hour | 13.0 |
| htg_setpoint | 15.0 |
| month | 8.0 |
| outdoor_humidity | 42.0 |
| outdoor_temperature | 24.97303 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 3369927.5 |
| wind_direction | 110.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------******************************---------------------------------| 66%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 5573.891 |
| air_humidity | 57.449215 |
| air_temperature | 24.29049 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 145.5 |
| direct_solar_radiation | 682.0 |
| hour | 14.0 |
| htg_setpoint | 19.0 |
| month | 8.0 |
| outdoor_humidity | 60.0 |
| outdoor_temperature | 25.14221 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 5003182.5 |
| wind_direction | 190.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------******************************---------------------------------| 66%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 5860.3286 |
| air_humidity | 37.1701 |
| air_temperature | 24.564209 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 114.0 |
| direct_solar_radiation | 581.0 |
| hour | 15.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 20.0 |
| outdoor_temperature | 28.693348 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 5268518.0 |
| wind_direction | 30.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------******************************---------------------------------| 66%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 46.263493 |
| air_temperature | 28.117382 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 57.5 |
| direct_solar_radiation | 492.5 |
| hour | 16.0 |
| htg_setpoint | 22.0 |
| month | 8.0 |
| outdoor_humidity | 76.0 |
| outdoor_temperature | 24.179312 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 200.0 |
| wind_speed | 9.3 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------******************************---------------------------------| 66%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 45.025 |
| air_temperature | 28.550064 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 31.5 |
| direct_solar_radiation | 19.5 |
| hour | 17.0 |
| htg_setpoint | 17.0 |
| month | 9.0 |
| outdoor_humidity | 35.0 |
| outdoor_temperature | 27.793127 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 330.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*******************************--------------------------------| 67%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 54.289627 |
| air_temperature | 29.596655 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 20.0 |
| month | 9.0 |
| outdoor_humidity | 73.0 |
| outdoor_temperature | 22.908802 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*******************************--------------------------------| 67%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 53.948284 |
| air_temperature | 28.350603 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 19.0 |
| month | 9.0 |
| outdoor_humidity | 55.0 |
| outdoor_temperature | 22.92673 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*******************************--------------------------------| 67%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 66.87577 |
| air_temperature | 27.287249 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 20.0 |
| month | 9.0 |
| outdoor_humidity | 64.0 |
| outdoor_temperature | 24.204128 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------********************************-------------------------------| 68%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 72.05659 |
| air_temperature | 25.802189 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 19.0 |
| month | 9.0 |
| outdoor_humidity | 69.0 |
| outdoor_temperature | 23.501991 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 250.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------********************************-------------------------------| 68%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 73.47732 |
| air_temperature | 25.126427 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 22.0 |
| month | 9.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | 22.370691 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 150.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
Progress: |********************************************************************-------------------------------| 68%-----------------------------------------------
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 73.2652 |
| air_temperature | 25.092297 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 18.0 |
| month | 9.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | 21.099207 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 230.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*********************************------------------------------| 69%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 83.47031 |
| air_temperature | 22.970182 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 21.0 |
| month | 9.0 |
| outdoor_humidity | 81.0 |
| outdoor_temperature | 19.91283 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 70.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*********************************------------------------------| 69%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 82.12721 |
| air_temperature | 22.954018 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 22.0 |
| month | 9.0 |
| outdoor_humidity | 75.0 |
| outdoor_temperature | 21.076895 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 340.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*********************************------------------------------| 69%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 70.639404 |
| air_temperature | 21.706398 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 15.0 |
| month | 9.0 |
| outdoor_humidity | 62.0 |
| outdoor_temperature | 12.323276 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 20.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*********************************------------------------------| 69%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 67.0841 |
| air_temperature | 21.694613 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 22.0 |
| month | 9.0 |
| outdoor_humidity | 67.0 |
| outdoor_temperature | 15.121407 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 290.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------**********************************-----------------------------| 70%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 82.34458 |
| air_temperature | 22.672228 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 15.0 |
| month | 9.0 |
| outdoor_humidity | 76.0 |
| outdoor_temperature | 23.33292 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 220.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------**********************************-----------------------------| 70%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 80.37435 |
| air_temperature | 23.098211 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 22.0 |
| direct_solar_radiation | 1.5 |
| hour | 5.0 |
| htg_setpoint | 16.0 |
| month | 9.0 |
| outdoor_humidity | 97.0 |
| outdoor_temperature | 22.74073 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 220.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------**********************************-----------------------------| 70%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 83.09637 |
| air_temperature | 22.759245 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 83.5 |
| direct_solar_radiation | 286.5 |
| hour | 6.0 |
| htg_setpoint | 16.0 |
| month | 9.0 |
| outdoor_humidity | 63.0 |
| outdoor_temperature | 17.67871 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 20.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------***********************************----------------------------| 71%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 1371.7104 |
| air_humidity | 78.25826 |
| air_temperature | 20.039146 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 174.0 |
| direct_solar_radiation | 104.5 |
| hour | 7.0 |
| htg_setpoint | 16.0 |
| month | 9.0 |
| outdoor_humidity | 61.0 |
| outdoor_temperature | 19.290564 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1234998.8 |
| wind_direction | 120.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------***********************************----------------------------| 71%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 4050.8926 |
| air_humidity | 67.34919 |
| air_temperature | 21.52845 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 127.5 |
| direct_solar_radiation | 0.0 |
| hour | 8.0 |
| htg_setpoint | 21.0 |
| month | 9.0 |
| outdoor_humidity | 97.0 |
| outdoor_temperature | 19.215305 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 3645803.2 |
| wind_direction | 50.0 |
| wind_speed | 7.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------***********************************----------------------------| 71%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 3004.9004 |
| air_humidity | 60.246204 |
| air_temperature | 22.964148 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 299.0 |
| direct_solar_radiation | 364.5 |
| hour | 9.0 |
| htg_setpoint | 17.0 |
| month | 9.0 |
| outdoor_humidity | 51.0 |
| outdoor_temperature | 21.560059 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 2684185.5 |
| wind_direction | 0.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------***********************************----------------------------| 71%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 3808.9343 |
| air_humidity | 59.175064 |
| air_temperature | 23.599087 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 426.5 |
| direct_solar_radiation | 111.5 |
| hour | 10.0 |
| htg_setpoint | 18.0 |
| month | 9.0 |
| outdoor_humidity | 65.0 |
| outdoor_temperature | 21.939615 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 3428041.0 |
| wind_direction | 110.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------************************************---------------------------| 72%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 3255.3972 |
| air_humidity | 55.725792 |
| air_temperature | 23.779278 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 262.5 |
| direct_solar_radiation | 437.0 |
| hour | 11.0 |
| htg_setpoint | 15.0 |
| month | 9.0 |
| outdoor_humidity | 49.0 |
| outdoor_temperature | 23.010288 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 2929857.5 |
| wind_direction | 180.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------************************************---------------------------| 72%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 82.00577 |
| air_temperature | 23.490236 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 209.5 |
| direct_solar_radiation | 0.0 |
| hour | 12.0 |
| htg_setpoint | 22.0 |
| month | 9.0 |
| outdoor_humidity | 71.0 |
| outdoor_temperature | 22.610647 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 200.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------************************************---------------------------| 72%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 80.138374 |
| air_temperature | 23.77459 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 160.5 |
| direct_solar_radiation | 0.0 |
| hour | 13.0 |
| htg_setpoint | 15.0 |
| month | 9.0 |
| outdoor_humidity | 94.0 |
| outdoor_temperature | 22.711033 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------************************************---------------------------| 72%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 501.99664 |
| air_humidity | 30.882849 |
| air_temperature | 25.489855 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 178.0 |
| direct_solar_radiation | 0.0 |
| hour | 14.0 |
| htg_setpoint | 15.0 |
| month | 9.0 |
| outdoor_humidity | 42.0 |
| outdoor_temperature | 16.924389 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 451796.97 |
| wind_direction | 0.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*************************************--------------------------| 73%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 1538.3142 |
| air_humidity | 40.38514 |
| air_temperature | 24.152029 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 80.0 |
| direct_solar_radiation | 0.0 |
| hour | 15.0 |
| htg_setpoint | 21.0 |
| month | 9.0 |
| outdoor_humidity | 56.0 |
| outdoor_temperature | 16.750742 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1361756.1 |
| wind_direction | 120.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*************************************--------------------------| 73%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 1562.5536 |
| air_humidity | 57.7763 |
| air_temperature | 23.69666 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 33.0 |
| direct_solar_radiation | 0.0 |
| hour | 16.0 |
| htg_setpoint | 21.0 |
| month | 9.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 15.362766 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1406267.5 |
| wind_direction | 70.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*************************************--------------------------| 73%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 52.56485 |
| air_temperature | 27.943174 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 17.0 |
| month | 9.0 |
| outdoor_humidity | 86.0 |
| outdoor_temperature | 18.228003 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 100.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------**************************************-------------------------| 74%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 56.614223 |
| air_temperature | 29.171944 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 20.0 |
| month | 9.0 |
| outdoor_humidity | 53.0 |
| outdoor_temperature | 19.968481 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 230.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------**************************************-------------------------| 74%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 71.62621 |
| air_temperature | 26.012545 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 22.0 |
| month | 9.0 |
| outdoor_humidity | 41.0 |
| outdoor_temperature | 16.645796 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 30.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------**************************************-------------------------| 74%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 78.65945 |
| air_temperature | 24.503786 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 21.0 |
| month | 9.0 |
| outdoor_humidity | 75.0 |
| outdoor_temperature | 14.65261 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 50.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------**************************************-------------------------| 74%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 67.32206 |
| air_temperature | 24.97646 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 19.0 |
| month | 9.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | 14.896741 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 50.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------***************************************------------------------| 75%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 193.37918 |
| air_humidity | 53.04839 |
| air_temperature | 20.552856 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 18.0 |
| month | 10.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 11.888072 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 170850.98 |
| wind_direction | 250.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
Progress: |***************************************************************************------------------------| 75%-----------------------------------------------
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 91.6472 |
| air_humidity | 62.588314 |
| air_temperature | 21.2672 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 16.0 |
| month | 10.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 14.231259 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 81602.86 |
| wind_direction | 220.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------***************************************------------------------| 75%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 70.04255 |
| air_temperature | 21.505669 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 15.0 |
| month | 10.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | 16.890583 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 220.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------****************************************-----------------------| 76%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 59.37259 |
| air_temperature | 21.999968 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 86.0 |
| outdoor_temperature | 15.308412 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 200.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------****************************************-----------------------| 76%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 61.38226 |
| air_temperature | 23.647644 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | 20.262846 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 180.0 |
| wind_speed | 11.3 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------****************************************-----------------------| 76%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 608.0272 |
| air_humidity | 31.537436 |
| air_temperature | 19.350393 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 18.0 |
| month | 10.0 |
| outdoor_humidity | 63.0 |
| outdoor_temperature | 8.612885 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 518107.1 |
| wind_direction | 290.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*****************************************----------------------| 77%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 21.907684 |
| air_temperature | 18.80182 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 18.0 |
| month | 10.0 |
| outdoor_humidity | 58.0 |
| outdoor_temperature | 4.671904 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 320.0 |
| wind_speed | 7.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*****************************************----------------------| 77%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 24.602402 |
| air_temperature | 19.819515 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 19.0 |
| month | 10.0 |
| outdoor_humidity | 92.0 |
| outdoor_temperature | 2.654228 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*****************************************----------------------| 77%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 244.03078 |
| air_humidity | 34.41275 |
| air_temperature | 20.909618 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 64.0 |
| direct_solar_radiation | 174.0 |
| hour | 6.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 80.0 |
| outdoor_temperature | 8.19851 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 219627.69 |
| wind_direction | 240.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*****************************************----------------------| 77%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 160.70125 |
| air_humidity | 66.53428 |
| air_temperature | 19.79378 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 134.0 |
| direct_solar_radiation | 379.0 |
| hour | 7.0 |
| htg_setpoint | 15.0 |
| month | 10.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 14.136075 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 146586.66 |
| wind_direction | 240.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------******************************************---------------------| 78%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 57.88952 |
| air_temperature | 22.00054 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 125.5 |
| direct_solar_radiation | 694.0 |
| hour | 8.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 81.0 |
| outdoor_temperature | 17.635881 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------******************************************---------------------| 78%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 62.214733 |
| air_temperature | 23.414923 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 241.0 |
| direct_solar_radiation | 416.5 |
| hour | 9.0 |
| htg_setpoint | 17.0 |
| month | 10.0 |
| outdoor_humidity | 73.0 |
| outdoor_temperature | 20.859966 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 150.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------******************************************---------------------| 78%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 192.45067 |
| air_humidity | 57.880604 |
| air_temperature | 26.456223 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 316.5 |
| direct_solar_radiation | 189.5 |
| hour | 10.0 |
| htg_setpoint | 15.0 |
| month | 10.0 |
| outdoor_humidity | 86.0 |
| outdoor_temperature | 17.595821 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 173205.6 |
| wind_direction | 110.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------******************************************---------------------| 78%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 34.765224 |
| air_temperature | 27.755896 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 202.0 |
| direct_solar_radiation | 596.5 |
| hour | 11.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 49.0 |
| outdoor_temperature | 19.118748 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 270.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*******************************************--------------------| 79%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 50.221325 |
| air_temperature | 25.792042 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 256.0 |
| direct_solar_radiation | 306.5 |
| hour | 12.0 |
| htg_setpoint | 20.0 |
| month | 10.0 |
| outdoor_humidity | 73.0 |
| outdoor_temperature | 18.685549 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 120.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*******************************************--------------------| 79%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 29.643288 |
| air_temperature | 25.088284 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 145.5 |
| direct_solar_radiation | 614.5 |
| hour | 13.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 39.0 |
| outdoor_temperature | 16.19097 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 270.0 |
| wind_speed | 11.8 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*******************************************--------------------| 79%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 455.85727 |
| air_humidity | 23.233004 |
| air_temperature | 25.580149 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 105.5 |
| direct_solar_radiation | 518.0 |
| hour | 14.0 |
| htg_setpoint | 19.0 |
| month | 10.0 |
| outdoor_humidity | 32.0 |
| outdoor_temperature | 14.719782 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 410271.56 |
| wind_direction | 300.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------********************************************-------------------| 80%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 32.46782 |
| air_temperature | 23.050169 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 54.5 |
| direct_solar_radiation | 427.0 |
| hour | 15.0 |
| htg_setpoint | 15.0 |
| month | 10.0 |
| outdoor_humidity | 62.0 |
| outdoor_temperature | 15.007018 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 170.0 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------********************************************-------------------| 80%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 104.0049 |
| air_humidity | 40.469254 |
| air_temperature | 25.762215 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 15.5 |
| direct_solar_radiation | 136.5 |
| hour | 16.0 |
| htg_setpoint | 18.0 |
| month | 10.0 |
| outdoor_humidity | 65.0 |
| outdoor_temperature | 16.85201 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 110891.28 |
| wind_direction | 200.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------********************************************-------------------| 80%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 47.767754 |
| air_temperature | 25.61364 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 72.0 |
| outdoor_temperature | 16.869762 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 190.0 |
| wind_speed | 10.8 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------********************************************-------------------| 80%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 109.77352 |
| air_humidity | 62.86594 |
| air_temperature | 25.587751 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 15.0 |
| month | 10.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 16.71503 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 98810.305 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*********************************************------------------| 81%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 72.662445 |
| air_temperature | 22.676569 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 18.0 |
| month | 10.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 17.344183 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 160.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*********************************************------------------| 81%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 71.353714 |
| air_temperature | 24.358383 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 15.0 |
| month | 10.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 18.299417 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 190.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*********************************************------------------| 81%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 18.589087 |
| air_temperature | 22.21219 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 21.0 |
| month | 10.0 |
| outdoor_humidity | 28.0 |
| outdoor_temperature | 16.183949 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 250.0 |
| wind_speed | 7.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*********************************************------------------| 81%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 18.206142 |
| air_temperature | 21.999985 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 48.0 |
| outdoor_temperature | 7.863268 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 270.0 |
| wind_speed | 10.3 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------**********************************************-----------------| 82%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 70.79328 |
| air_humidity | 21.031765 |
| air_temperature | 20.74373 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 15.0 |
| month | 10.0 |
| outdoor_humidity | 50.0 |
| outdoor_temperature | 7.3715158 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 61825.062 |
| wind_direction | 310.0 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------**********************************************-----------------| 82%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 23.970629 |
| air_temperature | 18.405119 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 16.0 |
| month | 10.0 |
| outdoor_humidity | 61.0 |
| outdoor_temperature | 5.1220016 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------***********************************************----------------| 83%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 201.19078 |
| air_humidity | 28.49424 |
| air_temperature | 21.015865 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 47.0 |
| outdoor_temperature | 12.931328 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 188645.92 |
| wind_direction | 280.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------***********************************************----------------| 83%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 948.2007 |
| air_humidity | 20.858418 |
| air_temperature | 19.403952 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 19.0 |
| month | 10.0 |
| outdoor_humidity | 53.0 |
| outdoor_temperature | 4.6075807 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 801069.8 |
| wind_direction | 0.0 |
| wind_speed | 1.5 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------***********************************************----------------| 83%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 41.18096 |
| air_temperature | 18.634413 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 16.0 |
| month | 11.0 |
| outdoor_humidity | 70.0 |
| outdoor_temperature | 4.9571757 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 350.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------***********************************************----------------| 83%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 95.37267 |
| air_humidity | 24.629105 |
| air_temperature | 20.14481 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 18.0 |
| month | 11.0 |
| outdoor_humidity | 62.0 |
| outdoor_temperature | 6.937596 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 82714.27 |
| wind_direction | 340.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------************************************************---------------| 84%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 68.66032 |
| air_humidity | 28.74519 |
| air_temperature | 20.179213 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 19.0 |
| month | 11.0 |
| outdoor_humidity | 71.0 |
| outdoor_temperature | 8.355169 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 61025.4 |
| wind_direction | 350.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------************************************************---------------| 84%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 244.03078 |
| air_humidity | 42.22224 |
| air_temperature | 20.154366 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 34.5 |
| direct_solar_radiation | 136.0 |
| hour | 6.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 86.0 |
| outdoor_temperature | 8.601156 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 219627.69 |
| wind_direction | 330.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------************************************************---------------| 84%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 300.50192 |
| air_humidity | 34.13722 |
| air_temperature | 20.724718 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 91.0 |
| direct_solar_radiation | 372.0 |
| hour | 7.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 68.0 |
| outdoor_temperature | 10.182717 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 260286.92 |
| wind_direction | 310.0 |
| wind_speed | 7.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------************************************************---------------| 84%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 871.7625 |
| air_humidity | 27.324717 |
| air_temperature | 21.460247 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 116.5 |
| direct_solar_radiation | 556.5 |
| hour | 8.0 |
| htg_setpoint | 19.0 |
| month | 11.0 |
| outdoor_humidity | 55.0 |
| outdoor_temperature | 7.6401772 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 784586.25 |
| wind_direction | 0.0 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*************************************************--------------| 85%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 663.8411 |
| air_humidity | 35.611805 |
| air_temperature | 23.279047 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 158.0 |
| direct_solar_radiation | 527.0 |
| hour | 9.0 |
| htg_setpoint | 17.0 |
| month | 11.0 |
| outdoor_humidity | 66.0 |
| outdoor_temperature | 11.253662 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 610932.6 |
| wind_direction | 290.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*************************************************--------------| 85%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 39.466824 |
| air_temperature | 23.828392 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 255.0 |
| direct_solar_radiation | 190.0 |
| hour | 10.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 69.0 |
| outdoor_temperature | 12.27229 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 300.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*************************************************--------------| 85%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 46.755707 |
| air_temperature | 21.837193 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 237.5 |
| direct_solar_radiation | 86.5 |
| hour | 11.0 |
| htg_setpoint | 21.0 |
| month | 11.0 |
| outdoor_humidity | 64.0 |
| outdoor_temperature | 16.700947 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 130.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------**************************************************-------------| 86%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 54.78266 |
| air_temperature | 21.810846 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 189.0 |
| direct_solar_radiation | 15.0 |
| hour | 12.0 |
| htg_setpoint | 18.0 |
| month | 11.0 |
| outdoor_humidity | 75.0 |
| outdoor_temperature | 14.556306 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 270.0 |
| wind_speed | 7.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------**************************************************-------------| 86%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 295.21298 |
| air_humidity | 48.13714 |
| air_temperature | 25.021883 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 194.0 |
| direct_solar_radiation | 123.0 |
| hour | 13.0 |
| htg_setpoint | 16.0 |
| month | 11.0 |
| outdoor_humidity | 84.0 |
| outdoor_temperature | 13.235451 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 266980.47 |
| wind_direction | 350.0 |
| wind_speed | 8.8 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------**************************************************-------------| 86%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 539.43866 |
| air_humidity | 36.041264 |
| air_temperature | 24.243587 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 115.5 |
| direct_solar_radiation | 82.0 |
| hour | 14.0 |
| htg_setpoint | 20.0 |
| month | 11.0 |
| outdoor_humidity | 66.0 |
| outdoor_temperature | 10.984423 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 485494.8 |
| wind_direction | 340.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------**************************************************-------------| 86%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 902.7748 |
| air_humidity | 41.3403 |
| air_temperature | 23.527079 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 38.0 |
| direct_solar_radiation | 53.5 |
| hour | 15.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 83.0 |
| outdoor_temperature | 9.99827 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 812497.3 |
| wind_direction | 150.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------***************************************************------------| 87%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 650.0828 |
| air_humidity | 52.764694 |
| air_temperature | 23.35507 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 16.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 10.372531 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 585074.56 |
| wind_direction | 160.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------***************************************************------------| 87%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 28.211124 |
| air_temperature | 22.976395 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 16.0 |
| month | 11.0 |
| outdoor_humidity | 56.0 |
| outdoor_temperature | 6.600526 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 280.0 |
| wind_speed | 7.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------***************************************************------------| 87%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 27.771719 |
| air_temperature | 20.824842 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 15.0 |
| month | 11.0 |
| outdoor_humidity | 63.0 |
| outdoor_temperature | 9.77587 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 210.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------***************************************************------------| 87%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 27.479923 |
| air_temperature | 20.357563 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 17.0 |
| month | 11.0 |
| outdoor_humidity | 57.0 |
| outdoor_temperature | 6.0292397 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 290.0 |
| wind_speed | 10.3 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------****************************************************-----------| 88%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 727.8344 |
| air_humidity | 25.417734 |
| air_temperature | 21.000147 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 21.0 |
| month | 11.0 |
| outdoor_humidity | 64.0 |
| outdoor_temperature | 5.013974 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 646563.2 |
| wind_direction | 270.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------****************************************************-----------| 88%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 31.97609 |
| air_temperature | 21.428865 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 74.0 |
| outdoor_temperature | 3.8844962 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 250.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------****************************************************-----------| 88%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1003.0152 |
| air_humidity | 36.79652 |
| air_temperature | 21.041609 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 85.0 |
| outdoor_temperature | 1.7418324 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 902713.7 |
| wind_direction | 270.0 |
| wind_speed | 7.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
Progress: |*****************************************************************************************----------| 89%------------------------------------------------
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 1480.0426 |
| air_humidity | 29.446743 |
| air_temperature | 20.312044 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 21.0 |
| month | 11.0 |
| outdoor_humidity | 69.0 |
| outdoor_temperature | -0.6433044 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1332038.4 |
| wind_direction | 270.0 |
| wind_speed | 9.8 |
| rollout/ | |
| exploration_rate | 0.05 |
------------------------------------------------
-----------------------------------------------*****************************************************----------| 89%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 2032.5927 |
| air_humidity | 19.228872 |
| air_temperature | 20.992287 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 59.0 |
| outdoor_temperature | -3.406055 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1829333.4 |
| wind_direction | 290.0 |
| wind_speed | 9.3 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
------------------------------------------------****************************************************----------| 89%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1643.0013 |
| air_humidity | 15.219239 |
| air_temperature | 19.81402 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 16.0 |
| month | 11.0 |
| outdoor_humidity | 51.0 |
| outdoor_temperature | -1.4580983 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1478701.2 |
| wind_direction | 340.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
------------------------------------------------
------------------------------------------------*****************************************************---------| 90%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 1875.4172 |
| air_humidity | 13.846659 |
| air_temperature | 21.000006 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 21.0 |
| month | 11.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | -2.6201777 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1687875.5 |
| wind_direction | 50.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
------------------------------------------------
-----------------------------------------------******************************************************---------| 90%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 41.86057 |
| air_temperature | 19.003658 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 15.0 |
| month | 11.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 5.8098664 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 60.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------******************************************************---------| 90%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 807.836 |
| air_humidity | 43.12251 |
| air_temperature | 19.362911 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 19.0 |
| month | 11.0 |
| outdoor_humidity | 89.0 |
| outdoor_temperature | 6.156244 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 681375.3 |
| wind_direction | 250.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------******************************************************---------| 90%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 789.04456 |
| air_humidity | 30.17224 |
| air_temperature | 20.636475 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 86.0 |
| outdoor_temperature | 5.1578355 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 708359.0 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*******************************************************--------| 91%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 295.55637 |
| air_humidity | 25.87323 |
| air_temperature | 19.928282 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 6.0 |
| htg_setpoint | 17.0 |
| month | 11.0 |
| outdoor_humidity | 72.0 |
| outdoor_temperature | 1.742372 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 266000.75 |
| wind_direction | 280.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*******************************************************--------| 91%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 92.7553 |
| air_humidity | 35.296833 |
| air_temperature | 20.206911 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 25.5 |
| direct_solar_radiation | 0.0 |
| hour | 7.0 |
| htg_setpoint | 19.0 |
| month | 11.0 |
| outdoor_humidity | 79.0 |
| outdoor_temperature | 7.921776 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 80147.33 |
| wind_direction | 300.0 |
| wind_speed | 8.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*******************************************************--------| 91%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 163.46709 |
| air_humidity | 29.838661 |
| air_temperature | 19.29609 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 79.5 |
| direct_solar_radiation | 369.5 |
| hour | 8.0 |
| htg_setpoint | 19.0 |
| month | 12.0 |
| outdoor_humidity | 51.0 |
| outdoor_temperature | 5.922729 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 146838.22 |
| wind_direction | 320.0 |
| wind_speed | 12.9 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------********************************************************-------| 92%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 17.84719 |
| air_temperature | 22.499722 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 93.5 |
| direct_solar_radiation | 623.0 |
| hour | 9.0 |
| htg_setpoint | 16.0 |
| month | 12.0 |
| outdoor_humidity | 39.0 |
| outdoor_temperature | 4.658013 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 310.0 |
| wind_speed | 10.8 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------********************************************************-------| 92%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 871.7625 |
| air_humidity | 23.96115 |
| air_temperature | 23.561268 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 68.0 |
| direct_solar_radiation | 804.0 |
| hour | 10.0 |
| htg_setpoint | 16.0 |
| month | 12.0 |
| outdoor_humidity | 47.0 |
| outdoor_temperature | 6.4370503 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 784586.25 |
| wind_direction | 250.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------********************************************************-------| 92%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 755.6465 |
| air_humidity | 39.127277 |
| air_temperature | 22.928534 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 97.5 |
| direct_solar_radiation | 0.0 |
| hour | 11.0 |
| htg_setpoint | 16.0 |
| month | 12.0 |
| outdoor_humidity | 89.0 |
| outdoor_temperature | 7.2501626 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 680081.9 |
| wind_direction | 250.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------********************************************************-------| 92%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 476.10425 |
| air_humidity | 31.817347 |
| air_temperature | 22.907028 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 119.0 |
| direct_solar_radiation | 0.0 |
| hour | 12.0 |
| htg_setpoint | 18.0 |
| month | 12.0 |
| outdoor_humidity | 76.0 |
| outdoor_temperature | 5.028878 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 428493.84 |
| wind_direction | 260.0 |
| wind_speed | 7.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*********************************************************------| 93%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 755.6465 |
| air_humidity | 18.59975 |
| air_temperature | 22.932472 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 96.5 |
| direct_solar_radiation | 104.5 |
| hour | 13.0 |
| htg_setpoint | 21.0 |
| month | 12.0 |
| outdoor_humidity | 43.0 |
| outdoor_temperature | 2.591026 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 680081.9 |
| wind_direction | 270.0 |
| wind_speed | 8.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*********************************************************------| 93%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 70.20606 |
| air_humidity | 18.017624 |
| air_temperature | 21.000004 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 73.5 |
| direct_solar_radiation | 92.5 |
| hour | 14.0 |
| htg_setpoint | 21.0 |
| month | 12.0 |
| outdoor_humidity | 48.0 |
| outdoor_temperature | 4.7990522 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 62652.8 |
| wind_direction | 320.0 |
| wind_speed | 10.3 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
------------------------------------------------********************************************************------| 93%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 124.541046 |
| air_humidity | 18.26065 |
| air_temperature | 22.0 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 15.0 |
| direct_solar_radiation | 308.5 |
| hour | 15.0 |
| htg_setpoint | 22.0 |
| month | 12.0 |
| outdoor_humidity | 45.0 |
| outdoor_temperature | 7.0755606 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 112187.96 |
| wind_direction | 320.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
------------------------------------------------
-----------------------------------------------*********************************************************------| 93%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 27.55016 |
| air_temperature | 23.214323 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 16.0 |
| htg_setpoint | 17.0 |
| month | 12.0 |
| outdoor_humidity | 61.0 |
| outdoor_temperature | 4.394281 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 20.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------**********************************************************-----| 94%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 35.79371 |
| air_temperature | 22.532774 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 15.0 |
| month | 12.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 3.9512193 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 40.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------**********************************************************-----| 94%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 1089.2335 |
| air_humidity | 28.00922 |
| air_temperature | 23.024275 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 17.0 |
| month | 12.0 |
| outdoor_humidity | 83.0 |
| outdoor_temperature | 1.3107407 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 980310.2 |
| wind_direction | 50.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------**********************************************************-----| 94%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 33.208553 |
| air_temperature | 21.219198 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 20.0 |
| month | 12.0 |
| outdoor_humidity | 82.0 |
| outdoor_temperature | 3.8873405 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 280.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------***********************************************************----| 95%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 835.0407 |
| air_humidity | 21.99288 |
| air_temperature | 20.598116 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 21.0 |
| month | 12.0 |
| outdoor_humidity | 52.0 |
| outdoor_temperature | 4.8464212 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 755553.3 |
| wind_direction | 270.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
------------------------------------------------**********************************************************----| 95%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1402.3055 |
| air_humidity | 14.491304 |
| air_temperature | 21.000223 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 21.0 |
| month | 12.0 |
| outdoor_humidity | 25.0 |
| outdoor_temperature | -0.2546195 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1262075.0 |
| wind_direction | 300.0 |
| wind_speed | 7.7 |
| rollout/ | |
| exploration_rate | 0.05 |
------------------------------------------------
-----------------------------------------------***********************************************************----| 95%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 17.221281 |
| air_temperature | 20.598158 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 21.0 |
| month | 12.0 |
| outdoor_humidity | 63.0 |
| outdoor_temperature | 3.1798205 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 240.0 |
| wind_speed | 7.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------************************************************************---| 96%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 700.85516 |
| air_humidity | 24.989456 |
| air_temperature | 20.595875 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 22.0 |
| month | 12.0 |
| outdoor_humidity | 63.0 |
| outdoor_temperature | 5.265303 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 630769.7 |
| wind_direction | 230.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------************************************************************---| 96%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 21.265991 |
| air_temperature | 20.496058 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 22.0 |
| month | 12.0 |
| outdoor_humidity | 65.0 |
| outdoor_temperature | 2.0358138 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 340.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------************************************************************---| 96%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 30.325657 |
| air_temperature | 20.983091 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 21.0 |
| month | 12.0 |
| outdoor_humidity | 75.0 |
| outdoor_temperature | 2.0346045 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 240.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------************************************************************---| 96%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 29.342453 |
| air_temperature | 19.903067 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 20.0 |
| month | 12.0 |
| outdoor_humidity | 79.0 |
| outdoor_temperature | 5.899701 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 250.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
------------------------------------------------************************************************************--| 97%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1322.4016 |
| air_humidity | 22.932446 |
| air_temperature | 20.642416 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 22.0 |
| month | 12.0 |
| outdoor_humidity | 50.0 |
| outdoor_temperature | 0.14490029 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1190161.5 |
| wind_direction | 30.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
------------------------------------------------
------------------------------------------------************************************************************--| 97%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 2363.602 |
| air_humidity | 17.392849 |
| air_temperature | 19.999958 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 20.0 |
| month | 12.0 |
| outdoor_humidity | 46.0 |
| outdoor_temperature | -5.0611014 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2127241.8 |
| wind_direction | 20.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
------------------------------------------------
-----------------------------------------------*************************************************************--| 97%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 20.27708 |
| air_temperature | 19.663279 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 21.0 |
| month | 12.0 |
| outdoor_humidity | 92.0 |
| outdoor_temperature | 2.7721448 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 20.0 |
| wind_speed | 9.3 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------**************************************************************-| 98%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 244.03078 |
| air_humidity | 29.299072 |
| air_temperature | 20.000072 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 6.0 |
| htg_setpoint | 20.0 |
| month | 12.0 |
| outdoor_humidity | 72.0 |
| outdoor_temperature | 3.103836 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 219627.69 |
| wind_direction | 30.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------**************************************************************-| 98%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 244.03078 |
| air_humidity | 47.617172 |
| air_temperature | 19.266903 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 16.5 |
| direct_solar_radiation | 0.0 |
| hour | 7.0 |
| htg_setpoint | 15.0 |
| month | 12.0 |
| outdoor_humidity | 97.0 |
| outdoor_temperature | 10.236619 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 219627.69 |
| wind_direction | 130.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------**************************************************************-| 98%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 34.94709 |
| air_temperature | 21.744276 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 53.5 |
| direct_solar_radiation | 532.0 |
| hour | 8.0 |
| htg_setpoint | 17.0 |
| month | 12.0 |
| outdoor_humidity | 65.0 |
| outdoor_temperature | 8.176662 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 260.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------**************************************************************-| 98%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 972.799 |
| air_humidity | 26.80679 |
| air_temperature | 22.411669 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 70.5 |
| direct_solar_radiation | 0.0 |
| hour | 9.0 |
| htg_setpoint | 18.0 |
| month | 12.0 |
| outdoor_humidity | 92.0 |
| outdoor_temperature | 1.435689 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 875519.1 |
| wind_direction | 320.0 |
| wind_speed | 1.5 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------***************************************************************| 99%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 29.151196 |
| air_temperature | 21.667337 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 115.0 |
| direct_solar_radiation | 582.5 |
| hour | 10.0 |
| htg_setpoint | 17.0 |
| month | 12.0 |
| outdoor_humidity | 59.0 |
| outdoor_temperature | 3.6377366 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 310.0 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------***************************************************************| 99%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 101.81965 |
| air_humidity | 19.736752 |
| air_temperature | 20.999989 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 133.5 |
| direct_solar_radiation | 0.0 |
| hour | 11.0 |
| htg_setpoint | 21.0 |
| month | 12.0 |
| outdoor_humidity | 60.0 |
| outdoor_temperature | 5.0482287 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 91637.68 |
| wind_direction | 50.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------***************************************************************| 99%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 519.18353 |
| air_humidity | 28.049627 |
| air_temperature | 22.243378 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 103.5 |
| direct_solar_radiation | 24.0 |
| hour | 12.0 |
| htg_setpoint | 21.0 |
| month | 12.0 |
| outdoor_humidity | 88.0 |
| outdoor_temperature | 1.7846037 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 467265.2 |
| wind_direction | 240.0 |
| wind_speed | 14.9 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
------------------------------------------------**************************************************************| 99%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 1437.554 |
| air_humidity | 14.440469 |
| air_temperature | 23.035702 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 102.0 |
| direct_solar_radiation | 487.5 |
| hour | 13.0 |
| htg_setpoint | 19.0 |
| month | 12.0 |
| outdoor_humidity | 38.0 |
| outdoor_temperature | -1.0675281 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 1293798.5 |
| wind_direction | 300.0 |
| wind_speed | 10.8 |
| rollout/ | |
| exploration_rate | 0.05 |
------------------------------------------------
[WRAPPER LoggerWrapper] (INFO) : End of episode detected, recording summary (progress.csv) if logger is active
#----------------------------------------------------------------------------------------------#
[ENVIRONMENT] (INFO) : Starting a new episode... [SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43] [Episode 2]
#----------------------------------------------------------------------------------------------#
[MODELING] (INFO) : Episode directory created [/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43-res1/Eplus-env-sub_run2]
[MODELING] (INFO) : Weather file USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3.epw used.
[MODELING] (INFO) : Updated building model with whole Output:Variable available names
[MODELING] (INFO) : Updated building model with whole Output:Meter available names
[MODELING] (INFO) : Adapting weather to building model. [USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3.epw]
[ENVIRONMENT] (INFO) : Saving episode output path... [/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43-res1/Eplus-env-sub_run2/output]
[SIMULATOR] (INFO) : Running EnergyPlus with args: ['-w', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43-res1/Eplus-env-sub_run2/USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3_Random_1.0_0.0_0.001.epw', '-d', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43-res1/Eplus-env-sub_run2/output', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43-res1/Eplus-env-sub_run2/5ZoneAutoDXVAV.epJSON']
[ENVIRONMENT] (INFO) : Episode 2 started.
/usr/local/lib/python3.10/dist-packages/opyplus/weather_data/weather_data.py:493: FutureWarning: the 'line_terminator'' keyword is deprecated, use 'lineterminator' instead.
epw_content = self._headers_to_epw(use_datetimes=use_datetimes) + df.to_csv(
[SIMULATOR] (INFO) : handlers are ready.
[SIMULATOR] (INFO) : System is ready.
[WRAPPER LoggerWrapper] (INFO) : Creating monitor.csv for current episode (episode 2) if logger is active
-----------------------------------------------------
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| episode/ | |
| comfort_violation_time(%) | 42.6 |
| cumulative_comfort_penalty | -1e+04 |
| cumulative_energy_penalty | -1.79e+03 |
| cumulative_power | 3.57e+07 |
| cumulative_reward | -11781.918 |
| cumulative_temperature_violation | 2e+04 |
| episode_length | 35040 |
| mean_comfort_penalty | -0.285 |
| mean_energy_penalty | -0.0509 |
| mean_power | 1.02e+03 |
| mean_reward | -0.33624196 |
| mean_temperature_violation | 0.571 |
| observation/ | |
| HVAC_electricity_demand_rate | 3206.5505 |
| air_humidity | 16.606789 |
| air_temperature | 16.834461 |
| clg_setpoint | 40.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 12.8 |
| month | 1.0 |
| outdoor_humidity | 43.25 |
| outdoor_temperature | -9.275844 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2885895.5 |
| wind_direction | 305.0 |
| wind_speed | 8.4 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------------
-----------------------------------
| rollout/ | |
| ep_len_mean | 3.5e+04 |
| ep_rew_mean | -1.18e+04 |
| exploration_rate | 0.05 |
| time/ | |
| episodes | 1 |
| fps | 1779 |
| time_elapsed | 19 |
| total_timesteps | 35040 |
-----------------------------------
------------------------------------------------
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1804.2375 |
| air_humidity | 14.429458 |
| air_temperature | 22.996721 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 95.5 |
| direct_solar_radiation | 157.0 |
| hour | 14.0 |
| htg_setpoint | 18.0 |
| month | 1.0 |
| outdoor_humidity | 32.0 |
| outdoor_temperature | -2.7215035 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1623813.8 |
| wind_direction | 290.0 |
| wind_speed | 9.8 |
| rollout/ | |
| exploration_rate | 0.05 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 1%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 2286.7896 |
| air_humidity | 13.794168 |
| air_temperature | 23.17125 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 33.0 |
| direct_solar_radiation | 54.5 |
| hour | 15.0 |
| htg_setpoint | 22.0 |
| month | 1.0 |
| outdoor_humidity | 35.0 |
| outdoor_temperature | -5.134264 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 2058110.6 |
| wind_direction | 70.0 |
| wind_speed | 1.5 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 1%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 51.033295 |
| air_temperature | 23.009144 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 16.0 |
| htg_setpoint | 15.0 |
| month | 1.0 |
| outdoor_humidity | 92.0 |
| outdoor_temperature | 6.7510724 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 340.0 |
| wind_speed | 8.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 1%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 2267.5652 |
| air_humidity | 15.053332 |
| air_temperature | 22.429335 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 21.0 |
| month | 1.0 |
| outdoor_humidity | 38.0 |
| outdoor_temperature | -5.0381413 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 2040808.6 |
| wind_direction | 270.0 |
| wind_speed | 9.8 |
| rollout/ | |
| exploration_rate | 0.05 |
------------------------------------------------
/usr/local/lib/python3.10/dist-packages/gymnasium/core.py:311: UserWarning: WARN: env.observation_variables to get variables from other wrappers is deprecated and will be removed in v1.0, to get this variable you can do `env.unwrapped.observation_variables` for environment variables or `env.get_wrapper_attr('observation_variables')` that will search the reminding wrappers.
logger.warn(
/usr/local/lib/python3.10/dist-packages/gymnasium/core.py:311: UserWarning: WARN: env.action_variables to get variables from other wrappers is deprecated and will be removed in v1.0, to get this variable you can do `env.unwrapped.action_variables` for environment variables or `env.get_wrapper_attr('action_variables')` that will search the reminding wrappers.
logger.warn(
/usr/local/lib/python3.10/dist-packages/gymnasium/core.py:311: UserWarning: WARN: env.is_discrete to get variables from other wrappers is deprecated and will be removed in v1.0, to get this variable you can do `env.unwrapped.is_discrete` for environment variables or `env.get_wrapper_attr('is_discrete')` that will search the reminding wrappers.
logger.warn(
/usr/local/lib/python3.10/dist-packages/gymnasium/spaces/box.py:240: UserWarning: WARN: Casting input x to numpy array.
gym.logger.warn("Casting input x to numpy array.")
--------------------------------------------------------------------------------------------------------------| 1%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 2373.0137 |
| air_humidity | 11.418623 |
| air_temperature | 20.962894 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 20.0 |
| month | 1.0 |
| outdoor_humidity | 38.0 |
| outdoor_temperature | -5.1081605 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2135712.5 |
| wind_direction | 340.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 2%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 1758.2157 |
| air_humidity | 9.940797 |
| air_temperature | 20.618435 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 21.0 |
| month | 1.0 |
| outdoor_humidity | 54.0 |
| outdoor_temperature | -2.03417 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1582394.1 |
| wind_direction | 210.0 |
| wind_speed | 9.3 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 2%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 2139.4778 |
| air_humidity | 17.66047 |
| air_temperature | 21.4038 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 21.0 |
| month | 1.0 |
| outdoor_humidity | 32.0 |
| outdoor_temperature | -3.9404805 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 1925530.0 |
| wind_direction | 280.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 2%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 1455.3401 |
| air_humidity | 33.264008 |
| air_temperature | 20.997766 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 21.0 |
| month | 1.0 |
| outdoor_humidity | 92.0 |
| outdoor_temperature | -0.5197919 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1309806.1 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 2%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 1222.3441 |
| air_humidity | 34.790493 |
| air_temperature | 20.846558 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 21.0 |
| month | 1.0 |
| outdoor_humidity | 76.0 |
| outdoor_temperature | 0.645188 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1100109.8 |
| wind_direction | 330.0 |
| wind_speed | 9.3 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 3%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 1883.9517 |
| air_humidity | 21.349102 |
| air_temperature | 19.073694 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 17.0 |
| month | 1.0 |
| outdoor_humidity | 51.0 |
| outdoor_temperature | -2.66285 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1695556.5 |
| wind_direction | 230.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 3%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 1546.6324 |
| air_humidity | 16.957151 |
| air_temperature | 18.970556 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 18.0 |
| month | 1.0 |
| outdoor_humidity | 51.0 |
| outdoor_temperature | -0.97625375 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1391969.2 |
| wind_direction | 180.0 |
| wind_speed | 7.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 4%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 148.38998 |
| air_humidity | 25.182379 |
| air_temperature | 20.113134 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 16.0 |
| month | 1.0 |
| outdoor_humidity | 89.0 |
| outdoor_temperature | 4.72036 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 134166.44 |
| wind_direction | 190.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 4%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 2781.4126 |
| air_humidity | 23.80908 |
| air_temperature | 19.716034 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 22.0 |
| month | 1.0 |
| outdoor_humidity | 86.0 |
| outdoor_temperature | -7.150154 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2503271.2 |
| wind_direction | 30.0 |
| wind_speed | 7.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 4%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1030.1261 |
| air_humidity | 23.667334 |
| air_temperature | 19.352226 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 20.0 |
| month | 1.0 |
| outdoor_humidity | 95.0 |
| outdoor_temperature | 1.6062777 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 927113.56 |
| wind_direction | 50.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 4%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 1950.5087 |
| air_humidity | 29.971148 |
| air_temperature | 18.999996 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 19.0 |
| month | 1.0 |
| outdoor_humidity | 61.0 |
| outdoor_temperature | -2.9956346 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1755457.8 |
| wind_direction | 260.0 |
| wind_speed | 8.2 |
| rollout/ | |
| exploration_rate | 0.05 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 5%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 1148.0468 |
| air_humidity | 34.12383 |
| air_temperature | 19.986454 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 16.0 |
| month | 1.0 |
| outdoor_humidity | 91.0 |
| outdoor_temperature | 1.0166746 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1033242.06 |
| wind_direction | 210.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 5%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 244.03078 |
| air_humidity | 41.51869 |
| air_temperature | 19.999989 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 6.0 |
| htg_setpoint | 20.0 |
| month | 1.0 |
| outdoor_humidity | 97.0 |
| outdoor_temperature | 9.285549 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 219627.69 |
| wind_direction | 120.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 5%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 35.05086 |
| air_temperature | 20.530643 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 39.5 |
| direct_solar_radiation | 326.0 |
| hour | 7.0 |
| htg_setpoint | 15.0 |
| month | 1.0 |
| outdoor_humidity | 89.0 |
| outdoor_temperature | 2.3865857 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 240.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 5%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 122.68099 |
| air_humidity | 16.205442 |
| air_temperature | 20.136335 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 110.0 |
| direct_solar_radiation | 362.0 |
| hour | 8.0 |
| htg_setpoint | 17.0 |
| month | 1.0 |
| outdoor_humidity | 33.0 |
| outdoor_temperature | 4.9920626 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 107556.51 |
| wind_direction | 270.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 6%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 31.507713 |
| air_temperature | 22.5794 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 172.5 |
| direct_solar_radiation | 34.0 |
| hour | 9.0 |
| htg_setpoint | 18.0 |
| month | 1.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 4.3967648 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 70.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 6%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 40.34489 |
| air_temperature | 23.049335 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 81.0 |
| direct_solar_radiation | 0.0 |
| hour | 10.0 |
| htg_setpoint | 17.0 |
| month | 1.0 |
| outdoor_humidity | 92.0 |
| outdoor_temperature | 7.3515596 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 40.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 6%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 748.64197 |
| air_humidity | 45.458416 |
| air_temperature | 23.0614 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 103.0 |
| direct_solar_radiation | 0.0 |
| hour | 11.0 |
| htg_setpoint | 22.0 |
| month | 1.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 9.038888 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 673777.8 |
| wind_direction | 50.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 7%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 231.8666 |
| air_humidity | 53.649773 |
| air_temperature | 24.061403 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 82.0 |
| direct_solar_radiation | 0.0 |
| hour | 12.0 |
| htg_setpoint | 15.0 |
| month | 1.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 13.094668 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 208679.92 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 7%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 755.6465 |
| air_humidity | 28.547411 |
| air_temperature | 22.799263 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 67.5 |
| direct_solar_radiation | 0.0 |
| hour | 13.0 |
| htg_setpoint | 18.0 |
| month | 1.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 2.623999 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 680081.9 |
| wind_direction | 260.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 7%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 26.4357 |
| air_temperature | 21.611109 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 108.5 |
| direct_solar_radiation | 93.0 |
| hour | 14.0 |
| htg_setpoint | 19.0 |
| month | 1.0 |
| outdoor_humidity | 53.0 |
| outdoor_temperature | 4.027222 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 260.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 7%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 20.152868 |
| air_temperature | 21.999857 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 58.0 |
| direct_solar_radiation | 189.5 |
| hour | 15.0 |
| htg_setpoint | 22.0 |
| month | 1.0 |
| outdoor_humidity | 58.0 |
| outdoor_temperature | 3.5773697 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 180.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 8%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 33.50244 |
| air_temperature | 22.925869 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 8.5 |
| direct_solar_radiation | 0.0 |
| hour | 16.0 |
| htg_setpoint | 20.0 |
| month | 1.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 3.5959754 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 40.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 8%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 1462.5768 |
| air_humidity | 20.541664 |
| air_temperature | 23.24956 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 21.0 |
| month | 1.0 |
| outdoor_humidity | 54.0 |
| outdoor_temperature | -1.0131997 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1316319.1 |
| wind_direction | 50.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 8%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1349.1754 |
| air_humidity | 24.63473 |
| air_temperature | 23.735115 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 18.0 |
| month | 1.0 |
| outdoor_humidity | 66.0 |
| outdoor_temperature | 0.0110311955 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 1214257.9 |
| wind_direction | 0.0 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.05 |
--------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 8%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1886.4735 |
| air_humidity | 16.737734 |
| air_temperature | 22.882967 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 19.0 |
| month | 1.0 |
| outdoor_humidity | 42.0 |
| outdoor_temperature | -2.6754591 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 1697826.1 |
| wind_direction | 230.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 9%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 585.49194 |
| air_humidity | 28.731716 |
| air_temperature | 20.996204 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 21.0 |
| month | 2.0 |
| outdoor_humidity | 67.0 |
| outdoor_temperature | 6.31725 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 526942.75 |
| wind_direction | 280.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 9%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 12.700426 |
| air_temperature | 20.538958 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 17.0 |
| month | 2.0 |
| outdoor_humidity | 32.0 |
| outdoor_temperature | -2.3895953 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 10.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 9%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 1319.7701 |
| air_humidity | 11.374336 |
| air_temperature | 20.237171 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 19.0 |
| month | 2.0 |
| outdoor_humidity | 66.0 |
| outdoor_temperature | 0.15805787 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1187793.1 |
| wind_direction | 150.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
------------------------------------------------
Progress: |**********-----------------------------------------------------------------------------------------| 10%------------------------------------------------
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 1625.1184 |
| air_humidity | 29.68434 |
| air_temperature | 19.554268 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 15.0 |
| month | 2.0 |
| outdoor_humidity | 62.0 |
| outdoor_temperature | -1.3686837 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1462606.6 |
| wind_direction | 320.0 |
| wind_speed | 9.3 |
| rollout/ | |
| exploration_rate | 0.05 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 10%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 3791.435 |
| air_humidity | 16.024805 |
| air_temperature | 18.399399 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 22.0 |
| month | 2.0 |
| outdoor_humidity | 39.0 |
| outdoor_temperature | -12.200267 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 3412291.5 |
| wind_direction | 300.0 |
| wind_speed | 9.8 |
| rollout/ | |
| exploration_rate | 0.05 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 10%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 3162.4832 |
| air_humidity | 16.801003 |
| air_temperature | 17.480116 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 15.0 |
| month | 2.0 |
| outdoor_humidity | 52.0 |
| outdoor_temperature | -9.055507 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2846234.8 |
| wind_direction | 300.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 11%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 2316.3386 |
| air_humidity | 18.613413 |
| air_temperature | 19.890545 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 21.0 |
| month | 2.0 |
| outdoor_humidity | 46.0 |
| outdoor_temperature | -4.8247848 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2084704.8 |
| wind_direction | 310.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 11%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 3050.6633 |
| air_humidity | 16.25804 |
| air_temperature | 18.83905 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 16.0 |
| month | 2.0 |
| outdoor_humidity | 49.0 |
| outdoor_temperature | -8.496408 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2745597.0 |
| wind_direction | 310.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 11%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 1886.6011 |
| air_humidity | 12.712721 |
| air_temperature | 18.790092 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 18.0 |
| month | 2.0 |
| outdoor_humidity | 62.0 |
| outdoor_temperature | -2.6760972 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1697941.0 |
| wind_direction | 270.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 11%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1514.844 |
| air_humidity | 16.692741 |
| air_temperature | 19.63072 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 16.0 |
| month | 2.0 |
| outdoor_humidity | 80.0 |
| outdoor_temperature | -0.81731147 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1363359.6 |
| wind_direction | 250.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 12%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 2945.1492 |
| air_humidity | 18.053753 |
| air_temperature | 18.193132 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 18.0 |
| direct_solar_radiation | 40.0 |
| hour | 6.0 |
| htg_setpoint | 15.0 |
| month | 2.0 |
| outdoor_humidity | 29.0 |
| outdoor_temperature | -11.505592 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2650634.2 |
| wind_direction | 320.0 |
| wind_speed | 9.3 |
| rollout/ | |
| exploration_rate | 0.05 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 12%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 2493.5735 |
| air_humidity | 10.131987 |
| air_temperature | 18.372742 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 69.0 |
| direct_solar_radiation | 187.0 |
| hour | 7.0 |
| htg_setpoint | 18.0 |
| month | 2.0 |
| outdoor_humidity | 44.0 |
| outdoor_temperature | -9.247714 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2244216.2 |
| wind_direction | 300.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 12%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 1821.0771 |
| air_humidity | 13.044007 |
| air_temperature | 21.999996 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 106.0 |
| direct_solar_radiation | 395.0 |
| hour | 8.0 |
| htg_setpoint | 22.0 |
| month | 2.0 |
| outdoor_humidity | 38.0 |
| outdoor_temperature | -2.7465734 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1638969.5 |
| wind_direction | 300.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 13%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 1367.605 |
| air_humidity | 18.204165 |
| air_temperature | 22.377993 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 190.5 |
| direct_solar_radiation | 29.5 |
| hour | 9.0 |
| htg_setpoint | 18.0 |
| month | 2.0 |
| outdoor_humidity | 60.0 |
| outdoor_temperature | -0.5383407 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1230844.5 |
| wind_direction | 110.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 13%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 151.11612 |
| air_humidity | 31.204664 |
| air_temperature | 20.458956 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 224.5 |
| direct_solar_radiation | 314.0 |
| hour | 10.0 |
| htg_setpoint | 21.0 |
| month | 2.0 |
| outdoor_humidity | 65.0 |
| outdoor_temperature | 6.2909584 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 144360.28 |
| wind_direction | 300.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 13%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 76.76114 |
| air_humidity | 17.126703 |
| air_temperature | 22.000053 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 124.0 |
| direct_solar_radiation | 727.5 |
| hour | 11.0 |
| htg_setpoint | 22.0 |
| month | 2.0 |
| outdoor_humidity | 36.0 |
| outdoor_temperature | 6.369709 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 70249.89 |
| wind_direction | 0.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 13%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 476.10425 |
| air_humidity | 25.14942 |
| air_temperature | 23.491219 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 241.0 |
| direct_solar_radiation | 284.5 |
| hour | 12.0 |
| htg_setpoint | 16.0 |
| month | 2.0 |
| outdoor_humidity | 62.0 |
| outdoor_temperature | 6.582677 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 428493.84 |
| wind_direction | 190.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 14%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 24.28904 |
| air_temperature | 24.073921 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 183.5 |
| direct_solar_radiation | 475.5 |
| hour | 13.0 |
| htg_setpoint | 22.0 |
| month | 2.0 |
| outdoor_humidity | 44.0 |
| outdoor_temperature | 7.3682384 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 200.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 14%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 688.9026 |
| air_humidity | 25.790758 |
| air_temperature | 24.51302 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 101.5 |
| direct_solar_radiation | 584.0 |
| hour | 14.0 |
| htg_setpoint | 17.0 |
| month | 2.0 |
| outdoor_humidity | 56.0 |
| outdoor_temperature | 9.043416 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 593599.75 |
| wind_direction | 120.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 14%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 31.605486 |
| air_temperature | 23.198685 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 63.5 |
| direct_solar_radiation | 0.0 |
| hour | 15.0 |
| htg_setpoint | 21.0 |
| month | 2.0 |
| outdoor_humidity | 82.0 |
| outdoor_temperature | 3.3204484 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 30.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 14%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 917.7176 |
| air_humidity | 19.038803 |
| air_temperature | 23.908525 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 27.5 |
| direct_solar_radiation | 273.0 |
| hour | 16.0 |
| htg_setpoint | 21.0 |
| month | 2.0 |
| outdoor_humidity | 45.0 |
| outdoor_temperature | 1.9434859 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 825945.9 |
| wind_direction | 250.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 15%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 22.889832 |
| air_temperature | 21.24511 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 18.0 |
| month | 2.0 |
| outdoor_humidity | 89.0 |
| outdoor_temperature | 3.2798805 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 120.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 15%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 1150.1122 |
| air_humidity | 18.517626 |
| air_temperature | 20.998045 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 16.0 |
| month | 2.0 |
| outdoor_humidity | 39.0 |
| outdoor_temperature | 1.0063478 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1035100.94 |
| wind_direction | 300.0 |
| wind_speed | 9.8 |
| rollout/ | |
| exploration_rate | 0.05 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 15%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 533.8715 |
| air_humidity | 22.031479 |
| air_temperature | 22.000027 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 22.0 |
| month | 2.0 |
| outdoor_humidity | 49.0 |
| outdoor_temperature | 4.355374 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 474075.22 |
| wind_direction | 250.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 16%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 1502.4996 |
| air_humidity | 17.550522 |
| air_temperature | 21.454084 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 20.0 |
| month | 2.0 |
| outdoor_humidity | 27.0 |
| outdoor_temperature | -0.75558984 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 1352249.8 |
| wind_direction | 70.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 16%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 1700.4568 |
| air_humidity | 38.744617 |
| air_temperature | 19.918364 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 19.0 |
| month | 2.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | -1.7453753 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1530411.1 |
| wind_direction | 0.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 16%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 840.8903 |
| air_humidity | 40.83299 |
| air_temperature | 19.238083 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 19.0 |
| month | 2.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 5.5238667 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 725613.3 |
| wind_direction | 20.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
Progress: |*****************----------------------------------------------------------------------------------| 17%-----------------------------------------------
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 708.3994 |
| air_humidity | 36.43662 |
| air_temperature | 19.460455 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 15.0 |
| month | 3.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 6.6392717 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 593720.8 |
| wind_direction | 100.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 17%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 33.624523 |
| air_temperature | 21.84918 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 22.0 |
| month | 3.0 |
| outdoor_humidity | 54.0 |
| outdoor_temperature | 10.09713 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 280.0 |
| wind_speed | 8.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 17%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 474.4464 |
| air_humidity | 32.48305 |
| air_temperature | 19.565111 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 16.0 |
| month | 3.0 |
| outdoor_humidity | 71.0 |
| outdoor_temperature | 9.315889 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 406927.03 |
| wind_direction | 170.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 17%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 927.30707 |
| air_humidity | 43.51403 |
| air_temperature | 19.22073 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 18.0 |
| month | 3.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 5.875117 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 781413.8 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 18%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 575.30975 |
| air_humidity | 45.782436 |
| air_temperature | 20.171947 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 21.0 |
| month | 3.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 9.522872 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 555115.25 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 18%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 782.5654 |
| air_humidity | 24.75921 |
| air_temperature | 20.15559 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 21.0 |
| month | 3.0 |
| outdoor_humidity | 53.0 |
| outdoor_temperature | 6.049054 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 704308.94 |
| wind_direction | 300.0 |
| wind_speed | 8.8 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 18%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 35.990368 |
| air_temperature | 19.806833 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 16.0 |
| month | 3.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 3.5513284 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 60.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 19%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 37.737408 |
| air_temperature | 20.442892 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 72.0 |
| direct_solar_radiation | 175.0 |
| hour | 6.0 |
| htg_setpoint | 16.0 |
| month | 3.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 2.1105442 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 240.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 19%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 32.52455 |
| air_temperature | 20.50352 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 141.5 |
| direct_solar_radiation | 118.0 |
| hour | 7.0 |
| htg_setpoint | 16.0 |
| month | 3.0 |
| outdoor_humidity | 89.0 |
| outdoor_temperature | 2.6969094 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 60.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 19%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 871.7625 |
| air_humidity | 22.176426 |
| air_temperature | 21.72639 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 132.5 |
| direct_solar_radiation | 687.5 |
| hour | 8.0 |
| htg_setpoint | 18.0 |
| month | 3.0 |
| outdoor_humidity | 42.0 |
| outdoor_temperature | 7.6411133 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 784586.25 |
| wind_direction | 0.0 |
| wind_speed | 9.3 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 19%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 871.7625 |
| air_humidity | 23.683573 |
| air_temperature | 22.5603 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 291.0 |
| direct_solar_radiation | 353.5 |
| hour | 9.0 |
| htg_setpoint | 22.0 |
| month | 3.0 |
| outdoor_humidity | 37.0 |
| outdoor_temperature | 4.719579 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 784586.25 |
| wind_direction | 260.0 |
| wind_speed | 15.5 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 20%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 947.8628 |
| air_humidity | 17.563786 |
| air_temperature | 23.31355 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 146.5 |
| direct_solar_radiation | 820.0 |
| hour | 10.0 |
| htg_setpoint | 22.0 |
| month | 3.0 |
| outdoor_humidity | 34.0 |
| outdoor_temperature | 4.4292946 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 853076.5 |
| wind_direction | 310.0 |
| wind_speed | 9.8 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 20%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 819.6643 |
| air_humidity | 17.929089 |
| air_temperature | 23.582941 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 310.5 |
| direct_solar_radiation | 462.0 |
| hour | 11.0 |
| htg_setpoint | 16.0 |
| month | 3.0 |
| outdoor_humidity | 53.0 |
| outdoor_temperature | 1.6799109 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 737697.9 |
| wind_direction | 150.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 20%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 183.02463 |
| air_humidity | 22.134924 |
| air_temperature | 25.33988 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 140.5 |
| direct_solar_radiation | 840.0 |
| hour | 12.0 |
| htg_setpoint | 16.0 |
| month | 3.0 |
| outdoor_humidity | 35.0 |
| outdoor_temperature | 12.848213 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 165756.19 |
| wind_direction | 270.0 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 20%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1372.1312 |
| air_humidity | 16.91895 |
| air_temperature | 20.388855 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 103.5 |
| direct_solar_radiation | 0.0 |
| hour | 13.0 |
| htg_setpoint | 19.0 |
| month | 3.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | -0.10374769 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1234918.1 |
| wind_direction | 50.0 |
| wind_speed | 9.3 |
| rollout/ | |
| exploration_rate | 0.05 |
-------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 21%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1435.1711 |
| air_humidity | 18.643396 |
| air_temperature | 21.139025 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 168.5 |
| direct_solar_radiation | 643.0 |
| hour | 14.0 |
| htg_setpoint | 16.0 |
| month | 3.0 |
| outdoor_humidity | 83.0 |
| outdoor_temperature | -0.41894728 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1291654.0 |
| wind_direction | 20.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 21%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 906.4148 |
| air_humidity | 26.15411 |
| air_temperature | 24.043047 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 132.5 |
| direct_solar_radiation | 552.0 |
| hour | 15.0 |
| htg_setpoint | 19.0 |
| month | 3.0 |
| outdoor_humidity | 68.0 |
| outdoor_temperature | 3.821802 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 815773.3 |
| wind_direction | 170.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 21%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 26.803059 |
| air_temperature | 23.57881 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 61.5 |
| direct_solar_radiation | 360.0 |
| hour | 16.0 |
| htg_setpoint | 15.0 |
| month | 3.0 |
| outdoor_humidity | 58.0 |
| outdoor_temperature | 3.7118824 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 350.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 22%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 838.38043 |
| air_humidity | 32.72246 |
| air_temperature | 23.509342 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 16.5 |
| direct_solar_radiation | 91.5 |
| hour | 17.0 |
| htg_setpoint | 16.0 |
| month | 3.0 |
| outdoor_humidity | 76.0 |
| outdoor_temperature | 5.683642 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 746401.25 |
| wind_direction | 180.0 |
| wind_speed | 11.8 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 22%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 722.22064 |
| air_humidity | 21.36357 |
| air_temperature | 21.937164 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 21.0 |
| month | 3.0 |
| outdoor_humidity | 39.0 |
| outdoor_temperature | 4.786942 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 636776.6 |
| wind_direction | 290.0 |
| wind_speed | 14.4 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 22%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1420.1923 |
| air_humidity | 15.754087 |
| air_temperature | 23.23445 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 16.0 |
| month | 3.0 |
| outdoor_humidity | 22.0 |
| outdoor_temperature | -0.34405267 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 1278173.0 |
| wind_direction | 300.0 |
| wind_speed | 8.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 22%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 15.957253 |
| air_temperature | 20.980701 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 16.0 |
| month | 3.0 |
| outdoor_humidity | 53.0 |
| outdoor_temperature | 3.6350272 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 220.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 23%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 28.30168 |
| air_temperature | 21.18839 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 17.0 |
| month | 3.0 |
| outdoor_humidity | 97.0 |
| outdoor_temperature | 6.6833673 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 23%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 785.40643 |
| air_humidity | 43.216587 |
| air_temperature | 19.139505 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 15.0 |
| month | 3.0 |
| outdoor_humidity | 97.0 |
| outdoor_temperature | 6.2410784 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 701437.75 |
| wind_direction | 170.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
Progress: |***********************----------------------------------------------------------------------------| 23%-----------------------------------------------
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 481.7842 |
| air_humidity | 47.513245 |
| air_temperature | 20.326927 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 22.0 |
| month | 3.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 8.220632 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 451453.1 |
| wind_direction | 170.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 24%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 194.08734 |
| air_humidity | 43.19421 |
| air_temperature | 20.993734 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 21.0 |
| month | 3.0 |
| outdoor_humidity | 74.0 |
| outdoor_temperature | 11.772061 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 170518.66 |
| wind_direction | 0.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 24%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 28.857058 |
| air_temperature | 20.061483 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 18.0 |
| month | 3.0 |
| outdoor_humidity | 57.0 |
| outdoor_temperature | 2.9912403 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 30.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 24%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 37.21652 |
| air_temperature | 20.998018 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 21.0 |
| month | 3.0 |
| outdoor_humidity | 81.0 |
| outdoor_temperature | 2.1145651 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 80.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 25%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 143.43005 |
| air_humidity | 36.30297 |
| air_temperature | 20.30996 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 22.0 |
| month | 3.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 6.333977 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 140959.28 |
| wind_direction | 20.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 25%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 51.015564 |
| air_temperature | 18.754827 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 18.0 |
| month | 4.0 |
| outdoor_humidity | 75.0 |
| outdoor_temperature | 2.8850982 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 40.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 25%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 69.60917 |
| air_temperature | 18.369095 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 27.0 |
| direct_solar_radiation | 165.0 |
| hour | 5.0 |
| htg_setpoint | 21.0 |
| month | 4.0 |
| outdoor_humidity | 75.0 |
| outdoor_temperature | 4.028294 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 280.0 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 25%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 58.062473 |
| air_temperature | 18.538546 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 52.5 |
| direct_solar_radiation | 541.5 |
| hour | 6.0 |
| htg_setpoint | 21.0 |
| month | 4.0 |
| outdoor_humidity | 60.0 |
| outdoor_temperature | 3.634861 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 0.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 26%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 244.03078 |
| air_humidity | 43.828773 |
| air_temperature | 18.841476 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 146.5 |
| direct_solar_radiation | 403.0 |
| hour | 7.0 |
| htg_setpoint | 20.0 |
| month | 4.0 |
| outdoor_humidity | 55.0 |
| outdoor_temperature | 6.183797 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 219627.69 |
| wind_direction | 260.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 26%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 572.3576 |
| air_humidity | 44.74038 |
| air_temperature | 21.419844 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 173.0 |
| direct_solar_radiation | 567.0 |
| hour | 8.0 |
| htg_setpoint | 21.0 |
| month | 4.0 |
| outdoor_humidity | 69.0 |
| outdoor_temperature | 10.205873 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 523577.4 |
| wind_direction | 180.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 26%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 76.707 |
| air_temperature | 22.388348 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 220.5 |
| direct_solar_radiation | 635.0 |
| hour | 9.0 |
| htg_setpoint | 16.0 |
| month | 4.0 |
| outdoor_humidity | 48.0 |
| outdoor_temperature | 19.456167 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 30.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 26%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 70.24044 |
| air_temperature | 23.77905 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 143.5 |
| direct_solar_radiation | 847.0 |
| hour | 10.0 |
| htg_setpoint | 21.0 |
| month | 4.0 |
| outdoor_humidity | 37.0 |
| outdoor_temperature | 22.28776 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 250.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 27%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 3445.089 |
| air_humidity | 53.412476 |
| air_temperature | 23.671152 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 283.0 |
| direct_solar_radiation | 626.0 |
| hour | 11.0 |
| htg_setpoint | 16.0 |
| month | 4.0 |
| outdoor_humidity | 43.0 |
| outdoor_temperature | 23.813982 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 3108444.2 |
| wind_direction | 190.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 27%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 1941.4698 |
| air_humidity | 57.059998 |
| air_temperature | 23.39732 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 248.0 |
| direct_solar_radiation | 449.5 |
| hour | 12.0 |
| htg_setpoint | 16.0 |
| month | 4.0 |
| outdoor_humidity | 68.0 |
| outdoor_temperature | 19.118326 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 1746407.5 |
| wind_direction | 190.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 27%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 3975.0776 |
| air_humidity | 32.96631 |
| air_temperature | 23.598097 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 153.5 |
| direct_solar_radiation | 773.5 |
| hour | 13.0 |
| htg_setpoint | 21.0 |
| month | 4.0 |
| outdoor_humidity | 25.0 |
| outdoor_temperature | 20.640772 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 3574334.0 |
| wind_direction | 290.0 |
| wind_speed | 7.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 28%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 306.31296 |
| air_humidity | 14.682458 |
| air_temperature | 25.941786 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 142.5 |
| direct_solar_radiation | 728.5 |
| hour | 14.0 |
| htg_setpoint | 16.0 |
| month | 4.0 |
| outdoor_humidity | 14.0 |
| outdoor_temperature | 15.408057 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 275078.9 |
| wind_direction | 310.0 |
| wind_speed | 12.9 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 28%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 315.61072 |
| air_humidity | 12.921776 |
| air_temperature | 25.000605 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 72.0 |
| direct_solar_radiation | 766.0 |
| hour | 15.0 |
| htg_setpoint | 15.0 |
| month | 4.0 |
| outdoor_humidity | 11.0 |
| outdoor_temperature | 11.472333 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 280739.22 |
| wind_direction | 0.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 28%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 37.093914 |
| air_temperature | 20.82961 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 69.0 |
| direct_solar_radiation | 0.0 |
| hour | 16.0 |
| htg_setpoint | 18.0 |
| month | 4.0 |
| outdoor_humidity | 86.0 |
| outdoor_temperature | 6.4494166 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 170.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 28%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 32.12789 |
| air_temperature | 23.169945 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 45.5 |
| direct_solar_radiation | 25.5 |
| hour | 17.0 |
| htg_setpoint | 22.0 |
| month | 4.0 |
| outdoor_humidity | 70.0 |
| outdoor_temperature | 6.8088455 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 140.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 29%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 54.234688 |
| air_temperature | 26.290154 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 22.0 |
| month | 4.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 8.991571 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 170.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 29%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 49.74599 |
| air_temperature | 27.69215 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 22.0 |
| month | 4.0 |
| outdoor_humidity | 60.0 |
| outdoor_temperature | 15.791064 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 250.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 29%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 60.868176 |
| air_temperature | 23.51201 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 16.0 |
| month | 4.0 |
| outdoor_humidity | 97.0 |
| outdoor_temperature | 7.527386 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 110.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 29%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 56.438843 |
| air_temperature | 22.010849 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 19.0 |
| month | 4.0 |
| outdoor_humidity | 90.0 |
| outdoor_temperature | 6.091949 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 140.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 30%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 56.108757 |
| air_temperature | 22.255705 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 17.0 |
| month | 4.0 |
| outdoor_humidity | 65.0 |
| outdoor_temperature | 6.9153886 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 140.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 30%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 64.31344 |
| air_temperature | 19.858194 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 16.0 |
| month | 4.0 |
| outdoor_humidity | 86.0 |
| outdoor_temperature | 8.460917 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 60.0 |
| wind_speed | 7.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 31%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 68.07449 |
| air_temperature | 18.641197 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 16.0 |
| month | 4.0 |
| outdoor_humidity | 89.0 |
| outdoor_temperature | 5.7216463 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 240.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 31%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 60.987324 |
| air_temperature | 20.40382 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 21.0 |
| month | 4.0 |
| outdoor_humidity | 68.0 |
| outdoor_temperature | 10.535965 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 260.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 31%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 73.64444 |
| air_temperature | 20.592913 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 18.0 |
| month | 4.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 7.241513 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 160.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 31%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 84.43422 |
| air_temperature | 19.880894 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 20.0 |
| month | 4.0 |
| outdoor_humidity | 89.0 |
| outdoor_temperature | 10.051851 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 320.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 32%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 72.292465 |
| air_temperature | 20.055376 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 21.0 |
| month | 4.0 |
| outdoor_humidity | 90.0 |
| outdoor_temperature | 6.9597707 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 50.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 32%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 75.59889 |
| air_temperature | 21.129635 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 41.5 |
| direct_solar_radiation | 18.0 |
| hour | 5.0 |
| htg_setpoint | 22.0 |
| month | 4.0 |
| outdoor_humidity | 86.0 |
| outdoor_temperature | 10.039303 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 32%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 73.81694 |
| air_temperature | 21.566832 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 78.5 |
| direct_solar_radiation | 588.0 |
| hour | 6.0 |
| htg_setpoint | 21.0 |
| month | 4.0 |
| outdoor_humidity | 52.0 |
| outdoor_temperature | 12.721492 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 60.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 32%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 105.58764 |
| air_humidity | 68.87817 |
| air_temperature | 18.685684 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 221.0 |
| direct_solar_radiation | 50.5 |
| hour | 7.0 |
| htg_setpoint | 16.0 |
| month | 4.0 |
| outdoor_humidity | 71.0 |
| outdoor_temperature | 13.024408 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 95277.984 |
| wind_direction | 110.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 33%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 554.63495 |
| air_humidity | 60.94167 |
| air_temperature | 20.760542 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 133.5 |
| direct_solar_radiation | 0.0 |
| hour | 8.0 |
| htg_setpoint | 15.0 |
| month | 4.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 10.734792 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 499171.47 |
| wind_direction | 210.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 33%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 494.29865 |
| air_humidity | 26.91579 |
| air_temperature | 24.698816 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 227.5 |
| direct_solar_radiation | 676.0 |
| hour | 9.0 |
| htg_setpoint | 15.0 |
| month | 5.0 |
| outdoor_humidity | 25.0 |
| outdoor_temperature | 16.345572 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 441835.44 |
| wind_direction | 30.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 33%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 1669.414 |
| air_humidity | 27.572773 |
| air_temperature | 24.118586 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 263.5 |
| direct_solar_radiation | 662.0 |
| hour | 10.0 |
| htg_setpoint | 21.0 |
| month | 5.0 |
| outdoor_humidity | 35.0 |
| outdoor_temperature | 16.663635 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1502472.6 |
| wind_direction | 110.0 |
| wind_speed | 8.8 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 34%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1423.8939 |
| air_humidity | 49.750465 |
| air_temperature | 23.154497 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 225.0 |
| direct_solar_radiation | 0.0 |
| hour | 11.0 |
| htg_setpoint | 21.0 |
| month | 5.0 |
| outdoor_humidity | 74.0 |
| outdoor_temperature | 15.606137 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 1281504.5 |
| wind_direction | 30.0 |
| wind_speed | 9.3 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 34%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 69.523636 |
| air_temperature | 21.496876 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 183.0 |
| direct_solar_radiation | 0.0 |
| hour | 12.0 |
| htg_setpoint | 22.0 |
| month | 5.0 |
| outdoor_humidity | 83.0 |
| outdoor_temperature | 17.305464 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 20.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 34%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 60.981228 |
| air_temperature | 23.672033 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 296.0 |
| direct_solar_radiation | 101.0 |
| hour | 13.0 |
| htg_setpoint | 16.0 |
| month | 5.0 |
| outdoor_humidity | 73.0 |
| outdoor_temperature | 18.12477 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 160.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 34%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 263.9766 |
| air_humidity | 49.505367 |
| air_temperature | 24.917562 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 243.0 |
| direct_solar_radiation | 62.5 |
| hour | 14.0 |
| htg_setpoint | 16.0 |
| month | 5.0 |
| outdoor_humidity | 83.0 |
| outdoor_temperature | 14.965244 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 237583.92 |
| wind_direction | 110.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 35%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 441.78152 |
| air_humidity | 59.375774 |
| air_temperature | 25.219263 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 81.5 |
| direct_solar_radiation | 0.0 |
| hour | 15.0 |
| htg_setpoint | 18.0 |
| month | 5.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 15.51826 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 397603.38 |
| wind_direction | 80.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 35%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 291.60434 |
| air_humidity | 60.253563 |
| air_temperature | 24.001564 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 116.5 |
| direct_solar_radiation | 8.5 |
| hour | 16.0 |
| htg_setpoint | 21.0 |
| month | 5.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 14.3150015 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 426840.75 |
| wind_direction | 130.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 35%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 47.826717 |
| air_temperature | 29.443428 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 66.0 |
| direct_solar_radiation | 76.5 |
| hour | 17.0 |
| htg_setpoint | 21.0 |
| month | 5.0 |
| outdoor_humidity | 44.0 |
| outdoor_temperature | 24.326218 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 270.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 35%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 41.45334 |
| air_temperature | 29.770283 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 22.0 |
| month | 5.0 |
| outdoor_humidity | 33.0 |
| outdoor_temperature | 21.403486 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 320.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 36%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 56.864246 |
| air_temperature | 25.409126 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 20.0 |
| month | 5.0 |
| outdoor_humidity | 77.0 |
| outdoor_temperature | 14.024936 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 36%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 56.58497 |
| air_temperature | 25.376099 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 21.0 |
| month | 5.0 |
| outdoor_humidity | 83.0 |
| outdoor_temperature | 13.580284 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 36%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 51.845596 |
| air_temperature | 24.4386 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 20.0 |
| month | 5.0 |
| outdoor_humidity | 77.0 |
| outdoor_temperature | 8.686298 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 90.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*--------------------------------------------------------------| 37%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 60.85383 |
| air_temperature | 23.408401 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 19.0 |
| month | 5.0 |
| outdoor_humidity | 68.0 |
| outdoor_temperature | 10.669418 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*--------------------------------------------------------------| 37%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 64.44686 |
| air_temperature | 22.478897 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 16.0 |
| month | 5.0 |
| outdoor_humidity | 63.0 |
| outdoor_temperature | 11.021046 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 30.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 37%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 69.71836 |
| air_temperature | 22.096245 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 17.0 |
| month | 5.0 |
| outdoor_humidity | 83.0 |
| outdoor_temperature | 10.4822035 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 40.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
------------------------------------------------
-----------------------------------------------**-------------------------------------------------------------| 38%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 75.24246 |
| air_temperature | 22.697903 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 22.0 |
| month | 5.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 14.038016 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 10.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------**-------------------------------------------------------------| 38%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 79.10041 |
| air_temperature | 21.779947 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 16.0 |
| month | 5.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 15.543995 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 90.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------**-------------------------------------------------------------| 38%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 85.470825 |
| air_temperature | 20.502432 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 18.0 |
| month | 5.0 |
| outdoor_humidity | 84.0 |
| outdoor_temperature | 14.700728 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 330.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------**-------------------------------------------------------------| 38%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 69.664185 |
| air_temperature | 20.969315 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 39.5 |
| direct_solar_radiation | 78.0 |
| hour | 4.0 |
| htg_setpoint | 21.0 |
| month | 5.0 |
| outdoor_humidity | 59.0 |
| outdoor_temperature | 11.760994 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 320.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------***------------------------------------------------------------| 39%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 76.82759 |
| air_temperature | 20.638756 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 119.5 |
| direct_solar_radiation | 39.5 |
| hour | 5.0 |
| htg_setpoint | 16.0 |
| month | 5.0 |
| outdoor_humidity | 78.0 |
| outdoor_temperature | 12.853281 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 30.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
------------------------------------------------**------------------------------------------------------------| 39%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 79.742096 |
| air_temperature | 20.965656 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 202.5 |
| direct_solar_radiation | 121.5 |
| hour | 6.0 |
| htg_setpoint | 19.0 |
| month | 5.0 |
| outdoor_humidity | 97.0 |
| outdoor_temperature | 15.2559595 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 120.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
------------------------------------------------
-----------------------------------------------***------------------------------------------------------------| 39%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 175.05946 |
| air_humidity | 85.569885 |
| air_temperature | 20.361977 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 166.5 |
| direct_solar_radiation | 3.0 |
| hour | 7.0 |
| htg_setpoint | 17.0 |
| month | 5.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 15.519688 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 157553.52 |
| wind_direction | 110.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------****-----------------------------------------------------------| 40%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 88.35559 |
| air_temperature | 23.295433 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 251.5 |
| direct_solar_radiation | 597.5 |
| hour | 8.0 |
| htg_setpoint | 15.0 |
| month | 5.0 |
| outdoor_humidity | 72.0 |
| outdoor_temperature | 16.08074 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 180.0 |
| wind_speed | 8.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------****-----------------------------------------------------------| 40%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 82.33773 |
| air_temperature | 24.468657 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 330.5 |
| direct_solar_radiation | 485.5 |
| hour | 9.0 |
| htg_setpoint | 21.0 |
| month | 5.0 |
| outdoor_humidity | 44.0 |
| outdoor_temperature | 21.730036 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 230.0 |
| wind_speed | 11.3 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------****-----------------------------------------------------------| 40%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 3473.914 |
| air_humidity | 50.87384 |
| air_temperature | 23.874031 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 396.0 |
| direct_solar_radiation | 283.0 |
| hour | 10.0 |
| htg_setpoint | 21.0 |
| month | 5.0 |
| outdoor_humidity | 50.0 |
| outdoor_temperature | 19.557518 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 3113888.5 |
| wind_direction | 300.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------****-----------------------------------------------------------| 40%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 4798.8096 |
| air_humidity | 45.506927 |
| air_temperature | 24.022305 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 333.0 |
| direct_solar_radiation | 576.0 |
| hour | 11.0 |
| htg_setpoint | 21.0 |
| month | 5.0 |
| outdoor_humidity | 33.0 |
| outdoor_temperature | 25.908749 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 4307226.5 |
| wind_direction | 300.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*****----------------------------------------------------------| 41%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 4961.3433 |
| air_humidity | 53.536194 |
| air_temperature | 23.69982 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 270.0 |
| direct_solar_radiation | 682.5 |
| hour | 12.0 |
| htg_setpoint | 22.0 |
| month | 5.0 |
| outdoor_humidity | 35.0 |
| outdoor_temperature | 27.257017 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 4465209.0 |
| wind_direction | 180.0 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*****----------------------------------------------------------| 41%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 5542.6147 |
| air_humidity | 46.478977 |
| air_temperature | 24.297695 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 213.0 |
| direct_solar_radiation | 710.0 |
| hour | 13.0 |
| htg_setpoint | 22.0 |
| month | 5.0 |
| outdoor_humidity | 27.0 |
| outdoor_temperature | 28.636808 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 4984936.0 |
| wind_direction | 190.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*****----------------------------------------------------------| 41%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 5475.4956 |
| air_humidity | 57.36404 |
| air_temperature | 24.47104 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 233.5 |
| direct_solar_radiation | 564.5 |
| hour | 14.0 |
| htg_setpoint | 18.0 |
| month | 5.0 |
| outdoor_humidity | 54.0 |
| outdoor_temperature | 26.003632 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 4915775.5 |
| wind_direction | 160.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*****----------------------------------------------------------| 41%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 61.53134 |
| air_temperature | 27.312569 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 178.5 |
| direct_solar_radiation | 469.5 |
| hour | 15.0 |
| htg_setpoint | 21.0 |
| month | 6.0 |
| outdoor_humidity | 27.0 |
| outdoor_temperature | 27.807915 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 290.0 |
| wind_speed | 8.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------******---------------------------------------------------------| 42%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 62.3855 |
| air_temperature | 27.161943 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 103.0 |
| direct_solar_radiation | 524.0 |
| hour | 16.0 |
| htg_setpoint | 15.0 |
| month | 6.0 |
| outdoor_humidity | 35.0 |
| outdoor_temperature | 20.942236 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 330.0 |
| wind_speed | 7.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------******---------------------------------------------------------| 42%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 39.917088 |
| air_temperature | 30.10571 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 62.0 |
| direct_solar_radiation | 335.0 |
| hour | 17.0 |
| htg_setpoint | 21.0 |
| month | 6.0 |
| outdoor_humidity | 63.0 |
| outdoor_temperature | 17.514065 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 210.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------******---------------------------------------------------------| 42%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 47.715076 |
| air_temperature | 29.770834 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 28.5 |
| direct_solar_radiation | 11.5 |
| hour | 18.0 |
| htg_setpoint | 15.0 |
| month | 6.0 |
| outdoor_humidity | 56.0 |
| outdoor_temperature | 18.896332 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*******--------------------------------------------------------| 43%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 60.85728 |
| air_temperature | 27.400461 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 20.0 |
| month | 6.0 |
| outdoor_humidity | 78.0 |
| outdoor_temperature | 16.325865 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 170.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
-----------------------------------------------
-----------------------------------------------*******--------------------------------------------------------| 43%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 71.10968 |
| air_temperature | 25.510035 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 20.0 |
| month | 6.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 20.222506 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.73e+04 |
| n_updates | 24 |
-----------------------------------------------
-----------------------------------------------*******--------------------------------------------------------| 43%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 68.88185 |
| air_temperature | 26.473099 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 19.0 |
| month | 6.0 |
| outdoor_humidity | 57.0 |
| outdoor_temperature | 23.432787 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 40.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.69e+04 |
| n_updates | 49 |
-----------------------------------------------
-----------------------------------------------*******--------------------------------------------------------| 43%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 77.250916 |
| air_temperature | 24.624996 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 15.0 |
| month | 6.0 |
| outdoor_humidity | 50.0 |
| outdoor_temperature | 19.427858 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 320.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.96e+04 |
| n_updates | 74 |
-----------------------------------------------
-----------------------------------------------********-------------------------------------------------------| 44%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 78.379234 |
| air_temperature | 24.415615 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 20.0 |
| month | 6.0 |
| outdoor_humidity | 70.0 |
| outdoor_temperature | 18.959192 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 250.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.74e+04 |
| n_updates | 99 |
-----------------------------------------------
-----------------------------------------------********-------------------------------------------------------| 44%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 70.947784 |
| air_temperature | 22.702192 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 20.0 |
| month | 6.0 |
| outdoor_humidity | 90.0 |
| outdoor_temperature | 16.782215 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 120.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.7e+04 |
| n_updates | 124 |
-----------------------------------------------
-----------------------------------------------********-------------------------------------------------------| 44%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 90.11013 |
| air_temperature | 22.181059 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 20.0 |
| month | 6.0 |
| outdoor_humidity | 90.0 |
| outdoor_temperature | 17.962767 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 180.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.4e+04 |
| n_updates | 149 |
-----------------------------------------------
-----------------------------------------------*********------------------------------------------------------| 45%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 88.01869 |
| air_temperature | 22.351809 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 20.0 |
| month | 6.0 |
| outdoor_humidity | 97.0 |
| outdoor_temperature | 20.203293 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 200.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.25e+04 |
| n_updates | 174 |
-----------------------------------------------
-----------------------------------------------*********------------------------------------------------------| 45%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 89.761375 |
| air_temperature | 21.895535 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 20.0 |
| month | 6.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 17.647366 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 160.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.48e+04 |
| n_updates | 199 |
-----------------------------------------------
-----------------------------------------------*********------------------------------------------------------| 45%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 86.31044 |
| air_temperature | 23.202625 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 49.0 |
| direct_solar_radiation | 3.0 |
| hour | 4.0 |
| htg_setpoint | 21.0 |
| month | 6.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 21.554924 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 100.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.79e+03 |
| n_updates | 224 |
-----------------------------------------------
-----------------------------------------------**********-----------------------------------------------------| 46%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 84.13197 |
| air_temperature | 23.67505 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 41.5 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 21.0 |
| month | 6.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 23.571669 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 50.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 8.94e+03 |
| n_updates | 249 |
-----------------------------------------------
-----------------------------------------------**********-----------------------------------------------------| 46%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 93.96759 |
| air_temperature | 21.819794 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 83.0 |
| direct_solar_radiation | 0.0 |
| hour | 6.0 |
| htg_setpoint | 15.0 |
| month | 6.0 |
| outdoor_humidity | 90.0 |
| outdoor_temperature | 19.235605 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 180.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.99e+03 |
| n_updates | 274 |
-----------------------------------------------
-----------------------------------------------**********-----------------------------------------------------| 46%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 1854.7126 |
| air_humidity | 82.8579 |
| air_temperature | 19.94703 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 116.0 |
| direct_solar_radiation | 0.0 |
| hour | 7.0 |
| htg_setpoint | 16.0 |
| month | 6.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 19.453636 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1662493.1 |
| wind_direction | 260.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.25e+03 |
| n_updates | 299 |
-----------------------------------------------
-----------------------------------------------**********-----------------------------------------------------| 46%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 8928.022 |
| air_humidity | 64.12279 |
| air_temperature | 22.995796 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 172.0 |
| direct_solar_radiation | 645.5 |
| hour | 8.0 |
| htg_setpoint | 17.0 |
| month | 6.0 |
| outdoor_humidity | 54.0 |
| outdoor_temperature | 32.729115 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 8035220.5 |
| wind_direction | 280.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.26e+03 |
| n_updates | 324 |
-----------------------------------------------
-----------------------------------------------***********----------------------------------------------------| 47%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 6259.42 |
| air_humidity | 58.960857 |
| air_temperature | 23.959463 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 289.0 |
| direct_solar_radiation | 563.0 |
| hour | 9.0 |
| htg_setpoint | 22.0 |
| month | 6.0 |
| outdoor_humidity | 53.0 |
| outdoor_temperature | 27.882483 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 5633478.0 |
| wind_direction | 100.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.46e+03 |
| n_updates | 349 |
-----------------------------------------------
-----------------------------------------------***********----------------------------------------------------| 47%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 4542.525 |
| air_humidity | 61.181717 |
| air_temperature | 23.151531 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 300.0 |
| direct_solar_radiation | 0.0 |
| hour | 10.0 |
| htg_setpoint | 22.0 |
| month | 6.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 19.840796 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 4088272.2 |
| wind_direction | 180.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.69e+03 |
| n_updates | 374 |
-----------------------------------------------
-----------------------------------------------***********----------------------------------------------------| 47%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 66.28868 |
| air_temperature | 27.314816 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 189.0 |
| direct_solar_radiation | 684.0 |
| hour | 11.0 |
| htg_setpoint | 16.0 |
| month | 6.0 |
| outdoor_humidity | 33.0 |
| outdoor_temperature | 29.14851 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 10.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.48e+04 |
| n_updates | 399 |
-----------------------------------------------
-----------------------------------------------***********----------------------------------------------------| 47%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 65.29333 |
| air_temperature | 27.590292 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 362.0 |
| direct_solar_radiation | 368.0 |
| hour | 12.0 |
| htg_setpoint | 20.0 |
| month | 6.0 |
| outdoor_humidity | 35.0 |
| outdoor_temperature | 30.175938 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 220.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.64e+03 |
| n_updates | 424 |
-----------------------------------------------
-----------------------------------------------************---------------------------------------------------| 48%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 6536.5103 |
| air_humidity | 56.910072 |
| air_temperature | 24.24192 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 344.5 |
| direct_solar_radiation | 48.0 |
| hour | 13.0 |
| htg_setpoint | 17.0 |
| month | 6.0 |
| outdoor_humidity | 74.0 |
| outdoor_temperature | 26.203909 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 5882859.5 |
| wind_direction | 100.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.87e+03 |
| n_updates | 449 |
-----------------------------------------------
-----------------------------------------------************---------------------------------------------------| 48%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 8832.593 |
| air_humidity | 58.894646 |
| air_temperature | 24.245432 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 190.0 |
| direct_solar_radiation | 446.5 |
| hour | 14.0 |
| htg_setpoint | 22.0 |
| month | 6.0 |
| outdoor_humidity | 71.0 |
| outdoor_temperature | 28.251022 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 7949333.0 |
| wind_direction | 200.0 |
| wind_speed | 9.3 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.16e+03 |
| n_updates | 474 |
-----------------------------------------------
-----------------------------------------------************---------------------------------------------------| 48%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 5994.488 |
| air_humidity | 57.70945 |
| air_temperature | 24.269371 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 127.0 |
| direct_solar_radiation | 657.0 |
| hour | 15.0 |
| htg_setpoint | 20.0 |
| month | 6.0 |
| outdoor_humidity | 52.0 |
| outdoor_temperature | 26.748756 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 5394630.0 |
| wind_direction | 200.0 |
| wind_speed | 11.3 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.01e+03 |
| n_updates | 499 |
-----------------------------------------------
-----------------------------------------------*************--------------------------------------------------| 49%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 5868.593 |
| air_humidity | 59.702705 |
| air_temperature | 23.673388 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 117.0 |
| direct_solar_radiation | 8.5 |
| hour | 16.0 |
| htg_setpoint | 15.0 |
| month | 6.0 |
| outdoor_humidity | 76.0 |
| outdoor_temperature | 23.925201 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 5300543.0 |
| wind_direction | 190.0 |
| wind_speed | 10.3 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 9.08e+03 |
| n_updates | 524 |
-----------------------------------------------
-----------------------------------------------*************--------------------------------------------------| 49%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 49.323895 |
| air_temperature | 29.75314 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 89.0 |
| direct_solar_radiation | 182.0 |
| hour | 17.0 |
| htg_setpoint | 21.0 |
| month | 6.0 |
| outdoor_humidity | 73.0 |
| outdoor_temperature | 24.83241 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 230.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.49e+03 |
| n_updates | 549 |
-----------------------------------------------
-----------------------------------------------*************--------------------------------------------------| 49%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 71.10587 |
| air_temperature | 25.321625 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 29.0 |
| direct_solar_radiation | 29.5 |
| hour | 18.0 |
| htg_setpoint | 18.0 |
| month | 6.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | 19.11446 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 210.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.5e+03 |
| n_updates | 574 |
-----------------------------------------------
----------------------------------------------**************--------------------------------------------------| 49%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 73.58216 |
| air_temperature | 24.81363 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 16.0 |
| month | 6.0 |
| outdoor_humidity | 81.0 |
| outdoor_temperature | 19.323 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 230.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.13e+03 |
| n_updates | 599 |
----------------------------------------------
-----------------------------------------------**************-------------------------------------------------| 50%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 64.26106 |
| air_temperature | 27.285131 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 22.0 |
| month | 7.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | 19.510527 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 170.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.57e+03 |
| n_updates | 624 |
-----------------------------------------------
-----------------------------------------------**************-------------------------------------------------| 50%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 70.97226 |
| air_temperature | 25.634449 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 16.0 |
| month | 7.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | 20.972343 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.97e+03 |
| n_updates | 649 |
-----------------------------------------------
-----------------------------------------------**************-------------------------------------------------| 50%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 78.34892 |
| air_temperature | 24.954485 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 20.0 |
| month | 7.0 |
| outdoor_humidity | 85.0 |
| outdoor_temperature | 21.94895 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 120.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.54e+03 |
| n_updates | 674 |
-----------------------------------------------
-----------------------------------------------***************------------------------------------------------| 51%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 78.28426 |
| air_temperature | 24.376232 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 21.0 |
| month | 7.0 |
| outdoor_humidity | 90.0 |
| outdoor_temperature | 20.423975 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 8.99e+03 |
| n_updates | 699 |
-----------------------------------------------
-----------------------------------------------***************------------------------------------------------| 51%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 77.33825 |
| air_temperature | 24.507927 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 21.0 |
| month | 7.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | 23.793901 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 230.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.98e+03 |
| n_updates | 724 |
-----------------------------------------------
-----------------------------------------------***************------------------------------------------------| 51%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 73.71556 |
| air_temperature | 25.245878 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 22.0 |
| month | 7.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | 25.436129 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 220.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.81e+03 |
| n_updates | 749 |
-----------------------------------------------
-----------------------------------------------***************------------------------------------------------| 51%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 79.0488 |
| air_temperature | 24.126446 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 16.0 |
| month | 7.0 |
| outdoor_humidity | 88.0 |
| outdoor_temperature | 24.582035 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.78e+03 |
| n_updates | 774 |
-----------------------------------------------
-----------------------------------------------****************-----------------------------------------------| 52%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 87.71491 |
| air_temperature | 22.90714 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 22.0 |
| month | 7.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 22.584349 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 965 |
| n_updates | 799 |
-----------------------------------------------
-----------------------------------------------****************-----------------------------------------------| 52%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 93.72923 |
| air_temperature | 23.959242 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 25.5 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 20.0 |
| month | 7.0 |
| outdoor_humidity | 62.0 |
| outdoor_temperature | 27.785337 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 330.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.47e+03 |
| n_updates | 824 |
-----------------------------------------------
-----------------------------------------------****************-----------------------------------------------| 52%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 84.162704 |
| air_temperature | 23.086092 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 76.0 |
| direct_solar_radiation | 262.0 |
| hour | 5.0 |
| htg_setpoint | 21.0 |
| month | 7.0 |
| outdoor_humidity | 59.0 |
| outdoor_temperature | 21.191248 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 0.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.96e+03 |
| n_updates | 849 |
-----------------------------------------------
-----------------------------------------------*****************----------------------------------------------| 53%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 79.09719 |
| air_temperature | 22.966927 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 84.5 |
| direct_solar_radiation | 444.5 |
| hour | 6.0 |
| htg_setpoint | 15.0 |
| month | 7.0 |
| outdoor_humidity | 56.0 |
| outdoor_temperature | 25.49166 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.62e+03 |
| n_updates | 874 |
-----------------------------------------------
-----------------------------------------------*****************----------------------------------------------| 53%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 71.842354 |
| air_temperature | 24.912615 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 188.5 |
| direct_solar_radiation | 444.0 |
| hour | 7.0 |
| htg_setpoint | 22.0 |
| month | 7.0 |
| outdoor_humidity | 69.0 |
| outdoor_temperature | 27.981579 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 240.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.47e+03 |
| n_updates | 899 |
-----------------------------------------------
-----------------------------------------------*****************----------------------------------------------| 53%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 66.29015 |
| air_temperature | 26.284555 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 109.0 |
| direct_solar_radiation | 791.5 |
| hour | 8.0 |
| htg_setpoint | 19.0 |
| month | 7.0 |
| outdoor_humidity | 48.0 |
| outdoor_temperature | 27.723755 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 80.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.41e+03 |
| n_updates | 924 |
-----------------------------------------------
-----------------------------------------------*****************----------------------------------------------| 53%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 5701.992 |
| air_humidity | 62.445957 |
| air_temperature | 22.877975 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 198.0 |
| direct_solar_radiation | 0.0 |
| hour | 9.0 |
| htg_setpoint | 22.0 |
| month | 7.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | 22.844303 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 5131793.0 |
| wind_direction | 0.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 9.48e+03 |
| n_updates | 949 |
-----------------------------------------------
-----------------------------------------------******************---------------------------------------------| 54%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 6329.585 |
| air_humidity | 58.633747 |
| air_temperature | 24.058687 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 351.0 |
| direct_solar_radiation | 285.0 |
| hour | 10.0 |
| htg_setpoint | 19.0 |
| month | 7.0 |
| outdoor_humidity | 48.0 |
| outdoor_temperature | 29.750559 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 5696626.5 |
| wind_direction | 350.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.12e+03 |
| n_updates | 974 |
-----------------------------------------------
-----------------------------------------------******************---------------------------------------------| 54%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 7090.5063 |
| air_humidity | 57.64953 |
| air_temperature | 24.19502 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 229.5 |
| direct_solar_radiation | 590.5 |
| hour | 11.0 |
| htg_setpoint | 22.0 |
| month | 7.0 |
| outdoor_humidity | 59.0 |
| outdoor_temperature | 27.872087 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 6384696.5 |
| wind_direction | 190.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 8.12e+03 |
| n_updates | 999 |
-----------------------------------------------
-----------------------------------------------******************---------------------------------------------| 54%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 5688.2856 |
| air_humidity | 61.284252 |
| air_temperature | 22.551647 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 260.0 |
| direct_solar_radiation | 0.0 |
| hour | 12.0 |
| htg_setpoint | 21.0 |
| month | 7.0 |
| outdoor_humidity | 88.0 |
| outdoor_temperature | 22.795156 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 5119457.0 |
| wind_direction | 90.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.4e+03 |
| n_updates | 1024 |
-----------------------------------------------
-----------------------------------------------******************---------------------------------------------| 54%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 7841.701 |
| air_humidity | 57.82436 |
| air_temperature | 24.11316 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 301.5 |
| direct_solar_radiation | 544.5 |
| hour | 13.0 |
| htg_setpoint | 20.0 |
| month | 7.0 |
| outdoor_humidity | 72.0 |
| outdoor_temperature | 26.77382 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 7034948.0 |
| wind_direction | 200.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3e+03 |
| n_updates | 1049 |
-----------------------------------------------
-----------------------------------------------*******************--------------------------------------------| 55%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 62.2651 |
| air_temperature | 28.586027 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 332.0 |
| direct_solar_radiation | 416.0 |
| hour | 14.0 |
| htg_setpoint | 15.0 |
| month | 7.0 |
| outdoor_humidity | 79.0 |
| outdoor_temperature | 27.283964 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 180.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.07e+03 |
| n_updates | 1074 |
-----------------------------------------------
-----------------------------------------------*******************--------------------------------------------| 55%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 62.894302 |
| air_temperature | 28.345287 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 267.5 |
| direct_solar_radiation | 310.5 |
| hour | 15.0 |
| htg_setpoint | 15.0 |
| month | 7.0 |
| outdoor_humidity | 74.0 |
| outdoor_temperature | 25.579243 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 200.0 |
| wind_speed | 8.8 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.46e+03 |
| n_updates | 1099 |
-----------------------------------------------
-----------------------------------------------*******************--------------------------------------------| 55%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 7106.787 |
| air_humidity | 59.247807 |
| air_temperature | 23.88788 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 135.0 |
| direct_solar_radiation | 332.0 |
| hour | 16.0 |
| htg_setpoint | 20.0 |
| month | 7.0 |
| outdoor_humidity | 76.0 |
| outdoor_temperature | 25.603895 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 6396108.5 |
| wind_direction | 200.0 |
| wind_speed | 9.8 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.13e+03 |
| n_updates | 1124 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 56%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 52.444363 |
| air_temperature | 28.380396 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 52.5 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 21.0 |
| month | 7.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 21.795992 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 200.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.86e+03 |
| n_updates | 1149 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 56%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 52.730785 |
| air_temperature | 30.277372 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 21.0 |
| direct_solar_radiation | 82.5 |
| hour | 18.0 |
| htg_setpoint | 18.0 |
| month | 7.0 |
| outdoor_humidity | 60.0 |
| outdoor_temperature | 24.305302 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.09e+03 |
| n_updates | 1174 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 56%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 62.063698 |
| air_temperature | 28.064104 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 21.0 |
| month | 7.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | 22.021494 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 210.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.7e+03 |
| n_updates | 1199 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 56%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 70.99026 |
| air_temperature | 26.231157 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 19.0 |
| month | 7.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | 23.473911 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 180.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.4e+03 |
| n_updates | 1224 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 57%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 72.17343 |
| air_temperature | 25.960754 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 22.0 |
| month | 7.0 |
| outdoor_humidity | 90.0 |
| outdoor_temperature | 21.79974 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 80.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.6e+03 |
| n_updates | 1249 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 57%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 78.62412 |
| air_temperature | 24.571651 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 17.0 |
| month | 7.0 |
| outdoor_humidity | 71.0 |
| outdoor_temperature | 23.37696 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 280.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.05e+03 |
| n_updates | 1274 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 57%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 79.07557 |
| air_temperature | 24.251059 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 22.0 |
| month | 7.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 21.277117 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 180.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.37e+03 |
| n_updates | 1299 |
-----------------------------------------------
-----------------------------------------------*********************------------------------------------------| 58%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 82.77418 |
| air_temperature | 23.679039 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 17.0 |
| month | 7.0 |
| outdoor_humidity | 91.0 |
| outdoor_temperature | 23.076662 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 220.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 756 |
| n_updates | 1324 |
-----------------------------------------------
-----------------------------------------------*********************------------------------------------------| 58%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 83.70116 |
| air_temperature | 23.391863 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 16.0 |
| month | 8.0 |
| outdoor_humidity | 64.0 |
| outdoor_temperature | 23.102135 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 250.0 |
| wind_speed | 3.5 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.37e+03 |
| n_updates | 1349 |
-----------------------------------------------
-----------------------------------------------*********************------------------------------------------| 58%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 80.7172 |
| air_temperature | 23.848103 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 16.0 |
| month | 8.0 |
| outdoor_humidity | 77.0 |
| outdoor_temperature | 24.195494 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 250.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 891 |
| n_updates | 1374 |
-----------------------------------------------
-----------------------------------------------***********************----------------------------------------| 59%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 81.705536 |
| air_temperature | 24.714365 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 16.0 |
| month | 8.0 |
| outdoor_humidity | 85.0 |
| outdoor_temperature | 25.90795 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 250.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.09e+03 |
| n_updates | 1399 |
-----------------------------------------------
wandb: WARNING Step only supports monotonically increasing values, use define_metric to set a custom x axis. For details see: https://wandb.me/define-metric
wandb: WARNING (User provided step: 35040 is less than current step: 35041. Dropping entry: {'rollout/ep_len_mean': 35040.0, '_timestamp': 1700214247.6063654}).
wandb: WARNING (User provided step: 35040 is less than current step: 35041. Dropping entry: {'rollout/ep_rew_mean': -11781.918385, '_timestamp': 1700214247.6064198}).
wandb: WARNING (User provided step: 35040 is less than current step: 35041. Dropping entry: {'rollout/exploration_rate': 0.05, '_timestamp': 1700214247.606459}).
wandb: WARNING (User provided step: 35040 is less than current step: 35041. Dropping entry: {'time/episodes': 1, '_timestamp': 1700214247.6064918}).
wandb: WARNING (User provided step: 35040 is less than current step: 35041. Dropping entry: {'time/fps': 1779, '_timestamp': 1700214247.6065187}).
wandb: WARNING (User provided step: 35040 is less than current step: 35041. Dropping entry: {'time/time_elapsed': 19, '_timestamp': 1700214247.6065419}).
wandb: WARNING (User provided step: 35040 is less than current step: 35041. Dropping entry: {'time/total_timesteps': 35040, '_timestamp': 1700214247.6065679}).
-----------------------------------------------***********************----------------------------------------| 59%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 83.14272 |
| air_temperature | 24.373743 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 16.5 |
| direct_solar_radiation | 30.0 |
| hour | 4.0 |
| htg_setpoint | 22.0 |
| month | 8.0 |
| outdoor_humidity | 94.0 |
| outdoor_temperature | 24.067757 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 210.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.97e+03 |
| n_updates | 1424 |
-----------------------------------------------
-----------------------------------------------***********************----------------------------------------| 59%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 84.859985 |
| air_temperature | 23.91815 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 71.0 |
| direct_solar_radiation | 21.0 |
| hour | 5.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 76.0 |
| outdoor_temperature | 25.750177 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 20.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.66e+03 |
| n_updates | 1449 |
-----------------------------------------------
-----------------------------------------------***********************----------------------------------------| 59%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 87.054276 |
| air_temperature | 22.867567 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 58.5 |
| direct_solar_radiation | 0.0 |
| hour | 6.0 |
| htg_setpoint | 22.0 |
| month | 8.0 |
| outdoor_humidity | 94.0 |
| outdoor_temperature | 22.91997 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 40.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.85e+03 |
| n_updates | 1474 |
-----------------------------------------------
-----------------------------------------------************************---------------------------------------| 60%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1521.9896 |
| air_humidity | 75.063614 |
| air_temperature | 21.000452 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 205.5 |
| direct_solar_radiation | 112.5 |
| hour | 7.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 76.0 |
| outdoor_temperature | 19.201933 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1378782.4 |
| wind_direction | 30.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.7e+03 |
| n_updates | 1499 |
-----------------------------------------------
-----------------------------------------------************************---------------------------------------| 60%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 3598.6003 |
| air_humidity | 65.23126 |
| air_temperature | 22.027729 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 182.0 |
| direct_solar_radiation | 648.5 |
| hour | 8.0 |
| htg_setpoint | 18.0 |
| month | 8.0 |
| outdoor_humidity | 50.0 |
| outdoor_temperature | 24.144733 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 3229639.5 |
| wind_direction | 80.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.07e+04 |
| n_updates | 1524 |
-----------------------------------------------
-----------------------------------------------************************---------------------------------------| 60%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 3934.8455 |
| air_humidity | 58.38924 |
| air_temperature | 23.422209 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 310.5 |
| direct_solar_radiation | 375.5 |
| hour | 9.0 |
| htg_setpoint | 16.0 |
| month | 8.0 |
| outdoor_humidity | 39.0 |
| outdoor_temperature | 26.24799 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 3516591.0 |
| wind_direction | 160.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.85e+03 |
| n_updates | 1549 |
-----------------------------------------------
-----------------------------------------------************************---------------------------------------| 60%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 66.500626 |
| air_temperature | 26.288742 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 156.0 |
| direct_solar_radiation | 685.0 |
| hour | 10.0 |
| htg_setpoint | 20.0 |
| month | 8.0 |
| outdoor_humidity | 67.0 |
| outdoor_temperature | 26.0001 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.36e+03 |
| n_updates | 1574 |
-----------------------------------------------
-----------------------------------------------*************************--------------------------------------| 61%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 64.475006 |
| air_temperature | 26.78031 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 356.5 |
| direct_solar_radiation | 509.0 |
| hour | 11.0 |
| htg_setpoint | 17.0 |
| month | 8.0 |
| outdoor_humidity | 63.0 |
| outdoor_temperature | 27.915373 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.96e+03 |
| n_updates | 1599 |
-----------------------------------------------
-----------------------------------------------*************************--------------------------------------| 61%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 6470.7974 |
| air_humidity | 55.524296 |
| air_temperature | 24.516663 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 422.0 |
| direct_solar_radiation | 107.5 |
| hour | 12.0 |
| htg_setpoint | 18.0 |
| month | 8.0 |
| outdoor_humidity | 67.0 |
| outdoor_temperature | 29.62802 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 5823717.5 |
| wind_direction | 180.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.38e+03 |
| n_updates | 1624 |
-----------------------------------------------
-----------------------------------------------*************************--------------------------------------| 61%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 5735.0757 |
| air_humidity | 55.520737 |
| air_temperature | 24.371014 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 273.0 |
| direct_solar_radiation | 581.5 |
| hour | 13.0 |
| htg_setpoint | 20.0 |
| month | 8.0 |
| outdoor_humidity | 33.0 |
| outdoor_temperature | 31.163658 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 5156594.5 |
| wind_direction | 20.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.22e+03 |
| n_updates | 1649 |
-----------------------------------------------
-----------------------------------------------**************************-------------------------------------| 62%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 7858.42 |
| air_humidity | 57.489624 |
| air_temperature | 24.390175 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 262.0 |
| direct_solar_radiation | 375.5 |
| hour | 14.0 |
| htg_setpoint | 22.0 |
| month | 8.0 |
| outdoor_humidity | 65.0 |
| outdoor_temperature | 27.542755 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 7072577.5 |
| wind_direction | 180.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.03e+03 |
| n_updates | 1674 |
-----------------------------------------------
-----------------------------------------------**************************-------------------------------------| 62%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 8580.111 |
| air_humidity | 57.767204 |
| air_temperature | 24.379463 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 83.0 |
| direct_solar_radiation | 731.0 |
| hour | 15.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 58.0 |
| outdoor_temperature | 29.715965 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 7714040.5 |
| wind_direction | 150.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.01e+03 |
| n_updates | 1699 |
-----------------------------------------------
-----------------------------------------------**************************-------------------------------------| 62%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 9275.7295 |
| air_humidity | 62.564636 |
| air_temperature | 25.44268 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 127.0 |
| direct_solar_radiation | 260.5 |
| hour | 16.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 65.0 |
| outdoor_temperature | 30.933622 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 8348156.5 |
| wind_direction | 140.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.51e+03 |
| n_updates | 1724 |
-----------------------------------------------
-----------------------------------------------**************************-------------------------------------| 62%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 72.23363 |
| air_temperature | 28.494257 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 52.5 |
| direct_solar_radiation | 14.5 |
| hour | 17.0 |
| htg_setpoint | 16.0 |
| month | 8.0 |
| outdoor_humidity | 61.0 |
| outdoor_temperature | 30.450134 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 50.0 |
| wind_speed | 7.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.09e+03 |
| n_updates | 1749 |
-----------------------------------------------
-----------------------------------------------***************************------------------------------------| 63%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 68.8516 |
| air_temperature | 29.39136 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 16.0 |
| month | 8.0 |
| outdoor_humidity | 60.0 |
| outdoor_temperature | 25.882673 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 100.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.12e+03 |
| n_updates | 1774 |
-----------------------------------------------
-----------------------------------------------***************************------------------------------------| 63%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 56.655693 |
| air_temperature | 28.701569 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 64.0 |
| outdoor_temperature | 22.626251 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 90.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.82e+03 |
| n_updates | 1799 |
-----------------------------------------------
-----------------------------------------------***************************------------------------------------| 63%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 62.070488 |
| air_temperature | 27.431053 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 81.0 |
| outdoor_temperature | 21.76395 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 230.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.24e+03 |
| n_updates | 1824 |
-----------------------------------------------
-----------------------------------------------***************************------------------------------------| 63%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 70.41705 |
| air_temperature | 27.251905 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 16.0 |
| month | 8.0 |
| outdoor_humidity | 69.0 |
| outdoor_temperature | 28.404594 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 240.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.03e+03 |
| n_updates | 1849 |
-----------------------------------------------
-----------------------------------------------****************************-----------------------------------| 64%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 62.179195 |
| air_temperature | 25.328423 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 18.0 |
| month | 8.0 |
| outdoor_humidity | 40.0 |
| outdoor_temperature | 21.58744 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 350.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.1e+03 |
| n_updates | 1874 |
-----------------------------------------------
-----------------------------------------------****************************-----------------------------------| 64%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 77.25168 |
| air_temperature | 24.274105 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 17.0 |
| month | 8.0 |
| outdoor_humidity | 73.0 |
| outdoor_temperature | 21.448235 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 220.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.17e+04 |
| n_updates | 1899 |
-----------------------------------------------
-----------------------------------------------*****************************----------------------------------| 65%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 72.27385 |
| air_temperature | 25.37339 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 18.0 |
| month | 8.0 |
| outdoor_humidity | 42.0 |
| outdoor_temperature | 21.546675 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 350.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.33e+03 |
| n_updates | 1924 |
-----------------------------------------------
-----------------------------------------------*****************************----------------------------------| 65%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 80.70976 |
| air_temperature | 23.610817 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 20.0 |
| month | 8.0 |
| outdoor_humidity | 65.0 |
| outdoor_temperature | 18.41828 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 50.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 7.6e+03 |
| n_updates | 1949 |
-----------------------------------------------
-----------------------------------------------*****************************----------------------------------| 65%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 88.37996 |
| air_temperature | 22.81223 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 17.0 |
| month | 8.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | 21.526308 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 240.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.1e+04 |
| n_updates | 1974 |
-----------------------------------------------
-----------------------------------------------*****************************----------------------------------| 65%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 87.88873 |
| air_temperature | 22.679972 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 15.0 |
| month | 8.0 |
| outdoor_humidity | 78.0 |
| outdoor_temperature | 18.887882 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 40.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.85e+03 |
| n_updates | 1999 |
-----------------------------------------------
-----------------------------------------------******************************---------------------------------| 66%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 85.374725 |
| air_temperature | 21.886457 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 15.0 |
| month | 8.0 |
| outdoor_humidity | 78.0 |
| outdoor_temperature | 16.744215 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 40.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.91e+03 |
| n_updates | 2024 |
-----------------------------------------------
-----------------------------------------------******************************---------------------------------| 66%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 86.8004 |
| air_temperature | 22.291016 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 37.0 |
| direct_solar_radiation | 165.0 |
| hour | 5.0 |
| htg_setpoint | 20.0 |
| month | 8.0 |
| outdoor_humidity | 65.0 |
| outdoor_temperature | 19.125746 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 20.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.76e+03 |
| n_updates | 2049 |
-----------------------------------------------
-----------------------------------------------******************************---------------------------------| 66%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 61.356754 |
| air_temperature | 23.098063 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 101.0 |
| direct_solar_radiation | 282.5 |
| hour | 6.0 |
| htg_setpoint | 15.0 |
| month | 8.0 |
| outdoor_humidity | 79.0 |
| outdoor_temperature | 22.395697 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 260.0 |
| wind_speed | 1.5 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.03e+03 |
| n_updates | 2074 |
-----------------------------------------------
-----------------------------------------------******************************---------------------------------| 66%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 56.98781 |
| air_temperature | 24.176632 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 94.0 |
| direct_solar_radiation | 588.0 |
| hour | 7.0 |
| htg_setpoint | 18.0 |
| month | 9.0 |
| outdoor_humidity | 56.0 |
| outdoor_temperature | 24.20977 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 320.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.64e+03 |
| n_updates | 2099 |
-----------------------------------------------
-----------------------------------------------*******************************--------------------------------| 67%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 2963.5293 |
| air_humidity | 59.67123 |
| air_temperature | 22.155758 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 234.5 |
| direct_solar_radiation | 275.5 |
| hour | 8.0 |
| htg_setpoint | 20.0 |
| month | 9.0 |
| outdoor_humidity | 55.0 |
| outdoor_temperature | 20.741188 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 2657805.0 |
| wind_direction | 20.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.34e+03 |
| n_updates | 2124 |
-----------------------------------------------
-----------------------------------------------*******************************--------------------------------| 67%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 3690.9102 |
| air_humidity | 49.598965 |
| air_temperature | 23.265598 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 181.0 |
| direct_solar_radiation | 702.0 |
| hour | 9.0 |
| htg_setpoint | 21.0 |
| month | 9.0 |
| outdoor_humidity | 37.0 |
| outdoor_temperature | 22.536015 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 3293101.2 |
| wind_direction | 50.0 |
| wind_speed | 1.5 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.84e+03 |
| n_updates | 2149 |
-----------------------------------------------
-----------------------------------------------*******************************--------------------------------| 67%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 4099.727 |
| air_humidity | 57.16755 |
| air_temperature | 23.969181 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 159.0 |
| direct_solar_radiation | 780.0 |
| hour | 10.0 |
| htg_setpoint | 22.0 |
| month | 9.0 |
| outdoor_humidity | 45.0 |
| outdoor_temperature | 23.952784 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 3676153.8 |
| wind_direction | 200.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.06e+03 |
| n_updates | 2174 |
-----------------------------------------------
-----------------------------------------------********************************-------------------------------| 68%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 4844.1577 |
| air_humidity | 58.401505 |
| air_temperature | 23.993696 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 166.5 |
| direct_solar_radiation | 795.0 |
| hour | 11.0 |
| htg_setpoint | 15.0 |
| month | 9.0 |
| outdoor_humidity | 58.0 |
| outdoor_temperature | 26.339314 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 4359742.0 |
| wind_direction | 200.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.43e+03 |
| n_updates | 2199 |
-----------------------------------------------
-----------------------------------------------********************************-------------------------------| 68%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 3168.1628 |
| air_humidity | 56.45405 |
| air_temperature | 24.310589 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 165.0 |
| direct_solar_radiation | 565.5 |
| hour | 12.0 |
| htg_setpoint | 18.0 |
| month | 9.0 |
| outdoor_humidity | 56.0 |
| outdoor_temperature | 25.505985 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 2851346.5 |
| wind_direction | 190.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.81e+03 |
| n_updates | 2224 |
-----------------------------------------------
-----------------------------------------------********************************-------------------------------| 68%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 66.15802 |
| air_temperature | 26.812828 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 148.0 |
| direct_solar_radiation | 730.5 |
| hour | 13.0 |
| htg_setpoint | 22.0 |
| month | 9.0 |
| outdoor_humidity | 74.0 |
| outdoor_temperature | 27.384958 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 180.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.59e+03 |
| n_updates | 2249 |
-----------------------------------------------
-----------------------------------------------********************************-------------------------------| 68%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 66.70742 |
| air_temperature | 26.722502 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 150.0 |
| direct_solar_radiation | 0.0 |
| hour | 14.0 |
| htg_setpoint | 22.0 |
| month | 9.0 |
| outdoor_humidity | 78.0 |
| outdoor_temperature | 21.76044 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 60.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.26e+03 |
| n_updates | 2274 |
-----------------------------------------------
-----------------------------------------------*********************************------------------------------| 69%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 4615.9355 |
| air_humidity | 59.687176 |
| air_temperature | 23.604136 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 102.5 |
| direct_solar_radiation | 0.0 |
| hour | 15.0 |
| htg_setpoint | 16.0 |
| month | 9.0 |
| outdoor_humidity | 76.0 |
| outdoor_temperature | 22.033457 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 4154342.0 |
| wind_direction | 150.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.18e+03 |
| n_updates | 2299 |
-----------------------------------------------
-----------------------------------------------*********************************------------------------------| 69%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 4519.266 |
| air_humidity | 39.110023 |
| air_temperature | 23.892767 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 43.0 |
| direct_solar_radiation | 499.5 |
| hour | 16.0 |
| htg_setpoint | 22.0 |
| month | 9.0 |
| outdoor_humidity | 29.0 |
| outdoor_temperature | 22.987827 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 4067339.8 |
| wind_direction | 330.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.94e+03 |
| n_updates | 2324 |
-----------------------------------------------
-----------------------------------------------*********************************------------------------------| 69%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 36.519215 |
| air_temperature | 29.639103 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 22.0 |
| direct_solar_radiation | 50.5 |
| hour | 17.0 |
| htg_setpoint | 20.0 |
| month | 9.0 |
| outdoor_humidity | 44.0 |
| outdoor_temperature | 19.65552 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 170.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.31e+03 |
| n_updates | 2349 |
-----------------------------------------------
-----------------------------------------------*********************************------------------------------| 69%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 52.53205 |
| air_temperature | 28.84091 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 19.0 |
| month | 9.0 |
| outdoor_humidity | 79.0 |
| outdoor_temperature | 21.706072 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 180.0 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.33e+03 |
| n_updates | 2374 |
-----------------------------------------------
-----------------------------------------------**********************************-----------------------------| 70%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 65.03585 |
| air_temperature | 28.089901 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 18.0 |
| month | 9.0 |
| outdoor_humidity | 88.0 |
| outdoor_temperature | 24.84705 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 210.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.56e+03 |
| n_updates | 2399 |
-----------------------------------------------
-----------------------------------------------**********************************-----------------------------| 70%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 73.22182 |
| air_temperature | 26.433327 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 18.0 |
| month | 9.0 |
| outdoor_humidity | 50.0 |
| outdoor_temperature | 26.2683 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 0.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 9.75e+03 |
| n_updates | 2424 |
-----------------------------------------------
-----------------------------------------------**********************************-----------------------------| 70%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 81.19492 |
| air_temperature | 24.888763 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 21.0 |
| month | 9.0 |
| outdoor_humidity | 52.0 |
| outdoor_temperature | 17.709038 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 50.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 570 |
| n_updates | 2449 |
-----------------------------------------------
-----------------------------------------------***********************************----------------------------| 71%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 77.14824 |
| air_temperature | 23.543236 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 17.0 |
| month | 9.0 |
| outdoor_humidity | 76.0 |
| outdoor_temperature | 19.323315 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 150.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.74e+03 |
| n_updates | 2474 |
-----------------------------------------------
-----------------------------------------------***********************************----------------------------| 71%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 83.54942 |
| air_temperature | 22.843735 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 20.0 |
| month | 9.0 |
| outdoor_humidity | 84.0 |
| outdoor_temperature | 17.800474 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 10.0 |
| wind_speed | 1.5 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.49e+03 |
| n_updates | 2499 |
-----------------------------------------------
-----------------------------------------------***********************************----------------------------| 71%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 75.72044 |
| air_temperature | 22.46577 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 15.0 |
| month | 9.0 |
| outdoor_humidity | 60.0 |
| outdoor_temperature | 15.107563 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 20.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.3e+03 |
| n_updates | 2524 |
-----------------------------------------------
-----------------------------------------------************************************---------------------------| 72%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 88.59096 |
| air_temperature | 22.127878 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 17.0 |
| month | 9.0 |
| outdoor_humidity | 84.0 |
| outdoor_temperature | 13.45269 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 50.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.05e+04 |
| n_updates | 2549 |
-----------------------------------------------
-----------------------------------------------************************************---------------------------| 72%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 84.00199 |
| air_temperature | 23.281334 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 16.0 |
| month | 9.0 |
| outdoor_humidity | 84.0 |
| outdoor_temperature | 19.630474 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 230.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.74e+03 |
| n_updates | 2574 |
-----------------------------------------------
-----------------------------------------------************************************---------------------------| 72%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 83.08445 |
| air_temperature | 23.414421 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 22.0 |
| month | 9.0 |
| outdoor_humidity | 90.0 |
| outdoor_temperature | 22.659573 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 210.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.85e+03 |
| n_updates | 2599 |
-----------------------------------------------
-----------------------------------------------************************************---------------------------| 72%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 95.95372 |
| air_temperature | 21.113327 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 17.0 |
| month | 9.0 |
| outdoor_humidity | 47.0 |
| outdoor_temperature | 14.836081 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 340.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.24e+04 |
| n_updates | 2624 |
-----------------------------------------------
-----------------------------------------------*************************************--------------------------| 73%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 74.788414 |
| air_temperature | 20.551414 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 18.5 |
| direct_solar_radiation | 158.5 |
| hour | 5.0 |
| htg_setpoint | 21.0 |
| month | 9.0 |
| outdoor_humidity | 83.0 |
| outdoor_temperature | 9.522072 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 40.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.68e+03 |
| n_updates | 2649 |
-----------------------------------------------
-----------------------------------------------*************************************--------------------------| 73%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 74.74622 |
| air_temperature | 20.638317 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 57.0 |
| direct_solar_radiation | 16.5 |
| hour | 6.0 |
| htg_setpoint | 17.0 |
| month | 9.0 |
| outdoor_humidity | 81.0 |
| outdoor_temperature | 15.213166 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 70.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.12e+03 |
| n_updates | 2674 |
-----------------------------------------------
-----------------------------------------------*************************************--------------------------| 73%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 82.323364 |
| air_temperature | 19.57355 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 87.0 |
| direct_solar_radiation | 0.0 |
| hour | 7.0 |
| htg_setpoint | 15.0 |
| month | 9.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 14.822417 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 350.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.52e+03 |
| n_updates | 2699 |
-----------------------------------------------
-----------------------------------------------**************************************-------------------------| 74%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 572.085 |
| air_humidity | 66.755165 |
| air_temperature | 22.665863 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 180.0 |
| direct_solar_radiation | 35.5 |
| hour | 8.0 |
| htg_setpoint | 20.0 |
| month | 9.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | 16.186356 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 514876.53 |
| wind_direction | 260.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 9.45e+03 |
| n_updates | 2724 |
-----------------------------------------------
-----------------------------------------------**************************************-------------------------| 74%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 81.31932 |
| air_temperature | 23.860435 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 121.0 |
| direct_solar_radiation | 726.5 |
| hour | 9.0 |
| htg_setpoint | 15.0 |
| month | 9.0 |
| outdoor_humidity | 46.0 |
| outdoor_temperature | 22.112099 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 20.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.63e+03 |
| n_updates | 2749 |
-----------------------------------------------
-----------------------------------------------**************************************-------------------------| 74%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 83.81175 |
| air_temperature | 23.48872 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 172.0 |
| direct_solar_radiation | 689.5 |
| hour | 10.0 |
| htg_setpoint | 22.0 |
| month | 9.0 |
| outdoor_humidity | 45.0 |
| outdoor_temperature | 18.881742 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 120.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.67e+03 |
| n_updates | 2774 |
-----------------------------------------------
-----------------------------------------------**************************************-------------------------| 74%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 3226.1812 |
| air_humidity | 40.99132 |
| air_temperature | 24.449615 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 94.0 |
| direct_solar_radiation | 891.5 |
| hour | 11.0 |
| htg_setpoint | 16.0 |
| month | 9.0 |
| outdoor_humidity | 39.0 |
| outdoor_temperature | 19.979425 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 2914970.8 |
| wind_direction | 30.0 |
| wind_speed | 1.5 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.57e+03 |
| n_updates | 2799 |
-----------------------------------------------
-----------------------------------------------***************************************------------------------| 75%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 820.8608 |
| air_humidity | 35.66342 |
| air_temperature | 22.958992 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 157.5 |
| direct_solar_radiation | 0.0 |
| hour | 12.0 |
| htg_setpoint | 21.0 |
| month | 10.0 |
| outdoor_humidity | 69.0 |
| outdoor_temperature | 11.693288 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 738774.7 |
| wind_direction | 340.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 7.76e+03 |
| n_updates | 2824 |
-----------------------------------------------
-----------------------------------------------***************************************------------------------| 75%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 39.326733 |
| air_temperature | 30.015965 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 161.5 |
| direct_solar_radiation | 622.5 |
| hour | 13.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 57.0 |
| outdoor_temperature | 20.66599 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 250.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.57e+03 |
| n_updates | 2849 |
-----------------------------------------------
-----------------------------------------------***************************************------------------------| 75%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 50.05312 |
| air_temperature | 31.606455 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 120.5 |
| direct_solar_radiation | 593.0 |
| hour | 14.0 |
| htg_setpoint | 21.0 |
| month | 10.0 |
| outdoor_humidity | 69.0 |
| outdoor_temperature | 22.639921 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 180.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.34e+03 |
| n_updates | 2874 |
-----------------------------------------------
-----------------------------------------------***************************************------------------------| 75%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 48.417835 |
| air_temperature | 32.195496 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 82.5 |
| direct_solar_radiation | 455.5 |
| hour | 15.0 |
| htg_setpoint | 18.0 |
| month | 10.0 |
| outdoor_humidity | 71.0 |
| outdoor_temperature | 22.904902 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 180.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.69e+03 |
| n_updates | 2899 |
-----------------------------------------------
-----------------------------------------------****************************************-----------------------| 76%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 42.599163 |
| air_temperature | 28.21489 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 27.5 |
| direct_solar_radiation | 186.5 |
| hour | 16.0 |
| htg_setpoint | 21.0 |
| month | 10.0 |
| outdoor_humidity | 78.0 |
| outdoor_temperature | 19.765554 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 180.0 |
| wind_speed | 8.8 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.67e+03 |
| n_updates | 2924 |
-----------------------------------------------
-----------------------------------------------****************************************-----------------------| 76%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 36.224995 |
| air_temperature | 22.329985 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 44.0 |
| outdoor_temperature | 13.891856 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 300.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.35e+03 |
| n_updates | 2949 |
-----------------------------------------------
-----------------------------------------------****************************************-----------------------| 76%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 323.90488 |
| air_humidity | 23.180758 |
| air_temperature | 23.656872 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 15.0 |
| month | 10.0 |
| outdoor_humidity | 36.0 |
| outdoor_temperature | 10.270113 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 287990.53 |
| wind_direction | 300.0 |
| wind_speed | 7.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.87e+03 |
| n_updates | 2974 |
-----------------------------------------------
-----------------------------------------------*****************************************----------------------| 77%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 335.21765 |
| air_humidity | 23.816273 |
| air_temperature | 22.013376 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 21.0 |
| month | 10.0 |
| outdoor_humidity | 46.0 |
| outdoor_temperature | 6.8252945 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 293124.94 |
| wind_direction | 340.0 |
| wind_speed | 1.5 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.88e+03 |
| n_updates | 2999 |
-----------------------------------------------
-----------------------------------------------*****************************************----------------------| 77%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 236.74127 |
| air_humidity | 37.661133 |
| air_temperature | 22.00012 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 66.0 |
| outdoor_temperature | 9.965243 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 213067.14 |
| wind_direction | 230.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.21e+03 |
| n_updates | 3024 |
-----------------------------------------------
-----------------------------------------------*****************************************----------------------| 77%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 135.69014 |
| air_humidity | 59.9251 |
| air_temperature | 21.229746 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 17.0 |
| month | 10.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 12.369878 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 120074.22 |
| wind_direction | 220.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.25e+03 |
| n_updates | 3049 |
-----------------------------------------------
-----------------------------------------------*****************************************----------------------| 77%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 67.62455 |
| air_humidity | 66.001045 |
| air_temperature | 21.45983 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 21.0 |
| month | 10.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 15.532434 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60862.094 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.77e+03 |
| n_updates | 3074 |
-----------------------------------------------
-----------------------------------------------******************************************---------------------| 78%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 70.07431 |
| air_temperature | 21.899416 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 21.0 |
| month | 10.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 15.607584 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 170.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.46e+03 |
| n_updates | 3099 |
-----------------------------------------------
------------------------------------------------*****************************************---------------------| 78%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 140.72768 |
| air_humidity | 65.1292 |
| air_temperature | 20.091452 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 15.0 |
| month | 10.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 13.924392 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 126654.914 |
| wind_direction | 100.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 790 |
| n_updates | 3124 |
------------------------------------------------
-----------------------------------------------******************************************---------------------| 78%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.97703 |
| air_humidity | 76.32632 |
| air_temperature | 20.053425 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 19.0 |
| month | 10.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 16.104088 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60279.33 |
| wind_direction | 150.0 |
| wind_speed | 8.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.61e+03 |
| n_updates | 3149 |
-----------------------------------------------
-----------------------------------------------*******************************************--------------------| 79%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 251.67865 |
| air_humidity | 41.05674 |
| air_temperature | 21.99997 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 89.0 |
| outdoor_temperature | 10.149518 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 226510.78 |
| wind_direction | 60.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.61e+03 |
| n_updates | 3174 |
-----------------------------------------------
-----------------------------------------------*******************************************--------------------| 79%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 485.2683 |
| air_humidity | 45.11187 |
| air_temperature | 19.242134 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 19.0 |
| month | 10.0 |
| outdoor_humidity | 83.0 |
| outdoor_temperature | 9.477785 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 436741.5 |
| wind_direction | 230.0 |
| wind_speed | 8.8 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.16e+03 |
| n_updates | 3199 |
-----------------------------------------------
-----------------------------------------------*******************************************--------------------| 79%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 585.45746 |
| air_humidity | 19.761223 |
| air_temperature | 21.440136 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 56.0 |
| outdoor_temperature | 5.395967 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 526911.7 |
| wind_direction | 270.0 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.85e+03 |
| n_updates | 3224 |
-----------------------------------------------
-----------------------------------------------********************************************-------------------| 80%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 218.11995 |
| air_humidity | 38.327847 |
| air_temperature | 19.056837 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 18.0 |
| month | 10.0 |
| outdoor_humidity | 90.0 |
| outdoor_temperature | 7.760579 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 196307.95 |
| wind_direction | 240.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 764 |
| n_updates | 3249 |
-----------------------------------------------
----------------------------------------------*********************************************-------------------| 80%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 87.81368 |
| air_humidity | 50.32354 |
| air_temperature | 19.49754 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 41.5 |
| direct_solar_radiation | 0.0 |
| hour | 6.0 |
| htg_setpoint | 17.0 |
| month | 10.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 10.19011 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 79032.31 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 8.16e+03 |
| n_updates | 3274 |
----------------------------------------------
-----------------------------------------------********************************************-------------------| 80%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 159.16617 |
| air_humidity | 59.31339 |
| air_temperature | 19.080135 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 105.5 |
| direct_solar_radiation | 468.0 |
| hour | 7.0 |
| htg_setpoint | 19.0 |
| month | 10.0 |
| outdoor_humidity | 90.0 |
| outdoor_temperature | 14.962557 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 145666.97 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.25e+03 |
| n_updates | 3299 |
-----------------------------------------------
-----------------------------------------------********************************************-------------------| 80%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 70.01712 |
| air_temperature | 23.176098 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 156.5 |
| direct_solar_radiation | 474.5 |
| hour | 8.0 |
| htg_setpoint | 21.0 |
| month | 10.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 17.920376 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 210.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.12e+03 |
| n_updates | 3324 |
-----------------------------------------------
-----------------------------------------------*********************************************------------------| 81%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 408.1777 |
| air_humidity | 59.842438 |
| air_temperature | 23.999256 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 179.0 |
| direct_solar_radiation | 6.0 |
| hour | 9.0 |
| htg_setpoint | 21.0 |
| month | 10.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 15.534999 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 372273.7 |
| wind_direction | 120.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.15e+03 |
| n_updates | 3349 |
-----------------------------------------------
-----------------------------------------------*********************************************------------------| 81%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 62.294548 |
| air_temperature | 28.43155 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 217.0 |
| direct_solar_radiation | 530.0 |
| hour | 10.0 |
| htg_setpoint | 20.0 |
| month | 10.0 |
| outdoor_humidity | 81.0 |
| outdoor_temperature | 21.39629 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 160.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.16e+03 |
| n_updates | 3374 |
-----------------------------------------------
-----------------------------------------------*********************************************------------------| 81%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 53.51948 |
| air_temperature | 30.693748 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 149.0 |
| direct_solar_radiation | 0.0 |
| hour | 11.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 57.0 |
| outdoor_temperature | 25.369297 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 250.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.03e+03 |
| n_updates | 3399 |
-----------------------------------------------
-----------------------------------------------*********************************************------------------| 81%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 17.327251 |
| air_temperature | 22.722736 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 143.0 |
| direct_solar_radiation | 714.5 |
| hour | 12.0 |
| htg_setpoint | 17.0 |
| month | 10.0 |
| outdoor_humidity | 28.0 |
| outdoor_temperature | 13.306188 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 250.0 |
| wind_speed | 9.8 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.56e+03 |
| n_updates | 3424 |
-----------------------------------------------
-----------------------------------------------**********************************************-----------------| 82%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 19.840294 |
| air_temperature | 22.38459 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 165.5 |
| direct_solar_radiation | 514.0 |
| hour | 13.0 |
| htg_setpoint | 16.0 |
| month | 10.0 |
| outdoor_humidity | 35.0 |
| outdoor_temperature | 11.596086 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 300.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.99e+03 |
| n_updates | 3449 |
-----------------------------------------------
-----------------------------------------------**********************************************-----------------| 82%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 18.47451 |
| air_temperature | 24.671492 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 94.5 |
| direct_solar_radiation | 484.5 |
| hour | 14.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 27.0 |
| outdoor_temperature | 11.386773 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 0.0 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.49e+03 |
| n_updates | 3474 |
-----------------------------------------------
-----------------------------------------------**********************************************-----------------| 82%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 439.10754 |
| air_humidity | 26.441185 |
| air_temperature | 26.733648 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 54.0 |
| direct_solar_radiation | 272.0 |
| hour | 15.0 |
| htg_setpoint | 18.0 |
| month | 10.0 |
| outdoor_humidity | 37.0 |
| outdoor_temperature | 14.764248 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 395254.56 |
| wind_direction | 240.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.95e+03 |
| n_updates | 3499 |
-----------------------------------------------
-----------------------------------------------***********************************************----------------| 83%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 554.31964 |
| air_humidity | 23.395735 |
| air_temperature | 24.999321 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 16.0 |
| htg_setpoint | 20.0 |
| month | 10.0 |
| outdoor_humidity | 32.0 |
| outdoor_temperature | 14.393337 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 503426.9 |
| wind_direction | 30.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.45e+03 |
| n_updates | 3524 |
-----------------------------------------------
-----------------------------------------------***********************************************----------------| 83%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 317.96118 |
| air_humidity | 51.266266 |
| air_temperature | 24.655975 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 15.0 |
| month | 10.0 |
| outdoor_humidity | 80.0 |
| outdoor_temperature | 14.171899 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 286165.06 |
| wind_direction | 180.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.68e+03 |
| n_updates | 3549 |
-----------------------------------------------
-----------------------------------------------***********************************************----------------| 83%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 233.69771 |
| air_humidity | 29.034204 |
| air_temperature | 23.883009 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 17.0 |
| month | 11.0 |
| outdoor_humidity | 48.0 |
| outdoor_temperature | 12.430132 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 210327.94 |
| wind_direction | 0.0 |
| wind_speed | 7.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.14e+03 |
| n_updates | 3574 |
-----------------------------------------------
-----------------------------------------------***********************************************----------------| 83%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 23.601944 |
| air_temperature | 21.99998 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 41.0 |
| outdoor_temperature | 14.13013 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 10.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.35e+03 |
| n_updates | 3599 |
-----------------------------------------------
-----------------------------------------------************************************************---------------| 84%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 37.767708 |
| air_temperature | 21.999975 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 72.0 |
| outdoor_temperature | 12.770189 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 220.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.51e+03 |
| n_updates | 3624 |
-----------------------------------------------
-----------------------------------------------************************************************---------------| 84%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 187.09816 |
| air_humidity | 31.238272 |
| air_temperature | 21.996042 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 59.0 |
| outdoor_temperature | 11.031565 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 167724.73 |
| wind_direction | 310.0 |
| wind_speed | 7.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.4e+03 |
| n_updates | 3649 |
-----------------------------------------------
-----------------------------------------------************************************************---------------| 84%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 505.19986 |
| air_humidity | 30.942884 |
| air_temperature | 19.999975 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 20.0 |
| month | 11.0 |
| outdoor_humidity | 65.0 |
| outdoor_temperature | 9.365477 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 454679.88 |
| wind_direction | 320.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.42e+03 |
| n_updates | 3674 |
-----------------------------------------------
-----------------------------------------------*************************************************--------------| 85%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 33.550316 |
| air_temperature | 18.521523 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 15.0 |
| month | 11.0 |
| outdoor_humidity | 74.0 |
| outdoor_temperature | 4.5707517 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 340.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.95e+03 |
| n_updates | 3699 |
-----------------------------------------------
-----------------------------------------------*************************************************--------------| 85%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 791.0938 |
| air_humidity | 39.81718 |
| air_temperature | 20.038563 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 21.0 |
| month | 11.0 |
| outdoor_humidity | 97.0 |
| outdoor_temperature | 6.485105 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 729618.75 |
| wind_direction | 350.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.61e+03 |
| n_updates | 3724 |
-----------------------------------------------
-----------------------------------------------*************************************************--------------| 85%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 45.82144 |
| air_temperature | 22.00016 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 7.2294836 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.21e+03 |
| n_updates | 3749 |
-----------------------------------------------
-----------------------------------------------**************************************************-------------| 86%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 56.175064 |
| air_temperature | 20.339254 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 20.0 |
| month | 11.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 12.948975 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 110.0 |
| wind_speed | 11.3 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.31e+03 |
| n_updates | 3774 |
-----------------------------------------------
-----------------------------------------------**************************************************-------------| 86%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 252.6262 |
| air_humidity | 51.662975 |
| air_temperature | 21.000048 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 21.0 |
| month | 11.0 |
| outdoor_humidity | 92.0 |
| outdoor_temperature | 11.568501 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 227363.6 |
| wind_direction | 310.0 |
| wind_speed | 9.8 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.87e+03 |
| n_updates | 3799 |
-----------------------------------------------
-----------------------------------------------**************************************************-------------| 86%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 755.2801 |
| air_humidity | 42.572395 |
| air_temperature | 19.000004 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 19.0 |
| month | 11.0 |
| outdoor_humidity | 77.0 |
| outdoor_temperature | 9.591404 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 679752.06 |
| wind_direction | 0.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.51e+03 |
| n_updates | 3824 |
-----------------------------------------------
-----------------------------------------------**************************************************-------------| 86%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 38.447903 |
| air_temperature | 17.8544 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 17.0 |
| month | 11.0 |
| outdoor_humidity | 85.0 |
| outdoor_temperature | 4.4505453 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 20.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.17e+03 |
| n_updates | 3849 |
-----------------------------------------------
-----------------------------------------------***************************************************------------| 87%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 244.03078 |
| air_humidity | 63.961487 |
| air_temperature | 18.345997 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 39.5 |
| direct_solar_radiation | 46.5 |
| hour | 6.0 |
| htg_setpoint | 15.0 |
| month | 11.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 11.001679 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 219627.69 |
| wind_direction | 90.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 591 |
| n_updates | 3874 |
-----------------------------------------------
-----------------------------------------------***************************************************------------| 87%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 244.03078 |
| air_humidity | 23.122137 |
| air_temperature | 21.613598 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 101.0 |
| direct_solar_radiation | 137.0 |
| hour | 7.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 69.0 |
| outdoor_temperature | 4.2041984 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 219627.69 |
| wind_direction | 270.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.42e+03 |
| n_updates | 3899 |
-----------------------------------------------
-----------------------------------------------***************************************************------------| 87%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 27.911211 |
| air_temperature | 18.84073 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 149.5 |
| direct_solar_radiation | 296.5 |
| hour | 8.0 |
| htg_setpoint | 16.0 |
| month | 11.0 |
| outdoor_humidity | 67.0 |
| outdoor_temperature | 3.8583755 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 270.0 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.62e+03 |
| n_updates | 3924 |
-----------------------------------------------
-----------------------------------------------***************************************************------------| 87%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 35.511806 |
| air_temperature | 20.975248 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 152.0 |
| direct_solar_radiation | 461.0 |
| hour | 9.0 |
| htg_setpoint | 17.0 |
| month | 11.0 |
| outdoor_humidity | 59.0 |
| outdoor_temperature | 11.71295 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 260.0 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.94e+03 |
| n_updates | 3949 |
-----------------------------------------------
-----------------------------------------------****************************************************-----------| 88%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 888.0539 |
| air_humidity | 30.422834 |
| air_temperature | 23.68936 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 108.0 |
| direct_solar_radiation | 740.0 |
| hour | 10.0 |
| htg_setpoint | 21.0 |
| month | 11.0 |
| outdoor_humidity | 62.0 |
| outdoor_temperature | 7.5188694 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 803709.56 |
| wind_direction | 280.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.02e+03 |
| n_updates | 3974 |
-----------------------------------------------
-----------------------------------------------****************************************************-----------| 88%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 755.6465 |
| air_humidity | 24.836256 |
| air_temperature | 23.50795 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 238.5 |
| direct_solar_radiation | 129.0 |
| hour | 11.0 |
| htg_setpoint | 19.0 |
| month | 11.0 |
| outdoor_humidity | 55.0 |
| outdoor_temperature | 4.671093 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 680081.9 |
| wind_direction | 290.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.35e+03 |
| n_updates | 3999 |
-----------------------------------------------
-----------------------------------------------****************************************************-----------| 88%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 29.07606 |
| air_temperature | 23.440237 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 186.0 |
| direct_solar_radiation | 295.0 |
| hour | 12.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 70.0 |
| outdoor_temperature | 7.70772 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 200.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.29e+03 |
| n_updates | 4024 |
-----------------------------------------------
-----------------------------------------------*****************************************************----------| 89%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 755.6465 |
| air_humidity | 23.929407 |
| air_temperature | 23.6828 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 146.0 |
| direct_solar_radiation | 366.0 |
| hour | 13.0 |
| htg_setpoint | 15.0 |
| month | 11.0 |
| outdoor_humidity | 61.0 |
| outdoor_temperature | 4.7432737 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 680081.9 |
| wind_direction | 270.0 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 7.81e+03 |
| n_updates | 4049 |
-----------------------------------------------
-----------------------------------------------*****************************************************----------| 89%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 1043.0057 |
| air_humidity | 17.89227 |
| air_temperature | 23.43317 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 97.5 |
| direct_solar_radiation | 246.5 |
| hour | 14.0 |
| htg_setpoint | 17.0 |
| month | 11.0 |
| outdoor_humidity | 41.0 |
| outdoor_temperature | 1.0846555 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 938705.1 |
| wind_direction | 290.0 |
| wind_speed | 9.3 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.53e+03 |
| n_updates | 4074 |
-----------------------------------------------
---------------------------------------------------*************************************************----------| 89%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1352.0616 |
| air_humidity | 14.324686 |
| air_temperature | 22.706608 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 28.0 |
| direct_solar_radiation | 122.5 |
| hour | 15.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 41.0 |
| outdoor_temperature | -0.0033998208 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1216855.5 |
| wind_direction | 300.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.53e+03 |
| n_updates | 4099 |
---------------------------------------------------
------------------------------------------------****************************************************----------| 89%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1246.1344 |
| air_humidity | 12.315995 |
| air_temperature | 21.914276 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 16.0 |
| htg_setpoint | 21.0 |
| month | 11.0 |
| outdoor_humidity | 44.0 |
| outdoor_temperature | 0.52623665 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1121520.9 |
| wind_direction | 290.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.29e+03 |
| n_updates | 4124 |
------------------------------------------------
-----------------------------------------------******************************************************---------| 90%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 33.015846 |
| air_temperature | 22.779686 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 21.0 |
| month | 11.0 |
| outdoor_humidity | 89.0 |
| outdoor_temperature | 2.373768 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 60.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.36e+03 |
| n_updates | 4149 |
-----------------------------------------------
-----------------------------------------------******************************************************---------| 90%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 400.23813 |
| air_humidity | 56.14992 |
| air_temperature | 22.67191 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 18.0 |
| month | 11.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 10.212911 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 360214.3 |
| wind_direction | 320.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.98e+03 |
| n_updates | 4174 |
-----------------------------------------------
-----------------------------------------------******************************************************---------| 90%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 299.3965 |
| air_humidity | 33.190514 |
| air_temperature | 22.000067 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 66.0 |
| outdoor_temperature | 9.364509 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 269456.88 |
| wind_direction | 260.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.13e+03 |
| n_updates | 4199 |
-----------------------------------------------
-----------------------------------------------******************************************************---------| 90%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 623.09314 |
| air_humidity | 34.904884 |
| air_temperature | 21.000025 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 21.0 |
| month | 11.0 |
| outdoor_humidity | 89.0 |
| outdoor_temperature | 6.079979 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 560783.8 |
| wind_direction | 180.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.15e+03 |
| n_updates | 4224 |
-----------------------------------------------
-----------------------------------------------*******************************************************--------| 91%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 35.073902 |
| air_temperature | 18.654799 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 16.0 |
| month | 11.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 4.2901263 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 190.0 |
| wind_speed | 5.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.7e+03 |
| n_updates | 4249 |
-----------------------------------------------
-----------------------------------------------*******************************************************--------| 91%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 168.6232 |
| air_humidity | 22.533516 |
| air_temperature | 20.000008 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 20.0 |
| month | 11.0 |
| outdoor_humidity | 52.0 |
| outdoor_temperature | 5.392105 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 151760.88 |
| wind_direction | 300.0 |
| wind_speed | 8.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.72e+03 |
| n_updates | 4274 |
-----------------------------------------------
-----------------------------------------------********************************************************-------| 92%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 19.730844 |
| air_temperature | 17.761995 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 17.0 |
| month | 12.0 |
| outdoor_humidity | 48.0 |
| outdoor_temperature | 2.2258358 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 320.0 |
| wind_speed | 9.8 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.55e+03 |
| n_updates | 4299 |
-----------------------------------------------
------------------------------------------------*******************************************************-------| 92%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1018.85645 |
| air_humidity | 17.41438 |
| air_temperature | 21.999998 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 22.0 |
| month | 12.0 |
| outdoor_humidity | 55.0 |
| outdoor_temperature | 1.6626261 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 916970.8 |
| wind_direction | 290.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.13e+03 |
| n_updates | 4324 |
------------------------------------------------
-----------------------------------------------********************************************************-------| 92%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 679.9791 |
| air_humidity | 29.711246 |
| air_temperature | 19.999962 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 20.0 |
| month | 12.0 |
| outdoor_humidity | 70.0 |
| outdoor_temperature | 8.013161 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 611981.2 |
| wind_direction | 220.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.28e+03 |
| n_updates | 4349 |
-----------------------------------------------
-----------------------------------------------********************************************************-------| 92%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 722.9635 |
| air_humidity | 47.509262 |
| air_temperature | 19.000923 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 19.0 |
| month | 12.0 |
| outdoor_humidity | 89.0 |
| outdoor_temperature | 9.301074 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 654445.4 |
| wind_direction | 40.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.8e+03 |
| n_updates | 4374 |
-----------------------------------------------
-----------------------------------------------*********************************************************------| 93%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1232.7352 |
| air_humidity | 22.427 |
| air_temperature | 21.999992 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 22.0 |
| month | 12.0 |
| outdoor_humidity | 60.0 |
| outdoor_temperature | 0.5932324 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1109461.8 |
| wind_direction | 270.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.39e+03 |
| n_updates | 4399 |
-----------------------------------------------
------------------------------------------------********************************************************------| 93%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1655.0643 |
| air_humidity | 18.618853 |
| air_temperature | 20.999966 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 21.0 |
| month | 12.0 |
| outdoor_humidity | 57.0 |
| outdoor_temperature | -1.5184134 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1489557.9 |
| wind_direction | 260.0 |
| wind_speed | 8.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.17e+03 |
| n_updates | 4424 |
------------------------------------------------
-----------------------------------------------*********************************************************------| 93%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 149.19484 |
| air_humidity | 19.301876 |
| air_temperature | 19.773636 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 19.0 |
| month | 12.0 |
| outdoor_humidity | 58.0 |
| outdoor_temperature | 4.3118777 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 134275.34 |
| wind_direction | 300.0 |
| wind_speed | 7.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.67e+03 |
| n_updates | 4449 |
-----------------------------------------------
------------------------------------------------********************************************************------| 93%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 624.0063 |
| air_humidity | 18.470314 |
| air_temperature | 20.999937 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 6.0 |
| htg_setpoint | 21.0 |
| month | 12.0 |
| outdoor_humidity | 79.0 |
| outdoor_temperature | 0.10012235 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 561605.7 |
| wind_direction | 20.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.83e+03 |
| n_updates | 4474 |
------------------------------------------------
-----------------------------------------------**********************************************************-----| 94%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 244.03078 |
| air_humidity | 26.461197 |
| air_temperature | 19.000006 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 29.5 |
| direct_solar_radiation | 20.5 |
| hour | 7.0 |
| htg_setpoint | 19.0 |
| month | 12.0 |
| outdoor_humidity | 63.0 |
| outdoor_temperature | 5.065284 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 219627.69 |
| wind_direction | 50.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.57e+03 |
| n_updates | 4499 |
-----------------------------------------------
------------------------------------------------*********************************************************-----| 94%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 1124.0399 |
| air_humidity | 30.274063 |
| air_temperature | 20.561508 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 48.0 |
| direct_solar_radiation | 0.0 |
| hour | 8.0 |
| htg_setpoint | 16.0 |
| month | 12.0 |
| outdoor_humidity | 92.0 |
| outdoor_temperature | 0.6794843 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1011635.94 |
| wind_direction | 30.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.14e+03 |
| n_updates | 4524 |
------------------------------------------------
-----------------------------------------------**********************************************************-----| 94%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 32.120804 |
| air_temperature | 22.681646 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 58.0 |
| direct_solar_radiation | 0.0 |
| hour | 9.0 |
| htg_setpoint | 21.0 |
| month | 12.0 |
| outdoor_humidity | 92.0 |
| outdoor_temperature | 3.6939497 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 10.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.21e+03 |
| n_updates | 4549 |
-----------------------------------------------
-----------------------------------------------***********************************************************----| 95%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 24.096895 |
| air_temperature | 23.350782 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 97.5 |
| direct_solar_radiation | 571.5 |
| hour | 10.0 |
| htg_setpoint | 15.0 |
| month | 12.0 |
| outdoor_humidity | 53.0 |
| outdoor_temperature | 5.244436 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 270.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.11e+03 |
| n_updates | 4574 |
-----------------------------------------------
-----------------------------------------------***********************************************************----| 95%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 18.148342 |
| air_temperature | 21.113705 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 111.0 |
| direct_solar_radiation | 636.0 |
| hour | 11.0 |
| htg_setpoint | 17.0 |
| month | 12.0 |
| outdoor_humidity | 41.0 |
| outdoor_temperature | 6.757193 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 280.0 |
| wind_speed | 8.8 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.85e+03 |
| n_updates | 4599 |
-----------------------------------------------
-----------------------------------------------***********************************************************----| 95%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 11.95327 |
| air_temperature | 22.296478 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 61.0 |
| direct_solar_radiation | 801.5 |
| hour | 12.0 |
| htg_setpoint | 21.0 |
| month | 12.0 |
| outdoor_humidity | 46.0 |
| outdoor_temperature | 2.5401795 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 230.0 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 8.09e+03 |
| n_updates | 4624 |
-----------------------------------------------
-----------------------------------------------***********************************************************----| 95%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 758.01276 |
| air_humidity | 20.702787 |
| air_temperature | 24.030527 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 96.5 |
| direct_solar_radiation | 462.0 |
| hour | 13.0 |
| htg_setpoint | 21.0 |
| month | 12.0 |
| outdoor_humidity | 38.0 |
| outdoor_temperature | 9.224388 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 682211.44 |
| wind_direction | 230.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.49e+03 |
| n_updates | 4649 |
-----------------------------------------------
-----------------------------------------------************************************************************---| 96%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 470.51666 |
| air_humidity | 22.118244 |
| air_temperature | 26.149982 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 40.5 |
| direct_solar_radiation | 562.0 |
| hour | 14.0 |
| htg_setpoint | 16.0 |
| month | 12.0 |
| outdoor_humidity | 33.0 |
| outdoor_temperature | 12.667169 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 423465.0 |
| wind_direction | 330.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.83e+03 |
| n_updates | 4674 |
-----------------------------------------------
-----------------------------------------------************************************************************---| 96%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 894.3857 |
| air_humidity | 23.486473 |
| air_temperature | 24.198273 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 18.0 |
| direct_solar_radiation | 265.0 |
| hour | 15.0 |
| htg_setpoint | 18.0 |
| month | 12.0 |
| outdoor_humidity | 58.0 |
| outdoor_temperature | 3.9825225 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 804947.06 |
| wind_direction | 230.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.04e+03 |
| n_updates | 4699 |
-----------------------------------------------
-----------------------------------------------************************************************************---| 96%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 656.4523 |
| air_humidity | 27.297323 |
| air_temperature | 23.39459 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 16.0 |
| htg_setpoint | 22.0 |
| month | 12.0 |
| outdoor_humidity | 49.0 |
| outdoor_temperature | 9.97379 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 590807.06 |
| wind_direction | 270.0 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.37e+03 |
| n_updates | 4724 |
-----------------------------------------------
-----------------------------------------------************************************************************---| 96%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 29.489573 |
| air_temperature | 22.93118 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 16.0 |
| month | 12.0 |
| outdoor_humidity | 59.0 |
| outdoor_temperature | 5.396768 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 310.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.54e+03 |
| n_updates | 4749 |
-----------------------------------------------
------------------------------------------------************************************************************--| 97%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1223.6066 |
| air_humidity | 17.345486 |
| air_temperature | 21.348074 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 21.0 |
| month | 12.0 |
| outdoor_humidity | 45.0 |
| outdoor_temperature | 0.63887537 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1101246.0 |
| wind_direction | 40.0 |
| wind_speed | 1.5 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.74e+03 |
| n_updates | 4774 |
------------------------------------------------
-----------------------------------------------*************************************************************--| 97%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 16.512764 |
| air_temperature | 19.293253 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 16.0 |
| month | 12.0 |
| outdoor_humidity | 73.0 |
| outdoor_temperature | 2.127042 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 50.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.39e+03 |
| n_updates | 4799 |
-----------------------------------------------
-----------------------------------------------*************************************************************--| 97%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 35.35599 |
| air_temperature | 20.465097 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 16.0 |
| month | 12.0 |
| outdoor_humidity | 82.0 |
| outdoor_temperature | 3.7489338 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 340.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 232 |
| n_updates | 4824 |
-----------------------------------------------
-----------------------------------------------**************************************************************-| 98%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 33.52633 |
| air_temperature | 21.060165 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 21.0 |
| month | 12.0 |
| outdoor_humidity | 69.0 |
| outdoor_temperature | 3.7001107 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 130.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.44e+03 |
| n_updates | 4849 |
-----------------------------------------------
-----------------------------------------------**************************************************************-| 98%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 740.5445 |
| air_humidity | 35.829098 |
| air_temperature | 20.000029 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 20.0 |
| month | 12.0 |
| outdoor_humidity | 82.0 |
| outdoor_temperature | 6.2892137 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 666490.0 |
| wind_direction | 250.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.15e+03 |
| n_updates | 4874 |
-----------------------------------------------
-----------------------------------------------**************************************************************-| 98%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 27.159826 |
| air_temperature | 18.131216 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 15.0 |
| month | 12.0 |
| outdoor_humidity | 63.0 |
| outdoor_temperature | 5.0486894 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 250.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.03e+03 |
| n_updates | 4899 |
-----------------------------------------------
-------------------------------------------------*************************************************************| 99%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 1383.9827 |
| air_humidity | 33.605206 |
| air_temperature | 20.00006 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 20.0 |
| month | 12.0 |
| outdoor_humidity | 84.0 |
| outdoor_temperature | -0.16300492 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1245584.4 |
| wind_direction | 340.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.03e+04 |
| n_updates | 4924 |
-------------------------------------------------
-------------------------------------------------*************************************************************| 99%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1419.2175 |
| air_humidity | 21.13316 |
| air_temperature | 22.0 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 22.0 |
| month | 12.0 |
| outdoor_humidity | 63.0 |
| outdoor_temperature | -0.33917913 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1277295.8 |
| wind_direction | 60.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.91e+03 |
| n_updates | 4949 |
-------------------------------------------------
-----------------------------------------------***************************************************************| 99%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 28.017467 |
| air_temperature | 21.999989 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 22.0 |
| month | 12.0 |
| outdoor_humidity | 92.0 |
| outdoor_temperature | 2.999275 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 220.0 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.12e+03 |
| n_updates | 4974 |
-----------------------------------------------
------------------------------------------------**************************************************************| 99%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 1589.4012 |
| air_humidity | 30.838787 |
| air_temperature | 17.382097 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 17.0 |
| month | 12.0 |
| outdoor_humidity | 51.0 |
| outdoor_temperature | -1.1900978 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1430461.1 |
| wind_direction | 260.0 |
| wind_speed | 8.8 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.68e+03 |
| n_updates | 4999 |
------------------------------------------------
[WRAPPER LoggerWrapper] (INFO) : End of episode, recording summary (progress.csv) if logger is active
[ENVIRONMENT] (INFO) : Environment closed. [SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43]
#----------------------------------------------------------------------------------------------#
[ENVIRONMENT] (INFO) : Starting a new episode... [SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43_EVALUATION] [Episode 1]
#----------------------------------------------------------------------------------------------#
[MODELING] (INFO) : Episode directory created [/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43_EVALUATION-res1/Eplus-env-sub_run1]
[MODELING] (INFO) : Weather file USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3.epw used.
[MODELING] (INFO) : Updated building model with whole Output:Variable available names
[MODELING] (INFO) : Updated building model with whole Output:Meter available names
[MODELING] (INFO) : Adapting weather to building model. [USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3.epw]
[ENVIRONMENT] (INFO) : Saving episode output path... [/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43_EVALUATION-res1/Eplus-env-sub_run1/output]
[SIMULATOR] (INFO) : Running EnergyPlus with args: ['-w', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43_EVALUATION-res1/Eplus-env-sub_run1/USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3_Random_1.0_0.0_0.001.epw', '-d', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43_EVALUATION-res1/Eplus-env-sub_run1/output', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43_EVALUATION-res1/Eplus-env-sub_run1/5ZoneAutoDXVAV.epJSON']
[ENVIRONMENT] (INFO) : Episode 1 started.
/usr/local/lib/python3.10/dist-packages/opyplus/weather_data/weather_data.py:493: FutureWarning: the 'line_terminator'' keyword is deprecated, use 'lineterminator' instead.
epw_content = self._headers_to_epw(use_datetimes=use_datetimes) + df.to_csv(
[SIMULATOR] (INFO) : handlers initialized.
[SIMULATOR] (INFO) : handlers are ready.
[SIMULATOR] (INFO) : System is ready.
[WRAPPER LoggerWrapper] (INFO) : Creating monitor.csv for current episode (episode 1) if logger is active
Progress: |**-------------------------------------------------------------------------------------------------| 2%
/usr/local/lib/python3.10/dist-packages/gymnasium/spaces/box.py:240: UserWarning: WARN: Casting input x to numpy array.
gym.logger.warn("Casting input x to numpy array.")
Progress: |***************************************************************************************************| 99%
[WRAPPER LoggerWrapper] (INFO) : End of episode, recording summary (progress.csv) if logger is active
[ENVIRONMENT] (INFO) : Environment closed. [SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43_EVALUATION]
#----------------------------------------------------------------------------------------------#
[ENVIRONMENT] (INFO) : Starting a new episode... [SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43] [Episode 3]
#----------------------------------------------------------------------------------------------#
[MODELING] (INFO) : Episode directory created [/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43-res1/Eplus-env-sub_run3]
[MODELING] (INFO) : Weather file USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3.epw used.
[MODELING] (INFO) : Updated building model with whole Output:Variable available names
[MODELING] (INFO) : Updated building model with whole Output:Meter available names
[MODELING] (INFO) : Adapting weather to building model. [USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3.epw]
[ENVIRONMENT] (INFO) : Saving episode output path... [/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43-res1/Eplus-env-sub_run3/output]
[SIMULATOR] (INFO) : Running EnergyPlus with args: ['-w', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43-res1/Eplus-env-sub_run3/USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3_Random_1.0_0.0_0.001.epw', '-d', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43-res1/Eplus-env-sub_run3/output', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43-res1/Eplus-env-sub_run3/5ZoneAutoDXVAV.epJSON']
[ENVIRONMENT] (INFO) : Episode 3 started.
/usr/local/lib/python3.10/dist-packages/opyplus/weather_data/weather_data.py:493: FutureWarning: the 'line_terminator'' keyword is deprecated, use 'lineterminator' instead.
epw_content = self._headers_to_epw(use_datetimes=use_datetimes) + df.to_csv(
[WRAPPER LoggerWrapper] (INFO) : Creating monitor.csv for current episode (episode 3) if logger is active
Eval num_timesteps=70077, episode_reward=-14968.47 +/- 0.00
Episode length: 35040.00 +/- 0.00
---------------------------------------------------
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| eval/ | |
| comfort_violation(%) | 56.8 |
| cumulative_comfort_penalty | -1.33e+04 |
| cumulative_energy_penalty | -1.71e+03 |
| cumulative_power_consumption | 3.43e+07 |
| cumulative_reward | -1.5e+04 |
| cumulative_temperature_violation | 2.65e+04 |
| episode_length | 3.5e+04 |
| mean_comfort_penalty | -0.378 |
| mean_energy_penalty | -0.0489 |
| mean_power_consumption | 978 |
| mean_reward | -0.427 |
| mean_temperature_violation | 0.757 |
| std_cumulative_reward | 0 |
| std_reward | 0 |
| observation/ | |
| HVAC_electricity_demand_rate | 2943.2556 |
| air_humidity | 16.751154 |
| air_temperature | 19.999994 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 20.0 |
| month | 12.0 |
| outdoor_humidity | 38.0 |
| outdoor_temperature | -7.959369 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2648930.0 |
| wind_direction | 312.5 |
| wind_speed | 8.925 |
| rollout/ | |
| exploration_rate | 0.05 |
| time/ | |
| total_timesteps | 70077 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.86e+03 |
| n_updates | 5019 |
---------------------------------------------------
New best mean reward!
[SIMULATOR] (INFO) : handlers are ready.
[SIMULATOR] (INFO) : System is ready.
-----------------------------------------------
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 1979.7937 |
| air_humidity | 14.373043 |
| air_temperature | 18.999998 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 19.0 |
| month | 1.0 |
| outdoor_humidity | 60.5 |
| outdoor_temperature | -3.14206 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1781814.4 |
| wind_direction | 265.0 |
| wind_speed | 8.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.53e+03 |
| n_updates | 5024 |
-----------------------------------------------
/usr/local/lib/python3.10/dist-packages/gymnasium/core.py:311: UserWarning: WARN: env.observation_variables to get variables from other wrappers is deprecated and will be removed in v1.0, to get this variable you can do `env.unwrapped.observation_variables` for environment variables or `env.get_wrapper_attr('observation_variables')` that will search the reminding wrappers.
logger.warn(
/usr/local/lib/python3.10/dist-packages/gymnasium/core.py:311: UserWarning: WARN: env.action_variables to get variables from other wrappers is deprecated and will be removed in v1.0, to get this variable you can do `env.unwrapped.action_variables` for environment variables or `env.get_wrapper_attr('action_variables')` that will search the reminding wrappers.
logger.warn(
/usr/local/lib/python3.10/dist-packages/gymnasium/core.py:311: UserWarning: WARN: env.is_discrete to get variables from other wrappers is deprecated and will be removed in v1.0, to get this variable you can do `env.unwrapped.is_discrete` for environment variables or `env.get_wrapper_attr('is_discrete')` that will search the reminding wrappers.
logger.warn(
/usr/local/lib/python3.10/dist-packages/gymnasium/spaces/box.py:240: UserWarning: WARN: Casting input x to numpy array.
gym.logger.warn("Casting input x to numpy array.")
--------------------------------------------------------------------------------------------------------------| 1%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 2914.7188 |
| air_humidity | 12.686562 |
| air_temperature | 18.000069 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 6.0 |
| htg_setpoint | 18.0 |
| month | 1.0 |
| outdoor_humidity | 49.0 |
| outdoor_temperature | -11.35344 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2623247.0 |
| wind_direction | 300.0 |
| wind_speed | 2.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.1e+03 |
| n_updates | 5049 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 1%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 244.03078 |
| air_humidity | 29.281803 |
| air_temperature | 18.999775 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 1.0 |
| direct_solar_radiation | 0.0 |
| hour | 7.0 |
| htg_setpoint | 19.0 |
| month | 1.0 |
| outdoor_humidity | 92.5 |
| outdoor_temperature | 7.188634 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 219627.69 |
| wind_direction | 95.0 |
| wind_speed | 12.9 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.79e+03 |
| n_updates | 5074 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 1%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 1958.0961 |
| air_humidity | 19.965443 |
| air_temperature | 19.137955 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 79.0 |
| direct_solar_radiation | 145.0 |
| hour | 8.0 |
| htg_setpoint | 15.0 |
| month | 1.0 |
| outdoor_humidity | 44.0 |
| outdoor_temperature | -3.490796 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1762286.4 |
| wind_direction | 275.0 |
| wind_speed | 9.05 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.35e+03 |
| n_updates | 5099 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 1%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 2621.8708 |
| air_humidity | 15.586883 |
| air_temperature | 18.63774 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 98.0 |
| direct_solar_radiation | 564.0 |
| hour | 9.0 |
| htg_setpoint | 17.0 |
| month | 1.0 |
| outdoor_humidity | 49.5 |
| outdoor_temperature | -6.352446 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2359683.8 |
| wind_direction | 290.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.8e+03 |
| n_updates | 5124 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 2%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 2009.7571 |
| air_humidity | 10.284857 |
| air_temperature | 22.0 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 154.0 |
| direct_solar_radiation | 483.0 |
| hour | 10.0 |
| htg_setpoint | 22.0 |
| month | 1.0 |
| outdoor_humidity | 47.0 |
| outdoor_temperature | -3.2918768 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1808781.4 |
| wind_direction | 225.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.32e+03 |
| n_updates | 5149 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 2%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 969.7436 |
| air_humidity | 17.372368 |
| air_temperature | 22.860384 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 104.0 |
| direct_solar_radiation | 700.0 |
| hour | 11.0 |
| htg_setpoint | 17.0 |
| month | 1.0 |
| outdoor_humidity | 30.0 |
| outdoor_temperature | 1.0222852 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 876343.25 |
| wind_direction | 310.0 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.39e+03 |
| n_updates | 5174 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 2%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1507.6636 |
| air_humidity | 18.65628 |
| air_temperature | 22.48924 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 77.0 |
| direct_solar_radiation | 0.0 |
| hour | 12.0 |
| htg_setpoint | 22.0 |
| month | 1.0 |
| outdoor_humidity | 88.5 |
| outdoor_temperature | -3.1577966 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 1356897.2 |
| wind_direction | 55.0 |
| wind_speed | 4.4 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.23e+04 |
| n_updates | 5199 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 2%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 617.50195 |
| air_humidity | 40.59938 |
| air_temperature | 23.053951 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 179.0 |
| direct_solar_radiation | 65.0 |
| hour | 13.0 |
| htg_setpoint | 18.0 |
| month | 1.0 |
| outdoor_humidity | 86.0 |
| outdoor_temperature | 10.169581 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 560649.7 |
| wind_direction | 190.0 |
| wind_speed | 10.05 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.07e+04 |
| n_updates | 5224 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 3%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 1731.4452 |
| air_humidity | 14.901506 |
| air_temperature | 23.613556 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 82.0 |
| direct_solar_radiation | 599.0 |
| hour | 14.0 |
| htg_setpoint | 16.0 |
| month | 1.0 |
| outdoor_humidity | 39.0 |
| outdoor_temperature | -2.357542 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1558300.8 |
| wind_direction | 250.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.46e+03 |
| n_updates | 5249 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 3%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1856.9801 |
| air_humidity | 13.100141 |
| air_temperature | 23.295666 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 52.0 |
| direct_solar_radiation | 496.0 |
| hour | 15.0 |
| htg_setpoint | 22.0 |
| month | 1.0 |
| outdoor_humidity | 28.5 |
| outdoor_temperature | -2.8129723 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1671282.1 |
| wind_direction | 260.0 |
| wind_speed | 9.3 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.42e+03 |
| n_updates | 5274 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 3%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 21.320126 |
| air_temperature | 20.383184 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 13.0 |
| direct_solar_radiation | 133.0 |
| hour | 16.0 |
| htg_setpoint | 15.0 |
| month | 1.0 |
| outdoor_humidity | 56.0 |
| outdoor_temperature | 7.669841 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 225.0 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.36e+03 |
| n_updates | 5299 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 4%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1178.2567 |
| air_humidity | 25.377169 |
| air_temperature | 21.999947 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 22.0 |
| month | 1.0 |
| outdoor_humidity | 59.0 |
| outdoor_temperature | 0.86562514 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1060431.0 |
| wind_direction | 15.0 |
| wind_speed | 10.8 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 937 |
| n_updates | 5324 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 4%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 2288.4697 |
| air_humidity | 21.389498 |
| air_temperature | 22.192562 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 21.0 |
| month | 1.0 |
| outdoor_humidity | 91.0 |
| outdoor_temperature | -4.6854405 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 2059622.9 |
| wind_direction | 35.0 |
| wind_speed | 9.05 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.17e+03 |
| n_updates | 5349 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 4%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 1020.70496 |
| air_humidity | 32.219017 |
| air_temperature | 22.359734 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 16.0 |
| month | 1.0 |
| outdoor_humidity | 69.5 |
| outdoor_temperature | 1.6533837 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 918634.44 |
| wind_direction | 250.0 |
| wind_speed | 10.8 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.8e+03 |
| n_updates | 5374 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 4%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 728.1792 |
| air_humidity | 36.853565 |
| air_temperature | 20.2277 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 15.0 |
| month | 1.0 |
| outdoor_humidity | 71.0 |
| outdoor_temperature | 4.2651987 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 631941.0 |
| wind_direction | 235.0 |
| wind_speed | 4.9 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.26e+03 |
| n_updates | 5399 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 5%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 27.562044 |
| air_temperature | 21.547703 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 16.0 |
| month | 1.0 |
| outdoor_humidity | 90.5 |
| outdoor_temperature | 3.256225 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 55.0 |
| wind_speed | 0.75 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.05e+03 |
| n_updates | 5424 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 5%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 44.820778 |
| air_temperature | 18.46046 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 16.0 |
| month | 1.0 |
| outdoor_humidity | 82.5 |
| outdoor_temperature | 5.064962 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 245.0 |
| wind_speed | 6.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.34e+03 |
| n_updates | 5449 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 5%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 146.1293 |
| air_humidity | 18.085037 |
| air_temperature | 20.000011 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 20.0 |
| month | 1.0 |
| outdoor_humidity | 40.0 |
| outdoor_temperature | 5.086813 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 131516.38 |
| wind_direction | 260.0 |
| wind_speed | 6.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 827 |
| n_updates | 5474 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 6%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 1114.0479 |
| air_humidity | 23.16856 |
| air_temperature | 18.734001 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 16.0 |
| month | 1.0 |
| outdoor_humidity | 98.0 |
| outdoor_temperature | 1.1866692 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1002643.06 |
| wind_direction | 200.0 |
| wind_speed | 2.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.43e+03 |
| n_updates | 5499 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 6%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 846.8715 |
| air_humidity | 37.760624 |
| air_temperature | 20.225866 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 21.0 |
| month | 1.0 |
| outdoor_humidity | 92.0 |
| outdoor_temperature | 5.4928055 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 762184.4 |
| wind_direction | 35.0 |
| wind_speed | 4.9 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.62e+03 |
| n_updates | 5524 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 6%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 577.6642 |
| air_humidity | 39.027035 |
| air_temperature | 20.987038 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 22.0 |
| month | 1.0 |
| outdoor_humidity | 95.0 |
| outdoor_temperature | 6.158876 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 519897.78 |
| wind_direction | 50.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.41e+03 |
| n_updates | 5549 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 7%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 371.0242 |
| air_humidity | 50.039307 |
| air_temperature | 20.999393 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 21.0 |
| month | 1.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 9.968978 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 333921.78 |
| wind_direction | 180.0 |
| wind_speed | 11.05 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.59e+03 |
| n_updates | 5574 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 7%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 24.129557 |
| air_temperature | 21.972383 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 22.0 |
| month | 1.0 |
| outdoor_humidity | 57.0 |
| outdoor_temperature | 3.7546635 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 300.0 |
| wind_speed | 7.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.4e+03 |
| n_updates | 5599 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 7%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 1735.4952 |
| air_humidity | 31.633846 |
| air_temperature | 20.511398 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 20.0 |
| month | 1.0 |
| outdoor_humidity | 60.0 |
| outdoor_temperature | -1.9205676 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1561945.8 |
| wind_direction | 315.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.91e+03 |
| n_updates | 5624 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 7%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1448.3888 |
| air_humidity | 20.905155 |
| air_temperature | 22.0 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 6.0 |
| htg_setpoint | 22.0 |
| month | 1.0 |
| outdoor_humidity | 82.0 |
| outdoor_temperature | -0.4850352 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1303549.9 |
| wind_direction | 265.0 |
| wind_speed | 2.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 8.26e+03 |
| n_updates | 5649 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 8%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 270.76407 |
| air_humidity | 22.189915 |
| air_temperature | 20.999899 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 7.0 |
| htg_setpoint | 21.0 |
| month | 1.0 |
| outdoor_humidity | 85.5 |
| outdoor_temperature | 1.8663336 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 243687.66 |
| wind_direction | 65.0 |
| wind_speed | 2.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.06e+03 |
| n_updates | 5674 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 8%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1268.7618 |
| air_humidity | 32.23605 |
| air_temperature | 19.832317 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 92.0 |
| direct_solar_radiation | 60.0 |
| hour | 8.0 |
| htg_setpoint | 18.0 |
| month | 1.0 |
| outdoor_humidity | 91.0 |
| outdoor_temperature | -0.04412536 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1141885.8 |
| wind_direction | 20.0 |
| wind_speed | 4.4 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.17e+03 |
| n_updates | 5699 |
-------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 8%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 1885.5887 |
| air_humidity | 18.774643 |
| air_temperature | 20.993729 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 94.0 |
| direct_solar_radiation | 684.0 |
| hour | 9.0 |
| htg_setpoint | 15.0 |
| month | 1.0 |
| outdoor_humidity | 64.0 |
| outdoor_temperature | -3.12826 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1697029.9 |
| wind_direction | 350.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.85e+03 |
| n_updates | 5724 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 8%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 2407.4321 |
| air_humidity | 12.659005 |
| air_temperature | 23.013084 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 111.0 |
| direct_solar_radiation | 737.0 |
| hour | 10.0 |
| htg_setpoint | 22.0 |
| month | 1.0 |
| outdoor_humidity | 29.0 |
| outdoor_temperature | -5.6783485 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 2166689.0 |
| wind_direction | 30.0 |
| wind_speed | 5.15 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 9.9e+03 |
| n_updates | 5749 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 9%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 25.744223 |
| air_temperature | 23.33259 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 146.0 |
| direct_solar_radiation | 201.0 |
| hour | 11.0 |
| htg_setpoint | 21.0 |
| month | 2.0 |
| outdoor_humidity | 48.5 |
| outdoor_temperature | 8.006251 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 280.0 |
| wind_speed | 5.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.57e+03 |
| n_updates | 5774 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 9%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 21.89128 |
| air_temperature | 20.242847 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 77.0 |
| direct_solar_radiation | 789.0 |
| hour | 12.0 |
| htg_setpoint | 19.0 |
| month | 2.0 |
| outdoor_humidity | 34.0 |
| outdoor_temperature | 8.502991 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 330.0 |
| wind_speed | 6.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.32e+03 |
| n_updates | 5799 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 9%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1623.7172 |
| air_humidity | 8.896154 |
| air_temperature | 21.603312 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 148.0 |
| direct_solar_radiation | 570.0 |
| hour | 13.0 |
| htg_setpoint | 21.0 |
| month | 2.0 |
| outdoor_humidity | 30.0 |
| outdoor_temperature | -1.3616774 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1461345.5 |
| wind_direction | 295.0 |
| wind_speed | 2.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.24e+03 |
| n_updates | 5824 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 10%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 1193.3862 |
| air_humidity | 28.279856 |
| air_temperature | 22.578747 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 77.0 |
| direct_solar_radiation | 0.0 |
| hour | 14.0 |
| htg_setpoint | 19.0 |
| month | 2.0 |
| outdoor_humidity | 94.0 |
| outdoor_temperature | 0.33275306 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1074047.6 |
| wind_direction | 355.0 |
| wind_speed | 7.75 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 9.51e+03 |
| n_updates | 5849 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 10%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 2749.4739 |
| air_humidity | 12.780788 |
| air_temperature | 22.953503 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 68.0 |
| direct_solar_radiation | 150.0 |
| hour | 15.0 |
| htg_setpoint | 22.0 |
| month | 2.0 |
| outdoor_humidity | 40.0 |
| outdoor_temperature | -7.4476857 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 2474526.5 |
| wind_direction | 305.0 |
| wind_speed | 9.8 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 7.17e+03 |
| n_updates | 5874 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 10%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 2649.97 |
| air_humidity | 13.885911 |
| air_temperature | 22.413828 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 29.0 |
| direct_solar_radiation | 0.0 |
| hour | 16.0 |
| htg_setpoint | 21.0 |
| month | 2.0 |
| outdoor_humidity | 45.0 |
| outdoor_temperature | -6.9501653 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 2384972.8 |
| wind_direction | 275.0 |
| wind_speed | 8.75 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.11e+03 |
| n_updates | 5899 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 10%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 1924.2723 |
| air_humidity | 16.133593 |
| air_temperature | 22.803274 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 15.0 |
| month | 2.0 |
| outdoor_humidity | 46.0 |
| outdoor_temperature | -3.3216777 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1731845.1 |
| wind_direction | 270.0 |
| wind_speed | 5.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.56e+03 |
| n_updates | 5924 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 11%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 2374.6277 |
| air_humidity | 14.483408 |
| air_temperature | 23.601479 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 15.0 |
| month | 2.0 |
| outdoor_humidity | 34.0 |
| outdoor_temperature | -5.11623 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 2137165.0 |
| wind_direction | 325.0 |
| wind_speed | 6.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.73e+03 |
| n_updates | 5949 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 11%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1881.4619 |
| air_humidity | 10.946623 |
| air_temperature | 20.999998 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 21.0 |
| month | 2.0 |
| outdoor_humidity | 44.0 |
| outdoor_temperature | -2.6504009 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1693315.6 |
| wind_direction | 260.0 |
| wind_speed | 6.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.29e+03 |
| n_updates | 5974 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 11%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 140.76978 |
| air_humidity | 17.480413 |
| air_temperature | 19.392883 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 19.0 |
| month | 2.0 |
| outdoor_humidity | 68.0 |
| outdoor_temperature | 4.4756484 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 126692.8 |
| wind_direction | 220.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.6e+03 |
| n_updates | 5999 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 11%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 21.333809 |
| air_temperature | 19.543318 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 18.0 |
| month | 2.0 |
| outdoor_humidity | 33.5 |
| outdoor_temperature | 3.4271114 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 300.0 |
| wind_speed | 8.25 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 7.64e+03 |
| n_updates | 6024 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 12%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 2648.4836 |
| air_humidity | 14.186273 |
| air_temperature | 20.95374 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 21.0 |
| month | 2.0 |
| outdoor_humidity | 34.0 |
| outdoor_temperature | -6.4855103 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2383635.5 |
| wind_direction | 310.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.21e+03 |
| n_updates | 6049 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 12%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 2084.3047 |
| air_humidity | 21.145824 |
| air_temperature | 18.761835 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 18.0 |
| month | 2.0 |
| outdoor_humidity | 50.0 |
| outdoor_temperature | -3.6646152 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1875874.2 |
| wind_direction | 270.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.44e+03 |
| n_updates | 6074 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 13%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 2165.2742 |
| air_humidity | 22.902588 |
| air_temperature | 18.895075 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 15.0 |
| month | 2.0 |
| outdoor_humidity | 45.0 |
| outdoor_temperature | -4.069463 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1948746.9 |
| wind_direction | 55.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.67e+03 |
| n_updates | 6099 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 13%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 954.6932 |
| air_humidity | 36.295982 |
| air_temperature | 19.999998 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 20.0 |
| month | 2.0 |
| outdoor_humidity | 86.5 |
| outdoor_temperature | 1.9834425 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 859223.9 |
| wind_direction | 245.0 |
| wind_speed | 5.65 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 7.84e+03 |
| n_updates | 6124 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 13%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 975.0257 |
| air_humidity | 24.071327 |
| air_temperature | 18.109482 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 15.0 |
| month | 2.0 |
| outdoor_humidity | 56.5 |
| outdoor_temperature | 1.88178 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 877523.1 |
| wind_direction | 10.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.44e+03 |
| n_updates | 6149 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 13%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 1413.4546 |
| air_humidity | 18.455126 |
| air_temperature | 20.0 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 20.0 |
| month | 2.0 |
| outdoor_humidity | 70.0 |
| outdoor_temperature | -0.31036434 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1272109.1 |
| wind_direction | 235.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.49e+03 |
| n_updates | 6174 |
-------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 14%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 2057.9175 |
| air_humidity | 33.432148 |
| air_temperature | 19.999971 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 20.0 |
| month | 2.0 |
| outdoor_humidity | 93.5 |
| outdoor_temperature | -3.5326786 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1852125.6 |
| wind_direction | 55.0 |
| wind_speed | 2.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.78e+03 |
| n_updates | 6199 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 14%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 27.786938 |
| air_temperature | 18.214876 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 18.0 |
| month | 2.0 |
| outdoor_humidity | 78.0 |
| outdoor_temperature | 3.168115 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 55.0 |
| wind_speed | 2.05 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.2e+03 |
| n_updates | 6224 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 14%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 244.03078 |
| air_humidity | 37.185356 |
| air_temperature | 17.73231 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 6.0 |
| htg_setpoint | 17.0 |
| month | 2.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | 2.5003846 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 219627.69 |
| wind_direction | 55.0 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.54e+03 |
| n_updates | 6249 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 14%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 875.0743 |
| air_humidity | 17.010324 |
| air_temperature | 21.195412 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 36.0 |
| direct_solar_radiation | 263.0 |
| hour | 7.0 |
| htg_setpoint | 22.0 |
| month | 2.0 |
| outdoor_humidity | 47.0 |
| outdoor_temperature | -1.1552175 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 787566.8 |
| wind_direction | 335.0 |
| wind_speed | 5.9 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.1e+04 |
| n_updates | 6274 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 15%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 128.47087 |
| air_humidity | 24.034351 |
| air_temperature | 21.000004 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 74.0 |
| direct_solar_radiation | 0.0 |
| hour | 8.0 |
| htg_setpoint | 21.0 |
| month | 2.0 |
| outdoor_humidity | 70.0 |
| outdoor_temperature | 4.4333735 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 115623.79 |
| wind_direction | 255.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.05e+03 |
| n_updates | 6299 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 15%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 90.19991 |
| air_humidity | 29.049053 |
| air_temperature | 21.000017 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 183.0 |
| direct_solar_radiation | 163.0 |
| hour | 9.0 |
| htg_setpoint | 21.0 |
| month | 2.0 |
| outdoor_humidity | 81.0 |
| outdoor_temperature | 4.225305 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 76612.64 |
| wind_direction | 280.0 |
| wind_speed | 7.5 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 9.99e+03 |
| n_updates | 6324 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 15%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1083.9386 |
| air_humidity | 14.645807 |
| air_temperature | 23.179724 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 94.0 |
| direct_solar_radiation | 795.0 |
| hour | 10.0 |
| htg_setpoint | 22.0 |
| month | 2.0 |
| outdoor_humidity | 34.0 |
| outdoor_temperature | 0.93911934 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 975544.8 |
| wind_direction | 275.0 |
| wind_speed | 6.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 7.38e+03 |
| n_updates | 6349 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 16%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 2489.8848 |
| air_humidity | 17.072931 |
| air_temperature | 22.479544 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 194.0 |
| direct_solar_radiation | 0.0 |
| hour | 11.0 |
| htg_setpoint | 16.0 |
| month | 2.0 |
| outdoor_humidity | 75.0 |
| outdoor_temperature | -6.671191 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 2240896.2 |
| wind_direction | 35.0 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.29e+03 |
| n_updates | 6374 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 16%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 1146.3035 |
| air_humidity | 24.533276 |
| air_temperature | 22.500486 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 134.0 |
| direct_solar_radiation | 0.0 |
| hour | 12.0 |
| htg_setpoint | 20.0 |
| month | 2.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | -1.350996 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 1031673.1 |
| wind_direction | 45.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.53e+03 |
| n_updates | 6399 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 16%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 755.6465 |
| air_humidity | 37.321774 |
| air_temperature | 22.816954 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 119.0 |
| direct_solar_radiation | 0.0 |
| hour | 13.0 |
| htg_setpoint | 19.0 |
| month | 2.0 |
| outdoor_humidity | 96.5 |
| outdoor_temperature | 5.335506 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 680081.9 |
| wind_direction | 10.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.75e+03 |
| n_updates | 6424 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 16%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 467.1646 |
| air_humidity | 21.171333 |
| air_temperature | 26.25289 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 179.0 |
| direct_solar_radiation | 639.0 |
| hour | 14.0 |
| htg_setpoint | 17.0 |
| month | 3.0 |
| outdoor_humidity | 29.5 |
| outdoor_temperature | 12.6892805 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 420448.16 |
| wind_direction | 150.0 |
| wind_speed | 5.4 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.75e+03 |
| n_updates | 6449 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 17%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 36.445587 |
| air_temperature | 22.380398 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 181.0 |
| direct_solar_radiation | 93.0 |
| hour | 15.0 |
| htg_setpoint | 19.0 |
| month | 3.0 |
| outdoor_humidity | 74.5 |
| outdoor_temperature | 13.105264 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 205.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.48e+03 |
| n_updates | 6474 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 17%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 23.927788 |
| air_temperature | 22.772154 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 89.0 |
| direct_solar_radiation | 410.0 |
| hour | 16.0 |
| htg_setpoint | 16.0 |
| month | 3.0 |
| outdoor_humidity | 40.5 |
| outdoor_temperature | 13.871598 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 305.0 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.34e+03 |
| n_updates | 6499 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 17%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 42.74886 |
| air_temperature | 23.01816 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 18.0 |
| direct_solar_radiation | 68.0 |
| hour | 17.0 |
| htg_setpoint | 20.0 |
| month | 3.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 6.287525 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 185.0 |
| wind_speed | 2.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.49e+04 |
| n_updates | 6524 |
----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 17%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 668.89575 |
| air_humidity | 45.53613 |
| air_temperature | 22.081665 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 20.0 |
| month | 3.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 6.980969 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 590484.25 |
| wind_direction | 190.0 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.32e+03 |
| n_updates | 6549 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 18%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 152.26994 |
| air_humidity | 42.321407 |
| air_temperature | 22.286524 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 22.0 |
| month | 3.0 |
| outdoor_humidity | 64.5 |
| outdoor_temperature | 12.418407 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 137042.95 |
| wind_direction | 305.0 |
| wind_speed | 10.05 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.63e+03 |
| n_updates | 6574 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 18%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 464.7534 |
| air_humidity | 34.08908 |
| air_temperature | 20.413956 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 20.0 |
| month | 3.0 |
| outdoor_humidity | 83.5 |
| outdoor_temperature | 6.7095146 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 403879.12 |
| wind_direction | 145.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.76e+03 |
| n_updates | 6599 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 18%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 38.898567 |
| air_temperature | 21.18225 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 17.0 |
| month | 3.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 2.3518631 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 125.0 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.79e+03 |
| n_updates | 6624 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 19%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 35.09905 |
| air_temperature | 19.368822 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 19.0 |
| month | 3.0 |
| outdoor_humidity | 90.5 |
| outdoor_temperature | 3.9090285 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 110.0 |
| wind_speed | 5.4 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.25e+03 |
| n_updates | 6649 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 19%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.8499 |
| air_humidity | 23.85285 |
| air_temperature | 19.461779 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 18.0 |
| month | 3.0 |
| outdoor_humidity | 58.0 |
| outdoor_temperature | 1.9976588 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856664.94 |
| wind_direction | 5.0 |
| wind_speed | 7.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.07e+03 |
| n_updates | 6674 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 19%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 31.365232 |
| air_temperature | 18.261238 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 16.0 |
| month | 3.0 |
| outdoor_humidity | 70.0 |
| outdoor_temperature | 5.429393 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 240.0 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.25e+03 |
| n_updates | 6699 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 20%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 16.056242 |
| air_temperature | 21.999687 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 22.0 |
| month | 3.0 |
| outdoor_humidity | 45.5 |
| outdoor_temperature | 2.379026 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 295.0 |
| wind_speed | 9.3 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.33e+03 |
| n_updates | 6724 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 20%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 1718.1122 |
| air_humidity | 24.954447 |
| air_temperature | 18.076813 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 16.0 |
| month | 3.0 |
| outdoor_humidity | 40.0 |
| outdoor_temperature | -1.8336521 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1546300.9 |
| wind_direction | 340.0 |
| wind_speed | 3.9 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 9.98e+03 |
| n_updates | 6749 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 20%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 746.1307 |
| air_humidity | 31.589094 |
| air_temperature | 20.72473 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 22.0 |
| month | 3.0 |
| outdoor_humidity | 85.5 |
| outdoor_temperature | 4.810494 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 671517.6 |
| wind_direction | 285.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.05e+03 |
| n_updates | 6774 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 20%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 1189.1144 |
| air_humidity | 17.219078 |
| air_temperature | 18.536963 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 18.0 |
| month | 3.0 |
| outdoor_humidity | 54.0 |
| outdoor_temperature | 0.8113365 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1070203.0 |
| wind_direction | 35.0 |
| wind_speed | 4.65 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.56e+03 |
| n_updates | 6799 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 21%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 1760.4196 |
| air_humidity | 17.643135 |
| air_temperature | 21.909454 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 22.0 |
| month | 3.0 |
| outdoor_humidity | 77.5 |
| outdoor_temperature | -2.0451891 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1584377.6 |
| wind_direction | 10.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.13e+03 |
| n_updates | 6824 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 21%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 1259.0308 |
| air_humidity | 21.857689 |
| air_temperature | 18.455622 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 6.0 |
| htg_setpoint | 18.0 |
| month | 3.0 |
| outdoor_humidity | 91.0 |
| outdoor_temperature | -3.0750003 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1133127.8 |
| wind_direction | 50.0 |
| wind_speed | 1.8 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.61e+03 |
| n_updates | 6849 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 21%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 282.3766 |
| air_humidity | 29.170164 |
| air_temperature | 21.000095 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 102.0 |
| direct_solar_radiation | 0.0 |
| hour | 7.0 |
| htg_setpoint | 21.0 |
| month | 3.0 |
| outdoor_humidity | 98.0 |
| outdoor_temperature | 1.8082709 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 254138.92 |
| wind_direction | 25.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.05e+03 |
| n_updates | 6874 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 22%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 978.2873 |
| air_humidity | 23.684679 |
| air_temperature | 20.935505 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 182.0 |
| direct_solar_radiation | 539.0 |
| hour | 8.0 |
| htg_setpoint | 18.0 |
| month | 3.0 |
| outdoor_humidity | 69.5 |
| outdoor_temperature | 1.4673761 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 880458.56 |
| wind_direction | 190.0 |
| wind_speed | 4.15 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.67e+03 |
| n_updates | 6899 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 22%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 871.7625 |
| air_humidity | 33.1851 |
| air_temperature | 22.755598 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 122.0 |
| direct_solar_radiation | 0.0 |
| hour | 9.0 |
| htg_setpoint | 21.0 |
| month | 3.0 |
| outdoor_humidity | 62.0 |
| outdoor_temperature | 8.619181 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 784586.25 |
| wind_direction | 280.0 |
| wind_speed | 8.5 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 617 |
| n_updates | 6924 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 22%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1314.9203 |
| air_humidity | 13.52808 |
| air_temperature | 22.85324 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 148.0 |
| direct_solar_radiation | 828.0 |
| hour | 10.0 |
| htg_setpoint | 22.0 |
| month | 3.0 |
| outdoor_humidity | 26.0 |
| outdoor_temperature | -0.21578881 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1183428.2 |
| wind_direction | 315.0 |
| wind_speed | 11.05 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.41e+03 |
| n_updates | 6949 |
-------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 22%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 12.919759 |
| air_temperature | 22.333363 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 145.0 |
| direct_solar_radiation | 866.0 |
| hour | 11.0 |
| htg_setpoint | 22.0 |
| month | 3.0 |
| outdoor_humidity | 28.0 |
| outdoor_temperature | 3.917022 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 225.0 |
| wind_speed | 8.25 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.94e+03 |
| n_updates | 6974 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 23%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 89.78595 |
| air_humidity | 21.541876 |
| air_temperature | 22.142008 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 145.0 |
| direct_solar_radiation | 869.0 |
| hour | 12.0 |
| htg_setpoint | 21.0 |
| month | 3.0 |
| outdoor_humidity | 54.0 |
| outdoor_temperature | 11.908008 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 80879.87 |
| wind_direction | 180.0 |
| wind_speed | 5.15 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.76e+03 |
| n_updates | 6999 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 23%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 755.6465 |
| air_humidity | 38.843994 |
| air_temperature | 23.04883 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 140.0 |
| direct_solar_radiation | 0.0 |
| hour | 13.0 |
| htg_setpoint | 21.0 |
| month | 3.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 5.6984205 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 680081.9 |
| wind_direction | 180.0 |
| wind_speed | 5.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.59e+03 |
| n_updates | 7024 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 23%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 550.6507 |
| air_humidity | 44.1667 |
| air_temperature | 25.99846 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 291.0 |
| direct_solar_radiation | 267.0 |
| hour | 14.0 |
| htg_setpoint | 19.0 |
| month | 3.0 |
| outdoor_humidity | 76.0 |
| outdoor_temperature | 14.344811 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 495585.66 |
| wind_direction | 140.0 |
| wind_speed | 4.15 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.44e+03 |
| n_updates | 7049 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 23%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 44.996563 |
| air_temperature | 26.92001 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 189.0 |
| direct_solar_radiation | 575.0 |
| hour | 15.0 |
| htg_setpoint | 22.0 |
| month | 3.0 |
| outdoor_humidity | 61.5 |
| outdoor_temperature | 19.1893 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 0.0 |
| wind_speed | 6.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.76e+03 |
| n_updates | 7074 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 24%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 407.94373 |
| air_humidity | 34.073017 |
| air_temperature | 25.267233 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 145.0 |
| direct_solar_radiation | 108.0 |
| hour | 16.0 |
| htg_setpoint | 19.0 |
| month | 3.0 |
| outdoor_humidity | 57.0 |
| outdoor_temperature | 11.922835 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 367149.34 |
| wind_direction | 40.0 |
| wind_speed | 9.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.23e+03 |
| n_updates | 7099 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 24%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 820.66797 |
| air_humidity | 34.85887 |
| air_temperature | 24.125118 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 42.0 |
| direct_solar_radiation | 314.0 |
| hour | 17.0 |
| htg_setpoint | 21.0 |
| month | 3.0 |
| outdoor_humidity | 79.0 |
| outdoor_temperature | 6.8898964 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 737382.6 |
| wind_direction | 115.0 |
| wind_speed | 5.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.01e+03 |
| n_updates | 7124 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 24%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 28.866589 |
| air_temperature | 21.999998 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 22.0 |
| month | 3.0 |
| outdoor_humidity | 85.5 |
| outdoor_temperature | 5.465069 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 100.0 |
| wind_speed | 4.65 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.3e+03 |
| n_updates | 7149 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 25%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 43.123447 |
| air_temperature | 20.99996 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 21.0 |
| month | 3.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 9.107789 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 5.0 |
| wind_speed | 4.65 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.23e+03 |
| n_updates | 7174 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 25%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 48.636272 |
| air_temperature | 23.684591 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 22.0 |
| month | 4.0 |
| outdoor_humidity | 83.5 |
| outdoor_temperature | 4.5161777 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 240.0 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.41e+03 |
| n_updates | 7199 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 25%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 44.006355 |
| air_temperature | 23.509317 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 22.0 |
| month | 4.0 |
| outdoor_humidity | 48.0 |
| outdoor_temperature | 4.1877465 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 330.0 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.37e+03 |
| n_updates | 7224 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 25%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 46.724228 |
| air_temperature | 22.732481 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 22.0 |
| month | 4.0 |
| outdoor_humidity | 71.5 |
| outdoor_temperature | 4.715655 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 220.0 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 859 |
| n_updates | 7249 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 26%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 63.647705 |
| air_temperature | 21.887827 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 22.0 |
| month | 4.0 |
| outdoor_humidity | 75.0 |
| outdoor_temperature | 6.674119 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 210.0 |
| wind_speed | 4.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.65e+03 |
| n_updates | 7274 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 26%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 73.95778 |
| air_temperature | 21.563845 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 21.0 |
| month | 4.0 |
| outdoor_humidity | 91.0 |
| outdoor_temperature | 10.680291 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 235.0 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 7.02e+03 |
| n_updates | 7299 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 26%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 71.15199 |
| air_temperature | 22.148827 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 15.0 |
| month | 4.0 |
| outdoor_humidity | 72.0 |
| outdoor_temperature | 16.010214 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 240.0 |
| wind_speed | 2.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.53e+03 |
| n_updates | 7324 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 27%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 70.10457 |
| air_temperature | 22.37624 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 22.0 |
| month | 4.0 |
| outdoor_humidity | 69.5 |
| outdoor_temperature | 16.720282 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 235.0 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.65e+03 |
| n_updates | 7349 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 27%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 85.93943 |
| air_temperature | 21.058844 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 17.0 |
| month | 4.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 12.752775 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.24e+03 |
| n_updates | 7374 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 27%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 91.5358 |
| air_temperature | 20.520086 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 18.0 |
| month | 4.0 |
| outdoor_humidity | 90.0 |
| outdoor_temperature | 12.529675 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 230.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.88e+03 |
| n_updates | 7399 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 28%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 71.90292 |
| air_temperature | 19.641085 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 8.0 |
| direct_solar_radiation | 18.0 |
| hour | 5.0 |
| htg_setpoint | 22.0 |
| month | 4.0 |
| outdoor_humidity | 43.0 |
| outdoor_temperature | 6.235198 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 310.0 |
| wind_speed | 8.75 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.08e+03 |
| n_updates | 7424 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 28%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 54.272633 |
| air_temperature | 19.044207 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 42.0 |
| direct_solar_radiation | 530.0 |
| hour | 6.0 |
| htg_setpoint | 21.0 |
| month | 4.0 |
| outdoor_humidity | 36.0 |
| outdoor_temperature | 2.7869196 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 335.0 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.83e+03 |
| n_updates | 7449 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 28%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 45.30996 |
| air_temperature | 19.577765 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 118.0 |
| direct_solar_radiation | 0.0 |
| hour | 7.0 |
| htg_setpoint | 22.0 |
| month | 4.0 |
| outdoor_humidity | 52.0 |
| outdoor_temperature | 6.176463 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 235.0 |
| wind_speed | 2.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.76e+03 |
| n_updates | 7474 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 28%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 47.805527 |
| air_temperature | 18.648262 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 277.0 |
| direct_solar_radiation | 93.0 |
| hour | 8.0 |
| htg_setpoint | 15.0 |
| month | 4.0 |
| outdoor_humidity | 74.5 |
| outdoor_temperature | 6.1966367 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 70.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.7e+03 |
| n_updates | 7499 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 29%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 817.9984 |
| air_humidity | 43.170815 |
| air_temperature | 21.434963 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 168.0 |
| direct_solar_radiation | 0.0 |
| hour | 9.0 |
| htg_setpoint | 21.0 |
| month | 4.0 |
| outdoor_humidity | 98.5 |
| outdoor_temperature | 7.863375 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 736198.56 |
| wind_direction | 125.0 |
| wind_speed | 4.65 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.87e+03 |
| n_updates | 7524 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 29%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1087.7322 |
| air_humidity | 42.018997 |
| air_temperature | 24.99819 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 128.0 |
| direct_solar_radiation | 880.0 |
| hour | 10.0 |
| htg_setpoint | 20.0 |
| month | 4.0 |
| outdoor_humidity | 51.0 |
| outdoor_temperature | 17.724447 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 976106.0 |
| wind_direction | 275.0 |
| wind_speed | 6.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.35e+03 |
| n_updates | 7549 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 29%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 902.5843 |
| air_humidity | 48.01306 |
| air_temperature | 22.805077 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 256.0 |
| direct_solar_radiation | 0.0 |
| hour | 11.0 |
| htg_setpoint | 22.0 |
| month | 4.0 |
| outdoor_humidity | 79.0 |
| outdoor_temperature | 13.262782 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 812325.8 |
| wind_direction | 45.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.53e+03 |
| n_updates | 7574 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 29%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 539.21356 |
| air_humidity | 36.537544 |
| air_temperature | 22.499413 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 255.0 |
| direct_solar_radiation | 0.0 |
| hour | 12.0 |
| htg_setpoint | 21.0 |
| month | 4.0 |
| outdoor_humidity | 71.0 |
| outdoor_temperature | 8.842577 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 485292.22 |
| wind_direction | 55.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.37e+03 |
| n_updates | 7599 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 30%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 27.141941 |
| air_temperature | 23.097776 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 244.0 |
| direct_solar_radiation | 640.0 |
| hour | 13.0 |
| htg_setpoint | 22.0 |
| month | 4.0 |
| outdoor_humidity | 50.0 |
| outdoor_temperature | 11.159419 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 135.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.21e+03 |
| n_updates | 7624 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 30%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 52.81628 |
| air_temperature | 20.676254 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 200.0 |
| direct_solar_radiation | 0.0 |
| hour | 14.0 |
| htg_setpoint | 16.0 |
| month | 4.0 |
| outdoor_humidity | 83.0 |
| outdoor_temperature | 8.347079 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 105.0 |
| wind_speed | 4.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.39e+03 |
| n_updates | 7649 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 30%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 55.26341 |
| air_temperature | 19.509224 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 122.0 |
| direct_solar_radiation | 0.0 |
| hour | 15.0 |
| htg_setpoint | 18.0 |
| month | 4.0 |
| outdoor_humidity | 95.0 |
| outdoor_temperature | 8.819503 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 320.0 |
| wind_speed | 6.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 7e+03 |
| n_updates | 7674 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 31%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 339.67972 |
| air_humidity | 34.732143 |
| air_temperature | 23.77928 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 220.0 |
| direct_solar_radiation | 25.0 |
| hour | 16.0 |
| htg_setpoint | 20.0 |
| month | 4.0 |
| outdoor_humidity | 60.0 |
| outdoor_temperature | 11.276463 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 305711.75 |
| wind_direction | 260.0 |
| wind_speed | 8.75 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.05e+03 |
| n_updates | 7699 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 31%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 32.76473 |
| air_temperature | 27.478088 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 47.0 |
| direct_solar_radiation | 489.0 |
| hour | 17.0 |
| htg_setpoint | 22.0 |
| month | 4.0 |
| outdoor_humidity | 60.5 |
| outdoor_temperature | 13.006176 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 195.0 |
| wind_speed | 8.75 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 416 |
| n_updates | 7724 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 31%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 49.08971 |
| air_temperature | 27.583792 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 9.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 22.0 |
| month | 4.0 |
| outdoor_humidity | 91.0 |
| outdoor_temperature | 9.646996 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 205.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2e+03 |
| n_updates | 7749 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 31%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 47.054016 |
| air_temperature | 28.166939 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 22.0 |
| month | 4.0 |
| outdoor_humidity | 61.0 |
| outdoor_temperature | 15.822657 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.17e+03 |
| n_updates | 7774 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 32%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 48.195282 |
| air_temperature | 26.09547 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 21.0 |
| month | 4.0 |
| outdoor_humidity | 65.5 |
| outdoor_temperature | 12.948526 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 195.0 |
| wind_speed | 8.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.92e+03 |
| n_updates | 7799 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 32%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 57.145077 |
| air_temperature | 23.427517 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 16.0 |
| month | 4.0 |
| outdoor_humidity | 85.5 |
| outdoor_temperature | 13.653486 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 165.0 |
| wind_speed | 2.05 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.72e+03 |
| n_updates | 7824 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 32%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 60.926395 |
| air_temperature | 22.539474 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 16.0 |
| month | 4.0 |
| outdoor_humidity | 69.5 |
| outdoor_temperature | 11.2723255 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 100.0 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.12e+03 |
| n_updates | 7849 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 32%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 68.501816 |
| air_temperature | 21.293674 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 16.0 |
| month | 4.0 |
| outdoor_humidity | 87.5 |
| outdoor_temperature | 10.341484 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 130.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.03e+03 |
| n_updates | 7874 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 33%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 84.79883 |
| air_temperature | 21.605139 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 19.0 |
| month | 5.0 |
| outdoor_humidity | 85.0 |
| outdoor_temperature | 10.22611 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 30.0 |
| wind_speed | 2.05 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 8.16e+03 |
| n_updates | 7899 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 33%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 47.870968 |
| air_temperature | 20.849094 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 16.0 |
| month | 5.0 |
| outdoor_humidity | 52.0 |
| outdoor_temperature | 8.045892 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 30.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.53e+03 |
| n_updates | 7924 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 34%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 55.716766 |
| air_temperature | 21.081879 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 16.0 |
| month | 5.0 |
| outdoor_humidity | 63.5 |
| outdoor_temperature | 11.166885 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 40.0 |
| wind_speed | 9.5 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.19e+03 |
| n_updates | 7949 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 34%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 61.2547 |
| air_temperature | 21.099758 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 18.0 |
| month | 5.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 11.363247 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 0.0 |
| wind_speed | 5.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.32e+03 |
| n_updates | 7974 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 34%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 63.022537 |
| air_temperature | 20.645359 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 18.0 |
| month | 5.0 |
| outdoor_humidity | 97.0 |
| outdoor_temperature | 11.923875 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 320.0 |
| wind_speed | 1.5 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 8.12e+03 |
| n_updates | 7999 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 34%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 64.795944 |
| air_temperature | 20.21981 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 66.0 |
| direct_solar_radiation | 25.0 |
| hour | 5.0 |
| htg_setpoint | 21.0 |
| month | 5.0 |
| outdoor_humidity | 91.5 |
| outdoor_temperature | 11.719052 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 120.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.75e+03 |
| n_updates | 8024 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 35%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 89.53074 |
| air_temperature | 20.418552 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 84.0 |
| direct_solar_radiation | 0.0 |
| hour | 6.0 |
| htg_setpoint | 17.0 |
| month | 5.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 13.64203 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 85.0 |
| wind_speed | 4.9 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.18e+03 |
| n_updates | 8049 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 35%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 86.189896 |
| air_humidity | 93.52504 |
| air_temperature | 20.571243 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 88.0 |
| direct_solar_radiation | 0.0 |
| hour | 7.0 |
| htg_setpoint | 17.0 |
| month | 5.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 14.903064 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 77570.83 |
| wind_direction | 90.0 |
| wind_speed | 5.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.93e+03 |
| n_updates | 8074 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 35%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 400.48703 |
| air_humidity | 74.71837 |
| air_temperature | 22.064402 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 314.0 |
| direct_solar_radiation | 201.0 |
| hour | 8.0 |
| htg_setpoint | 20.0 |
| month | 5.0 |
| outdoor_humidity | 96.5 |
| outdoor_temperature | 15.097484 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 360157.4 |
| wind_direction | 220.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.9e+03 |
| n_updates | 8099 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 35%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1178.8964 |
| air_humidity | 43.18821 |
| air_temperature | 23.995653 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 227.0 |
| direct_solar_radiation | 653.0 |
| hour | 9.0 |
| htg_setpoint | 21.0 |
| month | 5.0 |
| outdoor_humidity | 50.5 |
| outdoor_temperature | 16.000217 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 925971.5 |
| wind_direction | 325.0 |
| wind_speed | 4.9 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 972 |
| n_updates | 8124 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 36%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 61.808674 |
| air_temperature | 24.230162 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 267.0 |
| direct_solar_radiation | 657.0 |
| hour | 10.0 |
| htg_setpoint | 21.0 |
| month | 5.0 |
| outdoor_humidity | 50.0 |
| outdoor_temperature | 13.990786 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 185.0 |
| wind_speed | 5.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.39e+03 |
| n_updates | 8149 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 36%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 59.38911 |
| air_temperature | 24.766394 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 286.0 |
| direct_solar_radiation | 655.0 |
| hour | 11.0 |
| htg_setpoint | 19.0 |
| month | 5.0 |
| outdoor_humidity | 42.5 |
| outdoor_temperature | 19.624998 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.17e+03 |
| n_updates | 8174 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 36%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 155.3502 |
| air_humidity | 24.00819 |
| air_temperature | 25.979362 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 278.0 |
| direct_solar_radiation | 671.0 |
| hour | 12.0 |
| htg_setpoint | 17.0 |
| month | 5.0 |
| outdoor_humidity | 46.5 |
| outdoor_temperature | 15.228561 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 139486.28 |
| wind_direction | 180.0 |
| wind_speed | 5.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.88e+03 |
| n_updates | 8199 |
-----------------------------------------------
-----------------------------------------------*--------------------------------------------------------------| 37%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 1030.0591 |
| air_humidity | 28.968252 |
| air_temperature | 23.402994 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 298.0 |
| direct_solar_radiation | 592.0 |
| hour | 13.0 |
| htg_setpoint | 22.0 |
| month | 5.0 |
| outdoor_humidity | 46.5 |
| outdoor_temperature | 14.540173 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 927053.2 |
| wind_direction | 150.0 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.21e+03 |
| n_updates | 8224 |
-----------------------------------------------
-----------------------------------------------*--------------------------------------------------------------| 37%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 797.6877 |
| air_humidity | 30.19432 |
| air_temperature | 26.263184 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 248.0 |
| direct_solar_radiation | 554.0 |
| hour | 14.0 |
| htg_setpoint | 18.0 |
| month | 5.0 |
| outdoor_humidity | 45.0 |
| outdoor_temperature | 17.258335 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 717918.94 |
| wind_direction | 160.0 |
| wind_speed | 8.75 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 9.15e+03 |
| n_updates | 8249 |
-----------------------------------------------
-----------------------------------------------*--------------------------------------------------------------| 37%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 874.74835 |
| air_humidity | 37.537148 |
| air_temperature | 27.0753 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 235.0 |
| direct_solar_radiation | 426.0 |
| hour | 15.0 |
| htg_setpoint | 17.0 |
| month | 5.0 |
| outdoor_humidity | 48.5 |
| outdoor_temperature | 18.415379 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 787273.56 |
| wind_direction | 115.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.27e+03 |
| n_updates | 8274 |
-----------------------------------------------
-----------------------------------------------*--------------------------------------------------------------| 37%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 3824.5125 |
| air_humidity | 48.882725 |
| air_temperature | 23.785929 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 148.0 |
| direct_solar_radiation | 496.0 |
| hour | 16.0 |
| htg_setpoint | 21.0 |
| month | 5.0 |
| outdoor_humidity | 51.0 |
| outdoor_temperature | 20.187895 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 3442061.2 |
| wind_direction | 105.0 |
| wind_speed | 5.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.99e+03 |
| n_updates | 8299 |
-----------------------------------------------
-----------------------------------------------**-------------------------------------------------------------| 38%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 67.68514 |
| air_temperature | 23.985237 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 82.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 15.0 |
| month | 5.0 |
| outdoor_humidity | 96.5 |
| outdoor_temperature | 17.166412 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 100.0 |
| wind_speed | 5.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.24e+03 |
| n_updates | 8324 |
-----------------------------------------------
-----------------------------------------------**-------------------------------------------------------------| 38%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 72.48008 |
| air_temperature | 22.753471 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 36.0 |
| direct_solar_radiation | 100.0 |
| hour | 18.0 |
| htg_setpoint | 22.0 |
| month | 5.0 |
| outdoor_humidity | 96.5 |
| outdoor_temperature | 18.091528 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 330.0 |
| wind_speed | 4.65 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.03e+03 |
| n_updates | 8349 |
-----------------------------------------------
-----------------------------------------------**-------------------------------------------------------------| 38%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 41.73392 |
| air_temperature | 28.945398 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 17.0 |
| month | 5.0 |
| outdoor_humidity | 30.5 |
| outdoor_temperature | 20.771217 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 355.0 |
| wind_speed | 5.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.39e+03 |
| n_updates | 8374 |
-----------------------------------------------
-----------------------------------------------**-------------------------------------------------------------| 38%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 60.760983 |
| air_temperature | 27.241547 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 16.0 |
| month | 5.0 |
| outdoor_humidity | 79.5 |
| outdoor_temperature | 18.400848 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 110.0 |
| wind_speed | 2.05 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.16e+03 |
| n_updates | 8399 |
-----------------------------------------------
-----------------------------------------------***------------------------------------------------------------| 39%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 65.40298 |
| air_temperature | 25.17516 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 20.0 |
| month | 5.0 |
| outdoor_humidity | 78.0 |
| outdoor_temperature | 13.861246 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 120.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.23e+03 |
| n_updates | 8424 |
-----------------------------------------------
-----------------------------------------------***------------------------------------------------------------| 39%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 82.80497 |
| air_temperature | 23.545568 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 21.0 |
| month | 5.0 |
| outdoor_humidity | 98.0 |
| outdoor_temperature | 14.885168 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 45.0 |
| wind_speed | 0.75 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.93e+03 |
| n_updates | 8449 |
-----------------------------------------------
-----------------------------------------------***------------------------------------------------------------| 39%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 85.57668 |
| air_temperature | 22.214247 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 20.0 |
| month | 5.0 |
| outdoor_humidity | 73.5 |
| outdoor_temperature | 14.508998 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 250.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.54e+03 |
| n_updates | 8474 |
-----------------------------------------------
-----------------------------------------------****-----------------------------------------------------------| 40%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 83.12802 |
| air_temperature | 22.749151 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 17.0 |
| month | 5.0 |
| outdoor_humidity | 69.5 |
| outdoor_temperature | 13.651919 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 195.0 |
| wind_speed | 4.4 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.92e+03 |
| n_updates | 8499 |
-----------------------------------------------
-----------------------------------------------****-----------------------------------------------------------| 40%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 85.21137 |
| air_temperature | 22.366076 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 22.0 |
| month | 5.0 |
| outdoor_humidity | 59.5 |
| outdoor_temperature | 15.96721 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 330.0 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.61e+03 |
| n_updates | 8524 |
-----------------------------------------------
-----------------------------------------------****-----------------------------------------------------------| 40%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 57.845642 |
| air_temperature | 21.960764 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 19.0 |
| month | 5.0 |
| outdoor_humidity | 53.0 |
| outdoor_temperature | 13.95008 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 260.0 |
| wind_speed | 2.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.06e+03 |
| n_updates | 8549 |
-----------------------------------------------
-----------------------------------------------*****----------------------------------------------------------| 41%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 83.49801 |
| air_temperature | 21.881857 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 19.0 |
| month | 5.0 |
| outdoor_humidity | 88.5 |
| outdoor_temperature | 16.46767 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 265.0 |
| wind_speed | 1.8 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 524 |
| n_updates | 8574 |
-----------------------------------------------
-----------------------------------------------*****----------------------------------------------------------| 41%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 88.733284 |
| air_temperature | 22.166264 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 15.0 |
| month | 5.0 |
| outdoor_humidity | 67.0 |
| outdoor_temperature | 18.524689 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 5.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.88e+03 |
| n_updates | 8599 |
-----------------------------------------------
-----------------------------------------------*****----------------------------------------------------------| 41%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 86.329315 |
| air_temperature | 21.884922 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 83.0 |
| direct_solar_radiation | 194.0 |
| hour | 5.0 |
| htg_setpoint | 19.0 |
| month | 5.0 |
| outdoor_humidity | 85.5 |
| outdoor_temperature | 17.687214 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 7.74e+03 |
| n_updates | 8624 |
-----------------------------------------------
-----------------------------------------------*****----------------------------------------------------------| 41%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 80.705185 |
| air_temperature | 22.81737 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 107.0 |
| direct_solar_radiation | 472.0 |
| hour | 6.0 |
| htg_setpoint | 18.0 |
| month | 6.0 |
| outdoor_humidity | 90.0 |
| outdoor_temperature | 18.693514 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 200.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.75e+03 |
| n_updates | 8649 |
-----------------------------------------------
-----------------------------------------------******---------------------------------------------------------| 42%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 80.16821 |
| air_temperature | 22.976574 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 114.0 |
| direct_solar_radiation | 646.0 |
| hour | 7.0 |
| htg_setpoint | 17.0 |
| month | 6.0 |
| outdoor_humidity | 39.0 |
| outdoor_temperature | 15.119333 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 305.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.08e+03 |
| n_updates | 8674 |
-----------------------------------------------
-----------------------------------------------******---------------------------------------------------------| 42%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 756.69354 |
| air_humidity | 54.817505 |
| air_temperature | 23.335524 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 147.0 |
| direct_solar_radiation | 744.0 |
| hour | 8.0 |
| htg_setpoint | 15.0 |
| month | 6.0 |
| outdoor_humidity | 51.5 |
| outdoor_temperature | 17.913471 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 680939.06 |
| wind_direction | 315.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.77e+03 |
| n_updates | 8699 |
-----------------------------------------------
-----------------------------------------------******---------------------------------------------------------| 42%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 3891.6194 |
| air_humidity | 46.111176 |
| air_temperature | 23.174507 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 253.0 |
| direct_solar_radiation | 639.0 |
| hour | 9.0 |
| htg_setpoint | 17.0 |
| month | 6.0 |
| outdoor_humidity | 26.0 |
| outdoor_temperature | 24.636395 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 3465989.0 |
| wind_direction | 0.0 |
| wind_speed | 2.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.21e+03 |
| n_updates | 8724 |
-----------------------------------------------
-----------------------------------------------*******--------------------------------------------------------| 43%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 3428.4062 |
| air_humidity | 57.121716 |
| air_temperature | 24.138863 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 308.0 |
| direct_solar_radiation | 611.0 |
| hour | 10.0 |
| htg_setpoint | 17.0 |
| month | 6.0 |
| outdoor_humidity | 67.5 |
| outdoor_temperature | 19.792252 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 3085565.8 |
| wind_direction | 195.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.48e+03 |
| n_updates | 8749 |
-----------------------------------------------
-----------------------------------------------*******--------------------------------------------------------| 43%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 1074.4849 |
| air_humidity | 57.604034 |
| air_temperature | 25.964142 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 277.0 |
| direct_solar_radiation | 0.0 |
| hour | 11.0 |
| htg_setpoint | 18.0 |
| month | 6.0 |
| outdoor_humidity | 90.0 |
| outdoor_temperature | 18.815947 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 967036.4 |
| wind_direction | 195.0 |
| wind_speed | 6.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.08e+03 |
| n_updates | 8774 |
-----------------------------------------------
-----------------------------------------------*******--------------------------------------------------------| 43%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 3687.3022 |
| air_humidity | 57.33517 |
| air_temperature | 23.877851 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 368.0 |
| direct_solar_radiation | 466.0 |
| hour | 12.0 |
| htg_setpoint | 15.0 |
| month | 6.0 |
| outdoor_humidity | 54.0 |
| outdoor_temperature | 28.489399 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 3303912.5 |
| wind_direction | 290.0 |
| wind_speed | 5.15 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.61e+03 |
| n_updates | 8799 |
-----------------------------------------------
-----------------------------------------------*******--------------------------------------------------------| 43%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 71.93651 |
| air_temperature | 25.779984 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 408.0 |
| direct_solar_radiation | 373.0 |
| hour | 13.0 |
| htg_setpoint | 15.0 |
| month | 6.0 |
| outdoor_humidity | 50.5 |
| outdoor_temperature | 22.955477 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 355.0 |
| wind_speed | 5.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.39e+03 |
| n_updates | 8824 |
-----------------------------------------------
-----------------------------------------------********-------------------------------------------------------| 44%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 67.38318 |
| air_temperature | 26.963749 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 243.0 |
| direct_solar_radiation | 610.0 |
| hour | 14.0 |
| htg_setpoint | 19.0 |
| month | 6.0 |
| outdoor_humidity | 47.5 |
| outdoor_temperature | 22.373495 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 215.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.63e+03 |
| n_updates | 8849 |
-----------------------------------------------
-----------------------------------------------********-------------------------------------------------------| 44%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 4487.8276 |
| air_humidity | 45.09316 |
| air_temperature | 24.21256 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 238.0 |
| direct_solar_radiation | 338.0 |
| hour | 15.0 |
| htg_setpoint | 20.0 |
| month | 6.0 |
| outdoor_humidity | 38.0 |
| outdoor_temperature | 23.55652 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 4039045.0 |
| wind_direction | 170.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 979 |
| n_updates | 8874 |
-----------------------------------------------
-----------------------------------------------********-------------------------------------------------------| 44%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 4089.4124 |
| air_humidity | 61.17132 |
| air_temperature | 23.19066 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 161.0 |
| direct_solar_radiation | 0.0 |
| hour | 16.0 |
| htg_setpoint | 21.0 |
| month | 6.0 |
| outdoor_humidity | 82.5 |
| outdoor_temperature | 19.431572 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 3680471.0 |
| wind_direction | 145.0 |
| wind_speed | 4.9 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.89e+03 |
| n_updates | 8899 |
-----------------------------------------------
-----------------------------------------------********-------------------------------------------------------| 44%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 52.438007 |
| air_temperature | 27.290419 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 72.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 21.0 |
| month | 6.0 |
| outdoor_humidity | 85.5 |
| outdoor_temperature | 20.92903 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 7.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.86e+03 |
| n_updates | 8924 |
-----------------------------------------------
-----------------------------------------------*********------------------------------------------------------| 45%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 51.02225 |
| air_temperature | 29.40269 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 56.0 |
| direct_solar_radiation | 103.0 |
| hour | 18.0 |
| htg_setpoint | 22.0 |
| month | 6.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | 21.791893 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 7.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.16e+03 |
| n_updates | 8949 |
-----------------------------------------------
-----------------------------------------------*********------------------------------------------------------| 45%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 60.121346 |
| air_temperature | 28.871252 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 22.0 |
| month | 6.0 |
| outdoor_humidity | 85.5 |
| outdoor_temperature | 20.415564 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 200.0 |
| wind_speed | 4.65 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.18e+03 |
| n_updates | 8974 |
-----------------------------------------------
-----------------------------------------------*********------------------------------------------------------| 45%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 68.434044 |
| air_temperature | 27.172163 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 17.0 |
| month | 6.0 |
| outdoor_humidity | 85.5 |
| outdoor_temperature | 23.622412 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 95.0 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.05e+03 |
| n_updates | 8999 |
-----------------------------------------------
-----------------------------------------------**********-----------------------------------------------------| 46%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 78.2435 |
| air_temperature | 24.923763 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 20.0 |
| month | 6.0 |
| outdoor_humidity | 88.5 |
| outdoor_temperature | 19.389812 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 90.0 |
| wind_speed | 2.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.67e+03 |
| n_updates | 9024 |
-----------------------------------------------
-----------------------------------------------**********-----------------------------------------------------| 46%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 74.24047 |
| air_temperature | 25.115957 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 17.0 |
| month | 6.0 |
| outdoor_humidity | 94.5 |
| outdoor_temperature | 18.886944 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 195.0 |
| wind_speed | 4.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.76e+03 |
| n_updates | 9049 |
-----------------------------------------------
-----------------------------------------------**********-----------------------------------------------------| 46%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 77.20639 |
| air_temperature | 24.541458 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 18.0 |
| month | 6.0 |
| outdoor_humidity | 86.0 |
| outdoor_temperature | 22.951208 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 235.0 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3e+03 |
| n_updates | 9074 |
-----------------------------------------------
-----------------------------------------------***********----------------------------------------------------| 47%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 78.05541 |
| air_temperature | 25.311417 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 20.0 |
| month | 6.0 |
| outdoor_humidity | 77.5 |
| outdoor_temperature | 23.508722 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 90.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.19e+03 |
| n_updates | 9099 |
-----------------------------------------------
-----------------------------------------------***********----------------------------------------------------| 47%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 80.42342 |
| air_temperature | 23.215261 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 16.0 |
| month | 6.0 |
| outdoor_humidity | 91.5 |
| outdoor_temperature | 19.310314 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 195.0 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.12e+03 |
| n_updates | 9124 |
-----------------------------------------------
-----------------------------------------------***********----------------------------------------------------| 47%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 81.96147 |
| air_temperature | 23.621758 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 18.0 |
| month | 6.0 |
| outdoor_humidity | 71.0 |
| outdoor_temperature | 24.771341 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 325.0 |
| wind_speed | 4.65 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.11e+03 |
| n_updates | 9149 |
-----------------------------------------------
-----------------------------------------------***********----------------------------------------------------| 47%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 77.23984 |
| air_temperature | 24.676752 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 22.0 |
| month | 6.0 |
| outdoor_humidity | 67.0 |
| outdoor_temperature | 22.543236 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 265.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 934 |
| n_updates | 9174 |
-----------------------------------------------
-----------------------------------------------************---------------------------------------------------| 48%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 85.025604 |
| air_temperature | 23.013933 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 3.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 20.0 |
| month | 6.0 |
| outdoor_humidity | 94.5 |
| outdoor_temperature | 21.945906 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 165.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.06e+03 |
| n_updates | 9199 |
-----------------------------------------------
-----------------------------------------------************---------------------------------------------------| 48%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 90.37305 |
| air_temperature | 22.211245 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 23.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 19.0 |
| month | 6.0 |
| outdoor_humidity | 98.0 |
| outdoor_temperature | 23.28667 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 95.0 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.53e+03 |
| n_updates | 9224 |
-----------------------------------------------
-----------------------------------------------************---------------------------------------------------| 48%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 84.31747 |
| air_temperature | 23.182339 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 67.0 |
| direct_solar_radiation | 604.0 |
| hour | 6.0 |
| htg_setpoint | 16.0 |
| month | 6.0 |
| outdoor_humidity | 65.0 |
| outdoor_temperature | 22.826544 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 260.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.78e+03 |
| n_updates | 9249 |
-----------------------------------------------
-----------------------------------------------*************--------------------------------------------------| 49%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 2593.8738 |
| air_humidity | 78.85662 |
| air_temperature | 20.91998 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 248.0 |
| direct_solar_radiation | 113.0 |
| hour | 7.0 |
| htg_setpoint | 15.0 |
| month | 6.0 |
| outdoor_humidity | 88.5 |
| outdoor_temperature | 23.730545 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2297572.2 |
| wind_direction | 205.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.1e+03 |
| n_updates | 9274 |
-----------------------------------------------
-----------------------------------------------*************--------------------------------------------------| 49%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 4826.358 |
| air_humidity | 69.02169 |
| air_temperature | 21.617256 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 341.0 |
| direct_solar_radiation | 83.0 |
| hour | 8.0 |
| htg_setpoint | 20.0 |
| month | 6.0 |
| outdoor_humidity | 85.5 |
| outdoor_temperature | 21.108011 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 4406347.5 |
| wind_direction | 205.0 |
| wind_speed | 4.65 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.86e+03 |
| n_updates | 9299 |
-----------------------------------------------
-----------------------------------------------*************--------------------------------------------------| 49%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 80.93762 |
| air_temperature | 23.177649 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 161.0 |
| direct_solar_radiation | 0.0 |
| hour | 9.0 |
| htg_setpoint | 20.0 |
| month | 6.0 |
| outdoor_humidity | 85.5 |
| outdoor_temperature | 22.97749 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 80.0 |
| wind_speed | 3.65 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.56e+03 |
| n_updates | 9324 |
-----------------------------------------------
-----------------------------------------------*************--------------------------------------------------| 49%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 76.66805 |
| air_temperature | 24.069008 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 322.0 |
| direct_solar_radiation | 0.0 |
| hour | 10.0 |
| htg_setpoint | 21.0 |
| month | 6.0 |
| outdoor_humidity | 77.5 |
| outdoor_temperature | 21.572285 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 4.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 573 |
| n_updates | 9349 |
-----------------------------------------------
-----------------------------------------------**************-------------------------------------------------| 50%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 4960.5977 |
| air_humidity | 57.92844 |
| air_temperature | 24.07896 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 267.0 |
| direct_solar_radiation | 534.0 |
| hour | 11.0 |
| htg_setpoint | 18.0 |
| month | 7.0 |
| outdoor_humidity | 58.0 |
| outdoor_temperature | 25.835115 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 4457515.5 |
| wind_direction | 190.0 |
| wind_speed | 5.15 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.01e+03 |
| n_updates | 9374 |
-----------------------------------------------
-----------------------------------------------**************-------------------------------------------------| 50%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 6130.1475 |
| air_humidity | 57.217648 |
| air_temperature | 23.999527 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 156.0 |
| direct_solar_radiation | 846.0 |
| hour | 12.0 |
| htg_setpoint | 21.0 |
| month | 7.0 |
| outdoor_humidity | 66.5 |
| outdoor_temperature | 25.839724 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 5570390.0 |
| wind_direction | 200.0 |
| wind_speed | 5.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.75e+03 |
| n_updates | 9399 |
-----------------------------------------------
-----------------------------------------------**************-------------------------------------------------| 50%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 6180.046 |
| air_humidity | 57.915764 |
| air_temperature | 23.824299 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 319.0 |
| direct_solar_radiation | 0.0 |
| hour | 13.0 |
| htg_setpoint | 22.0 |
| month | 7.0 |
| outdoor_humidity | 54.0 |
| outdoor_temperature | 27.998598 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 5562041.0 |
| wind_direction | 45.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.41e+03 |
| n_updates | 9424 |
-----------------------------------------------
-----------------------------------------------**************-------------------------------------------------| 50%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 5814.8086 |
| air_humidity | 57.336285 |
| air_temperature | 24.416656 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 240.0 |
| direct_solar_radiation | 639.0 |
| hour | 14.0 |
| htg_setpoint | 21.0 |
| month | 7.0 |
| outdoor_humidity | 42.0 |
| outdoor_temperature | 28.857347 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 5211308.0 |
| wind_direction | 145.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.25e+03 |
| n_updates | 9449 |
-----------------------------------------------
-----------------------------------------------***************------------------------------------------------| 51%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 8071.458 |
| air_humidity | 59.186604 |
| air_temperature | 24.030064 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 217.0 |
| direct_solar_radiation | 565.0 |
| hour | 15.0 |
| htg_setpoint | 18.0 |
| month | 7.0 |
| outdoor_humidity | 77.0 |
| outdoor_temperature | 26.988108 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 7237952.0 |
| wind_direction | 180.0 |
| wind_speed | 4.65 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 579 |
| n_updates | 9474 |
-----------------------------------------------
-----------------------------------------------***************------------------------------------------------| 51%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 59.32995 |
| air_temperature | 29.440195 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 132.0 |
| direct_solar_radiation | 584.0 |
| hour | 16.0 |
| htg_setpoint | 22.0 |
| month | 7.0 |
| outdoor_humidity | 72.0 |
| outdoor_temperature | 29.04921 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.13e+03 |
| n_updates | 9499 |
-----------------------------------------------
-----------------------------------------------***************------------------------------------------------| 51%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 58.903008 |
| air_temperature | 29.58267 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 122.0 |
| direct_solar_radiation | 263.0 |
| hour | 17.0 |
| htg_setpoint | 21.0 |
| month | 7.0 |
| outdoor_humidity | 68.5 |
| outdoor_temperature | 27.491972 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 195.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.14e+03 |
| n_updates | 9524 |
-----------------------------------------------
-----------------------------------------------***************------------------------------------------------| 51%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 54.37169 |
| air_temperature | 28.805046 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 54.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 18.0 |
| month | 7.0 |
| outdoor_humidity | 90.5 |
| outdoor_temperature | 23.013592 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 110.0 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.59e+03 |
| n_updates | 9549 |
-----------------------------------------------
-----------------------------------------------****************-----------------------------------------------| 52%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 56.407288 |
| air_temperature | 29.713375 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 18.0 |
| month | 7.0 |
| outdoor_humidity | 83.5 |
| outdoor_temperature | 27.13691 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 200.0 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.97e+03 |
| n_updates | 9574 |
-----------------------------------------------
-----------------------------------------------****************-----------------------------------------------| 52%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 59.091957 |
| air_temperature | 28.77042 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 21.0 |
| month | 7.0 |
| outdoor_humidity | 50.5 |
| outdoor_temperature | 29.924786 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 290.0 |
| wind_speed | 5.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.35e+04 |
| n_updates | 9599 |
-----------------------------------------------
-----------------------------------------------****************-----------------------------------------------| 52%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 62.7617 |
| air_temperature | 27.150831 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 21.0 |
| month | 7.0 |
| outdoor_humidity | 60.0 |
| outdoor_temperature | 23.236689 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 205.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.05e+03 |
| n_updates | 9624 |
-----------------------------------------------
-----------------------------------------------*****************----------------------------------------------| 53%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 67.4881 |
| air_temperature | 25.895061 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 15.0 |
| month | 7.0 |
| outdoor_humidity | 77.5 |
| outdoor_temperature | 24.448812 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 225.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.16e+03 |
| n_updates | 9649 |
-----------------------------------------------
-----------------------------------------------*****************----------------------------------------------| 53%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 65.81631 |
| air_temperature | 26.319372 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 20.0 |
| month | 7.0 |
| outdoor_humidity | 69.5 |
| outdoor_temperature | 26.388847 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 255.0 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.84e+03 |
| n_updates | 9674 |
-----------------------------------------------
-----------------------------------------------*****************----------------------------------------------| 53%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 75.7209 |
| air_temperature | 23.97351 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 21.0 |
| month | 7.0 |
| outdoor_humidity | 98.0 |
| outdoor_temperature | 21.806433 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 90.0 |
| wind_speed | 5.65 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.57e+03 |
| n_updates | 9699 |
-----------------------------------------------
-----------------------------------------------******************---------------------------------------------| 54%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 80.57992 |
| air_temperature | 23.869347 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 20.0 |
| month | 7.0 |
| outdoor_humidity | 94.0 |
| outdoor_temperature | 23.162914 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 115.0 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.64e+03 |
| n_updates | 9724 |
-----------------------------------------------
-----------------------------------------------******************---------------------------------------------| 54%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 83.63909 |
| air_temperature | 23.794798 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 16.0 |
| month | 7.0 |
| outdoor_humidity | 66.5 |
| outdoor_temperature | 24.551136 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 5.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.5e+03 |
| n_updates | 9749 |
-----------------------------------------------
-----------------------------------------------******************---------------------------------------------| 54%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 87.72499 |
| air_temperature | 23.30253 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 21.0 |
| month | 7.0 |
| outdoor_humidity | 89.0 |
| outdoor_temperature | 22.862804 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 115.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.5e+03 |
| n_updates | 9774 |
-----------------------------------------------
-----------------------------------------------******************---------------------------------------------| 54%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 89.009865 |
| air_temperature | 22.375265 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 20.0 |
| month | 7.0 |
| outdoor_humidity | 90.0 |
| outdoor_temperature | 21.891815 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 115.0 |
| wind_speed | 1.8 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.84e+03 |
| n_updates | 9799 |
-----------------------------------------------
-----------------------------------------------*******************--------------------------------------------| 55%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 88.44114 |
| air_temperature | 23.95633 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 55.0 |
| direct_solar_radiation | 9.0 |
| hour | 5.0 |
| htg_setpoint | 18.0 |
| month | 7.0 |
| outdoor_humidity | 95.0 |
| outdoor_temperature | 24.555973 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 210.0 |
| wind_speed | 2.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.1e+03 |
| n_updates | 9824 |
-----------------------------------------------
-----------------------------------------------*******************--------------------------------------------| 55%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 86.25535 |
| air_temperature | 24.365273 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 75.0 |
| direct_solar_radiation | 0.0 |
| hour | 6.0 |
| htg_setpoint | 22.0 |
| month | 7.0 |
| outdoor_humidity | 90.0 |
| outdoor_temperature | 26.62452 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 230.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 973 |
| n_updates | 9849 |
-----------------------------------------------
-----------------------------------------------*******************--------------------------------------------| 55%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 3477.5361 |
| air_humidity | 82.9503 |
| air_temperature | 21.206257 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 199.0 |
| direct_solar_radiation | 43.0 |
| hour | 7.0 |
| htg_setpoint | 16.0 |
| month | 7.0 |
| outdoor_humidity | 85.0 |
| outdoor_temperature | 26.23461 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 3106383.2 |
| wind_direction | 215.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.29e+03 |
| n_updates | 9874 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 56%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 6591.9224 |
| air_humidity | 68.924515 |
| air_temperature | 21.636711 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 181.0 |
| direct_solar_radiation | 0.0 |
| hour | 8.0 |
| htg_setpoint | 16.0 |
| month | 7.0 |
| outdoor_humidity | 85.0 |
| outdoor_temperature | 24.490088 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 6105625.0 |
| wind_direction | 205.0 |
| wind_speed | 5.4 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.13e+03 |
| n_updates | 9899 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 56%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 6579.233 |
| air_humidity | 62.79068 |
| air_temperature | 22.80446 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 212.0 |
| direct_solar_radiation | 618.0 |
| hour | 9.0 |
| htg_setpoint | 22.0 |
| month | 7.0 |
| outdoor_humidity | 78.5 |
| outdoor_temperature | 25.128534 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 5908606.0 |
| wind_direction | 240.0 |
| wind_speed | 2.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.93e+03 |
| n_updates | 9924 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 56%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 8556.943 |
| air_humidity | 58.34476 |
| air_temperature | 24.146399 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 443.0 |
| direct_solar_radiation | 298.0 |
| hour | 10.0 |
| htg_setpoint | 17.0 |
| month | 7.0 |
| outdoor_humidity | 61.0 |
| outdoor_temperature | 30.291105 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 7701249.0 |
| wind_direction | 245.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.23e+03 |
| n_updates | 9949 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 56%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 6612.603 |
| air_humidity | 58.736492 |
| air_temperature | 23.865717 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 207.0 |
| direct_solar_radiation | 705.0 |
| hour | 11.0 |
| htg_setpoint | 15.0 |
| month | 7.0 |
| outdoor_humidity | 76.5 |
| outdoor_temperature | 26.542692 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 5951342.5 |
| wind_direction | 190.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.36e+04 |
| n_updates | 9974 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 57%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 68.82043 |
| air_temperature | 26.714127 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 384.0 |
| direct_solar_radiation | 370.0 |
| hour | 12.0 |
| htg_setpoint | 17.0 |
| month | 7.0 |
| outdoor_humidity | 48.0 |
| outdoor_temperature | 30.392218 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 255.0 |
| wind_speed | 2.3 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.49e+03 |
| n_updates | 9999 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 57%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 71.67858 |
| air_temperature | 26.007689 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 248.0 |
| direct_solar_radiation | 0.0 |
| hour | 13.0 |
| htg_setpoint | 16.0 |
| month | 7.0 |
| outdoor_humidity | 84.5 |
| outdoor_temperature | 24.04101 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 210.0 |
| wind_speed | 6.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 804 |
| n_updates | 10024 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 57%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 6615.9927 |
| air_humidity | 58.55262 |
| air_temperature | 24.042582 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 289.0 |
| direct_solar_radiation | 484.0 |
| hour | 14.0 |
| htg_setpoint | 21.0 |
| month | 7.0 |
| outdoor_humidity | 61.0 |
| outdoor_temperature | 26.934774 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 5924812.5 |
| wind_direction | 195.0 |
| wind_speed | 4.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.87e+03 |
| n_updates | 10049 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 57%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 7803.733 |
| air_humidity | 58.594456 |
| air_temperature | 24.192398 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 234.0 |
| direct_solar_radiation | 372.0 |
| hour | 15.0 |
| htg_setpoint | 22.0 |
| month | 7.0 |
| outdoor_humidity | 79.0 |
| outdoor_temperature | 25.361639 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 7023360.0 |
| wind_direction | 185.0 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.38e+03 |
| n_updates | 10074 |
-----------------------------------------------
-----------------------------------------------*********************------------------------------------------| 58%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 6048.8022 |
| air_humidity | 59.633774 |
| air_temperature | 23.914898 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 269.0 |
| direct_solar_radiation | 84.0 |
| hour | 16.0 |
| htg_setpoint | 19.0 |
| month | 7.0 |
| outdoor_humidity | 64.0 |
| outdoor_temperature | 25.906448 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 5443922.0 |
| wind_direction | 195.0 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.34e+03 |
| n_updates | 10099 |
-----------------------------------------------
-----------------------------------------------*********************------------------------------------------| 58%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 48.285763 |
| air_temperature | 29.087503 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 83.0 |
| direct_solar_radiation | 401.0 |
| hour | 17.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 61.5 |
| outdoor_temperature | 27.432545 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 195.0 |
| wind_speed | 7.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.25e+03 |
| n_updates | 10124 |
-----------------------------------------------
-----------------------------------------------*********************------------------------------------------| 58%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 50.60229 |
| air_temperature | 30.327057 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 34.0 |
| direct_solar_radiation | 128.0 |
| hour | 18.0 |
| htg_setpoint | 22.0 |
| month | 8.0 |
| outdoor_humidity | 78.0 |
| outdoor_temperature | 26.598967 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 185.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.19e+03 |
| n_updates | 10149 |
-----------------------------------------------
-----------------------------------------------***********************----------------------------------------| 59%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 61.864407 |
| air_temperature | 28.372997 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 22.0 |
| month | 8.0 |
| outdoor_humidity | 92.0 |
| outdoor_temperature | 26.0429 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 180.0 |
| wind_speed | 5.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.11e+03 |
| n_updates | 10174 |
-----------------------------------------------
-----------------------------------------------***********************----------------------------------------| 59%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 61.675846 |
| air_temperature | 28.290186 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 20.0 |
| month | 8.0 |
| outdoor_humidity | 51.0 |
| outdoor_temperature | 31.614428 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 280.0 |
| wind_speed | 5.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 8.52e+03 |
| n_updates | 10199 |
-----------------------------------------------
-----------------------------------------------***********************----------------------------------------| 59%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 72.12505 |
| air_temperature | 26.204573 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 92.0 |
| outdoor_temperature | 26.163172 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.82e+03 |
| n_updates | 10224 |
-----------------------------------------------
-----------------------------------------------***********************----------------------------------------| 59%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 78.884186 |
| air_temperature | 24.134598 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | 20.089655 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 60.0 |
| wind_speed | 4.65 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.96e+03 |
| n_updates | 10249 |
-----------------------------------------------
-----------------------------------------------************************---------------------------------------| 60%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 76.48503 |
| air_temperature | 24.204605 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 22.0 |
| month | 8.0 |
| outdoor_humidity | 67.5 |
| outdoor_temperature | 15.094099 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 60.0 |
| wind_speed | 2.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.56e+03 |
| n_updates | 10274 |
-----------------------------------------------
-----------------------------------------------************************---------------------------------------| 60%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 79.38634 |
| air_temperature | 23.735037 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 75.0 |
| outdoor_temperature | 17.988451 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 30.0 |
| wind_speed | 1.05 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.87e+03 |
| n_updates | 10299 |
-----------------------------------------------
-----------------------------------------------************************---------------------------------------| 60%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 76.351166 |
| air_temperature | 23.945112 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 22.0 |
| month | 8.0 |
| outdoor_humidity | 82.5 |
| outdoor_temperature | 19.52576 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 245.0 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.56e+03 |
| n_updates | 10324 |
-----------------------------------------------
-----------------------------------------------*************************--------------------------------------| 61%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 74.00164 |
| air_temperature | 24.441584 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 20.0 |
| month | 8.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 22.688526 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 250.0 |
| wind_speed | 2.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.36e+03 |
| n_updates | 10349 |
-----------------------------------------------
-----------------------------------------------*************************--------------------------------------| 61%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 77.23835 |
| air_temperature | 23.654684 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 20.0 |
| month | 8.0 |
| outdoor_humidity | 94.0 |
| outdoor_temperature | 23.293741 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 215.0 |
| wind_speed | 2.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.17e+03 |
| n_updates | 10374 |
-----------------------------------------------
-----------------------------------------------*************************--------------------------------------| 61%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 80.260864 |
| air_temperature | 23.570625 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 16.0 |
| month | 8.0 |
| outdoor_humidity | 67.5 |
| outdoor_temperature | 23.852522 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 355.0 |
| wind_speed | 4.65 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.33e+03 |
| n_updates | 10399 |
-----------------------------------------------
-----------------------------------------------**************************-------------------------------------| 62%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 83.553215 |
| air_temperature | 22.783445 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 25.0 |
| direct_solar_radiation | 12.0 |
| hour | 5.0 |
| htg_setpoint | 20.0 |
| month | 8.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | 23.652273 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 240.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.37e+03 |
| n_updates | 10424 |
-----------------------------------------------
-----------------------------------------------**************************-------------------------------------| 62%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 89.74817 |
| air_temperature | 22.861992 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 96.0 |
| direct_solar_radiation | 78.0 |
| hour | 6.0 |
| htg_setpoint | 22.0 |
| month | 8.0 |
| outdoor_humidity | 85.0 |
| outdoor_temperature | 22.354202 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 155.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.64e+03 |
| n_updates | 10449 |
-----------------------------------------------
-----------------------------------------------**************************-------------------------------------| 62%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 2615.6394 |
| air_humidity | 76.129715 |
| air_temperature | 22.001867 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 74.0 |
| direct_solar_radiation | 0.0 |
| hour | 7.0 |
| htg_setpoint | 22.0 |
| month | 8.0 |
| outdoor_humidity | 89.0 |
| outdoor_temperature | 24.034338 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2354075.5 |
| wind_direction | 55.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.4e+04 |
| n_updates | 10474 |
-----------------------------------------------
-----------------------------------------------**************************-------------------------------------| 62%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 80.63669 |
| air_temperature | 24.72616 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 226.0 |
| direct_solar_radiation | 57.0 |
| hour | 8.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 78.0 |
| outdoor_temperature | 28.858715 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 50.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.43e+03 |
| n_updates | 10499 |
-----------------------------------------------
-----------------------------------------------***************************------------------------------------| 63%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 71.93833 |
| air_temperature | 26.650896 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 248.0 |
| direct_solar_radiation | 491.0 |
| hour | 9.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 38.0 |
| outdoor_temperature | 30.86335 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 35.0 |
| wind_speed | 7.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.17e+03 |
| n_updates | 10524 |
-----------------------------------------------
-----------------------------------------------***************************------------------------------------| 63%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 4059.3743 |
| air_humidity | 56.83726 |
| air_temperature | 23.812122 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 237.0 |
| direct_solar_radiation | 678.0 |
| hour | 10.0 |
| htg_setpoint | 15.0 |
| month | 8.0 |
| outdoor_humidity | 39.0 |
| outdoor_temperature | 26.226334 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 3653437.0 |
| wind_direction | 45.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.63e+03 |
| n_updates | 10549 |
-----------------------------------------------
-----------------------------------------------***************************------------------------------------| 63%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 4578.8765 |
| air_humidity | 49.022327 |
| air_temperature | 23.843649 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 261.0 |
| direct_solar_radiation | 675.0 |
| hour | 11.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 32.0 |
| outdoor_temperature | 26.02081 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 4099537.5 |
| wind_direction | 10.0 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.48e+03 |
| n_updates | 10574 |
-----------------------------------------------
-----------------------------------------------***************************------------------------------------| 63%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 6744.505 |
| air_humidity | 57.16785 |
| air_temperature | 23.750113 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 141.0 |
| direct_solar_radiation | 849.0 |
| hour | 12.0 |
| htg_setpoint | 22.0 |
| month | 8.0 |
| outdoor_humidity | 42.0 |
| outdoor_temperature | 31.268843 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 6105524.0 |
| wind_direction | 230.0 |
| wind_speed | 5.4 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.09e+04 |
| n_updates | 10599 |
-----------------------------------------------
-----------------------------------------------****************************-----------------------------------| 64%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 5744.1646 |
| air_humidity | 53.9114 |
| air_temperature | 24.341438 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 177.0 |
| direct_solar_radiation | 677.0 |
| hour | 13.0 |
| htg_setpoint | 20.0 |
| month | 8.0 |
| outdoor_humidity | 30.0 |
| outdoor_temperature | 31.381172 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 5169748.0 |
| wind_direction | 325.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.62e+03 |
| n_updates | 10624 |
-----------------------------------------------
-----------------------------------------------****************************-----------------------------------| 64%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 4735.62 |
| air_humidity | 54.2698 |
| air_temperature | 24.242298 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 281.0 |
| direct_solar_radiation | 478.0 |
| hour | 14.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 42.5 |
| outdoor_temperature | 25.902674 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 4254899.5 |
| wind_direction | 195.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 7.54e+03 |
| n_updates | 10649 |
-----------------------------------------------
-----------------------------------------------****************************-----------------------------------| 64%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 60.763294 |
| air_temperature | 28.425394 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 237.0 |
| direct_solar_radiation | 330.0 |
| hour | 15.0 |
| htg_setpoint | 22.0 |
| month | 8.0 |
| outdoor_humidity | 27.0 |
| outdoor_temperature | 34.236084 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 300.0 |
| wind_speed | 7.75 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 997 |
| n_updates | 10674 |
-----------------------------------------------
-----------------------------------------------*****************************----------------------------------| 65%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 63.033356 |
| air_temperature | 27.930851 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 116.0 |
| direct_solar_radiation | 463.0 |
| hour | 16.0 |
| htg_setpoint | 20.0 |
| month | 8.0 |
| outdoor_humidity | 28.0 |
| outdoor_temperature | 26.911022 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 40.0 |
| wind_speed | 4.9 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.25e+03 |
| n_updates | 10699 |
-----------------------------------------------
-----------------------------------------------*****************************----------------------------------| 65%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 49.931183 |
| air_temperature | 28.225517 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 91.0 |
| direct_solar_radiation | 117.0 |
| hour | 17.0 |
| htg_setpoint | 22.0 |
| month | 8.0 |
| outdoor_humidity | 61.0 |
| outdoor_temperature | 23.85916 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 185.0 |
| wind_speed | 8.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 837 |
| n_updates | 10724 |
-----------------------------------------------
-----------------------------------------------*****************************----------------------------------| 65%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 52.26508 |
| air_temperature | 29.295475 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 7.0 |
| direct_solar_radiation | 2.0 |
| hour | 18.0 |
| htg_setpoint | 22.0 |
| month | 8.0 |
| outdoor_humidity | 79.0 |
| outdoor_temperature | 24.975166 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 110.0 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 781 |
| n_updates | 10749 |
-----------------------------------------------
-----------------------------------------------*****************************----------------------------------| 65%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 50.48178 |
| air_temperature | 28.82346 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 22.0 |
| month | 8.0 |
| outdoor_humidity | 53.0 |
| outdoor_temperature | 19.281387 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 95.0 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.08e+03 |
| n_updates | 10774 |
-----------------------------------------------
-----------------------------------------------******************************---------------------------------| 66%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 59.380795 |
| air_temperature | 27.832817 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 16.0 |
| month | 8.0 |
| outdoor_humidity | 72.0 |
| outdoor_temperature | 21.996769 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 170.0 |
| wind_speed | 1.5 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.49e+03 |
| n_updates | 10799 |
-----------------------------------------------
-----------------------------------------------******************************---------------------------------| 66%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 44.240105 |
| air_temperature | 26.890566 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 15.0 |
| month | 8.0 |
| outdoor_humidity | 66.0 |
| outdoor_temperature | 22.243492 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 215.0 |
| wind_speed | 2.05 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.04e+03 |
| n_updates | 10824 |
-----------------------------------------------
-----------------------------------------------******************************---------------------------------| 66%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 46.84637 |
| air_temperature | 25.793453 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 15.0 |
| month | 8.0 |
| outdoor_humidity | 70.0 |
| outdoor_temperature | 23.776503 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 280.0 |
| wind_speed | 4.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.06e+03 |
| n_updates | 10849 |
-----------------------------------------------
-----------------------------------------------******************************---------------------------------| 66%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 47.336395 |
| air_temperature | 25.626314 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 18.0 |
| month | 9.0 |
| outdoor_humidity | 42.0 |
| outdoor_temperature | 25.760303 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 335.0 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.45e+03 |
| n_updates | 10874 |
-----------------------------------------------
-----------------------------------------------*******************************--------------------------------| 67%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 80.21505 |
| air_temperature | 23.487644 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 19.0 |
| month | 9.0 |
| outdoor_humidity | 59.0 |
| outdoor_temperature | 21.056015 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 30.0 |
| wind_speed | 2.05 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.11e+03 |
| n_updates | 10899 |
-----------------------------------------------
-----------------------------------------------*******************************--------------------------------| 67%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 82.421844 |
| air_temperature | 23.507233 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 21.0 |
| month | 9.0 |
| outdoor_humidity | 73.0 |
| outdoor_temperature | 20.46493 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 265.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.7e+03 |
| n_updates | 10924 |
-----------------------------------------------
-----------------------------------------------********************************-------------------------------| 68%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 85.71846 |
| air_temperature | 23.002916 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 22.0 |
| month | 9.0 |
| outdoor_humidity | 76.0 |
| outdoor_temperature | 19.411385 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 225.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.18e+03 |
| n_updates | 10949 |
-----------------------------------------------
-----------------------------------------------********************************-------------------------------| 68%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 85.809654 |
| air_temperature | 22.716314 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 20.0 |
| month | 9.0 |
| outdoor_humidity | 81.0 |
| outdoor_temperature | 19.075342 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 270.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.88e+03 |
| n_updates | 10974 |
-----------------------------------------------
-----------------------------------------------********************************-------------------------------| 68%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 79.86444 |
| air_temperature | 23.607555 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 20.0 |
| month | 9.0 |
| outdoor_humidity | 92.0 |
| outdoor_temperature | 22.198689 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 205.0 |
| wind_speed | 2.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.15e+03 |
| n_updates | 10999 |
-----------------------------------------------
-----------------------------------------------********************************-------------------------------| 68%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 80.55953 |
| air_temperature | 23.390306 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 20.0 |
| month | 9.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 20.709194 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 225.0 |
| wind_speed | 2.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.7e+03 |
| n_updates | 11024 |
-----------------------------------------------
-----------------------------------------------*********************************------------------------------| 69%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 90.97892 |
| air_temperature | 21.430555 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 35.0 |
| direct_solar_radiation | 0.0 |
| hour | 6.0 |
| htg_setpoint | 19.0 |
| month | 9.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | 18.381512 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 65.0 |
| wind_speed | 2.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.54e+04 |
| n_updates | 11049 |
-----------------------------------------------
-----------------------------------------------*********************************------------------------------| 69%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 522.8672 |
| air_humidity | 80.94645 |
| air_temperature | 21.47948 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 60.0 |
| direct_solar_radiation | 691.0 |
| hour | 7.0 |
| htg_setpoint | 18.0 |
| month | 9.0 |
| outdoor_humidity | 56.0 |
| outdoor_temperature | 18.247702 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 470580.44 |
| wind_direction | 340.0 |
| wind_speed | 5.15 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.29e+03 |
| n_updates | 11074 |
-----------------------------------------------
-----------------------------------------------*********************************------------------------------| 69%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 330.18005 |
| air_humidity | 49.793545 |
| air_temperature | 22.299383 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 147.0 |
| direct_solar_radiation | 597.0 |
| hour | 8.0 |
| htg_setpoint | 21.0 |
| month | 9.0 |
| outdoor_humidity | 44.5 |
| outdoor_temperature | 14.609633 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 292652.06 |
| wind_direction | 40.0 |
| wind_speed | 4.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.8e+03 |
| n_updates | 11099 |
-----------------------------------------------
-----------------------------------------------*********************************------------------------------| 69%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 2898.547 |
| air_humidity | 53.278496 |
| air_temperature | 22.61949 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 277.0 |
| direct_solar_radiation | 436.0 |
| hour | 9.0 |
| htg_setpoint | 20.0 |
| month | 9.0 |
| outdoor_humidity | 47.5 |
| outdoor_temperature | 20.475954 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 2573731.5 |
| wind_direction | 245.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.89e+03 |
| n_updates | 11124 |
-----------------------------------------------
-----------------------------------------------**********************************-----------------------------| 70%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 6191.668 |
| air_humidity | 61.839733 |
| air_temperature | 23.132393 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 315.0 |
| direct_solar_radiation | 94.0 |
| hour | 10.0 |
| htg_setpoint | 15.0 |
| month | 9.0 |
| outdoor_humidity | 82.0 |
| outdoor_temperature | 24.937683 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 5546784.0 |
| wind_direction | 210.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 8.82e+03 |
| n_updates | 11149 |
-----------------------------------------------
-----------------------------------------------**********************************-----------------------------| 70%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 77.328 |
| air_temperature | 25.108599 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 226.0 |
| direct_solar_radiation | 591.0 |
| hour | 11.0 |
| htg_setpoint | 20.0 |
| month | 9.0 |
| outdoor_humidity | 72.0 |
| outdoor_temperature | 27.525572 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 240.0 |
| wind_speed | 6.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.11e+03 |
| n_updates | 11174 |
-----------------------------------------------
-----------------------------------------------**********************************-----------------------------| 70%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 75.90909 |
| air_temperature | 25.643183 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 273.0 |
| direct_solar_radiation | 344.0 |
| hour | 12.0 |
| htg_setpoint | 22.0 |
| month | 9.0 |
| outdoor_humidity | 44.0 |
| outdoor_temperature | 21.85953 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 305.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.48e+03 |
| n_updates | 11199 |
-----------------------------------------------
-----------------------------------------------***********************************----------------------------| 71%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 3087.9355 |
| air_humidity | 49.51548 |
| air_temperature | 23.905464 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 420.0 |
| direct_solar_radiation | 123.0 |
| hour | 13.0 |
| htg_setpoint | 15.0 |
| month | 9.0 |
| outdoor_humidity | 52.0 |
| outdoor_temperature | 20.520428 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 2776982.5 |
| wind_direction | 135.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.28e+03 |
| n_updates | 11224 |
-----------------------------------------------
-----------------------------------------------***********************************----------------------------| 71%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 3701.2773 |
| air_humidity | 59.4393 |
| air_temperature | 23.357481 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 161.0 |
| direct_solar_radiation | 0.0 |
| hour | 14.0 |
| htg_setpoint | 18.0 |
| month | 9.0 |
| outdoor_humidity | 79.5 |
| outdoor_temperature | 19.842611 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 3331149.5 |
| wind_direction | 330.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.16e+03 |
| n_updates | 11249 |
-----------------------------------------------
-----------------------------------------------***********************************----------------------------| 71%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 4359.327 |
| air_humidity | 52.05942 |
| air_temperature | 24.033459 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 166.0 |
| direct_solar_radiation | 134.0 |
| hour | 15.0 |
| htg_setpoint | 21.0 |
| month | 9.0 |
| outdoor_humidity | 43.0 |
| outdoor_temperature | 24.21186 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 3923394.2 |
| wind_direction | 20.0 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.76e+03 |
| n_updates | 11274 |
-----------------------------------------------
-----------------------------------------------***********************************----------------------------| 71%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 3904.9595 |
| air_humidity | 58.766552 |
| air_temperature | 23.823164 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 87.0 |
| direct_solar_radiation | 308.0 |
| hour | 16.0 |
| htg_setpoint | 22.0 |
| month | 9.0 |
| outdoor_humidity | 58.0 |
| outdoor_temperature | 21.326008 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 3514463.5 |
| wind_direction | 100.0 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.39e+03 |
| n_updates | 11299 |
-----------------------------------------------
-----------------------------------------------************************************---------------------------| 72%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 48.20776 |
| air_temperature | 28.272985 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 19.0 |
| direct_solar_radiation | 8.0 |
| hour | 17.0 |
| htg_setpoint | 15.0 |
| month | 9.0 |
| outdoor_humidity | 70.5 |
| outdoor_temperature | 18.32586 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 160.0 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 863 |
| n_updates | 11324 |
-----------------------------------------------
-----------------------------------------------************************************---------------------------| 72%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 72.10212 |
| air_temperature | 25.217432 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 22.0 |
| month | 9.0 |
| outdoor_humidity | 90.0 |
| outdoor_temperature | 22.660082 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 180.0 |
| wind_speed | 4.65 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.12e+03 |
| n_updates | 11349 |
-----------------------------------------------
-----------------------------------------------************************************---------------------------| 72%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 78.44785 |
| air_temperature | 23.742256 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 15.0 |
| month | 9.0 |
| outdoor_humidity | 94.5 |
| outdoor_temperature | 21.647293 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 300.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.99e+03 |
| n_updates | 11374 |
-----------------------------------------------
-----------------------------------------------************************************---------------------------| 72%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 45.26872 |
| air_temperature | 24.988808 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 22.0 |
| month | 9.0 |
| outdoor_humidity | 55.0 |
| outdoor_temperature | 13.328288 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 50.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.08e+03 |
| n_updates | 11399 |
-----------------------------------------------
-----------------------------------------------*************************************--------------------------| 73%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 61.69315 |
| air_temperature | 25.202436 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 17.0 |
| month | 9.0 |
| outdoor_humidity | 75.0 |
| outdoor_temperature | 15.814474 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 65.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 8.44e+03 |
| n_updates | 11424 |
-----------------------------------------------
-----------------------------------------------*************************************--------------------------| 73%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 84.717415 |
| air_temperature | 23.804771 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 21.0 |
| month | 9.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 17.160044 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 45.0 |
| wind_speed | 2.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 7.67e+03 |
| n_updates | 11449 |
-----------------------------------------------
-----------------------------------------------*************************************--------------------------| 73%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 83.91682 |
| air_temperature | 23.10701 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 19.0 |
| month | 9.0 |
| outdoor_humidity | 97.0 |
| outdoor_temperature | 16.901966 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 50.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.58e+03 |
| n_updates | 11474 |
-----------------------------------------------
-----------------------------------------------**************************************-------------------------| 74%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 80.89759 |
| air_temperature | 23.867949 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 21.0 |
| month | 9.0 |
| outdoor_humidity | 71.5 |
| outdoor_temperature | 20.076616 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 260.0 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.88e+03 |
| n_updates | 11499 |
-----------------------------------------------
-----------------------------------------------**************************************-------------------------| 74%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 85.97487 |
| air_temperature | 23.029406 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 17.0 |
| month | 9.0 |
| outdoor_humidity | 52.5 |
| outdoor_temperature | 13.115639 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 45.0 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.96e+03 |
| n_updates | 11524 |
-----------------------------------------------
-----------------------------------------------**************************************-------------------------| 74%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 93.84955 |
| air_temperature | 21.605173 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 21.0 |
| month | 9.0 |
| outdoor_humidity | 86.0 |
| outdoor_temperature | 11.831333 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 40.0 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.51e+03 |
| n_updates | 11549 |
-----------------------------------------------
-----------------------------------------------***************************************------------------------| 75%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 321.20944 |
| air_humidity | 52.94715 |
| air_temperature | 21.00033 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 21.0 |
| month | 10.0 |
| outdoor_humidity | 83.0 |
| outdoor_temperature | 9.40463 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 289088.5 |
| wind_direction | 355.0 |
| wind_speed | 8.8 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.14e+03 |
| n_updates | 11574 |
-----------------------------------------------
-----------------------------------------------***************************************------------------------| 75%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 279.34213 |
| air_humidity | 47.79707 |
| air_temperature | 22.00002 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 10.137628 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 251407.92 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.66e+03 |
| n_updates | 11599 |
-----------------------------------------------
-----------------------------------------------***************************************------------------------| 75%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 86.035385 |
| air_humidity | 65.715164 |
| air_temperature | 22.000046 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 15.148232 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 77431.84 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.53e+03 |
| n_updates | 11624 |
-----------------------------------------------
-----------------------------------------------***************************************------------------------| 75%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 89.559746 |
| air_humidity | 62.382233 |
| air_temperature | 21.999939 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 58.0 |
| direct_solar_radiation | 91.0 |
| hour | 6.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 96.5 |
| outdoor_temperature | 15.210514 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 80603.77 |
| wind_direction | 230.0 |
| wind_speed | 4.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.43e+03 |
| n_updates | 11649 |
-----------------------------------------------
-----------------------------------------------****************************************-----------------------| 76%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 64.7902 |
| air_temperature | 21.465332 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 98.0 |
| direct_solar_radiation | 468.0 |
| hour | 7.0 |
| htg_setpoint | 20.0 |
| month | 10.0 |
| outdoor_humidity | 90.0 |
| outdoor_temperature | 17.777851 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 215.0 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.11e+03 |
| n_updates | 11674 |
-----------------------------------------------
-----------------------------------------------****************************************-----------------------| 76%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 69.57908 |
| air_temperature | 22.679138 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 68.0 |
| direct_solar_radiation | 0.0 |
| hour | 8.0 |
| htg_setpoint | 16.0 |
| month | 10.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | 19.479317 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 215.0 |
| wind_speed | 9.55 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.29e+03 |
| n_updates | 11699 |
-----------------------------------------------
-----------------------------------------------****************************************-----------------------| 76%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 660.2427 |
| air_humidity | 29.197695 |
| air_temperature | 23.115774 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 133.0 |
| direct_solar_radiation | 749.0 |
| hour | 9.0 |
| htg_setpoint | 21.0 |
| month | 10.0 |
| outdoor_humidity | 48.0 |
| outdoor_temperature | 11.404039 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 615147.3 |
| wind_direction | 300.0 |
| wind_speed | 7.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.38e+03 |
| n_updates | 11724 |
-----------------------------------------------
-----------------------------------------------*****************************************----------------------| 77%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 698.806 |
| air_humidity | 24.322908 |
| air_temperature | 23.732935 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 220.0 |
| direct_solar_radiation | 550.0 |
| hour | 10.0 |
| htg_setpoint | 21.0 |
| month | 10.0 |
| outdoor_humidity | 42.5 |
| outdoor_temperature | 10.196256 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 640776.94 |
| wind_direction | 330.0 |
| wind_speed | 8.25 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.12e+03 |
| n_updates | 11749 |
-----------------------------------------------
-----------------------------------------------*****************************************----------------------| 77%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 25.508892 |
| air_temperature | 24.289152 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 245.0 |
| direct_solar_radiation | 511.0 |
| hour | 11.0 |
| htg_setpoint | 21.0 |
| month | 10.0 |
| outdoor_humidity | 40.5 |
| outdoor_temperature | 11.32262 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 225.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.65e+03 |
| n_updates | 11774 |
-----------------------------------------------
-----------------------------------------------*****************************************----------------------| 77%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 38.35403 |
| air_temperature | 26.072796 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 202.0 |
| direct_solar_radiation | 688.0 |
| hour | 12.0 |
| htg_setpoint | 21.0 |
| month | 10.0 |
| outdoor_humidity | 54.0 |
| outdoor_temperature | 19.146742 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 230.0 |
| wind_speed | 8.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.1e+03 |
| n_updates | 11799 |
-----------------------------------------------
-----------------------------------------------*****************************************----------------------| 77%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 45.109554 |
| air_temperature | 29.182646 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 155.0 |
| direct_solar_radiation | 684.0 |
| hour | 13.0 |
| htg_setpoint | 21.0 |
| month | 10.0 |
| outdoor_humidity | 70.5 |
| outdoor_temperature | 20.280233 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 160.0 |
| wind_speed | 5.4 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.55e+03 |
| n_updates | 11824 |
-----------------------------------------------
-----------------------------------------------******************************************---------------------| 78%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 43.46771 |
| air_temperature | 26.831202 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 164.0 |
| direct_solar_radiation | 459.0 |
| hour | 14.0 |
| htg_setpoint | 15.0 |
| month | 10.0 |
| outdoor_humidity | 73.0 |
| outdoor_temperature | 19.983452 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 180.0 |
| wind_speed | 4.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.12e+03 |
| n_updates | 11849 |
-----------------------------------------------
-----------------------------------------------******************************************---------------------| 78%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 44.368893 |
| air_temperature | 27.992828 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 136.0 |
| direct_solar_radiation | 212.0 |
| hour | 15.0 |
| htg_setpoint | 20.0 |
| month | 10.0 |
| outdoor_humidity | 73.5 |
| outdoor_temperature | 19.311104 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 120.0 |
| wind_speed | 5.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.11e+03 |
| n_updates | 11874 |
-----------------------------------------------
-----------------------------------------------******************************************---------------------| 78%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 58.686783 |
| air_temperature | 27.221378 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 40.0 |
| direct_solar_radiation | 293.0 |
| hour | 16.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 88.5 |
| outdoor_temperature | 18.123085 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 110.0 |
| wind_speed | 6.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.51e+03 |
| n_updates | 11899 |
-----------------------------------------------
-----------------------------------------------******************************************---------------------| 78%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 774.1695 |
| air_humidity | 34.996456 |
| air_temperature | 27.246576 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 18.0 |
| month | 10.0 |
| outdoor_humidity | 47.5 |
| outdoor_temperature | 18.92559 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 703926.8 |
| wind_direction | 270.0 |
| wind_speed | 6.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 8.09e+03 |
| n_updates | 11924 |
-----------------------------------------------
------------------------------------------------******************************************--------------------| 79%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 112.674805 |
| air_humidity | 60.293446 |
| air_temperature | 25.96211 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 17.0 |
| month | 10.0 |
| outdoor_humidity | 98.0 |
| outdoor_temperature | 16.551872 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 101406.91 |
| wind_direction | 115.0 |
| wind_speed | 3.9 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.09e+03 |
| n_updates | 11949 |
------------------------------------------------
-----------------------------------------------*******************************************--------------------| 79%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 137.64085 |
| air_humidity | 28.135008 |
| air_temperature | 23.720987 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 15.0 |
| month | 10.0 |
| outdoor_humidity | 45.0 |
| outdoor_temperature | 11.987406 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 122232.64 |
| wind_direction | 265.0 |
| wind_speed | 10.8 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.5e+03 |
| n_updates | 11974 |
-----------------------------------------------
-----------------------------------------------*******************************************--------------------| 79%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 258.9177 |
| air_humidity | 30.75452 |
| air_temperature | 21.694313 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 16.0 |
| month | 10.0 |
| outdoor_humidity | 71.5 |
| outdoor_temperature | 9.033271 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 227640.38 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.1e+04 |
| n_updates | 11999 |
-----------------------------------------------
-----------------------------------------------********************************************-------------------| 80%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 44.34447 |
| air_temperature | 21.441462 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 21.0 |
| month | 10.0 |
| outdoor_humidity | 83.5 |
| outdoor_temperature | 11.766301 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 205.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.13e+03 |
| n_updates | 12024 |
-----------------------------------------------
-----------------------------------------------********************************************-------------------| 80%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 45.472637 |
| air_temperature | 21.698595 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 17.0 |
| month | 10.0 |
| outdoor_humidity | 80.5 |
| outdoor_temperature | 12.506051 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.2e+03 |
| n_updates | 12049 |
-----------------------------------------------
-----------------------------------------------********************************************-------------------| 80%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 96.831505 |
| air_humidity | 63.816128 |
| air_temperature | 21.0 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 21.0 |
| month | 10.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 14.555716 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 87148.36 |
| wind_direction | 215.0 |
| wind_speed | 5.15 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.05e+04 |
| n_updates | 12074 |
-----------------------------------------------
-----------------------------------------------*********************************************------------------| 81%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 143.056 |
| air_humidity | 49.87251 |
| air_temperature | 20.196104 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 16.0 |
| month | 10.0 |
| outdoor_humidity | 73.5 |
| outdoor_temperature | 13.250102 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 128750.41 |
| wind_direction | 20.0 |
| wind_speed | 1.05 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.35e+03 |
| n_updates | 12099 |
-----------------------------------------------
-----------------------------------------------*********************************************------------------| 81%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 69.9335 |
| air_temperature | 21.999865 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 16.482807 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 185.0 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.61e+03 |
| n_updates | 12124 |
-----------------------------------------------
-----------------------------------------------*********************************************------------------| 81%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 81.433624 |
| air_temperature | 20.767324 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 16.0 |
| month | 10.0 |
| outdoor_humidity | 98.0 |
| outdoor_temperature | 17.938904 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 185.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.91e+03 |
| n_updates | 12149 |
-----------------------------------------------
-----------------------------------------------*********************************************------------------| 81%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 19.583437 |
| air_temperature | 21.381088 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 19.0 |
| month | 10.0 |
| outdoor_humidity | 31.0 |
| outdoor_temperature | 12.771853 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 270.0 |
| wind_speed | 9.05 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.52e+03 |
| n_updates | 12174 |
-----------------------------------------------
-----------------------------------------------**********************************************-----------------| 82%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 414.9808 |
| air_humidity | 23.872995 |
| air_temperature | 18.743254 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 16.0 |
| month | 10.0 |
| outdoor_humidity | 60.5 |
| outdoor_temperature | 5.896669 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 373482.72 |
| wind_direction | 270.0 |
| wind_speed | 6.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.28e+04 |
| n_updates | 12199 |
-----------------------------------------------
-----------------------------------------------**********************************************-----------------| 82%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 821.85474 |
| air_humidity | 22.28704 |
| air_temperature | 20.656563 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 21.0 |
| month | 10.0 |
| outdoor_humidity | 63.0 |
| outdoor_temperature | 4.875373 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 739669.25 |
| wind_direction | 325.0 |
| wind_speed | 4.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 454 |
| n_updates | 12224 |
-----------------------------------------------
-----------------------------------------------**********************************************-----------------| 82%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 244.03078 |
| air_humidity | 27.547106 |
| air_temperature | 18.04457 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 2.0 |
| direct_solar_radiation | 63.0 |
| hour | 6.0 |
| htg_setpoint | 17.0 |
| month | 10.0 |
| outdoor_humidity | 75.0 |
| outdoor_temperature | 3.8614655 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 219627.69 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.19e+03 |
| n_updates | 12249 |
-----------------------------------------------
-----------------------------------------------***********************************************----------------| 83%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 244.03078 |
| air_humidity | 32.53162 |
| air_temperature | 19.037893 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 87.0 |
| direct_solar_radiation | 356.0 |
| hour | 7.0 |
| htg_setpoint | 17.0 |
| month | 10.0 |
| outdoor_humidity | 54.0 |
| outdoor_temperature | 11.73929 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 219627.69 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.29e+03 |
| n_updates | 12274 |
-----------------------------------------------
-----------------------------------------------***********************************************----------------| 83%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 21.744438 |
| air_temperature | 20.516462 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 161.0 |
| direct_solar_radiation | 270.0 |
| hour | 8.0 |
| htg_setpoint | 16.0 |
| month | 10.0 |
| outdoor_humidity | 49.5 |
| outdoor_temperature | 7.609495 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 60.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.4e+03 |
| n_updates | 12299 |
-----------------------------------------------
-----------------------------------------------***********************************************----------------| 83%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 918.473 |
| air_humidity | 30.203888 |
| air_temperature | 23.050997 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 131.0 |
| direct_solar_radiation | 667.0 |
| hour | 9.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 55.0 |
| outdoor_temperature | 10.156731 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 814822.44 |
| wind_direction | 345.0 |
| wind_speed | 8.25 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.78e+03 |
| n_updates | 12324 |
-----------------------------------------------
------------------------------------------------**********************************************----------------| 83%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 24.651491 |
| air_temperature | 20.29867 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 121.0 |
| direct_solar_radiation | 744.0 |
| hour | 10.0 |
| htg_setpoint | 15.0 |
| month | 11.0 |
| outdoor_humidity | 38.5 |
| outdoor_temperature | 13.3993225 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 0.0 |
| wind_speed | 8.25 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.56e+03 |
| n_updates | 12349 |
------------------------------------------------
-----------------------------------------------************************************************---------------| 84%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 28.324862 |
| air_temperature | 22.063183 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 276.0 |
| direct_solar_radiation | 198.0 |
| hour | 11.0 |
| htg_setpoint | 20.0 |
| month | 11.0 |
| outdoor_humidity | 44.5 |
| outdoor_temperature | 15.74168 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 355.0 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.86e+03 |
| n_updates | 12374 |
-----------------------------------------------
-----------------------------------------------************************************************---------------| 84%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 441.1365 |
| air_humidity | 37.676785 |
| air_temperature | 26.999302 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 184.0 |
| direct_solar_radiation | 597.0 |
| hour | 12.0 |
| htg_setpoint | 18.0 |
| month | 11.0 |
| outdoor_humidity | 55.0 |
| outdoor_temperature | 18.295261 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 396935.9 |
| wind_direction | 240.0 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 749 |
| n_updates | 12399 |
-----------------------------------------------
-----------------------------------------------************************************************---------------| 84%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 397.0408 |
| air_humidity | 25.999836 |
| air_temperature | 24.447517 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 85.0 |
| direct_solar_radiation | 0.0 |
| hour | 13.0 |
| htg_setpoint | 17.0 |
| month | 11.0 |
| outdoor_humidity | 44.0 |
| outdoor_temperature | 11.532406 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 357336.72 |
| wind_direction | 310.0 |
| wind_speed | 10.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.4e+04 |
| n_updates | 12424 |
-----------------------------------------------
-----------------------------------------------************************************************---------------| 84%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 493.84128 |
| air_humidity | 26.061975 |
| air_temperature | 25.247528 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 118.0 |
| direct_solar_radiation | 453.0 |
| hour | 14.0 |
| htg_setpoint | 17.0 |
| month | 11.0 |
| outdoor_humidity | 44.5 |
| outdoor_temperature | 12.655685 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 445882.44 |
| wind_direction | 330.0 |
| wind_speed | 4.9 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.79e+03 |
| n_updates | 12449 |
-----------------------------------------------
-----------------------------------------------*************************************************--------------| 85%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 337.6619 |
| air_humidity | 33.520164 |
| air_temperature | 25.640917 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 86.0 |
| direct_solar_radiation | 77.0 |
| hour | 15.0 |
| htg_setpoint | 16.0 |
| month | 11.0 |
| outdoor_humidity | 55.0 |
| outdoor_temperature | 13.122775 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 303895.72 |
| wind_direction | 320.0 |
| wind_speed | 5.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.89e+03 |
| n_updates | 12474 |
-----------------------------------------------
-----------------------------------------------*************************************************--------------| 85%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 871.74225 |
| air_humidity | 41.628914 |
| air_temperature | 24.081877 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 7.0 |
| direct_solar_radiation | 17.0 |
| hour | 16.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 80.0 |
| outdoor_temperature | 11.265384 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 785516.4 |
| wind_direction | 215.0 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.49e+03 |
| n_updates | 12499 |
-----------------------------------------------
-----------------------------------------------*************************************************--------------| 85%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 44.50846 |
| air_temperature | 21.999935 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 95.0 |
| outdoor_temperature | 11.621885 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 110.0 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 7.09e+03 |
| n_updates | 12524 |
-----------------------------------------------
-----------------------------------------------**************************************************-------------| 86%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 45.628036 |
| air_temperature | 22.000069 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 73.0 |
| outdoor_temperature | 12.389954 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 270.0 |
| wind_speed | 7.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 7.53e+03 |
| n_updates | 12549 |
-----------------------------------------------
-----------------------------------------------**************************************************-------------| 86%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 181.22653 |
| air_humidity | 43.488087 |
| air_temperature | 22.495659 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 18.0 |
| month | 11.0 |
| outdoor_humidity | 74.0 |
| outdoor_temperature | 11.260102 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 160043.17 |
| wind_direction | 355.0 |
| wind_speed | 7.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.77e+03 |
| n_updates | 12574 |
-----------------------------------------------
-----------------------------------------------**************************************************-------------| 86%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 488.6821 |
| air_humidity | 39.50117 |
| air_temperature | 20.336163 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 20.0 |
| month | 11.0 |
| outdoor_humidity | 77.5 |
| outdoor_temperature | 7.2215056 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 425114.0 |
| wind_direction | 5.0 |
| wind_speed | 4.65 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.17e+03 |
| n_updates | 12599 |
-----------------------------------------------
-----------------------------------------------**************************************************-------------| 86%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 180.7631 |
| air_humidity | 49.284153 |
| air_temperature | 21.999992 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 94.0 |
| outdoor_temperature | 11.821833 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 162686.8 |
| wind_direction | 150.0 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.16e+03 |
| n_updates | 12624 |
-----------------------------------------------
-----------------------------------------------***************************************************------------| 87%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 743.012 |
| air_humidity | 40.796642 |
| air_temperature | 19.002693 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 19.0 |
| month | 11.0 |
| outdoor_humidity | 65.5 |
| outdoor_temperature | 6.572727 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 668710.8 |
| wind_direction | 290.0 |
| wind_speed | 8.8 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.95e+03 |
| n_updates | 12649 |
-----------------------------------------------
-----------------------------------------------***************************************************------------| 87%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 628.6884 |
| air_humidity | 25.816118 |
| air_temperature | 21.093597 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 76.0 |
| outdoor_temperature | 4.599007 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 565819.56 |
| wind_direction | 260.0 |
| wind_speed | 6.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.35e+03 |
| n_updates | 12674 |
-----------------------------------------------
-----------------------------------------------***************************************************------------| 87%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 31.74596 |
| air_temperature | 21.000074 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 21.0 |
| month | 11.0 |
| outdoor_humidity | 89.5 |
| outdoor_temperature | 8.201467 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 220.0 |
| wind_speed | 5.9 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.17e+03 |
| n_updates | 12699 |
-----------------------------------------------
-----------------------------------------------****************************************************-----------| 88%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 756.4788 |
| air_humidity | 23.738033 |
| air_temperature | 20.86679 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 69.0 |
| outdoor_temperature | 4.1151543 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 680830.94 |
| wind_direction | 295.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.24e+03 |
| n_updates | 12724 |
-----------------------------------------------
-----------------------------------------------****************************************************-----------| 88%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 29.259447 |
| air_temperature | 17.8804 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 17.0 |
| month | 11.0 |
| outdoor_humidity | 63.0 |
| outdoor_temperature | 2.4538102 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 260.0 |
| wind_speed | 5.4 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.93e+03 |
| n_updates | 12749 |
-----------------------------------------------
-----------------------------------------------****************************************************-----------| 88%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 1109.8292 |
| air_humidity | 32.276184 |
| air_temperature | 20.002485 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 20.0 |
| month | 11.0 |
| outdoor_humidity | 80.0 |
| outdoor_temperature | 1.2077625 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 998846.3 |
| wind_direction | 270.0 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.17e+03 |
| n_updates | 12774 |
-----------------------------------------------
-------------------------------------------------***************************************************----------| 89%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1372.9894 |
| air_humidity | 33.068222 |
| air_temperature | 20.999987 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 21.0 |
| month | 11.0 |
| outdoor_humidity | 71.0 |
| outdoor_temperature | -0.10803817 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1235690.4 |
| wind_direction | 270.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.5e+03 |
| n_updates | 12799 |
-------------------------------------------------
------------------------------------------------****************************************************----------| 89%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1710.4203 |
| air_humidity | 26.788225 |
| air_temperature | 20.5662 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 21.0 |
| month | 11.0 |
| outdoor_humidity | 77.0 |
| outdoor_temperature | -1.7951931 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1539378.2 |
| wind_direction | 280.0 |
| wind_speed | 9.3 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.53e+03 |
| n_updates | 12824 |
------------------------------------------------
------------------------------------------------****************************************************----------| 89%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1841.4658 |
| air_humidity | 19.267988 |
| air_temperature | 20.999996 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 6.0 |
| htg_setpoint | 21.0 |
| month | 11.0 |
| outdoor_humidity | 63.5 |
| outdoor_temperature | -2.4504209 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1657319.2 |
| wind_direction | 295.0 |
| wind_speed | 6.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.28e+03 |
| n_updates | 12849 |
------------------------------------------------
------------------------------------------------****************************************************----------| 89%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 2306.6052 |
| air_humidity | 13.655644 |
| air_temperature | 22.000002 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 55.0 |
| direct_solar_radiation | 61.0 |
| hour | 7.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 66.0 |
| outdoor_temperature | -4.7761173 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2075944.6 |
| wind_direction | 25.0 |
| wind_speed | 0.75 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.16e+03 |
| n_updates | 12874 |
------------------------------------------------
-------------------------------------------------****************************************************---------| 90%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 1300.3312 |
| air_humidity | 21.154627 |
| air_temperature | 20.620234 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 53.0 |
| direct_solar_radiation | 0.0 |
| hour | 8.0 |
| htg_setpoint | 17.0 |
| month | 11.0 |
| outdoor_humidity | 76.5 |
| outdoor_temperature | -0.20197213 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1170298.1 |
| wind_direction | 60.0 |
| wind_speed | 4.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.56e+03 |
| n_updates | 12899 |
-------------------------------------------------
-----------------------------------------------******************************************************---------| 90%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 838.0061 |
| air_humidity | 55.374287 |
| air_temperature | 21.689898 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 154.0 |
| direct_solar_radiation | 56.0 |
| hour | 9.0 |
| htg_setpoint | 16.0 |
| month | 11.0 |
| outdoor_humidity | 98.5 |
| outdoor_temperature | 12.248131 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 767363.94 |
| wind_direction | 90.0 |
| wind_speed | 11.05 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.14e+03 |
| n_updates | 12924 |
-----------------------------------------------
-----------------------------------------------******************************************************---------| 90%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 41.32771 |
| air_temperature | 23.409586 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 178.0 |
| direct_solar_radiation | 547.0 |
| hour | 10.0 |
| htg_setpoint | 21.0 |
| month | 11.0 |
| outdoor_humidity | 81.5 |
| outdoor_temperature | 10.429253 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 250.0 |
| wind_speed | 6.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.12e+03 |
| n_updates | 12949 |
-----------------------------------------------
-----------------------------------------------******************************************************---------| 90%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 755.6465 |
| air_humidity | 31.469723 |
| air_temperature | 22.937748 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 158.0 |
| direct_solar_radiation | 0.0 |
| hour | 11.0 |
| htg_setpoint | 16.0 |
| month | 11.0 |
| outdoor_humidity | 57.0 |
| outdoor_temperature | 8.520331 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 680081.9 |
| wind_direction | 290.0 |
| wind_speed | 4.65 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.06e+03 |
| n_updates | 12974 |
-----------------------------------------------
-----------------------------------------------*******************************************************--------| 91%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 917.63416 |
| air_humidity | 25.424332 |
| air_temperature | 23.37107 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 120.0 |
| direct_solar_radiation | 644.0 |
| hour | 12.0 |
| htg_setpoint | 21.0 |
| month | 11.0 |
| outdoor_humidity | 57.0 |
| outdoor_temperature | 6.5279045 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 826909.6 |
| wind_direction | 225.0 |
| wind_speed | 4.65 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.54e+03 |
| n_updates | 12999 |
-----------------------------------------------
-----------------------------------------------*******************************************************--------| 91%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 370.86636 |
| air_humidity | 32.690987 |
| air_temperature | 18.534119 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 152.0 |
| direct_solar_radiation | 29.0 |
| hour | 13.0 |
| htg_setpoint | 17.0 |
| month | 11.0 |
| outdoor_humidity | 62.0 |
| outdoor_temperature | 6.47209 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 333779.75 |
| wind_direction | 310.0 |
| wind_speed | 9.25 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.29e+03 |
| n_updates | 13024 |
-----------------------------------------------
-----------------------------------------------*******************************************************--------| 91%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 21.081112 |
| air_temperature | 20.211607 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 64.0 |
| direct_solar_radiation | 234.0 |
| hour | 14.0 |
| htg_setpoint | 20.0 |
| month | 12.0 |
| outdoor_humidity | 39.5 |
| outdoor_temperature | 3.3783436 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 310.0 |
| wind_speed | 12.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.08e+03 |
| n_updates | 13049 |
-----------------------------------------------
-----------------------------------------------********************************************************-------| 92%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 861.6628 |
| air_humidity | 18.8145 |
| air_temperature | 23.979624 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 28.0 |
| direct_solar_radiation | 526.0 |
| hour | 15.0 |
| htg_setpoint | 16.0 |
| month | 12.0 |
| outdoor_humidity | 34.5 |
| outdoor_temperature | 5.8914924 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 775496.5 |
| wind_direction | 300.0 |
| wind_speed | 9.55 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 793 |
| n_updates | 13074 |
-----------------------------------------------
-----------------------------------------------********************************************************-------| 92%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 640.4554 |
| air_humidity | 25.776783 |
| air_temperature | 24.407007 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 16.0 |
| htg_setpoint | 15.0 |
| month | 12.0 |
| outdoor_humidity | 49.5 |
| outdoor_temperature | 8.198558 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 570274.1 |
| wind_direction | 220.0 |
| wind_speed | 4.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 7.59e+03 |
| n_updates | 13099 |
-----------------------------------------------
-----------------------------------------------********************************************************-------| 92%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 844.3286 |
| air_humidity | 41.763554 |
| air_temperature | 23.095621 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 21.0 |
| month | 12.0 |
| outdoor_humidity | 90.0 |
| outdoor_temperature | 7.7191653 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 759895.75 |
| wind_direction | 235.0 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.3e+03 |
| n_updates | 13124 |
-----------------------------------------------
-----------------------------------------------********************************************************-------| 92%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 874.85583 |
| air_humidity | 33.53786 |
| air_temperature | 21.60302 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 21.0 |
| month | 12.0 |
| outdoor_humidity | 71.0 |
| outdoor_temperature | 4.926668 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 774289.1 |
| wind_direction | 275.0 |
| wind_speed | 7.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.88e+03 |
| n_updates | 13149 |
-----------------------------------------------
------------------------------------------------********************************************************------| 93%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1264.9574 |
| air_humidity | 21.493917 |
| air_temperature | 22.395668 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 22.0 |
| month | 12.0 |
| outdoor_humidity | 53.0 |
| outdoor_temperature | 0.43212128 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 1138461.8 |
| wind_direction | 255.0 |
| wind_speed | 9.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.35e+03 |
| n_updates | 13174 |
------------------------------------------------
-----------------------------------------------*********************************************************------| 93%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 306.69937 |
| air_humidity | 19.631279 |
| air_temperature | 19.0 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 19.0 |
| month | 12.0 |
| outdoor_humidity | 53.0 |
| outdoor_temperature | 4.6707997 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 276029.44 |
| wind_direction | 285.0 |
| wind_speed | 8.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.58e+03 |
| n_updates | 13199 |
-----------------------------------------------
-----------------------------------------------*********************************************************------| 93%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 17.799517 |
| air_temperature | 22.0 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 22.0 |
| month | 12.0 |
| outdoor_humidity | 60.5 |
| outdoor_temperature | 2.2084277 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 330.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 542 |
| n_updates | 13224 |
-----------------------------------------------
-----------------------------------------------*********************************************************------| 93%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 32.181026 |
| air_temperature | 21.002357 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 21.0 |
| month | 12.0 |
| outdoor_humidity | 66.0 |
| outdoor_temperature | 3.265807 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.62e+03 |
| n_updates | 13249 |
-----------------------------------------------
-----------------------------------------------**********************************************************-----| 94%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 37.930477 |
| air_temperature | 19.753136 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 20.0 |
| month | 12.0 |
| outdoor_humidity | 94.0 |
| outdoor_temperature | 4.2085614 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 30.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 899 |
| n_updates | 13274 |
-----------------------------------------------
-------------------------------------------------********************************************************-----| 94%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1398.5908 |
| air_humidity | 31.410603 |
| air_temperature | 21.000002 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 21.0 |
| month | 12.0 |
| outdoor_humidity | 82.0 |
| outdoor_temperature | -0.23604545 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1258731.8 |
| wind_direction | 20.0 |
| wind_speed | 2.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.56e+03 |
| n_updates | 13299 |
-------------------------------------------------
-----------------------------------------------***********************************************************----| 95%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 30.992683 |
| air_temperature | 21.999996 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 22.0 |
| month | 12.0 |
| outdoor_humidity | 69.5 |
| outdoor_temperature | 2.9798114 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 275.0 |
| wind_speed | 5.4 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.53e+03 |
| n_updates | 13324 |
-----------------------------------------------
-----------------------------------------------***********************************************************----| 95%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 27.637766 |
| air_temperature | 18.669603 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 15.0 |
| month | 12.0 |
| outdoor_humidity | 48.0 |
| outdoor_temperature | 3.13234 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 280.0 |
| wind_speed | 5.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.43e+03 |
| n_updates | 13349 |
-----------------------------------------------
------------------------------------------------**********************************************************----| 95%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1793.6534 |
| air_humidity | 12.261504 |
| air_temperature | 21.999996 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 22.0 |
| month | 12.0 |
| outdoor_humidity | 48.5 |
| outdoor_temperature | -2.2113588 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1614288.1 |
| wind_direction | 325.0 |
| wind_speed | 6.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.96e+03 |
| n_updates | 13374 |
------------------------------------------------
-----------------------------------------------***********************************************************----| 95%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 1068.9926 |
| air_humidity | 20.901031 |
| air_temperature | 17.97254 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 15.0 |
| month | 12.0 |
| outdoor_humidity | 81.5 |
| outdoor_temperature | 1.4119455 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 962093.4 |
| wind_direction | 245.0 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.54e+03 |
| n_updates | 13399 |
-----------------------------------------------
-----------------------------------------------************************************************************---| 96%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 1037.5333 |
| air_humidity | 23.295412 |
| air_temperature | 19.985338 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 16.0 |
| month | 12.0 |
| outdoor_humidity | 75.5 |
| outdoor_temperature | 1.569242 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 933780.0 |
| wind_direction | 235.0 |
| wind_speed | 2.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.62e+03 |
| n_updates | 13424 |
-----------------------------------------------
------------------------------------------------***********************************************************---| 96%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 531.9781 |
| air_humidity | 21.137249 |
| air_temperature | 21.781822 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 6.0 |
| htg_setpoint | 22.0 |
| month | 12.0 |
| outdoor_humidity | 69.0 |
| outdoor_temperature | 0.56026334 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 478780.3 |
| wind_direction | 340.0 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.52e+03 |
| n_updates | 13449 |
------------------------------------------------
-----------------------------------------------************************************************************---| 96%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 244.03078 |
| air_humidity | 26.447454 |
| air_temperature | 20.000038 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 13.0 |
| direct_solar_radiation | 79.0 |
| hour | 7.0 |
| htg_setpoint | 20.0 |
| month | 12.0 |
| outdoor_humidity | 70.5 |
| outdoor_temperature | 4.3963323 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 219627.69 |
| wind_direction | 240.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.15e+03 |
| n_updates | 13474 |
-----------------------------------------------
-----------------------------------------------************************************************************---| 96%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 28.223364 |
| air_temperature | 21.120174 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 31.0 |
| direct_solar_radiation | 578.0 |
| hour | 8.0 |
| htg_setpoint | 16.0 |
| month | 12.0 |
| outdoor_humidity | 79.0 |
| outdoor_temperature | 4.0409384 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 230.0 |
| wind_speed | 1.8 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.93e+03 |
| n_updates | 13499 |
-----------------------------------------------
------------------------------------------------************************************************************--| 97%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1293.855 |
| air_humidity | 21.47397 |
| air_temperature | 21.0 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 92.0 |
| direct_solar_radiation | 547.0 |
| hour | 9.0 |
| htg_setpoint | 21.0 |
| month | 12.0 |
| outdoor_humidity | 49.5 |
| outdoor_temperature | 0.28763378 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1164469.5 |
| wind_direction | 355.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.72e+03 |
| n_updates | 13524 |
------------------------------------------------
------------------------------------------------************************************************************--| 97%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 2052.83 |
| air_humidity | 17.319937 |
| air_temperature | 18.667053 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 134.0 |
| direct_solar_radiation | 489.0 |
| hour | 10.0 |
| htg_setpoint | 15.0 |
| month | 12.0 |
| outdoor_humidity | 46.5 |
| outdoor_temperature | -3.5072422 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1847547.1 |
| wind_direction | 40.0 |
| wind_speed | 4.65 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.29e+04 |
| n_updates | 13549 |
------------------------------------------------
-----------------------------------------------*************************************************************--| 97%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 755.6465 |
| air_humidity | 29.388943 |
| air_temperature | 22.514286 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 120.0 |
| direct_solar_radiation | 0.0 |
| hour | 11.0 |
| htg_setpoint | 18.0 |
| month | 12.0 |
| outdoor_humidity | 84.0 |
| outdoor_temperature | 2.2709444 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 680081.9 |
| wind_direction | 345.0 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.61e+03 |
| n_updates | 13574 |
-----------------------------------------------
-----------------------------------------------**************************************************************-| 98%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 476.10425 |
| air_humidity | 23.907196 |
| air_temperature | 22.934315 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 303.0 |
| direct_solar_radiation | 45.0 |
| hour | 12.0 |
| htg_setpoint | 15.0 |
| month | 12.0 |
| outdoor_humidity | 56.5 |
| outdoor_temperature | 4.08747 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 428493.84 |
| wind_direction | 345.0 |
| wind_speed | 1.3 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.47e+03 |
| n_updates | 13599 |
-----------------------------------------------
-----------------------------------------------**************************************************************-| 98%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 782.1886 |
| air_humidity | 44.01096 |
| air_temperature | 22.976707 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 113.0 |
| direct_solar_radiation | 309.0 |
| hour | 13.0 |
| htg_setpoint | 22.0 |
| month | 12.0 |
| outdoor_humidity | 89.5 |
| outdoor_temperature | 9.240701 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 713822.94 |
| wind_direction | 270.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.53e+03 |
| n_updates | 13624 |
-----------------------------------------------
-----------------------------------------------**************************************************************-| 98%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 26.889645 |
| air_temperature | 23.825846 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 108.0 |
| direct_solar_radiation | 88.0 |
| hour | 14.0 |
| htg_setpoint | 22.0 |
| month | 12.0 |
| outdoor_humidity | 48.5 |
| outdoor_temperature | 9.648953 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 290.0 |
| wind_speed | 7.5 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.96e+03 |
| n_updates | 13649 |
-----------------------------------------------
-----------------------------------------------**************************************************************-| 98%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 33.47256 |
| air_temperature | 23.051739 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 28.0 |
| direct_solar_radiation | 0.0 |
| hour | 15.0 |
| htg_setpoint | 16.0 |
| month | 12.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 2.5098643 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 5.0 |
| wind_speed | 5.4 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.37e+03 |
| n_updates | 13674 |
-----------------------------------------------
-----------------------------------------------***************************************************************| 99%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 24.71378 |
| air_temperature | 22.259344 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 16.0 |
| htg_setpoint | 17.0 |
| month | 12.0 |
| outdoor_humidity | 40.5 |
| outdoor_temperature | 3.2563782 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 315.0 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.63e+03 |
| n_updates | 13699 |
-----------------------------------------------
-----------------------------------------------***************************************************************| 99%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 91.78562 |
| air_humidity | 25.185575 |
| air_temperature | 20.999916 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 21.0 |
| month | 12.0 |
| outdoor_humidity | 97.0 |
| outdoor_temperature | 4.861682 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 82607.06 |
| wind_direction | 55.0 |
| wind_speed | 10.05 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 7.92e+03 |
| n_updates | 13724 |
-----------------------------------------------
-----------------------------------------------***************************************************************| 99%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1024.272 |
| air_humidity | 27.808973 |
| air_temperature | 22.563572 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 16.0 |
| month | 12.0 |
| outdoor_humidity | 71.0 |
| outdoor_temperature | 1.6355486 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 921844.75 |
| wind_direction | 245.0 |
| wind_speed | 10.55 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.52e+03 |
| n_updates | 13749 |
-----------------------------------------------
-----------------------------------------------***************************************************************| 99%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 2743.635 |
| air_humidity | 16.346043 |
| air_temperature | 21.583727 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 20.0 |
| month | 12.0 |
| outdoor_humidity | 39.0 |
| outdoor_temperature | -6.961267 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 2469271.5 |
| wind_direction | 315.0 |
| wind_speed | 7.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 968 |
| n_updates | 13774 |
-----------------------------------------------
[WRAPPER LoggerWrapper] (INFO) : End of episode detected, recording summary (progress.csv) if logger is active
#----------------------------------------------------------------------------------------------#
[ENVIRONMENT] (INFO) : Starting a new episode... [SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43] [Episode 4]
#----------------------------------------------------------------------------------------------#
[MODELING] (INFO) : Episode directory created [/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43-res1/Eplus-env-sub_run4]
[MODELING] (INFO) : Weather file USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3.epw used.
[MODELING] (INFO) : Updated building model with whole Output:Variable available names
[MODELING] (INFO) : Updated building model with whole Output:Meter available names
[MODELING] (INFO) : Adapting weather to building model. [USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3.epw]
[ENVIRONMENT] (INFO) : Saving episode output path... [/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43-res1/Eplus-env-sub_run4/output]
[SIMULATOR] (INFO) : Running EnergyPlus with args: ['-w', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43-res1/Eplus-env-sub_run4/USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3_Random_1.0_0.0_0.001.epw', '-d', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43-res1/Eplus-env-sub_run4/output', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43-res1/Eplus-env-sub_run4/5ZoneAutoDXVAV.epJSON']
[ENVIRONMENT] (INFO) : Episode 4 started.
/usr/local/lib/python3.10/dist-packages/opyplus/weather_data/weather_data.py:493: FutureWarning: the 'line_terminator'' keyword is deprecated, use 'lineterminator' instead.
epw_content = self._headers_to_epw(use_datetimes=use_datetimes) + df.to_csv(
[SIMULATOR] (INFO) : handlers are ready.
[SIMULATOR] (INFO) : System is ready.
[WRAPPER LoggerWrapper] (INFO) : Creating monitor.csv for current episode (episode 4) if logger is active
----------------------------------------------------
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| episode/ | |
| comfort_violation_time(%) | 42.6 |
| cumulative_comfort_penalty | -2.13e+04 |
| cumulative_energy_penalty | -3.54e+03 |
| cumulative_power | 7.08e+07 |
| cumulative_reward | -24834.174 |
| cumulative_temperature_violation | 4.26e+04 |
| episode_length | 70077 |
| mean_comfort_penalty | -0.304 |
| mean_energy_penalty | -0.0505 |
| mean_power | 1.01e+03 |
| mean_reward | -0.3543841 |
| mean_temperature_violation | 0.608 |
| observation/ | |
| HVAC_electricity_demand_rate | 3264.773 |
| air_humidity | 16.017635 |
| air_temperature | 16.728548 |
| clg_setpoint | 40.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 12.8 |
| month | 1.0 |
| outdoor_humidity | 43.25 |
| outdoor_temperature | -9.5669565 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2938295.8 |
| wind_direction | 305.0 |
| wind_speed | 8.4 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.15e+03 |
| n_updates | 13779 |
----------------------------------------------------
-----------------------------------
| rollout/ | |
| ep_len_mean | 3.5e+04 |
| ep_rew_mean | -1.22e+04 |
| exploration_rate | 0.05 |
| time/ | |
| episodes | 2 |
| fps | 952 |
| time_elapsed | 110 |
| total_timesteps | 105117 |
-----------------------------------
/usr/local/lib/python3.10/dist-packages/gymnasium/core.py:311: UserWarning: WARN: env.observation_variables to get variables from other wrappers is deprecated and will be removed in v1.0, to get this variable you can do `env.unwrapped.observation_variables` for environment variables or `env.get_wrapper_attr('observation_variables')` that will search the reminding wrappers.
logger.warn(
/usr/local/lib/python3.10/dist-packages/gymnasium/core.py:311: UserWarning: WARN: env.action_variables to get variables from other wrappers is deprecated and will be removed in v1.0, to get this variable you can do `env.unwrapped.action_variables` for environment variables or `env.get_wrapper_attr('action_variables')` that will search the reminding wrappers.
logger.warn(
/usr/local/lib/python3.10/dist-packages/gymnasium/core.py:311: UserWarning: WARN: env.is_discrete to get variables from other wrappers is deprecated and will be removed in v1.0, to get this variable you can do `env.unwrapped.is_discrete` for environment variables or `env.get_wrapper_attr('is_discrete')` that will search the reminding wrappers.
logger.warn(
/usr/local/lib/python3.10/dist-packages/gymnasium/spaces/box.py:240: UserWarning: WARN: Casting input x to numpy array.
gym.logger.warn("Casting input x to numpy array.")
-----------------------------------------------
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 3178.6443 |
| air_humidity | 13.9179 |
| air_temperature | 21.00378 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 21.0 |
| month | 1.0 |
| outdoor_humidity | 25.5 |
| outdoor_temperature | -9.136313 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 2860780.0 |
| wind_direction | 320.0 |
| wind_speed | 10.925 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.82e+03 |
| n_updates | 13799 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 1%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 2588.9912 |
| air_humidity | 15.689926 |
| air_temperature | 20.000109 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 20.0 |
| month | 1.0 |
| outdoor_humidity | 29.0 |
| outdoor_temperature | -6.1880474 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2330092.0 |
| wind_direction | 60.0 |
| wind_speed | 4.875 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.14e+03 |
| n_updates | 13824 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 1%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 1323.0002 |
| air_humidity | 38.257965 |
| air_temperature | 19.999996 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 20.0 |
| month | 1.0 |
| outdoor_humidity | 87.25 |
| outdoor_temperature | 0.14190736 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1190700.2 |
| wind_direction | 247.5 |
| wind_speed | 8.275 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.11e+03 |
| n_updates | 13849 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 1%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 2258.7883 |
| air_humidity | 20.096224 |
| air_temperature | 17.745817 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 16.0 |
| month | 1.0 |
| outdoor_humidity | 42.5 |
| outdoor_temperature | -4.5370326 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2032909.4 |
| wind_direction | 280.0 |
| wind_speed | 8.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.91e+03 |
| n_updates | 13874 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 2%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 2483.813 |
| air_humidity | 14.061123 |
| air_temperature | 17.850971 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 15.0 |
| month | 1.0 |
| outdoor_humidity | 58.0 |
| outdoor_temperature | -5.6621566 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2235431.8 |
| wind_direction | 292.5 |
| wind_speed | 2.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 795 |
| n_updates | 13899 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 2%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1322.736 |
| air_humidity | 10.838393 |
| air_temperature | 22.000002 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 22.0 |
| month | 1.0 |
| outdoor_humidity | 56.25 |
| outdoor_temperature | 0.14322889 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1190462.4 |
| wind_direction | 240.0 |
| wind_speed | 5.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.74e+03 |
| n_updates | 13924 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 2%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 2446.134 |
| air_humidity | 16.319656 |
| air_temperature | 20.34714 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 21.0 |
| month | 1.0 |
| outdoor_humidity | 41.0 |
| outdoor_temperature | -5.473761 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2201520.5 |
| wind_direction | 335.0 |
| wind_speed | 5.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.43e+03 |
| n_updates | 13949 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 2%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 31.85662 |
| air_temperature | 21.999998 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 22.0 |
| month | 1.0 |
| outdoor_humidity | 97.0 |
| outdoor_temperature | 3.2131824 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 272.5 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.97e+03 |
| n_updates | 13974 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 3%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1941.4054 |
| air_humidity | 26.045143 |
| air_temperature | 21.82076 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 22.0 |
| month | 1.0 |
| outdoor_humidity | 67.25 |
| outdoor_temperature | -2.9501185 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1747264.9 |
| wind_direction | 337.5 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.89e+03 |
| n_updates | 13999 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 3%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 1955.8734 |
| air_humidity | 18.822397 |
| air_temperature | 19.999998 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 20.0 |
| month | 1.0 |
| outdoor_humidity | 48.75 |
| outdoor_temperature | -3.0224586 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1760286.1 |
| wind_direction | 270.0 |
| wind_speed | 7.075 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 902 |
| n_updates | 14024 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 3%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 17.606636 |
| air_temperature | 20.999992 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 6.0 |
| htg_setpoint | 21.0 |
| month | 1.0 |
| outdoor_humidity | 68.5 |
| outdoor_temperature | 2.3032584 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 245.0 |
| wind_speed | 3.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.06e+04 |
| n_updates | 14049 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 4%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 124.68914 |
| air_humidity | 29.865686 |
| air_temperature | 20.02376 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 19.5 |
| direct_solar_radiation | 0.0 |
| hour | 7.0 |
| htg_setpoint | 19.0 |
| month | 1.0 |
| outdoor_humidity | 83.75 |
| outdoor_temperature | 4.82571 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 107442.516 |
| wind_direction | 210.0 |
| wind_speed | 3.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 926 |
| n_updates | 14074 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 4%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 2462.6763 |
| air_humidity | 17.745747 |
| air_temperature | 19.46204 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 87.0 |
| direct_solar_radiation | 30.75 |
| hour | 8.0 |
| htg_setpoint | 17.0 |
| month | 1.0 |
| outdoor_humidity | 82.25 |
| outdoor_temperature | -6.0136967 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 2216408.5 |
| wind_direction | 32.5 |
| wind_speed | 7.725 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.83e+03 |
| n_updates | 14099 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 4%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 33.261345 |
| air_temperature | 22.545242 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 52.0 |
| direct_solar_radiation | 0.0 |
| hour | 9.0 |
| htg_setpoint | 22.0 |
| month | 1.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 7.8804245 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 132.5 |
| wind_speed | 7.425 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.45e+03 |
| n_updates | 14124 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 4%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1471.364 |
| air_humidity | 19.59662 |
| air_temperature | 22.959154 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 177.0 |
| direct_solar_radiation | 153.0 |
| hour | 10.0 |
| htg_setpoint | 21.0 |
| month | 1.0 |
| outdoor_humidity | 61.25 |
| outdoor_temperature | -0.99800754 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1324227.6 |
| wind_direction | 215.0 |
| wind_speed | 5.325 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.6e+03 |
| n_updates | 14149 |
-------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 5%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 745.9737 |
| air_humidity | 26.770702 |
| air_temperature | 23.468678 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 93.25 |
| direct_solar_radiation | 769.0 |
| hour | 11.0 |
| htg_setpoint | 15.0 |
| month | 1.0 |
| outdoor_humidity | 51.75 |
| outdoor_temperature | 7.255399 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 677180.0 |
| wind_direction | 255.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.13e+03 |
| n_updates | 14174 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 5%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 524.576 |
| air_humidity | 49.16026 |
| air_temperature | 22.537245 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 115.5 |
| direct_solar_radiation | 0.0 |
| hour | 12.0 |
| htg_setpoint | 21.0 |
| month | 1.0 |
| outdoor_humidity | 93.75 |
| outdoor_temperature | 10.697998 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 472118.4 |
| wind_direction | 140.0 |
| wind_speed | 10.05 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.49e+03 |
| n_updates | 14199 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 5%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 142.57867 |
| air_humidity | 22.805319 |
| air_temperature | 21.999964 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 99.75 |
| direct_solar_radiation | 666.25 |
| hour | 13.0 |
| htg_setpoint | 22.0 |
| month | 1.0 |
| outdoor_humidity | 37.25 |
| outdoor_temperature | 8.377738 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 128933.805 |
| wind_direction | 265.0 |
| wind_speed | 8.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.7e+03 |
| n_updates | 14224 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 5%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 17.845053 |
| air_temperature | 21.09502 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 127.5 |
| direct_solar_radiation | 477.5 |
| hour | 14.0 |
| htg_setpoint | 16.0 |
| month | 1.0 |
| outdoor_humidity | 53.5 |
| outdoor_temperature | 8.656128 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 227.5 |
| wind_speed | 4.875 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.25e+03 |
| n_updates | 14249 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 6%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 755.1569 |
| air_humidity | 40.648323 |
| air_temperature | 23.346079 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 39.5 |
| direct_solar_radiation | 0.0 |
| hour | 15.0 |
| htg_setpoint | 22.0 |
| month | 1.0 |
| outdoor_humidity | 85.25 |
| outdoor_temperature | 8.96482 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 679641.2 |
| wind_direction | 82.5 |
| wind_speed | 5.325 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.05e+04 |
| n_updates | 14274 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 6%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 42.070915 |
| air_temperature | 22.793812 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 16.5 |
| direct_solar_radiation | 20.25 |
| hour | 16.0 |
| htg_setpoint | 22.0 |
| month | 1.0 |
| outdoor_humidity | 89.0 |
| outdoor_temperature | 7.1089516 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 82.5 |
| wind_speed | 7.75 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.61e+03 |
| n_updates | 14299 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 6%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 637.3314 |
| air_humidity | 53.170254 |
| air_temperature | 23.19397 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 15.0 |
| month | 1.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 10.569685 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 573598.3 |
| wind_direction | 115.0 |
| wind_speed | 3.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.36e+03 |
| n_updates | 14324 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 7%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 371.55234 |
| air_humidity | 53.279606 |
| air_temperature | 22.224451 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 21.0 |
| month | 1.0 |
| outdoor_humidity | 87.25 |
| outdoor_temperature | 11.406227 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 334397.1 |
| wind_direction | 287.5 |
| wind_speed | 7.875 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.78e+03 |
| n_updates | 14349 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 7%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1022.66864 |
| air_humidity | 34.204594 |
| air_temperature | 22.414547 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 22.0 |
| month | 1.0 |
| outdoor_humidity | 89.0 |
| outdoor_temperature | 1.6435652 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 920401.8 |
| wind_direction | 270.0 |
| wind_speed | 5.325 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.54e+03 |
| n_updates | 14374 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 7%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 1139.4733 |
| air_humidity | 25.386675 |
| air_temperature | 20.21549 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 16.0 |
| month | 1.0 |
| outdoor_humidity | 81.75 |
| outdoor_temperature | 1.059542 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1025525.94 |
| wind_direction | 237.5 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.52e+03 |
| n_updates | 14399 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 7%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 24.400509 |
| air_temperature | 19.244875 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 16.0 |
| month | 1.0 |
| outdoor_humidity | 73.0 |
| outdoor_temperature | 3.6225793 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 35.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.52e+03 |
| n_updates | 14424 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 8%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 957.9209 |
| air_humidity | 39.998306 |
| air_temperature | 20.000904 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 20.0 |
| month | 1.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 1.9673041 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 862128.8 |
| wind_direction | 60.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.65e+03 |
| n_updates | 14449 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 8%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1848.209 |
| air_humidity | 23.906105 |
| air_temperature | 22.000002 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 22.0 |
| month | 1.0 |
| outdoor_humidity | 69.5 |
| outdoor_temperature | -2.4841363 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1663388.0 |
| wind_direction | 37.5 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 720 |
| n_updates | 14474 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 8%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 2414.4824 |
| air_humidity | 26.082052 |
| air_temperature | 20.003956 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 20.0 |
| month | 1.0 |
| outdoor_humidity | 40.75 |
| outdoor_temperature | -5.3155036 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2173034.2 |
| wind_direction | 12.5 |
| wind_speed | 11.75 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.69e+03 |
| n_updates | 14499 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 9%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 17.025675 |
| air_temperature | 21.0 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 21.0 |
| month | 2.0 |
| outdoor_humidity | 63.5 |
| outdoor_temperature | 0.79964656 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 267.5 |
| wind_speed | 4.65 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.48e+03 |
| n_updates | 14524 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 9%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 699.84283 |
| air_humidity | 31.964478 |
| air_temperature | 18.297335 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 15.0 |
| month | 2.0 |
| outdoor_humidity | 75.75 |
| outdoor_temperature | 4.0827537 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 602817.7 |
| wind_direction | 270.0 |
| wind_speed | 3.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.99e+03 |
| n_updates | 14549 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 9%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 3115.8237 |
| air_humidity | 15.136002 |
| air_temperature | 19.429403 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 16.0 |
| month | 2.0 |
| outdoor_humidity | 52.25 |
| outdoor_temperature | -8.82221 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2804241.5 |
| wind_direction | 5.0 |
| wind_speed | 5.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.07e+03 |
| n_updates | 14574 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 10%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 1468.3408 |
| air_humidity | 13.363356 |
| air_temperature | 20.0 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 20.0 |
| month | 2.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | -0.58479565 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1321506.8 |
| wind_direction | 90.0 |
| wind_speed | 4.675 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.97e+03 |
| n_updates | 14599 |
-------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 10%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1956.0592 |
| air_humidity | 21.415497 |
| air_temperature | 21.735435 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 22.0 |
| month | 2.0 |
| outdoor_humidity | 65.5 |
| outdoor_temperature | -3.0233877 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1760453.2 |
| wind_direction | 285.0 |
| wind_speed | 9.425 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.69e+03 |
| n_updates | 14624 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 10%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 3478.2593 |
| air_humidity | 11.524329 |
| air_temperature | 16.549387 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 6.0 |
| htg_setpoint | 16.0 |
| month | 2.0 |
| outdoor_humidity | 54.0 |
| outdoor_temperature | -14.171143 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 3130433.2 |
| wind_direction | 272.5 |
| wind_speed | 7.575 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.2e+03 |
| n_updates | 14649 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 10%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 2161.4868 |
| air_humidity | 11.50414 |
| air_temperature | 19.000036 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 39.75 |
| direct_solar_radiation | 153.25 |
| hour | 7.0 |
| htg_setpoint | 19.0 |
| month | 2.0 |
| outdoor_humidity | 64.75 |
| outdoor_temperature | -7.5872803 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1945338.1 |
| wind_direction | 282.5 |
| wind_speed | 5.575 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.1e+03 |
| n_updates | 14674 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 11%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 2298.961 |
| air_humidity | 12.468061 |
| air_temperature | 21.999899 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 68.75 |
| direct_solar_radiation | 501.0 |
| hour | 8.0 |
| htg_setpoint | 22.0 |
| month | 2.0 |
| outdoor_humidity | 41.5 |
| outdoor_temperature | -5.1359925 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 2069064.9 |
| wind_direction | 342.5 |
| wind_speed | 4.875 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2e+03 |
| n_updates | 14699 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 11%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 2482.3235 |
| air_humidity | 15.208434 |
| air_temperature | 19.0 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 58.5 |
| direct_solar_radiation | 837.25 |
| hour | 9.0 |
| htg_setpoint | 19.0 |
| month | 2.0 |
| outdoor_humidity | 50.75 |
| outdoor_temperature | -5.6547093 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2234091.2 |
| wind_direction | 302.5 |
| wind_speed | 7.575 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.71e+03 |
| n_updates | 14724 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 11%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1333.4619 |
| air_humidity | 10.839565 |
| air_temperature | 21.995874 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 218.25 |
| direct_solar_radiation | 219.75 |
| hour | 10.0 |
| htg_setpoint | 22.0 |
| month | 2.0 |
| outdoor_humidity | 54.0 |
| outdoor_temperature | 0.08959897 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1200115.8 |
| wind_direction | 252.5 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.4e+03 |
| n_updates | 14749 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 11%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 24.333763 |
| air_temperature | 23.36302 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 129.5 |
| direct_solar_radiation | 746.5 |
| hour | 11.0 |
| htg_setpoint | 21.0 |
| month | 2.0 |
| outdoor_humidity | 47.5 |
| outdoor_temperature | 5.126002 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 267.5 |
| wind_speed | 6.675 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.27e+03 |
| n_updates | 14774 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 12%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 2383.3894 |
| air_humidity | 8.774642 |
| air_temperature | 22.380083 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 158.75 |
| direct_solar_radiation | 683.75 |
| hour | 12.0 |
| htg_setpoint | 17.0 |
| month | 2.0 |
| outdoor_humidity | 27.5 |
| outdoor_temperature | -7.536425 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 2145050.5 |
| wind_direction | 292.5 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 8.95e+03 |
| n_updates | 14799 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 12%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 2361.432 |
| air_humidity | 11.286173 |
| air_temperature | 22.862402 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 171.25 |
| direct_solar_radiation | 540.0 |
| hour | 13.0 |
| htg_setpoint | 21.0 |
| month | 2.0 |
| outdoor_humidity | 37.0 |
| outdoor_temperature | -5.5457816 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 2125288.8 |
| wind_direction | 267.5 |
| wind_speed | 5.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.04e+03 |
| n_updates | 14824 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 12%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 1143.717 |
| air_humidity | 14.54144 |
| air_temperature | 23.631212 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 53.0 |
| direct_solar_radiation | 863.25 |
| hour | 14.0 |
| htg_setpoint | 21.0 |
| month | 2.0 |
| outdoor_humidity | 29.0 |
| outdoor_temperature | 0.75334334 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1029345.3 |
| wind_direction | 292.5 |
| wind_speed | 5.325 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.67e+03 |
| n_updates | 14849 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 13%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1158.7903 |
| air_humidity | 26.88448 |
| air_temperature | 23.092854 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 66.5 |
| direct_solar_radiation | 0.0 |
| hour | 15.0 |
| htg_setpoint | 17.0 |
| month | 2.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | 0.5057328 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1042911.25 |
| wind_direction | 182.5 |
| wind_speed | 3.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.99e+03 |
| n_updates | 14874 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 13%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 73.7766 |
| air_humidity | 25.315073 |
| air_temperature | 20.000492 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 38.75 |
| direct_solar_radiation | 0.0 |
| hour | 16.0 |
| htg_setpoint | 20.0 |
| month | 2.0 |
| outdoor_humidity | 43.75 |
| outdoor_temperature | 5.228516 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 66398.945 |
| wind_direction | 332.5 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.06e+03 |
| n_updates | 14899 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 13%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 15.07751 |
| air_temperature | 22.42386 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 22.0 |
| month | 2.0 |
| outdoor_humidity | 45.25 |
| outdoor_temperature | 3.532443 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 245.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 8.73e+03 |
| n_updates | 14924 |
----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 13%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 26.815527 |
| air_temperature | 24.792336 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 17.0 |
| month | 2.0 |
| outdoor_humidity | 73.5 |
| outdoor_temperature | 2.8700993 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 130.0 |
| wind_speed | 2.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.84e+03 |
| n_updates | 14949 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 14%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 24.373877 |
| air_temperature | 23.764162 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 21.0 |
| month | 2.0 |
| outdoor_humidity | 56.25 |
| outdoor_temperature | 3.9288108 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 227.5 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.23e+03 |
| n_updates | 14974 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 14%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 33.13989 |
| air_temperature | 22.858171 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 22.0 |
| month | 2.0 |
| outdoor_humidity | 85.0 |
| outdoor_temperature | 2.637647 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 75.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.47e+03 |
| n_updates | 14999 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 14%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 32.451893 |
| air_temperature | 19.095592 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 15.0 |
| month | 2.0 |
| outdoor_humidity | 63.25 |
| outdoor_temperature | 3.9432404 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 330.0 |
| wind_speed | 6.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.45e+03 |
| n_updates | 15024 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 14%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 962.5944 |
| air_humidity | 23.422165 |
| air_temperature | 22.000002 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 22.0 |
| month | 2.0 |
| outdoor_humidity | 54.75 |
| outdoor_temperature | 1.9439363 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 866335.0 |
| wind_direction | 250.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.13e+03 |
| n_updates | 15049 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 15%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 25.20381 |
| air_temperature | 22.000006 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 22.0 |
| month | 2.0 |
| outdoor_humidity | 92.0 |
| outdoor_temperature | 3.9635112 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 57.5 |
| wind_speed | 1.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.72e+03 |
| n_updates | 15074 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 15%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 1978.8235 |
| air_humidity | 17.958961 |
| air_temperature | 19.000006 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 19.0 |
| month | 2.0 |
| outdoor_humidity | 35.0 |
| outdoor_temperature | -3.1372092 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1780941.1 |
| wind_direction | 312.5 |
| wind_speed | 6.325 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.68e+03 |
| n_updates | 15099 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 16%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1651.2651 |
| air_humidity | 21.80585 |
| air_temperature | 21.99999 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 22.0 |
| month | 2.0 |
| outdoor_humidity | 55.0 |
| outdoor_temperature | -1.4994174 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1486138.6 |
| wind_direction | 35.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.74e+03 |
| n_updates | 15124 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 16%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 1477.7244 |
| air_humidity | 19.804928 |
| air_temperature | 19.0 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 19.0 |
| month | 2.0 |
| outdoor_humidity | 51.0 |
| outdoor_temperature | -0.6317136 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1329952.0 |
| wind_direction | 80.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.89e+03 |
| n_updates | 15149 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 16%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 1173.0521 |
| air_humidity | 40.49864 |
| air_temperature | 19.615831 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 18.0 |
| month | 2.0 |
| outdoor_humidity | 97.0 |
| outdoor_temperature | 0.89164776 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1055746.9 |
| wind_direction | 102.5 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.42e+03 |
| n_updates | 15174 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 16%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 810.88544 |
| air_humidity | 26.834318 |
| air_temperature | 20.000088 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 20.0 |
| month | 3.0 |
| outdoor_humidity | 59.25 |
| outdoor_temperature | 5.296541 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 729796.9 |
| wind_direction | 335.0 |
| wind_speed | 1.025 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.87e+03 |
| n_updates | 15199 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 17%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 566.93365 |
| air_humidity | 37.77567 |
| air_temperature | 18.473743 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 18.0 |
| month | 3.0 |
| outdoor_humidity | 97.0 |
| outdoor_temperature | 4.3673377 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 510240.28 |
| wind_direction | 30.0 |
| wind_speed | 0.375 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.1e+03 |
| n_updates | 15224 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 17%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 94.785126 |
| air_humidity | 36.622604 |
| air_temperature | 19.349455 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 22.0 |
| direct_solar_radiation | 146.25 |
| hour | 6.0 |
| htg_setpoint | 15.0 |
| month | 3.0 |
| outdoor_humidity | 61.0 |
| outdoor_temperature | 9.245056 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 83250.836 |
| wind_direction | 302.5 |
| wind_speed | 6.425 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.7e+03 |
| n_updates | 15249 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 17%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 244.03078 |
| air_humidity | 40.965393 |
| air_temperature | 18.794714 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 77.75 |
| direct_solar_radiation | 21.0 |
| hour | 7.0 |
| htg_setpoint | 20.0 |
| month | 3.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 6.478311 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 219627.69 |
| wind_direction | 140.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.13e+03 |
| n_updates | 15274 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 17%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 44.28175 |
| air_temperature | 21.336182 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 78.0 |
| direct_solar_radiation | 0.0 |
| hour | 8.0 |
| htg_setpoint | 21.0 |
| month | 3.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 7.5593534 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 95.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.61e+03 |
| n_updates | 15299 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 18%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 764.0725 |
| air_humidity | 50.251144 |
| air_temperature | 22.643566 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 88.75 |
| direct_solar_radiation | 0.0 |
| hour | 9.0 |
| htg_setpoint | 22.0 |
| month | 3.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 11.124284 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 687665.25 |
| wind_direction | 195.0 |
| wind_speed | 9.8 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.52e+03 |
| n_updates | 15324 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 18%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 871.7625 |
| air_humidity | 23.494152 |
| air_temperature | 23.261553 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 143.5 |
| direct_solar_radiation | 801.0 |
| hour | 10.0 |
| htg_setpoint | 15.0 |
| month | 3.0 |
| outdoor_humidity | 48.0 |
| outdoor_temperature | 6.961959 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 784586.25 |
| wind_direction | 292.5 |
| wind_speed | 5.375 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.61e+03 |
| n_updates | 15349 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 18%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 755.6465 |
| air_humidity | 33.514217 |
| air_temperature | 22.651453 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 200.25 |
| direct_solar_radiation | 1.5 |
| hour | 11.0 |
| htg_setpoint | 21.0 |
| month | 3.0 |
| outdoor_humidity | 99.0 |
| outdoor_temperature | 3.141846 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 680081.9 |
| wind_direction | 30.0 |
| wind_speed | 5.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 786 |
| n_updates | 15374 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 19%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 33.8206 |
| air_temperature | 20.309654 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 206.0 |
| direct_solar_radiation | 9.0 |
| hour | 12.0 |
| htg_setpoint | 17.0 |
| month | 3.0 |
| outdoor_humidity | 79.0 |
| outdoor_temperature | 6.0417705 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 150.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.67e+03 |
| n_updates | 15399 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 19%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 26.79647 |
| air_temperature | 20.653496 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 287.5 |
| direct_solar_radiation | 348.25 |
| hour | 13.0 |
| htg_setpoint | 16.0 |
| month | 3.0 |
| outdoor_humidity | 53.5 |
| outdoor_temperature | 5.8445897 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 37.5 |
| wind_speed | 9.675 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.97e+03 |
| n_updates | 15424 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 19%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 20.176092 |
| air_temperature | 24.43434 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 119.0 |
| direct_solar_radiation | 757.5 |
| hour | 14.0 |
| htg_setpoint | 22.0 |
| month | 3.0 |
| outdoor_humidity | 29.75 |
| outdoor_temperature | 14.736623 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 257.5 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.2e+03 |
| n_updates | 15449 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 19%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 19.772951 |
| air_temperature | 23.21499 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 108.75 |
| direct_solar_radiation | 49.25 |
| hour | 15.0 |
| htg_setpoint | 21.0 |
| month | 3.0 |
| outdoor_humidity | 36.0 |
| outdoor_temperature | 5.9866753 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 287.5 |
| wind_speed | 9.4 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 8.97e+03 |
| n_updates | 15474 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 20%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 809.15594 |
| air_humidity | 19.844421 |
| air_temperature | 23.992252 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 66.25 |
| direct_solar_radiation | 453.5 |
| hour | 16.0 |
| htg_setpoint | 20.0 |
| month | 3.0 |
| outdoor_humidity | 34.0 |
| outdoor_temperature | 7.26631 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 727706.06 |
| wind_direction | 310.0 |
| wind_speed | 10.55 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.22e+03 |
| n_updates | 15499 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 20%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 30.93134 |
| air_temperature | 23.089472 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 20.25 |
| direct_solar_radiation | 96.0 |
| hour | 17.0 |
| htg_setpoint | 20.0 |
| month | 3.0 |
| outdoor_humidity | 91.25 |
| outdoor_temperature | 3.1187332 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 170.0 |
| wind_speed | 9.025 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.82e+03 |
| n_updates | 15524 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 20%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 208.74844 |
| air_humidity | 18.482845 |
| air_temperature | 24.876616 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 18.0 |
| month | 3.0 |
| outdoor_humidity | 22.0 |
| outdoor_temperature | 12.427065 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 186888.42 |
| wind_direction | 292.5 |
| wind_speed | 5.575 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.58e+03 |
| n_updates | 15549 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 20%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1466.7415 |
| air_humidity | 17.671227 |
| air_temperature | 20.999994 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 21.0 |
| month | 3.0 |
| outdoor_humidity | 94.75 |
| outdoor_temperature | -0.5767989 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1320067.4 |
| wind_direction | 40.0 |
| wind_speed | 10.675 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 894 |
| n_updates | 15574 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 21%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1806.4314 |
| air_humidity | 19.1926 |
| air_temperature | 21.0 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 21.0 |
| month | 3.0 |
| outdoor_humidity | 90.0 |
| outdoor_temperature | -2.2752483 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1625788.2 |
| wind_direction | 32.5 |
| wind_speed | 3.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.17e+03 |
| n_updates | 15599 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 21%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 35.01203 |
| air_temperature | 21.75387 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 21.0 |
| month | 3.0 |
| outdoor_humidity | 89.0 |
| outdoor_temperature | 2.158653 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 145.0 |
| wind_speed | 4.375 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.04e+03 |
| n_updates | 15624 |
----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 21%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 31.351454 |
| air_temperature | 21.016546 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 21.0 |
| month | 3.0 |
| outdoor_humidity | 56.25 |
| outdoor_temperature | 2.4189992 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 5.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 999 |
| n_updates | 15649 |
-----------------------------------------------
Progress: |**********************-----------------------------------------------------------------------------| 22%-----------------------------------------------
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 449.45572 |
| air_humidity | 35.932343 |
| air_temperature | 21.472187 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 22.0 |
| month | 3.0 |
| outdoor_humidity | 95.0 |
| outdoor_temperature | 7.158784 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 404510.16 |
| wind_direction | 165.0 |
| wind_speed | 3.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.89e+03 |
| n_updates | 15674 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 22%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 1563.6162 |
| air_humidity | 24.247192 |
| air_temperature | 19.999989 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 20.0 |
| month | 3.0 |
| outdoor_humidity | 44.0 |
| outdoor_temperature | -1.0611725 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1407254.6 |
| wind_direction | 297.5 |
| wind_speed | 8.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.03e+03 |
| n_updates | 15699 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 22%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 2125.3613 |
| air_humidity | 17.254232 |
| air_temperature | 19.878681 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 17.0 |
| month | 3.0 |
| outdoor_humidity | 33.5 |
| outdoor_temperature | -3.8698978 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1912825.1 |
| wind_direction | 347.5 |
| wind_speed | 4.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.76e+03 |
| n_updates | 15724 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 23%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 20.619474 |
| air_temperature | 19.133396 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 15.0 |
| month | 3.0 |
| outdoor_humidity | 69.0 |
| outdoor_temperature | 2.4355738 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 250.0 |
| wind_speed | 6.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.29e+03 |
| n_updates | 15749 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 23%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 381.8151 |
| air_humidity | 37.59117 |
| air_temperature | 21.439528 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 22.0 |
| month | 3.0 |
| outdoor_humidity | 89.0 |
| outdoor_temperature | 8.856663 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 343633.6 |
| wind_direction | 172.5 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.48e+03 |
| n_updates | 15774 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 23%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 42.63189 |
| air_temperature | 19.000011 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 19.0 |
| month | 3.0 |
| outdoor_humidity | 97.0 |
| outdoor_temperature | 5.119897 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 100.0 |
| wind_speed | 1.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.31e+03 |
| n_updates | 15799 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 23%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 50.85754 |
| air_temperature | 18.377762 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 16.0 |
| month | 3.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 8.373751 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.95e+03 |
| n_updates | 15824 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 24%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 244.03078 |
| air_humidity | 35.561874 |
| air_temperature | 21.873852 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 74.0 |
| direct_solar_radiation | 11.25 |
| hour | 6.0 |
| htg_setpoint | 22.0 |
| month | 3.0 |
| outdoor_humidity | 71.0 |
| outdoor_temperature | 10.225957 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 219627.69 |
| wind_direction | 0.0 |
| wind_speed | 5.575 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.87e+03 |
| n_updates | 15849 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 24%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 244.03078 |
| air_humidity | 26.675598 |
| air_temperature | 18.554829 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 115.5 |
| direct_solar_radiation | 615.0 |
| hour | 7.0 |
| htg_setpoint | 17.0 |
| month | 3.0 |
| outdoor_humidity | 68.0 |
| outdoor_temperature | 4.2019925 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 219627.69 |
| wind_direction | 40.0 |
| wind_speed | 5.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.62e+03 |
| n_updates | 15874 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 24%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 40.36259 |
| air_temperature | 18.407398 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 130.75 |
| direct_solar_radiation | 0.0 |
| hour | 8.0 |
| htg_setpoint | 17.0 |
| month | 3.0 |
| outdoor_humidity | 84.25 |
| outdoor_temperature | 3.0041485 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 77.5 |
| wind_speed | 3.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 9.13e+03 |
| n_updates | 15899 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 25%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 219.27303 |
| air_humidity | 40.82527 |
| air_temperature | 19.000004 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 204.25 |
| direct_solar_radiation | 1.5 |
| hour | 9.0 |
| htg_setpoint | 19.0 |
| month | 3.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 6.623772 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 197345.73 |
| wind_direction | 7.5 |
| wind_speed | 5.575 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.89e+03 |
| n_updates | 15924 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 25%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 35.68691 |
| air_temperature | 21.785128 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 208.25 |
| direct_solar_radiation | 0.0 |
| hour | 10.0 |
| htg_setpoint | 16.0 |
| month | 4.0 |
| outdoor_humidity | 88.0 |
| outdoor_temperature | 5.421297 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 147.5 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.76e+03 |
| n_updates | 15949 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 25%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 840.3833 |
| air_humidity | 25.465723 |
| air_temperature | 22.999157 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 324.5 |
| direct_solar_radiation | 198.5 |
| hour | 11.0 |
| htg_setpoint | 22.0 |
| month | 4.0 |
| outdoor_humidity | 42.75 |
| outdoor_temperature | 7.6123447 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 755894.1 |
| wind_direction | 300.0 |
| wind_speed | 7.575 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.12e+03 |
| n_updates | 15974 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 25%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 734.6666 |
| air_humidity | 18.650326 |
| air_temperature | 22.999714 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 117.75 |
| direct_solar_radiation | 915.75 |
| hour | 12.0 |
| htg_setpoint | 22.0 |
| month | 4.0 |
| outdoor_humidity | 36.25 |
| outdoor_temperature | 8.88295 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 661284.7 |
| wind_direction | 237.5 |
| wind_speed | 5.05 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.51e+03 |
| n_updates | 15999 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 26%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 500.5111 |
| air_humidity | 24.855114 |
| air_temperature | 24.99908 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 231.25 |
| direct_solar_radiation | 663.5 |
| hour | 13.0 |
| htg_setpoint | 20.0 |
| month | 4.0 |
| outdoor_humidity | 46.5 |
| outdoor_temperature | 11.0018635 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 450460.0 |
| wind_direction | 197.5 |
| wind_speed | 6.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.02e+03 |
| n_updates | 16024 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 26%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 255.18027 |
| air_humidity | 40.3879 |
| air_temperature | 25.245584 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 182.25 |
| direct_solar_radiation | 0.0 |
| hour | 14.0 |
| htg_setpoint | 17.0 |
| month | 4.0 |
| outdoor_humidity | 76.0 |
| outdoor_temperature | 12.835742 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 229662.23 |
| wind_direction | 237.5 |
| wind_speed | 5.425 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.64e+03 |
| n_updates | 16049 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 26%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 61.144527 |
| air_temperature | 25.565943 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 157.25 |
| direct_solar_radiation | 440.5 |
| hour | 15.0 |
| htg_setpoint | 22.0 |
| month | 4.0 |
| outdoor_humidity | 72.0 |
| outdoor_temperature | 16.46367 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 6.575 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.01e+03 |
| n_updates | 16074 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 26%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 56.334118 |
| air_temperature | 26.882177 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 113.5 |
| direct_solar_radiation | 319.5 |
| hour | 16.0 |
| htg_setpoint | 18.0 |
| month | 4.0 |
| outdoor_humidity | 35.5 |
| outdoor_temperature | 26.066725 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 255.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.18e+03 |
| n_updates | 16099 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 27%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 43.002823 |
| air_temperature | 29.800293 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 55.75 |
| direct_solar_radiation | 1.5 |
| hour | 17.0 |
| htg_setpoint | 18.0 |
| month | 4.0 |
| outdoor_humidity | 72.5 |
| outdoor_temperature | 16.096746 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 187.5 |
| wind_speed | 5.425 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 979 |
| n_updates | 16124 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 27%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 48.986477 |
| air_temperature | 28.824877 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 20.0 |
| month | 4.0 |
| outdoor_humidity | 87.75 |
| outdoor_temperature | 14.492639 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 187.5 |
| wind_speed | 2.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.56e+03 |
| n_updates | 16149 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 27%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 42.606262 |
| air_temperature | 27.829678 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 17.0 |
| month | 4.0 |
| outdoor_humidity | 33.0 |
| outdoor_temperature | 16.676575 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 292.5 |
| wind_speed | 10.55 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.8e+03 |
| n_updates | 16174 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 28%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 33.19549 |
| air_temperature | 25.93673 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 18.0 |
| month | 4.0 |
| outdoor_humidity | 16.0 |
| outdoor_temperature | 9.1879835 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 327.5 |
| wind_speed | 9.4 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.69e+04 |
| n_updates | 16199 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 28%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 36.42534 |
| air_temperature | 24.6196 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 16.0 |
| month | 4.0 |
| outdoor_humidity | 36.5 |
| outdoor_temperature | 6.809757 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 180.0 |
| wind_speed | 2.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 834 |
| n_updates | 16224 |
----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 28%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 49.749786 |
| air_temperature | 19.409586 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 21.0 |
| month | 4.0 |
| outdoor_humidity | 96.75 |
| outdoor_temperature | 4.1420374 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 70.0 |
| wind_speed | 5.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 7.98e+03 |
| n_updates | 16249 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 29%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 46.242737 |
| air_temperature | 20.562092 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 21.0 |
| month | 4.0 |
| outdoor_humidity | 85.75 |
| outdoor_temperature | 7.1730056 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 107.5 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.95e+03 |
| n_updates | 16274 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 29%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 88.64957 |
| air_temperature | 20.738081 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 19.0 |
| month | 4.0 |
| outdoor_humidity | 95.25 |
| outdoor_temperature | 12.110972 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 270.0 |
| wind_speed | 5.825 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 958 |
| n_updates | 16299 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 29%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 72.871155 |
| air_temperature | 22.278757 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 19.0 |
| month | 4.0 |
| outdoor_humidity | 84.5 |
| outdoor_temperature | 13.553884 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.06e+03 |
| n_updates | 16324 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 29%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 81.26202 |
| air_temperature | 19.654593 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 22.0 |
| month | 4.0 |
| outdoor_humidity | 90.75 |
| outdoor_temperature | 7.9804134 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 62.5 |
| wind_speed | 5.425 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.12e+03 |
| n_updates | 16349 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 30%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 71.018555 |
| air_temperature | 19.43056 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 16.0 |
| month | 4.0 |
| outdoor_humidity | 70.0 |
| outdoor_temperature | 4.530317 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 47.5 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 854 |
| n_updates | 16374 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 30%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 56.09686 |
| air_temperature | 19.882326 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 16.0 |
| month | 4.0 |
| outdoor_humidity | 65.0 |
| outdoor_temperature | 7.630194 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 90.0 |
| wind_speed | 4.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.62e+03 |
| n_updates | 16399 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 30%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 58.39541 |
| air_temperature | 18.942545 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 21.5 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 21.0 |
| month | 4.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 4.826478 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 27.5 |
| wind_speed | 10.55 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.45e+03 |
| n_updates | 16424 |
-----------------------------------------------
wandb: WARNING (User provided step: 105117 is less than current step: 105118. Dropping entry: {'rollout/ep_len_mean': 35040.0, '_timestamp': 1700214338.3238974}).
wandb: WARNING (User provided step: 105117 is less than current step: 105118. Dropping entry: {'rollout/ep_rew_mean': -12207.1521785, '_timestamp': 1700214338.324025}).
wandb: WARNING (User provided step: 105117 is less than current step: 105118. Dropping entry: {'rollout/exploration_rate': 0.05, '_timestamp': 1700214338.3241777}).
wandb: WARNING (User provided step: 105117 is less than current step: 105118. Dropping entry: {'time/episodes': 2, '_timestamp': 1700214338.324233}).
wandb: WARNING (User provided step: 105117 is less than current step: 105118. Dropping entry: {'time/fps': 952, '_timestamp': 1700214338.324258}).
wandb: WARNING (User provided step: 105117 is less than current step: 105118. Dropping entry: {'time/time_elapsed': 110, '_timestamp': 1700214338.3242805}).
wandb: WARNING (User provided step: 105117 is less than current step: 105118. Dropping entry: {'time/total_timesteps': 105117, '_timestamp': 1700214338.3243134}).
--------------------------------------------------------------------------------------------------------------| 31%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 61.804073 |
| air_temperature | 17.933743 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 65.25 |
| direct_solar_radiation | 0.0 |
| hour | 6.0 |
| htg_setpoint | 20.0 |
| month | 4.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 5.314055 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 255.0 |
| wind_speed | 7.325 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.75e+03 |
| n_updates | 16449 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 31%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 139.62027 |
| air_humidity | 57.48164 |
| air_temperature | 19.324568 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 81.25 |
| direct_solar_radiation | 754.0 |
| hour | 7.0 |
| htg_setpoint | 15.0 |
| month | 4.0 |
| outdoor_humidity | 62.25 |
| outdoor_temperature | 11.337564 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 127898.09 |
| wind_direction | 242.5 |
| wind_speed | 4.5 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.4e+03 |
| n_updates | 16474 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 31%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 423.0795 |
| air_humidity | 54.558548 |
| air_temperature | 21.274569 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 249.5 |
| direct_solar_radiation | 331.5 |
| hour | 8.0 |
| htg_setpoint | 20.0 |
| month | 4.0 |
| outdoor_humidity | 73.75 |
| outdoor_temperature | 11.623175 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 391077.4 |
| wind_direction | 135.0 |
| wind_speed | 2.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.03e+03 |
| n_updates | 16499 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 31%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 426.25082 |
| air_humidity | 42.10922 |
| air_temperature | 24.387661 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 169.5 |
| direct_solar_radiation | 783.75 |
| hour | 9.0 |
| htg_setpoint | 17.0 |
| month | 4.0 |
| outdoor_humidity | 53.25 |
| outdoor_temperature | 15.680944 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 383625.75 |
| wind_direction | 355.0 |
| wind_speed | 7.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.35e+03 |
| n_updates | 16524 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 32%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 3278.4285 |
| air_humidity | 51.37957 |
| air_temperature | 23.440716 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 440.75 |
| direct_solar_radiation | 415.75 |
| hour | 10.0 |
| htg_setpoint | 21.0 |
| month | 4.0 |
| outdoor_humidity | 52.0 |
| outdoor_temperature | 19.777857 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 2925658.0 |
| wind_direction | 175.0 |
| wind_speed | 4.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.25e+03 |
| n_updates | 16549 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 32%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 67.33587 |
| air_temperature | 23.060978 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 423.25 |
| direct_solar_radiation | 154.25 |
| hour | 11.0 |
| htg_setpoint | 22.0 |
| month | 4.0 |
| outdoor_humidity | 70.0 |
| outdoor_temperature | 19.105343 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 227.5 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 8e+03 |
| n_updates | 16574 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 32%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 59.69443 |
| air_temperature | 25.178434 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 182.75 |
| direct_solar_radiation | 738.75 |
| hour | 12.0 |
| htg_setpoint | 21.0 |
| month | 4.0 |
| outdoor_humidity | 45.5 |
| outdoor_temperature | 16.890213 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 105.0 |
| wind_speed | 9.025 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 777 |
| n_updates | 16599 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 32%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 886.88434 |
| air_humidity | 43.01376 |
| air_temperature | 22.757463 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 231.5 |
| direct_solar_radiation | 0.0 |
| hour | 13.0 |
| htg_setpoint | 22.0 |
| month | 4.0 |
| outdoor_humidity | 76.25 |
| outdoor_temperature | 12.084203 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 798195.9 |
| wind_direction | 112.5 |
| wind_speed | 5.05 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.67e+03 |
| n_updates | 16624 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 33%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 202.23326 |
| air_humidity | 55.07219 |
| air_temperature | 25.159515 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 139.0 |
| direct_solar_radiation | 0.0 |
| hour | 14.0 |
| htg_setpoint | 18.0 |
| month | 4.0 |
| outdoor_humidity | 92.5 |
| outdoor_temperature | 14.396703 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 182009.94 |
| wind_direction | 347.5 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.39e+03 |
| n_updates | 16649 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 33%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 259.9499 |
| air_humidity | 24.07339 |
| air_temperature | 26.803598 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 273.5 |
| direct_solar_radiation | 169.0 |
| hour | 15.0 |
| htg_setpoint | 15.0 |
| month | 5.0 |
| outdoor_humidity | 29.75 |
| outdoor_temperature | 15.0922365 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 233954.9 |
| wind_direction | 117.5 |
| wind_speed | 6.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.28e+03 |
| n_updates | 16674 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 33%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1844.631 |
| air_humidity | 28.542942 |
| air_temperature | 24.000408 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 134.0 |
| direct_solar_radiation | 401.75 |
| hour | 16.0 |
| htg_setpoint | 21.0 |
| month | 5.0 |
| outdoor_humidity | 34.75 |
| outdoor_temperature | 17.741638 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1660167.9 |
| wind_direction | 95.0 |
| wind_speed | 8.925 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.33e+03 |
| n_updates | 16699 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 34%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 39.95888 |
| air_temperature | 28.465874 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 75.75 |
| direct_solar_radiation | 29.25 |
| hour | 17.0 |
| htg_setpoint | 20.0 |
| month | 5.0 |
| outdoor_humidity | 56.0 |
| outdoor_temperature | 14.858121 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 17.5 |
| wind_speed | 7.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.93e+03 |
| n_updates | 16724 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 34%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 70.26563 |
| air_temperature | 22.1756 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 12.75 |
| direct_solar_radiation | 27.75 |
| hour | 18.0 |
| htg_setpoint | 21.0 |
| month | 5.0 |
| outdoor_humidity | 84.75 |
| outdoor_temperature | 15.311967 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 100.0 |
| wind_speed | 2.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.59e+03 |
| n_updates | 16749 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 34%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 60.651237 |
| air_temperature | 24.642591 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 20.0 |
| month | 5.0 |
| outdoor_humidity | 89.75 |
| outdoor_temperature | 14.209018 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 137.5 |
| wind_speed | 2.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.33e+03 |
| n_updates | 16774 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 34%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 64.60104 |
| air_temperature | 25.386179 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 19.0 |
| month | 5.0 |
| outdoor_humidity | 93.75 |
| outdoor_temperature | 11.908129 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 97.5 |
| wind_speed | 3.725 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.87e+03 |
| n_updates | 16799 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 35%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 80.14912 |
| air_temperature | 23.695042 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 21.0 |
| month | 5.0 |
| outdoor_humidity | 99.0 |
| outdoor_temperature | 13.742707 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 100.0 |
| wind_speed | 6.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 653 |
| n_updates | 16824 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 35%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 83.65019 |
| air_temperature | 22.592537 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 15.0 |
| month | 5.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 14.277803 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 357.5 |
| wind_speed | 0.775 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.22e+03 |
| n_updates | 16849 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 35%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 70.704895 |
| air_temperature | 23.187885 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 22.0 |
| month | 5.0 |
| outdoor_humidity | 63.75 |
| outdoor_temperature | 14.677337 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 345.0 |
| wind_speed | 2.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.39e+03 |
| n_updates | 16874 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 36%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 62.997635 |
| air_temperature | 23.428854 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 22.0 |
| month | 5.0 |
| outdoor_humidity | 52.0 |
| outdoor_temperature | 14.541368 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 12.5 |
| wind_speed | 4.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.54e+03 |
| n_updates | 16899 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 36%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 69.104126 |
| air_temperature | 21.876242 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 20.0 |
| month | 5.0 |
| outdoor_humidity | 82.25 |
| outdoor_temperature | 9.96343 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 240.0 |
| wind_speed | 4.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.75e+03 |
| n_updates | 16924 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 36%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 69.86454 |
| air_temperature | 21.600565 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 16.0 |
| month | 5.0 |
| outdoor_humidity | 39.75 |
| outdoor_temperature | 14.400382 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 15.0 |
| wind_speed | 5.175 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.64e+03 |
| n_updates | 16949 |
-----------------------------------------------
-----------------------------------------------*--------------------------------------------------------------| 37%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 56.518505 |
| air_temperature | 20.212349 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 22.0 |
| month | 5.0 |
| outdoor_humidity | 85.0 |
| outdoor_temperature | 6.7360797 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 37.5 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.12e+04 |
| n_updates | 16974 |
-----------------------------------------------
-----------------------------------------------*--------------------------------------------------------------| 37%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 79.30012 |
| air_temperature | 20.133348 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 18.5 |
| direct_solar_radiation | 26.75 |
| hour | 4.0 |
| htg_setpoint | 22.0 |
| month | 5.0 |
| outdoor_humidity | 83.0 |
| outdoor_temperature | 6.6733184 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 55.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.85e+03 |
| n_updates | 16999 |
-----------------------------------------------
-----------------------------------------------*--------------------------------------------------------------| 37%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 71.16668 |
| air_temperature | 19.883177 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 87.5 |
| direct_solar_radiation | 222.25 |
| hour | 5.0 |
| htg_setpoint | 20.0 |
| month | 5.0 |
| outdoor_humidity | 80.75 |
| outdoor_temperature | 10.129374 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 27.5 |
| wind_speed | 3.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.58e+03 |
| n_updates | 17024 |
-----------------------------------------------
-----------------------------------------------*--------------------------------------------------------------| 37%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 79.16959 |
| air_temperature | 20.764967 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 133.75 |
| direct_solar_radiation | 459.75 |
| hour | 6.0 |
| htg_setpoint | 15.0 |
| month | 5.0 |
| outdoor_humidity | 73.25 |
| outdoor_temperature | 14.322216 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 45.0 |
| wind_speed | 3.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.48e+03 |
| n_updates | 17049 |
-----------------------------------------------
-----------------------------------------------**-------------------------------------------------------------| 38%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 81.00924 |
| air_temperature | 21.80383 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 247.75 |
| direct_solar_radiation | 337.25 |
| hour | 7.0 |
| htg_setpoint | 21.0 |
| month | 5.0 |
| outdoor_humidity | 85.5 |
| outdoor_temperature | 15.378115 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 40.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.81e+03 |
| n_updates | 17074 |
-----------------------------------------------
-----------------------------------------------**-------------------------------------------------------------| 38%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 79.258354 |
| air_temperature | 22.053274 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 219.25 |
| direct_solar_radiation | 9.0 |
| hour | 8.0 |
| htg_setpoint | 15.0 |
| month | 5.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 18.406048 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 80.0 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.04e+03 |
| n_updates | 17099 |
-----------------------------------------------
-----------------------------------------------**-------------------------------------------------------------| 38%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 691.146 |
| air_humidity | 46.539497 |
| air_temperature | 24.863531 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 200.0 |
| direct_solar_radiation | 737.25 |
| hour | 9.0 |
| htg_setpoint | 15.0 |
| month | 5.0 |
| outdoor_humidity | 52.5 |
| outdoor_temperature | 17.3546 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 622023.5 |
| wind_direction | 350.0 |
| wind_speed | 9.775 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.86e+03 |
| n_updates | 17124 |
-----------------------------------------------
-----------------------------------------------**-------------------------------------------------------------| 38%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 3710.4148 |
| air_humidity | 39.002415 |
| air_temperature | 23.876598 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 239.5 |
| direct_solar_radiation | 707.5 |
| hour | 10.0 |
| htg_setpoint | 21.0 |
| month | 5.0 |
| outdoor_humidity | 35.5 |
| outdoor_temperature | 20.152966 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 3339373.2 |
| wind_direction | 27.5 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.82e+03 |
| n_updates | 17149 |
-----------------------------------------------
-----------------------------------------------***------------------------------------------------------------| 39%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 3688.425 |
| air_humidity | 43.778458 |
| air_temperature | 23.66983 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 443.25 |
| direct_solar_radiation | 133.5 |
| hour | 11.0 |
| htg_setpoint | 20.0 |
| month | 5.0 |
| outdoor_humidity | 38.75 |
| outdoor_temperature | 22.107475 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 3319582.5 |
| wind_direction | 157.5 |
| wind_speed | 3.725 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.57e+03 |
| n_updates | 17174 |
-----------------------------------------------
-----------------------------------------------***------------------------------------------------------------| 39%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 917.6637 |
| air_humidity | 56.962753 |
| air_temperature | 23.999311 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 259.5 |
| direct_solar_radiation | 4.5 |
| hour | 12.0 |
| htg_setpoint | 21.0 |
| month | 5.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 15.511669 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 825897.3 |
| wind_direction | 127.5 |
| wind_speed | 4.875 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.99e+03 |
| n_updates | 17199 |
-----------------------------------------------
-----------------------------------------------***------------------------------------------------------------| 39%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 858.77216 |
| air_humidity | 57.474483 |
| air_temperature | 25.882996 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 163.5 |
| direct_solar_radiation | 0.0 |
| hour | 13.0 |
| htg_setpoint | 16.0 |
| month | 5.0 |
| outdoor_humidity | 97.0 |
| outdoor_temperature | 17.411175 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 772894.94 |
| wind_direction | 175.0 |
| wind_speed | 7.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.69e+03 |
| n_updates | 17224 |
-----------------------------------------------
-----------------------------------------------****-----------------------------------------------------------| 40%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 78.20754 |
| air_temperature | 25.337343 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 280.5 |
| direct_solar_radiation | 431.75 |
| hour | 14.0 |
| htg_setpoint | 19.0 |
| month | 5.0 |
| outdoor_humidity | 40.25 |
| outdoor_temperature | 19.513725 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 230.0 |
| wind_speed | 8.525 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.94e+03 |
| n_updates | 17249 |
-----------------------------------------------
-----------------------------------------------****-----------------------------------------------------------| 40%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 75.899506 |
| air_temperature | 25.854675 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 263.0 |
| direct_solar_radiation | 43.0 |
| hour | 15.0 |
| htg_setpoint | 18.0 |
| month | 5.0 |
| outdoor_humidity | 38.75 |
| outdoor_temperature | 19.162733 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 287.5 |
| wind_speed | 7.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.05e+03 |
| n_updates | 17274 |
-----------------------------------------------
-----------------------------------------------****-----------------------------------------------------------| 40%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 4173.7295 |
| air_humidity | 37.54742 |
| air_temperature | 23.964441 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 121.75 |
| direct_solar_radiation | 547.25 |
| hour | 16.0 |
| htg_setpoint | 15.0 |
| month | 5.0 |
| outdoor_humidity | 30.25 |
| outdoor_temperature | 22.092154 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 3756356.5 |
| wind_direction | 320.0 |
| wind_speed | 8.775 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.53e+03 |
| n_updates | 17299 |
-----------------------------------------------
-----------------------------------------------****-----------------------------------------------------------| 40%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 41.42586 |
| air_temperature | 29.204458 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 105.75 |
| direct_solar_radiation | 76.5 |
| hour | 17.0 |
| htg_setpoint | 16.0 |
| month | 5.0 |
| outdoor_humidity | 57.5 |
| outdoor_temperature | 19.358492 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 172.5 |
| wind_speed | 4.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.76e+03 |
| n_updates | 17324 |
-----------------------------------------------
-----------------------------------------------*****----------------------------------------------------------| 41%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 53.49464 |
| air_temperature | 29.286299 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 39.75 |
| direct_solar_radiation | 78.75 |
| hour | 18.0 |
| htg_setpoint | 19.0 |
| month | 5.0 |
| outdoor_humidity | 52.5 |
| outdoor_temperature | 24.95861 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 17.5 |
| wind_speed | 0.9 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.01e+03 |
| n_updates | 17349 |
-----------------------------------------------
-----------------------------------------------*****----------------------------------------------------------| 41%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 53.129776 |
| air_temperature | 29.402967 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 21.0 |
| month | 5.0 |
| outdoor_humidity | 60.5 |
| outdoor_temperature | 21.23685 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 197.5 |
| wind_speed | 2.325 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.58e+04 |
| n_updates | 17374 |
-----------------------------------------------
-----------------------------------------------*****----------------------------------------------------------| 41%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 62.3626 |
| air_temperature | 27.33943 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 15.0 |
| month | 5.0 |
| outdoor_humidity | 76.5 |
| outdoor_temperature | 18.870724 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 140.0 |
| wind_speed | 1.65 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.59e+03 |
| n_updates | 17399 |
-----------------------------------------------
----------------------------------------------******----------------------------------------------------------| 41%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 66.01241 |
| air_temperature | 26.20268 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 15.0 |
| month | 6.0 |
| outdoor_humidity | 47.75 |
| outdoor_temperature | 21.07225 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 347.5 |
| wind_speed | 7.325 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2e+03 |
| n_updates | 17424 |
----------------------------------------------
-----------------------------------------------******---------------------------------------------------------| 42%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 74.478065 |
| air_temperature | 24.307499 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 21.0 |
| month | 6.0 |
| outdoor_humidity | 48.25 |
| outdoor_temperature | 16.253143 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 327.5 |
| wind_speed | 6.575 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.35e+03 |
| n_updates | 17449 |
-----------------------------------------------
-----------------------------------------------******---------------------------------------------------------| 42%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 69.24167 |
| air_temperature | 23.637384 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 17.0 |
| month | 6.0 |
| outdoor_humidity | 38.25 |
| outdoor_temperature | 19.995474 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 337.5 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.55e+03 |
| n_updates | 17474 |
-----------------------------------------------
-----------------------------------------------*******--------------------------------------------------------| 43%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 72.323845 |
| air_temperature | 23.203812 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 21.0 |
| month | 6.0 |
| outdoor_humidity | 60.75 |
| outdoor_temperature | 16.461828 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 235.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 613 |
| n_updates | 17499 |
-----------------------------------------------
-----------------------------------------------*******--------------------------------------------------------| 43%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 86.80206 |
| air_temperature | 22.288834 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 16.0 |
| month | 6.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | 17.761728 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 4.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 922 |
| n_updates | 17524 |
-----------------------------------------------
-----------------------------------------------*******--------------------------------------------------------| 43%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 89.78475 |
| air_temperature | 21.46684 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 20.0 |
| month | 6.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 14.061084 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 215.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.62e+03 |
| n_updates | 17549 |
-----------------------------------------------
-----------------------------------------------*******--------------------------------------------------------| 43%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 81.15778 |
| air_temperature | 23.580736 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 19.0 |
| month | 6.0 |
| outdoor_humidity | 81.75 |
| outdoor_temperature | 17.122057 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 322.5 |
| wind_speed | 1.575 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.25e+03 |
| n_updates | 17574 |
-----------------------------------------------
----------------------------------------------*********-------------------------------------------------------| 44%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 88.29978 |
| air_temperature | 22.31859 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 20.0 |
| direct_solar_radiation | 40.25 |
| hour | 4.0 |
| htg_setpoint | 16.0 |
| month | 6.0 |
| outdoor_humidity | 54.5 |
| outdoor_temperature | 14.69055 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 10.0 |
| wind_speed | 5.05 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 940 |
| n_updates | 17599 |
----------------------------------------------
-----------------------------------------------********-------------------------------------------------------| 44%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 93.26164 |
| air_temperature | 21.451311 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 94.25 |
| direct_solar_radiation | 98.75 |
| hour | 5.0 |
| htg_setpoint | 18.0 |
| month | 6.0 |
| outdoor_humidity | 73.5 |
| outdoor_temperature | 15.18072 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 42.5 |
| wind_speed | 2.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 857 |
| n_updates | 17624 |
-----------------------------------------------
-----------------------------------------------********-------------------------------------------------------| 44%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 76.971344 |
| air_temperature | 21.424046 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 168.5 |
| direct_solar_radiation | 91.0 |
| hour | 6.0 |
| htg_setpoint | 15.0 |
| month | 6.0 |
| outdoor_humidity | 87.75 |
| outdoor_temperature | 18.039125 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 130.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.46e+03 |
| n_updates | 17649 |
-----------------------------------------------
-----------------------------------------------********-------------------------------------------------------| 44%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1732.2148 |
| air_humidity | 72.68931 |
| air_temperature | 21.707315 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 152.0 |
| direct_solar_radiation | 0.0 |
| hour | 7.0 |
| htg_setpoint | 22.0 |
| month | 6.0 |
| outdoor_humidity | 90.75 |
| outdoor_temperature | 19.883415 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1558993.4 |
| wind_direction | 195.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.28e+03 |
| n_updates | 17674 |
-----------------------------------------------
-----------------------------------------------*********------------------------------------------------------| 45%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 1228.1124 |
| air_humidity | 69.9077 |
| air_temperature | 22.623905 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 165.25 |
| direct_solar_radiation | 0.0 |
| hour | 8.0 |
| htg_setpoint | 18.0 |
| month | 6.0 |
| outdoor_humidity | 90.0 |
| outdoor_temperature | 18.969051 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1105301.1 |
| wind_direction | 192.5 |
| wind_speed | 3.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 517 |
| n_updates | 17699 |
-----------------------------------------------
-----------------------------------------------*********------------------------------------------------------| 45%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 4790.518 |
| air_humidity | 62.532368 |
| air_temperature | 22.88602 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 280.75 |
| direct_solar_radiation | 574.25 |
| hour | 9.0 |
| htg_setpoint | 17.0 |
| month | 6.0 |
| outdoor_humidity | 84.75 |
| outdoor_temperature | 21.947834 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 4281177.5 |
| wind_direction | 192.5 |
| wind_speed | 5.05 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.6e+03 |
| n_updates | 17724 |
-----------------------------------------------
-----------------------------------------------*********------------------------------------------------------| 45%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 73.33571 |
| air_temperature | 26.042614 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 299.0 |
| direct_solar_radiation | 563.75 |
| hour | 10.0 |
| htg_setpoint | 17.0 |
| month | 6.0 |
| outdoor_humidity | 68.75 |
| outdoor_temperature | 27.839651 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 217.5 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.9e+03 |
| n_updates | 17749 |
-----------------------------------------------
-----------------------------------------------**********-----------------------------------------------------| 46%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 83.68221 |
| air_temperature | 23.874613 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 272.75 |
| direct_solar_radiation | 136.25 |
| hour | 11.0 |
| htg_setpoint | 17.0 |
| month | 6.0 |
| outdoor_humidity | 79.0 |
| outdoor_temperature | 24.195335 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 115.0 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.52e+03 |
| n_updates | 17774 |
-----------------------------------------------
-----------------------------------------------**********-----------------------------------------------------| 46%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 5723.8325 |
| air_humidity | 59.568176 |
| air_temperature | 23.107014 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 259.75 |
| direct_solar_radiation | 706.5 |
| hour | 12.0 |
| htg_setpoint | 21.0 |
| month | 6.0 |
| outdoor_humidity | 75.5 |
| outdoor_temperature | 23.755472 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 5151449.5 |
| wind_direction | 195.0 |
| wind_speed | 4.675 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 452 |
| n_updates | 17799 |
-----------------------------------------------
-----------------------------------------------**********-----------------------------------------------------| 46%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 6552.195 |
| air_humidity | 58.304203 |
| air_temperature | 23.97524 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 169.75 |
| direct_solar_radiation | 637.5 |
| hour | 13.0 |
| htg_setpoint | 15.0 |
| month | 6.0 |
| outdoor_humidity | 71.75 |
| outdoor_temperature | 27.065159 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 5884490.0 |
| wind_direction | 197.5 |
| wind_speed | 5.3 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.65e+03 |
| n_updates | 17824 |
-----------------------------------------------
-----------------------------------------------**********-----------------------------------------------------| 46%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 7836.0625 |
| air_humidity | 56.81204 |
| air_temperature | 24.587852 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 381.0 |
| direct_solar_radiation | 266.0 |
| hour | 14.0 |
| htg_setpoint | 20.0 |
| month | 6.0 |
| outdoor_humidity | 42.5 |
| outdoor_temperature | 32.049313 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 7052456.5 |
| wind_direction | 0.0 |
| wind_speed | 5.675 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.44e+03 |
| n_updates | 17849 |
-----------------------------------------------
-----------------------------------------------***********----------------------------------------------------| 47%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 4793.1064 |
| air_humidity | 54.42562 |
| air_temperature | 24.286098 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 216.0 |
| direct_solar_radiation | 511.0 |
| hour | 15.0 |
| htg_setpoint | 21.0 |
| month | 6.0 |
| outdoor_humidity | 40.75 |
| outdoor_temperature | 25.641277 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 4312496.0 |
| wind_direction | 150.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.22e+03 |
| n_updates | 17874 |
-----------------------------------------------
-----------------------------------------------***********----------------------------------------------------| 47%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 7375.4526 |
| air_humidity | 61.23719 |
| air_temperature | 23.157116 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 121.5 |
| direct_solar_radiation | 495.75 |
| hour | 16.0 |
| htg_setpoint | 22.0 |
| month | 6.0 |
| outdoor_humidity | 90.25 |
| outdoor_temperature | 23.998201 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 6626954.5 |
| wind_direction | 190.0 |
| wind_speed | 5.325 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.98e+03 |
| n_updates | 17899 |
-----------------------------------------------
-----------------------------------------------***********----------------------------------------------------| 47%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 59.19129 |
| air_temperature | 29.05981 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 105.5 |
| direct_solar_radiation | 315.5 |
| hour | 17.0 |
| htg_setpoint | 22.0 |
| month | 6.0 |
| outdoor_humidity | 30.75 |
| outdoor_temperature | 28.943462 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 305.0 |
| wind_speed | 6.575 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.31e+03 |
| n_updates | 17924 |
-----------------------------------------------
-----------------------------------------------***********----------------------------------------------------| 47%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 60.36356 |
| air_temperature | 28.721018 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 52.0 |
| direct_solar_radiation | 52.5 |
| hour | 18.0 |
| htg_setpoint | 15.0 |
| month | 6.0 |
| outdoor_humidity | 81.5 |
| outdoor_temperature | 24.795532 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 180.0 |
| wind_speed | 5.825 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.29e+03 |
| n_updates | 17949 |
-----------------------------------------------
-----------------------------------------------************---------------------------------------------------| 48%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 63.818764 |
| air_temperature | 28.084414 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 22.0 |
| month | 6.0 |
| outdoor_humidity | 97.75 |
| outdoor_temperature | 24.247278 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 85.0 |
| wind_speed | 5.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.63e+03 |
| n_updates | 17974 |
-----------------------------------------------
-----------------------------------------------************---------------------------------------------------| 48%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 64.21806 |
| air_temperature | 27.403837 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 18.0 |
| month | 6.0 |
| outdoor_humidity | 50.5 |
| outdoor_temperature | 28.683372 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 272.5 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.69e+03 |
| n_updates | 17999 |
-----------------------------------------------
-----------------------------------------------************---------------------------------------------------| 48%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 68.10296 |
| air_temperature | 26.301935 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 22.0 |
| month | 6.0 |
| outdoor_humidity | 79.0 |
| outdoor_temperature | 21.085833 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 7.325 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.41e+03 |
| n_updates | 18024 |
-----------------------------------------------
-----------------------------------------------*************--------------------------------------------------| 49%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 79.1191 |
| air_temperature | 24.227379 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 22.0 |
| month | 6.0 |
| outdoor_humidity | 86.25 |
| outdoor_temperature | 20.546297 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 200.0 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.58e+03 |
| n_updates | 18049 |
-----------------------------------------------
-----------------------------------------------*************--------------------------------------------------| 49%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 75.33137 |
| air_temperature | 23.995317 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 19.0 |
| month | 6.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | 21.382294 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 200.0 |
| wind_speed | 4.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.79e+03 |
| n_updates | 18074 |
-----------------------------------------------
-----------------------------------------------*************--------------------------------------------------| 49%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 78.265434 |
| air_temperature | 23.291105 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 21.0 |
| month | 6.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 20.631296 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 200.0 |
| wind_speed | 5.3 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.06e+04 |
| n_updates | 18099 |
-----------------------------------------------
-----------------------------------------------**************-------------------------------------------------| 50%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 83.593895 |
| air_temperature | 22.290453 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 20.0 |
| month | 7.0 |
| outdoor_humidity | 96.25 |
| outdoor_temperature | 18.960316 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 230.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.9e+03 |
| n_updates | 18124 |
-----------------------------------------------
-----------------------------------------------**************-------------------------------------------------| 50%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 85.49853 |
| air_temperature | 22.546316 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 19.0 |
| month | 7.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 17.489908 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.57e+03 |
| n_updates | 18149 |
-----------------------------------------------
-----------------------------------------------**************-------------------------------------------------| 50%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 85.61108 |
| air_temperature | 22.441803 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 15.0 |
| month | 7.0 |
| outdoor_humidity | 88.0 |
| outdoor_temperature | 20.486403 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 237.5 |
| wind_speed | 2.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.51e+03 |
| n_updates | 18174 |
-----------------------------------------------
-----------------------------------------------**************-------------------------------------------------| 50%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 91.05344 |
| air_temperature | 22.388695 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 17.0 |
| direct_solar_radiation | 1.75 |
| hour | 4.0 |
| htg_setpoint | 19.0 |
| month | 7.0 |
| outdoor_humidity | 95.25 |
| outdoor_temperature | 19.99402 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 50.0 |
| wind_speed | 3.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.31e+03 |
| n_updates | 18199 |
-----------------------------------------------
-----------------------------------------------***************------------------------------------------------| 51%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 90.21326 |
| air_temperature | 22.180178 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 42.25 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 19.0 |
| month | 7.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 20.440664 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 67.5 |
| wind_speed | 2.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.93e+03 |
| n_updates | 18224 |
-----------------------------------------------
-----------------------------------------------***************------------------------------------------------| 51%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 83.37489 |
| air_temperature | 23.224543 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 110.0 |
| direct_solar_radiation | 160.0 |
| hour | 6.0 |
| htg_setpoint | 22.0 |
| month | 7.0 |
| outdoor_humidity | 88.75 |
| outdoor_temperature | 23.39646 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 232.5 |
| wind_speed | 4.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.86e+03 |
| n_updates | 18249 |
-----------------------------------------------
-----------------------------------------------***************------------------------------------------------| 51%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 76.447105 |
| air_temperature | 24.64745 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 173.0 |
| direct_solar_radiation | 0.0 |
| hour | 7.0 |
| htg_setpoint | 17.0 |
| month | 7.0 |
| outdoor_humidity | 72.75 |
| outdoor_temperature | 25.151327 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 242.5 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.17e+04 |
| n_updates | 18274 |
-----------------------------------------------
-----------------------------------------------***************------------------------------------------------| 51%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 8526.9795 |
| air_humidity | 64.30461 |
| air_temperature | 22.684814 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 288.25 |
| direct_solar_radiation | 412.5 |
| hour | 8.0 |
| htg_setpoint | 22.0 |
| month | 7.0 |
| outdoor_humidity | 73.5 |
| outdoor_temperature | 27.592764 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 7762197.0 |
| wind_direction | 195.0 |
| wind_speed | 3.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.26e+03 |
| n_updates | 18299 |
-----------------------------------------------
-----------------------------------------------****************-----------------------------------------------| 52%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 8381.347 |
| air_humidity | 60.01121 |
| air_temperature | 23.661592 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 254.25 |
| direct_solar_radiation | 629.25 |
| hour | 9.0 |
| htg_setpoint | 21.0 |
| month | 7.0 |
| outdoor_humidity | 71.75 |
| outdoor_temperature | 27.747707 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 7516141.0 |
| wind_direction | 210.0 |
| wind_speed | 3.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.75e+03 |
| n_updates | 18324 |
-----------------------------------------------
-----------------------------------------------****************-----------------------------------------------| 52%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 6758.7915 |
| air_humidity | 58.00684 |
| air_temperature | 24.18974 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 317.5 |
| direct_solar_radiation | 556.75 |
| hour | 10.0 |
| htg_setpoint | 22.0 |
| month | 7.0 |
| outdoor_humidity | 39.75 |
| outdoor_temperature | 31.748314 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 6082912.5 |
| wind_direction | 285.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.81e+03 |
| n_updates | 18349 |
-----------------------------------------------
-----------------------------------------------****************-----------------------------------------------| 52%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 4673.956 |
| air_humidity | 56.21035 |
| air_temperature | 24.077019 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 282.25 |
| direct_solar_radiation | 664.75 |
| hour | 11.0 |
| htg_setpoint | 21.0 |
| month | 7.0 |
| outdoor_humidity | 41.0 |
| outdoor_temperature | 26.647907 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 4197338.5 |
| wind_direction | 0.0 |
| wind_speed | 4.75 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.61e+03 |
| n_updates | 18374 |
-----------------------------------------------
-----------------------------------------------*****************----------------------------------------------| 53%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 3924.4778 |
| air_humidity | 55.328617 |
| air_temperature | 24.44708 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 161.25 |
| direct_solar_radiation | 755.5 |
| hour | 12.0 |
| htg_setpoint | 19.0 |
| month | 7.0 |
| outdoor_humidity | 35.75 |
| outdoor_temperature | 29.854593 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 3535004.5 |
| wind_direction | 207.5 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 915 |
| n_updates | 18399 |
-----------------------------------------------
-----------------------------------------------*****************----------------------------------------------| 53%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 58.166363 |
| air_temperature | 28.55074 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 226.0 |
| direct_solar_radiation | 707.25 |
| hour | 13.0 |
| htg_setpoint | 22.0 |
| month | 7.0 |
| outdoor_humidity | 57.5 |
| outdoor_temperature | 30.628448 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 195.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.23e+03 |
| n_updates | 18424 |
-----------------------------------------------
-----------------------------------------------*****************----------------------------------------------| 53%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 60.52538 |
| air_temperature | 27.901205 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 273.0 |
| direct_solar_radiation | 0.0 |
| hour | 14.0 |
| htg_setpoint | 15.0 |
| month | 7.0 |
| outdoor_humidity | 67.75 |
| outdoor_temperature | 25.93718 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 120.0 |
| wind_speed | 6.325 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.89e+03 |
| n_updates | 18449 |
-----------------------------------------------
-----------------------------------------------*****************----------------------------------------------| 53%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 7710.064 |
| air_humidity | 59.231922 |
| air_temperature | 23.880999 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 190.25 |
| direct_solar_radiation | 343.0 |
| hour | 15.0 |
| htg_setpoint | 22.0 |
| month | 7.0 |
| outdoor_humidity | 63.0 |
| outdoor_temperature | 28.25258 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 6939057.5 |
| wind_direction | 235.0 |
| wind_speed | 1.65 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.2e+04 |
| n_updates | 18474 |
-----------------------------------------------
-----------------------------------------------******************---------------------------------------------| 54%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 6280.664 |
| air_humidity | 59.72886 |
| air_temperature | 23.7811 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 155.5 |
| direct_solar_radiation | 91.25 |
| hour | 16.0 |
| htg_setpoint | 18.0 |
| month | 7.0 |
| outdoor_humidity | 50.25 |
| outdoor_temperature | 29.278086 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 5652597.5 |
| wind_direction | 267.5 |
| wind_speed | 4.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 995 |
| n_updates | 18499 |
-----------------------------------------------
-----------------------------------------------******************---------------------------------------------| 54%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 48.54388 |
| air_temperature | 29.638643 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 103.25 |
| direct_solar_radiation | 62.5 |
| hour | 17.0 |
| htg_setpoint | 17.0 |
| month | 7.0 |
| outdoor_humidity | 55.25 |
| outdoor_temperature | 25.908215 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 182.5 |
| wind_speed | 4.375 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.09e+03 |
| n_updates | 18524 |
-----------------------------------------------
-----------------------------------------------******************---------------------------------------------| 54%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 56.875576 |
| air_temperature | 28.546131 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 36.5 |
| direct_solar_radiation | 21.75 |
| hour | 18.0 |
| htg_setpoint | 22.0 |
| month | 7.0 |
| outdoor_humidity | 78.25 |
| outdoor_temperature | 25.278723 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 140.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.83e+03 |
| n_updates | 18549 |
-----------------------------------------------
-----------------------------------------------******************---------------------------------------------| 54%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 58.24339 |
| air_temperature | 29.165983 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 22.0 |
| month | 7.0 |
| outdoor_humidity | 88.75 |
| outdoor_temperature | 24.60015 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 4.875 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.46e+03 |
| n_updates | 18574 |
-----------------------------------------------
-----------------------------------------------*******************--------------------------------------------| 55%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 66.87542 |
| air_temperature | 27.153479 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 20.0 |
| month | 7.0 |
| outdoor_humidity | 85.0 |
| outdoor_temperature | 24.488916 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 200.0 |
| wind_speed | 5.125 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.19e+03 |
| n_updates | 18599 |
-----------------------------------------------
-----------------------------------------------*******************--------------------------------------------| 55%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 68.727104 |
| air_temperature | 26.621859 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 20.0 |
| month | 7.0 |
| outdoor_humidity | 86.5 |
| outdoor_temperature | 24.772013 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 7.325 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.62e+03 |
| n_updates | 18624 |
-----------------------------------------------
-----------------------------------------------*******************--------------------------------------------| 55%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 74.13177 |
| air_temperature | 25.110699 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 20.0 |
| month | 7.0 |
| outdoor_humidity | 89.25 |
| outdoor_temperature | 22.868048 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 200.0 |
| wind_speed | 5.575 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 8.89e+03 |
| n_updates | 18649 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 56%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 83.009995 |
| air_temperature | 23.295828 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 18.0 |
| month | 7.0 |
| outdoor_humidity | 91.5 |
| outdoor_temperature | 20.944084 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 222.5 |
| wind_speed | 3.625 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.68e+03 |
| n_updates | 18674 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 56%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 76.22931 |
| air_temperature | 24.215412 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 15.0 |
| month | 7.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | 23.454714 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 210.0 |
| wind_speed | 2.725 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.08e+03 |
| n_updates | 18699 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 56%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 84.98991 |
| air_temperature | 23.21452 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 20.0 |
| month | 7.0 |
| outdoor_humidity | 89.25 |
| outdoor_temperature | 21.339176 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 235.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 778 |
| n_updates | 18724 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 57%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 84.72173 |
| air_temperature | 23.91344 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 18.0 |
| month | 7.0 |
| outdoor_humidity | 92.5 |
| outdoor_temperature | 23.348045 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 240.0 |
| wind_speed | 3.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.08e+03 |
| n_updates | 18749 |
-----------------------------------------------
----------------------------------------------*********************-------------------------------------------| 57%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 86.47173 |
| air_temperature | 23.57375 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 20.0 |
| month | 7.0 |
| outdoor_humidity | 97.0 |
| outdoor_temperature | 21.85287 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 202.5 |
| wind_speed | 5.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 648 |
| n_updates | 18774 |
----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 57%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 91.6411 |
| air_temperature | 22.740074 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 17.0 |
| month | 7.0 |
| outdoor_humidity | 81.0 |
| outdoor_temperature | 23.466516 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 302.5 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 7.83e+03 |
| n_updates | 18799 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 57%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 85.37062 |
| air_temperature | 22.634445 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 49.5 |
| direct_solar_radiation | 17.75 |
| hour | 5.0 |
| htg_setpoint | 15.0 |
| month | 7.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 22.287907 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 197.5 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 839 |
| n_updates | 18824 |
-----------------------------------------------
----------------------------------------------**********************------------------------------------------| 58%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 87.94491 |
| air_temperature | 22.73523 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 84.25 |
| direct_solar_radiation | 0.0 |
| hour | 6.0 |
| htg_setpoint | 22.0 |
| month | 7.0 |
| outdoor_humidity | 89.5 |
| outdoor_temperature | 24.26252 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 127.5 |
| wind_speed | 1.125 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.38e+03 |
| n_updates | 18849 |
----------------------------------------------
-----------------------------------------------*********************------------------------------------------| 58%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 2807.4397 |
| air_humidity | 74.32868 |
| air_temperature | 21.33207 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 129.0 |
| direct_solar_radiation | 596.75 |
| hour | 7.0 |
| htg_setpoint | 16.0 |
| month | 8.0 |
| outdoor_humidity | 67.0 |
| outdoor_temperature | 26.407139 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2526695.5 |
| wind_direction | 225.0 |
| wind_speed | 2.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.81e+03 |
| n_updates | 18874 |
-----------------------------------------------
-----------------------------------------------*********************------------------------------------------| 58%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 9054.869 |
| air_humidity | 65.859116 |
| air_temperature | 23.2239 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 113.0 |
| direct_solar_radiation | 751.25 |
| hour | 8.0 |
| htg_setpoint | 16.0 |
| month | 8.0 |
| outdoor_humidity | 71.0 |
| outdoor_temperature | 29.98417 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 8148379.0 |
| wind_direction | 207.5 |
| wind_speed | 2.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.4e+03 |
| n_updates | 18899 |
-----------------------------------------------
----------------------------------------------************************----------------------------------------| 59%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 69.58006 |
| air_temperature | 26.38312 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 272.25 |
| direct_solar_radiation | 387.0 |
| hour | 9.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 69.5 |
| outdoor_temperature | 28.69311 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 182.5 |
| wind_speed | 2.725 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.88e+03 |
| n_updates | 18924 |
----------------------------------------------
-----------------------------------------------***********************----------------------------------------| 59%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 67.058 |
| air_temperature | 26.914494 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 180.75 |
| direct_solar_radiation | 689.25 |
| hour | 10.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 69.75 |
| outdoor_temperature | 29.624235 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 177.5 |
| wind_speed | 3.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.57e+03 |
| n_updates | 18949 |
-----------------------------------------------
-----------------------------------------------***********************----------------------------------------| 59%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 8801.524 |
| air_humidity | 58.136875 |
| air_temperature | 24.039986 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 265.75 |
| direct_solar_radiation | 10.75 |
| hour | 11.0 |
| htg_setpoint | 22.0 |
| month | 8.0 |
| outdoor_humidity | 72.25 |
| outdoor_temperature | 28.023397 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 7921372.0 |
| wind_direction | 112.5 |
| wind_speed | 5.4 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.12e+03 |
| n_updates | 18974 |
-----------------------------------------------
-----------------------------------------------***********************----------------------------------------| 59%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 3378.667 |
| air_humidity | 58.892483 |
| air_temperature | 23.367899 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 257.0 |
| direct_solar_radiation | 0.0 |
| hour | 12.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 84.75 |
| outdoor_temperature | 21.247084 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 3040800.2 |
| wind_direction | 62.5 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.18e+03 |
| n_updates | 18999 |
-----------------------------------------------
-----------------------------------------------************************---------------------------------------| 60%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 4014.445 |
| air_humidity | 57.45482 |
| air_temperature | 23.92956 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 368.0 |
| direct_solar_radiation | 307.25 |
| hour | 13.0 |
| htg_setpoint | 20.0 |
| month | 8.0 |
| outdoor_humidity | 49.75 |
| outdoor_temperature | 23.911158 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 3613000.5 |
| wind_direction | 65.0 |
| wind_speed | 5.325 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.18e+03 |
| n_updates | 19024 |
-----------------------------------------------
-----------------------------------------------************************---------------------------------------| 60%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 4817.473 |
| air_humidity | 52.57281 |
| air_temperature | 24.286257 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 237.75 |
| direct_solar_radiation | 550.0 |
| hour | 14.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 39.5 |
| outdoor_temperature | 26.177536 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 4331393.5 |
| wind_direction | 132.5 |
| wind_speed | 4.925 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.34e+03 |
| n_updates | 19049 |
-----------------------------------------------
-----------------------------------------------************************---------------------------------------| 60%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 5711.878 |
| air_humidity | 57.63123 |
| air_temperature | 24.354006 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 239.0 |
| direct_solar_radiation | 424.25 |
| hour | 15.0 |
| htg_setpoint | 20.0 |
| month | 8.0 |
| outdoor_humidity | 51.5 |
| outdoor_temperature | 26.314344 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 5136858.0 |
| wind_direction | 197.5 |
| wind_speed | 4.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 395 |
| n_updates | 19074 |
-----------------------------------------------
-----------------------------------------------************************---------------------------------------| 60%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 58.167454 |
| air_temperature | 28.583328 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 68.25 |
| direct_solar_radiation | 670.75 |
| hour | 16.0 |
| htg_setpoint | 22.0 |
| month | 8.0 |
| outdoor_humidity | 67.25 |
| outdoor_temperature | 26.150335 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 180.0 |
| wind_speed | 5.3 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.25e+03 |
| n_updates | 19099 |
-----------------------------------------------
-----------------------------------------------*************************--------------------------------------| 61%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 55.369625 |
| air_temperature | 29.384346 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 82.75 |
| direct_solar_radiation | 102.75 |
| hour | 17.0 |
| htg_setpoint | 17.0 |
| month | 8.0 |
| outdoor_humidity | 78.25 |
| outdoor_temperature | 25.75643 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 185.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 9.68e+03 |
| n_updates | 19124 |
-----------------------------------------------
-----------------------------------------------*************************--------------------------------------| 61%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 51.58527 |
| air_temperature | 29.717571 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 18.75 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 18.0 |
| month | 8.0 |
| outdoor_humidity | 60.0 |
| outdoor_temperature | 31.05684 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 260.0 |
| wind_speed | 5.425 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.17e+03 |
| n_updates | 19149 |
-----------------------------------------------
-----------------------------------------------*************************--------------------------------------| 61%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 57.148495 |
| air_temperature | 29.580566 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 22.0 |
| month | 8.0 |
| outdoor_humidity | 73.75 |
| outdoor_temperature | 26.079569 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 200.0 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 496 |
| n_updates | 19174 |
-----------------------------------------------
-----------------------------------------------**************************-------------------------------------| 62%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 66.282104 |
| air_temperature | 27.809978 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 74.0 |
| outdoor_temperature | 24.78124 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 180.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 7.12e+03 |
| n_updates | 19199 |
-----------------------------------------------
-----------------------------------------------**************************-------------------------------------| 62%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 64.98033 |
| air_temperature | 26.815897 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 83.75 |
| outdoor_temperature | 23.301914 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 105.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.78e+03 |
| n_updates | 19224 |
-----------------------------------------------
-----------------------------------------------**************************-------------------------------------| 62%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 80.27217 |
| air_temperature | 26.22684 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 94.0 |
| outdoor_temperature | 26.958303 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 102.5 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.37e+03 |
| n_updates | 19249 |
-----------------------------------------------
-----------------------------------------------**************************-------------------------------------| 62%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 81.57633 |
| air_temperature | 25.920828 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 20.0 |
| month | 8.0 |
| outdoor_humidity | 55.5 |
| outdoor_temperature | 26.734919 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 22.5 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4e+03 |
| n_updates | 19274 |
-----------------------------------------------
-----------------------------------------------***************************------------------------------------| 63%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 84.304825 |
| air_temperature | 25.465914 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 57.5 |
| outdoor_temperature | 22.813532 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 45.0 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.07e+03 |
| n_updates | 19299 |
-----------------------------------------------
-----------------------------------------------***************************------------------------------------| 63%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 78.35207 |
| air_temperature | 23.392422 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 73.75 |
| outdoor_temperature | 17.851112 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 52.5 |
| wind_speed | 2.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 7.42e+03 |
| n_updates | 19324 |
-----------------------------------------------
-----------------------------------------------***************************------------------------------------| 63%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 77.09457 |
| air_temperature | 23.109562 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 68.0 |
| outdoor_temperature | 21.00783 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 232.5 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.54e+03 |
| n_updates | 19349 |
-----------------------------------------------
-----------------------------------------------****************************-----------------------------------| 64%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 79.33025 |
| air_temperature | 23.843977 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 17.0 |
| month | 8.0 |
| outdoor_humidity | 43.5 |
| outdoor_temperature | 26.288761 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 312.5 |
| wind_speed | 3.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.09e+03 |
| n_updates | 19374 |
-----------------------------------------------
-----------------------------------------------****************************-----------------------------------| 64%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 75.405334 |
| air_temperature | 22.821547 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 19.0 |
| month | 8.0 |
| outdoor_humidity | 61.5 |
| outdoor_temperature | 18.559614 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 0.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.26e+03 |
| n_updates | 19399 |
-----------------------------------------------
-----------------------------------------------****************************-----------------------------------| 64%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 84.77596 |
| air_temperature | 23.128113 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 31.5 |
| direct_solar_radiation | 52.0 |
| hour | 5.0 |
| htg_setpoint | 20.0 |
| month | 8.0 |
| outdoor_humidity | 73.75 |
| outdoor_temperature | 20.81059 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 250.0 |
| wind_speed | 5.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.75e+03 |
| n_updates | 19424 |
-----------------------------------------------
-----------------------------------------------*****************************----------------------------------| 65%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 82.58572 |
| air_temperature | 23.639242 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 84.75 |
| direct_solar_radiation | 314.25 |
| hour | 6.0 |
| htg_setpoint | 18.0 |
| month | 8.0 |
| outdoor_humidity | 52.5 |
| outdoor_temperature | 20.048075 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 15.0 |
| wind_speed | 4.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.97e+03 |
| n_updates | 19449 |
-----------------------------------------------
-----------------------------------------------*****************************----------------------------------| 65%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1617.4988 |
| air_humidity | 79.90342 |
| air_temperature | 20.43247 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 139.75 |
| direct_solar_radiation | 442.0 |
| hour | 7.0 |
| htg_setpoint | 16.0 |
| month | 8.0 |
| outdoor_humidity | 60.0 |
| outdoor_temperature | 22.065544 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1478523.6 |
| wind_direction | 52.5 |
| wind_speed | 2.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.59e+03 |
| n_updates | 19474 |
-----------------------------------------------
-----------------------------------------------*****************************----------------------------------| 65%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 5886.132 |
| air_humidity | 66.80615 |
| air_temperature | 22.063503 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 180.25 |
| direct_solar_radiation | 508.5 |
| hour | 8.0 |
| htg_setpoint | 16.0 |
| month | 8.0 |
| outdoor_humidity | 81.0 |
| outdoor_temperature | 24.354967 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 5328320.5 |
| wind_direction | 232.5 |
| wind_speed | 5.325 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.15e+03 |
| n_updates | 19499 |
-----------------------------------------------
-----------------------------------------------*****************************----------------------------------| 65%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 3672.857 |
| air_humidity | 59.752323 |
| air_temperature | 23.272907 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 187.0 |
| direct_solar_radiation | 603.0 |
| hour | 9.0 |
| htg_setpoint | 18.0 |
| month | 8.0 |
| outdoor_humidity | 43.5 |
| outdoor_temperature | 24.80805 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 3281666.5 |
| wind_direction | 97.5 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.52e+03 |
| n_updates | 19524 |
-----------------------------------------------
-----------------------------------------------******************************---------------------------------| 66%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 4737.7534 |
| air_humidity | 58.999786 |
| air_temperature | 23.746513 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 222.5 |
| direct_solar_radiation | 627.75 |
| hour | 10.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 57.5 |
| outdoor_temperature | 24.401014 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 4263978.0 |
| wind_direction | 227.5 |
| wind_speed | 4.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.85e+03 |
| n_updates | 19549 |
-----------------------------------------------
-----------------------------------------------******************************---------------------------------| 66%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 4875.718 |
| air_humidity | 44.244587 |
| air_temperature | 24.019958 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 180.0 |
| direct_solar_radiation | 788.5 |
| hour | 11.0 |
| htg_setpoint | 20.0 |
| month | 8.0 |
| outdoor_humidity | 26.25 |
| outdoor_temperature | 26.552404 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 4371530.0 |
| wind_direction | 5.0 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.83e+03 |
| n_updates | 19574 |
-----------------------------------------------
-----------------------------------------------******************************---------------------------------| 66%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 45.898952 |
| air_temperature | 26.009037 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 127.75 |
| direct_solar_radiation | 850.75 |
| hour | 12.0 |
| htg_setpoint | 19.0 |
| month | 8.0 |
| outdoor_humidity | 70.5 |
| outdoor_temperature | 26.36101 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 200.0 |
| wind_speed | 5.8 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 7.82e+03 |
| n_updates | 19599 |
-----------------------------------------------
-----------------------------------------------******************************---------------------------------| 66%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 41.81277 |
| air_temperature | 27.505688 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 186.5 |
| direct_solar_radiation | 669.0 |
| hour | 13.0 |
| htg_setpoint | 19.0 |
| month | 9.0 |
| outdoor_humidity | 25.5 |
| outdoor_temperature | 29.788122 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 335.0 |
| wind_speed | 4.875 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.48e+03 |
| n_updates | 19624 |
-----------------------------------------------
-----------------------------------------------*******************************--------------------------------| 67%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 4886.1143 |
| air_humidity | 57.88376 |
| air_temperature | 23.773052 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 199.5 |
| direct_solar_radiation | 361.25 |
| hour | 14.0 |
| htg_setpoint | 22.0 |
| month | 9.0 |
| outdoor_humidity | 56.75 |
| outdoor_temperature | 24.97054 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 4363027.0 |
| wind_direction | 130.0 |
| wind_speed | 4.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.29e+03 |
| n_updates | 19649 |
-----------------------------------------------
-----------------------------------------------*******************************--------------------------------| 67%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 4029.3594 |
| air_humidity | 46.99753 |
| air_temperature | 24.343311 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 173.25 |
| direct_solar_radiation | 387.0 |
| hour | 15.0 |
| htg_setpoint | 16.0 |
| month | 9.0 |
| outdoor_humidity | 42.25 |
| outdoor_temperature | 22.448847 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 3626423.5 |
| wind_direction | 195.0 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.33e+03 |
| n_updates | 19674 |
-----------------------------------------------
-----------------------------------------------*******************************--------------------------------| 67%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 5349.853 |
| air_humidity | 58.909237 |
| air_temperature | 24.063595 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 93.5 |
| direct_solar_radiation | 335.5 |
| hour | 16.0 |
| htg_setpoint | 19.0 |
| month | 9.0 |
| outdoor_humidity | 57.5 |
| outdoor_temperature | 24.990084 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 4814867.5 |
| wind_direction | 190.0 |
| wind_speed | 6.075 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.78e+03 |
| n_updates | 19699 |
-----------------------------------------------
-----------------------------------------------********************************-------------------------------| 68%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 49.822647 |
| air_temperature | 29.09369 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 42.25 |
| direct_solar_radiation | 105.75 |
| hour | 17.0 |
| htg_setpoint | 21.0 |
| month | 9.0 |
| outdoor_humidity | 75.5 |
| outdoor_temperature | 23.82931 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 197.5 |
| wind_speed | 7.075 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 829 |
| n_updates | 19724 |
-----------------------------------------------
-----------------------------------------------********************************-------------------------------| 68%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 50.729893 |
| air_temperature | 30.093975 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 20.0 |
| month | 9.0 |
| outdoor_humidity | 79.75 |
| outdoor_temperature | 24.931477 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 175.0 |
| wind_speed | 4.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.61e+03 |
| n_updates | 19749 |
-----------------------------------------------
-----------------------------------------------********************************-------------------------------| 68%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 65.69394 |
| air_temperature | 26.956028 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 21.0 |
| month | 9.0 |
| outdoor_humidity | 82.75 |
| outdoor_temperature | 22.900484 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 192.5 |
| wind_speed | 7.825 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.27e+03 |
| n_updates | 19774 |
-----------------------------------------------
-----------------------------------------------********************************-------------------------------| 68%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 78.78566 |
| air_temperature | 23.96756 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 22.0 |
| month | 9.0 |
| outdoor_humidity | 76.5 |
| outdoor_temperature | 18.051031 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 60.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.14e+03 |
| n_updates | 19799 |
-----------------------------------------------
-----------------------------------------------*********************************------------------------------| 69%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 71.47217 |
| air_temperature | 24.990147 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 18.0 |
| month | 9.0 |
| outdoor_humidity | 87.75 |
| outdoor_temperature | 20.638704 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 62.5 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.32e+03 |
| n_updates | 19824 |
-----------------------------------------------
-----------------------------------------------*********************************------------------------------| 69%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 60.647545 |
| air_temperature | 24.419151 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 18.0 |
| month | 9.0 |
| outdoor_humidity | 45.5 |
| outdoor_temperature | 15.977511 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 20.0 |
| wind_speed | 3.725 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.29e+03 |
| n_updates | 19849 |
-----------------------------------------------
-----------------------------------------------*********************************------------------------------| 69%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 53.864487 |
| air_temperature | 23.427107 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 18.0 |
| month | 9.0 |
| outdoor_humidity | 64.0 |
| outdoor_temperature | 17.705479 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 255.0 |
| wind_speed | 3.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.49e+03 |
| n_updates | 19874 |
-----------------------------------------------
-----------------------------------------------**********************************-----------------------------| 70%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 77.79724 |
| air_temperature | 23.55326 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 18.0 |
| month | 9.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | 21.224926 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 212.5 |
| wind_speed | 5.575 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.07e+03 |
| n_updates | 19899 |
-----------------------------------------------
-----------------------------------------------**********************************-----------------------------| 70%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 80.70181 |
| air_temperature | 23.83317 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 17.0 |
| month | 9.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 21.874525 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 215.0 |
| wind_speed | 4.875 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 7.11e+03 |
| n_updates | 19924 |
-----------------------------------------------
-----------------------------------------------**********************************-----------------------------| 70%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 81.30007 |
| air_temperature | 23.849401 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 21.0 |
| month | 9.0 |
| outdoor_humidity | 63.0 |
| outdoor_temperature | 18.547737 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 10.0 |
| wind_speed | 4.925 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.04e+03 |
| n_updates | 19949 |
-----------------------------------------------
-----------------------------------------------***********************************----------------------------| 71%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 92.61786 |
| air_temperature | 21.799852 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 20.0 |
| month | 9.0 |
| outdoor_humidity | 72.0 |
| outdoor_temperature | 13.870208 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 60.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.82e+03 |
| n_updates | 19974 |
-----------------------------------------------
-----------------------------------------------***********************************----------------------------| 71%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 87.17677 |
| air_temperature | 22.119612 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 21.0 |
| month | 9.0 |
| outdoor_humidity | 88.5 |
| outdoor_temperature | 20.816065 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 137.5 |
| wind_speed | 4.125 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.47e+03 |
| n_updates | 19999 |
-----------------------------------------------
-----------------------------------------------***********************************----------------------------| 71%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 93.015495 |
| air_temperature | 21.31066 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 13.5 |
| direct_solar_radiation | 20.0 |
| hour | 5.0 |
| htg_setpoint | 21.0 |
| month | 9.0 |
| outdoor_humidity | 84.75 |
| outdoor_temperature | 18.328482 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 352.5 |
| wind_speed | 3.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.36e+03 |
| n_updates | 20024 |
-----------------------------------------------
-----------------------------------------------***********************************----------------------------| 71%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 84.57495 |
| air_temperature | 21.005348 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 61.0 |
| direct_solar_radiation | 262.0 |
| hour | 6.0 |
| htg_setpoint | 18.0 |
| month | 9.0 |
| outdoor_humidity | 62.5 |
| outdoor_temperature | 13.333364 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 25.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.48e+03 |
| n_updates | 20049 |
-----------------------------------------------
-----------------------------------------------************************************---------------------------| 72%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 301.6228 |
| air_humidity | 79.943886 |
| air_temperature | 20.113867 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 92.5 |
| direct_solar_radiation | 310.25 |
| hour | 7.0 |
| htg_setpoint | 15.0 |
| month | 9.0 |
| outdoor_humidity | 74.75 |
| outdoor_temperature | 16.825045 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 271460.53 |
| wind_direction | 67.5 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.59e+03 |
| n_updates | 20074 |
-----------------------------------------------
-----------------------------------------------************************************---------------------------| 72%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 86.96002 |
| air_temperature | 22.580376 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 144.25 |
| direct_solar_radiation | 0.0 |
| hour | 8.0 |
| htg_setpoint | 19.0 |
| month | 9.0 |
| outdoor_humidity | 78.75 |
| outdoor_temperature | 21.745192 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 227.5 |
| wind_speed | 4.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.96e+03 |
| n_updates | 20099 |
-----------------------------------------------
-----------------------------------------------************************************---------------------------| 72%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 81.88721 |
| air_temperature | 23.508745 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 170.75 |
| direct_solar_radiation | 0.0 |
| hour | 9.0 |
| htg_setpoint | 15.0 |
| month | 9.0 |
| outdoor_humidity | 91.5 |
| outdoor_temperature | 23.018007 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 192.5 |
| wind_speed | 4.5 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.54e+03 |
| n_updates | 20124 |
-----------------------------------------------
-----------------------------------------------************************************---------------------------| 72%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 344.5578 |
| air_humidity | 30.767315 |
| air_temperature | 24.99779 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 271.25 |
| direct_solar_radiation | 0.0 |
| hour | 10.0 |
| htg_setpoint | 20.0 |
| month | 9.0 |
| outdoor_humidity | 41.25 |
| outdoor_temperature | 14.921902 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 309548.22 |
| wind_direction | 5.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6e+03 |
| n_updates | 20149 |
-----------------------------------------------
-----------------------------------------------*************************************--------------------------| 73%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 2278.3 |
| air_humidity | 39.205063 |
| air_temperature | 24.005941 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 276.75 |
| direct_solar_radiation | 358.75 |
| hour | 11.0 |
| htg_setpoint | 21.0 |
| month | 9.0 |
| outdoor_humidity | 47.0 |
| outdoor_temperature | 18.965593 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 2050470.0 |
| wind_direction | 65.0 |
| wind_speed | 2.725 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.22e+03 |
| n_updates | 20174 |
-----------------------------------------------
-----------------------------------------------*************************************--------------------------| 73%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 1998.3851 |
| air_humidity | 52.785255 |
| air_temperature | 24.266527 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 219.0 |
| direct_solar_radiation | 202.75 |
| hour | 12.0 |
| htg_setpoint | 15.0 |
| month | 9.0 |
| outdoor_humidity | 63.75 |
| outdoor_temperature | 20.165852 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 1794946.1 |
| wind_direction | 122.5 |
| wind_speed | 4.75 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.81e+03 |
| n_updates | 20199 |
-----------------------------------------------
-----------------------------------------------*************************************--------------------------| 73%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1957.5281 |
| air_humidity | 55.09818 |
| air_temperature | 23.998634 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 179.5 |
| direct_solar_radiation | 0.0 |
| hour | 13.0 |
| htg_setpoint | 21.0 |
| month | 9.0 |
| outdoor_humidity | 75.5 |
| outdoor_temperature | 18.801235 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 1761775.2 |
| wind_direction | 67.5 |
| wind_speed | 2.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.35e+03 |
| n_updates | 20224 |
-----------------------------------------------
-----------------------------------------------**************************************-------------------------| 74%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 4070.7903 |
| air_humidity | 57.882877 |
| air_temperature | 23.809664 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 96.5 |
| direct_solar_radiation | 738.25 |
| hour | 14.0 |
| htg_setpoint | 22.0 |
| month | 9.0 |
| outdoor_humidity | 55.5 |
| outdoor_temperature | 22.709478 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 3636545.2 |
| wind_direction | 230.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.63e+03 |
| n_updates | 20249 |
-----------------------------------------------
-----------------------------------------------**************************************-------------------------| 74%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 68.24881 |
| air_temperature | 26.860281 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 74.25 |
| direct_solar_radiation | 600.0 |
| hour | 15.0 |
| htg_setpoint | 16.0 |
| month | 9.0 |
| outdoor_humidity | 24.5 |
| outdoor_temperature | 22.27375 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 15.0 |
| wind_speed | 4.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 8.31e+03 |
| n_updates | 20274 |
-----------------------------------------------
-----------------------------------------------**************************************-------------------------| 74%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 71.049194 |
| air_temperature | 26.28117 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 48.0 |
| direct_solar_radiation | 299.75 |
| hour | 16.0 |
| htg_setpoint | 20.0 |
| month | 9.0 |
| outdoor_humidity | 54.5 |
| outdoor_temperature | 18.562544 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 120.0 |
| wind_speed | 4.75 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.42e+03 |
| n_updates | 20299 |
-----------------------------------------------
-----------------------------------------------**************************************-------------------------| 74%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 38.25449 |
| air_temperature | 30.236038 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 22.0 |
| month | 9.0 |
| outdoor_humidity | 65.5 |
| outdoor_temperature | 17.029285 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 150.0 |
| wind_speed | 2.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 9.14e+03 |
| n_updates | 20324 |
-----------------------------------------------
-----------------------------------------------***************************************------------------------| 75%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 325.93085 |
| air_humidity | 38.41647 |
| air_temperature | 22.50031 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 21.0 |
| month | 10.0 |
| outdoor_humidity | 66.75 |
| outdoor_temperature | 12.274445 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 293337.75 |
| wind_direction | 292.5 |
| wind_speed | 1.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.07e+03 |
| n_updates | 20349 |
-----------------------------------------------
-----------------------------------------------***************************************------------------------| 75%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 72.12592 |
| air_humidity | 45.341778 |
| air_temperature | 25.65021 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 18.0 |
| month | 10.0 |
| outdoor_humidity | 79.5 |
| outdoor_temperature | 15.496785 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 64778.598 |
| wind_direction | 255.0 |
| wind_speed | 1.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.51e+03 |
| n_updates | 20374 |
-----------------------------------------------
-----------------------------------------------***************************************------------------------| 75%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 63.414883 |
| air_temperature | 28.577398 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 20.0 |
| month | 10.0 |
| outdoor_humidity | 82.5 |
| outdoor_temperature | 19.600908 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 210.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 948 |
| n_updates | 20399 |
-----------------------------------------------
-----------------------------------------------***************************************------------------------| 75%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 61.78261 |
| air_temperature | 22.69856 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 20.0 |
| month | 10.0 |
| outdoor_humidity | 89.25 |
| outdoor_temperature | 16.416159 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 210.0 |
| wind_speed | 1.9 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.32e+04 |
| n_updates | 20424 |
-----------------------------------------------
-----------------------------------------------****************************************-----------------------| 76%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 52.74851 |
| air_temperature | 25.398882 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 17.0 |
| month | 10.0 |
| outdoor_humidity | 91.5 |
| outdoor_temperature | 20.38683 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 180.0 |
| wind_speed | 8.525 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.64e+03 |
| n_updates | 20449 |
-----------------------------------------------
-----------------------------------------------****************************************-----------------------| 76%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 33.290733 |
| air_temperature | 21.287834 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 16.0 |
| month | 10.0 |
| outdoor_humidity | 50.5 |
| outdoor_temperature | 12.449082 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 295.0 |
| wind_speed | 7.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.15e+03 |
| n_updates | 20474 |
-----------------------------------------------
-----------------------------------------------*****************************************----------------------| 77%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 19.943989 |
| air_temperature | 18.339186 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 16.0 |
| month | 10.0 |
| outdoor_humidity | 46.75 |
| outdoor_temperature | 5.152613 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 287.5 |
| wind_speed | 7.05 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.67e+03 |
| n_updates | 20499 |
-----------------------------------------------
-----------------------------------------------*****************************************----------------------| 77%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 25.289444 |
| air_temperature | 18.999548 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 19.0 |
| month | 10.0 |
| outdoor_humidity | 70.0 |
| outdoor_temperature | 2.6775541 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.13e+03 |
| n_updates | 20524 |
-----------------------------------------------
-----------------------------------------------*****************************************----------------------| 77%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 417.08643 |
| air_humidity | 46.24744 |
| air_temperature | 18.841345 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 16.0 |
| month | 10.0 |
| outdoor_humidity | 69.75 |
| outdoor_temperature | 11.781759 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 375377.78 |
| wind_direction | 237.5 |
| wind_speed | 5.575 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.7e+03 |
| n_updates | 20549 |
-----------------------------------------------
------------------------------------------------****************************************----------------------| 77%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 135.26466 |
| air_humidity | 61.379833 |
| air_temperature | 22.000166 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 13.575298 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 121738.195 |
| wind_direction | 227.5 |
| wind_speed | 4.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.3e+03 |
| n_updates | 20574 |
------------------------------------------------
-----------------------------------------------******************************************---------------------| 78%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 64.60407 |
| air_temperature | 20.836473 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 17.0 |
| month | 10.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 13.240486 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.04e+04 |
| n_updates | 20599 |
-----------------------------------------------
-----------------------------------------------******************************************---------------------| 78%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 63.3282 |
| air_temperature | 22.00007 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 14.022585 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 35.0 |
| wind_speed | 0.375 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 7.56e+03 |
| n_updates | 20624 |
-----------------------------------------------
-----------------------------------------------******************************************---------------------| 78%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 188.70605 |
| air_humidity | 67.29689 |
| air_temperature | 19.1231 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 37.5 |
| direct_solar_radiation | 0.0 |
| hour | 6.0 |
| htg_setpoint | 19.0 |
| month | 10.0 |
| outdoor_humidity | 90.0 |
| outdoor_temperature | 14.237206 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 169835.45 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.08e+04 |
| n_updates | 20649 |
-----------------------------------------------
-----------------------------------------------******************************************---------------------| 78%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 168.05627 |
| air_humidity | 53.480873 |
| air_temperature | 22.000565 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 126.0 |
| direct_solar_radiation | 279.25 |
| hour | 7.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 73.25 |
| outdoor_temperature | 14.391249 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 151250.64 |
| wind_direction | 277.5 |
| wind_speed | 6.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.48e+03 |
| n_updates | 20674 |
-----------------------------------------------
-----------------------------------------------*******************************************--------------------| 79%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 677.43176 |
| air_humidity | 49.575752 |
| air_temperature | 22.503729 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 171.75 |
| direct_solar_radiation | 443.5 |
| hour | 8.0 |
| htg_setpoint | 21.0 |
| month | 10.0 |
| outdoor_humidity | 74.75 |
| outdoor_temperature | 16.459827 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 541536.8 |
| wind_direction | 102.5 |
| wind_speed | 5.3 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.78e+03 |
| n_updates | 20699 |
-----------------------------------------------
------------------------------------------------******************************************--------------------| 79%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 349.05792 |
| air_humidity | 42.49321 |
| air_temperature | 24.44578 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 193.25 |
| direct_solar_radiation | 579.75 |
| hour | 9.0 |
| htg_setpoint | 20.0 |
| month | 10.0 |
| outdoor_humidity | 60.75 |
| outdoor_temperature | 14.8956995 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 316582.8 |
| wind_direction | 257.5 |
| wind_speed | 12.4 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.2e+03 |
| n_updates | 20724 |
------------------------------------------------
-----------------------------------------------*******************************************--------------------| 79%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 25.344414 |
| air_temperature | 24.028534 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 202.0 |
| direct_solar_radiation | 571.75 |
| hour | 10.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 42.25 |
| outdoor_temperature | 10.90466 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 317.5 |
| wind_speed | 6.825 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.76e+04 |
| n_updates | 20749 |
-----------------------------------------------
-----------------------------------------------********************************************-------------------| 80%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 32.880497 |
| air_temperature | 21.89145 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 206.25 |
| direct_solar_radiation | 571.75 |
| hour | 11.0 |
| htg_setpoint | 19.0 |
| month | 10.0 |
| outdoor_humidity | 51.5 |
| outdoor_temperature | 15.797259 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 210.0 |
| wind_speed | 4.875 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.65e+03 |
| n_updates | 20774 |
-----------------------------------------------
-----------------------------------------------********************************************-------------------| 80%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 114.77949 |
| air_humidity | 48.73564 |
| air_temperature | 22.8385 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 197.75 |
| direct_solar_radiation | 617.5 |
| hour | 12.0 |
| htg_setpoint | 20.0 |
| month | 10.0 |
| outdoor_humidity | 59.5 |
| outdoor_temperature | 18.827942 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 103301.54 |
| wind_direction | 232.5 |
| wind_speed | 4.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.33e+03 |
| n_updates | 20799 |
-----------------------------------------------
-----------------------------------------------********************************************-------------------| 80%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 47.761047 |
| air_temperature | 29.14502 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 147.75 |
| direct_solar_radiation | 609.5 |
| hour | 13.0 |
| htg_setpoint | 17.0 |
| month | 10.0 |
| outdoor_humidity | 81.25 |
| outdoor_temperature | 19.072847 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 170.0 |
| wind_speed | 7.575 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.69e+03 |
| n_updates | 20824 |
-----------------------------------------------
-----------------------------------------------********************************************-------------------| 80%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 46.74231 |
| air_temperature | 31.30642 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 157.0 |
| direct_solar_radiation | 41.75 |
| hour | 14.0 |
| htg_setpoint | 15.0 |
| month | 10.0 |
| outdoor_humidity | 67.25 |
| outdoor_temperature | 21.932957 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 112.5 |
| wind_speed | 1.125 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.67e+03 |
| n_updates | 20849 |
-----------------------------------------------
-----------------------------------------------*********************************************------------------| 81%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 63.611557 |
| air_temperature | 28.221117 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 80.0 |
| direct_solar_radiation | 45.25 |
| hour | 15.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 98.25 |
| outdoor_temperature | 19.452662 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 145.0 |
| wind_speed | 6.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.66e+03 |
| n_updates | 20874 |
-----------------------------------------------
-----------------------------------------------*********************************************------------------| 81%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 56.281235 |
| air_temperature | 30.96048 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 20.25 |
| direct_solar_radiation | 161.25 |
| hour | 16.0 |
| htg_setpoint | 21.0 |
| month | 10.0 |
| outdoor_humidity | 85.5 |
| outdoor_temperature | 20.33139 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 145.0 |
| wind_speed | 4.8 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.83e+03 |
| n_updates | 20899 |
-----------------------------------------------
-----------------------------------------------*********************************************------------------| 81%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 23.261051 |
| air_temperature | 30.090052 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 19.0 |
| month | 10.0 |
| outdoor_humidity | 21.75 |
| outdoor_temperature | 19.227865 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 262.5 |
| wind_speed | 8.5 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.15e+03 |
| n_updates | 20924 |
-----------------------------------------------
-----------------------------------------------*********************************************------------------| 81%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 16.51077 |
| air_temperature | 21.999998 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 38.75 |
| outdoor_temperature | 9.069071 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 250.0 |
| wind_speed | 9.8 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.32e+03 |
| n_updates | 20949 |
-----------------------------------------------
-----------------------------------------------**********************************************-----------------| 82%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 20.737274 |
| air_temperature | 21.259157 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 18.0 |
| month | 10.0 |
| outdoor_humidity | 54.0 |
| outdoor_temperature | 6.756015 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 315.0 |
| wind_speed | 7.075 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.98e+03 |
| n_updates | 20974 |
-----------------------------------------------
-----------------------------------------------**********************************************-----------------| 82%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 406.1129 |
| air_humidity | 22.629057 |
| air_temperature | 20.645561 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 16.0 |
| month | 10.0 |
| outdoor_humidity | 44.5 |
| outdoor_temperature | 8.182513 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 353544.72 |
| wind_direction | 0.0 |
| wind_speed | 1.125 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.66e+03 |
| n_updates | 20999 |
-----------------------------------------------
-----------------------------------------------**********************************************-----------------| 82%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 176.19357 |
| air_humidity | 29.804996 |
| air_temperature | 21.000021 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 21.0 |
| month | 10.0 |
| outdoor_humidity | 52.25 |
| outdoor_temperature | 11.880113 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 158574.2 |
| wind_direction | 265.0 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 735 |
| n_updates | 21024 |
-----------------------------------------------
-----------------------------------------------***********************************************----------------| 83%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 465.4056 |
| air_humidity | 19.860521 |
| air_temperature | 21.00008 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 21.0 |
| month | 10.0 |
| outdoor_humidity | 44.25 |
| outdoor_temperature | 6.4531593 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 418865.06 |
| wind_direction | 7.5 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.64e+03 |
| n_updates | 21049 |
-----------------------------------------------
-----------------------------------------------***********************************************----------------| 83%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 301.59442 |
| air_humidity | 48.137383 |
| air_temperature | 21.000105 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 21.0 |
| month | 10.0 |
| outdoor_humidity | 87.5 |
| outdoor_temperature | 10.345593 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 271435.0 |
| wind_direction | 210.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.1e+04 |
| n_updates | 21074 |
-----------------------------------------------
-----------------------------------------------***********************************************----------------| 83%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 76.23822 |
| air_humidity | 26.149212 |
| air_temperature | 19.272993 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 19.0 |
| month | 11.0 |
| outdoor_humidity | 50.0 |
| outdoor_temperature | 8.594441 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 68614.4 |
| wind_direction | 0.0 |
| wind_speed | 8.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.87e+03 |
| n_updates | 21099 |
-----------------------------------------------
-----------------------------------------------************************************************---------------| 84%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 29.898169 |
| air_temperature | 20.22157 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 16.0 |
| month | 11.0 |
| outdoor_humidity | 57.75 |
| outdoor_temperature | 11.937977 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 357.5 |
| wind_speed | 5.05 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.6e+03 |
| n_updates | 21124 |
-----------------------------------------------
-----------------------------------------------************************************************---------------| 84%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 303.0912 |
| air_humidity | 41.484962 |
| air_temperature | 20.999952 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 21.0 |
| month | 11.0 |
| outdoor_humidity | 86.0 |
| outdoor_temperature | 10.393824 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 272782.06 |
| wind_direction | 45.0 |
| wind_speed | 0.375 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.32e+03 |
| n_updates | 21149 |
-----------------------------------------------
-----------------------------------------------************************************************---------------| 84%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 334.2243 |
| air_humidity | 30.540747 |
| air_temperature | 21.889717 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 66.75 |
| outdoor_temperature | 8.7990265 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 300801.88 |
| wind_direction | 310.0 |
| wind_speed | 6.075 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.17e+03 |
| n_updates | 21174 |
-----------------------------------------------
-----------------------------------------------************************************************---------------| 84%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 34.27362 |
| air_temperature | 18.00155 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 18.0 |
| month | 11.0 |
| outdoor_humidity | 67.75 |
| outdoor_temperature | 5.7800684 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 322.5 |
| wind_speed | 6.325 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.04e+03 |
| n_updates | 21199 |
-----------------------------------------------
-----------------------------------------------*************************************************--------------| 85%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 35.91788 |
| air_temperature | 17.852299 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 15.0 |
| month | 11.0 |
| outdoor_humidity | 85.0 |
| outdoor_temperature | 4.6286163 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 335.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.18e+03 |
| n_updates | 21224 |
-----------------------------------------------
-----------------------------------------------*************************************************--------------| 85%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 244.03078 |
| air_humidity | 40.212387 |
| air_temperature | 18.128834 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 17.0 |
| direct_solar_radiation | 55.25 |
| hour | 6.0 |
| htg_setpoint | 18.0 |
| month | 11.0 |
| outdoor_humidity | 97.0 |
| outdoor_temperature | 4.8678036 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 219627.69 |
| wind_direction | 332.5 |
| wind_speed | 3.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.77e+03 |
| n_updates | 21249 |
-----------------------------------------------
-----------------------------------------------*************************************************--------------| 85%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 48.87794 |
| air_temperature | 21.000011 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 92.5 |
| direct_solar_radiation | 142.75 |
| hour | 7.0 |
| htg_setpoint | 21.0 |
| month | 11.0 |
| outdoor_humidity | 89.0 |
| outdoor_temperature | 10.662078 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 45.0 |
| wind_speed | 1.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.72e+03 |
| n_updates | 21274 |
-----------------------------------------------
------------------------------------------------*************************************************-------------| 86%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 70.95457 |
| air_temperature | 19.950773 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 124.25 |
| direct_solar_radiation | 115.0 |
| hour | 8.0 |
| htg_setpoint | 15.0 |
| month | 11.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 15.6532345 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 212.5 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.83e+03 |
| n_updates | 21299 |
------------------------------------------------
-----------------------------------------------**************************************************-------------| 86%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 812.9243 |
| air_humidity | 47.064064 |
| air_temperature | 22.938717 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 214.75 |
| direct_solar_radiation | 160.75 |
| hour | 9.0 |
| htg_setpoint | 21.0 |
| month | 11.0 |
| outdoor_humidity | 99.0 |
| outdoor_temperature | 9.8358345 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 731631.9 |
| wind_direction | 330.0 |
| wind_speed | 8.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 786 |
| n_updates | 21324 |
-----------------------------------------------
-----------------------------------------------**************************************************-------------| 86%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 855.16016 |
| air_humidity | 34.934628 |
| air_temperature | 23.299423 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 161.5 |
| direct_solar_radiation | 0.0 |
| hour | 10.0 |
| htg_setpoint | 20.0 |
| month | 11.0 |
| outdoor_humidity | 71.5 |
| outdoor_temperature | 8.631185 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 769644.2 |
| wind_direction | 357.5 |
| wind_speed | 7.075 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.34e+03 |
| n_updates | 21349 |
-----------------------------------------------
-----------------------------------------------**************************************************-------------| 86%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 755.6465 |
| air_humidity | 37.76056 |
| air_temperature | 22.979378 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 105.25 |
| direct_solar_radiation | 0.0 |
| hour | 11.0 |
| htg_setpoint | 16.0 |
| month | 11.0 |
| outdoor_humidity | 76.75 |
| outdoor_temperature | 9.527214 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 680081.9 |
| wind_direction | 20.0 |
| wind_speed | 0.775 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.9e+03 |
| n_updates | 21374 |
-----------------------------------------------
-----------------------------------------------***************************************************------------| 87%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 255.54282 |
| air_humidity | 51.38102 |
| air_temperature | 24.256739 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 126.0 |
| direct_solar_radiation | 0.0 |
| hour | 12.0 |
| htg_setpoint | 20.0 |
| month | 11.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 11.949695 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 229988.53 |
| wind_direction | 127.5 |
| wind_speed | 2.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 994 |
| n_updates | 21399 |
-----------------------------------------------
-----------------------------------------------***************************************************------------| 87%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 746.01514 |
| air_humidity | 23.516073 |
| air_temperature | 23.465982 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 153.5 |
| direct_solar_radiation | 13.5 |
| hour | 13.0 |
| htg_setpoint | 18.0 |
| month | 11.0 |
| outdoor_humidity | 48.5 |
| outdoor_temperature | 6.8982987 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 671413.6 |
| wind_direction | 285.0 |
| wind_speed | 7.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.48e+03 |
| n_updates | 21424 |
-----------------------------------------------
-----------------------------------------------***************************************************------------| 87%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 26.107616 |
| air_temperature | 20.64333 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 101.75 |
| direct_solar_radiation | 26.25 |
| hour | 14.0 |
| htg_setpoint | 16.0 |
| month | 11.0 |
| outdoor_humidity | 57.5 |
| outdoor_temperature | 9.14588 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 230.0 |
| wind_speed | 5.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.53e+03 |
| n_updates | 21449 |
-----------------------------------------------
-----------------------------------------------***************************************************------------| 87%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 27.82852 |
| air_temperature | 21.930077 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 30.25 |
| direct_solar_radiation | 0.0 |
| hour | 15.0 |
| htg_setpoint | 18.0 |
| month | 11.0 |
| outdoor_humidity | 48.5 |
| outdoor_temperature | 10.184548 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 297.5 |
| wind_speed | 7.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.91e+03 |
| n_updates | 21474 |
-----------------------------------------------
-----------------------------------------------****************************************************-----------| 88%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 28.248968 |
| air_temperature | 23.18946 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 16.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 56.0 |
| outdoor_temperature | 6.470765 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 257.5 |
| wind_speed | 8.075 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.57e+03 |
| n_updates | 21499 |
-----------------------------------------------
-----------------------------------------------****************************************************-----------| 88%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 29.087454 |
| air_temperature | 22.994007 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 68.25 |
| outdoor_temperature | 4.6299367 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 237.5 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.27e+03 |
| n_updates | 21524 |
-----------------------------------------------
-----------------------------------------------****************************************************-----------| 88%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 765.85596 |
| air_humidity | 37.356014 |
| air_temperature | 21.712988 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 16.0 |
| month | 11.0 |
| outdoor_humidity | 86.5 |
| outdoor_temperature | 5.873901 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 678344.4 |
| wind_direction | 217.5 |
| wind_speed | 9.15 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2e+03 |
| n_updates | 21549 |
-----------------------------------------------
------------------------------------------------****************************************************----------| 89%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 1263.6243 |
| air_humidity | 27.212038 |
| air_temperature | 22.358484 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 19.0 |
| month | 11.0 |
| outdoor_humidity | 66.25 |
| outdoor_temperature | 0.43878692 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 1137261.9 |
| wind_direction | 280.0 |
| wind_speed | 8.925 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 8.11e+03 |
| n_updates | 21574 |
------------------------------------------------
------------------------------------------------****************************************************----------| 89%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1525.2096 |
| air_humidity | 21.43743 |
| air_temperature | 22.001425 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 56.25 |
| outdoor_temperature | -0.8691397 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 1372688.6 |
| wind_direction | 285.0 |
| wind_speed | 9.55 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.46e+03 |
| n_updates | 21599 |
------------------------------------------------
-----------------------------------------------*****************************************************----------| 89%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 1891.6239 |
| air_humidity | 17.67955 |
| air_temperature | 18.995008 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 16.0 |
| month | 11.0 |
| outdoor_humidity | 47.5 |
| outdoor_temperature | -2.701211 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1702461.5 |
| wind_direction | 307.5 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.71e+03 |
| n_updates | 21624 |
-----------------------------------------------
-----------------------------------------------*****************************************************----------| 89%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 13.117279 |
| air_temperature | 21.999998 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 62.25 |
| outdoor_temperature | 2.0829508 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 10.0 |
| wind_speed | 0.525 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.71e+03 |
| n_updates | 21649 |
-----------------------------------------------
-----------------------------------------------******************************************************---------| 90%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 636.2585 |
| air_humidity | 37.8067 |
| air_temperature | 21.249306 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 98.25 |
| outdoor_temperature | 4.57644 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 572632.7 |
| wind_direction | 60.0 |
| wind_speed | 4.375 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.48e+03 |
| n_updates | 21674 |
-----------------------------------------------
-----------------------------------------------******************************************************---------| 90%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 676.5342 |
| air_humidity | 58.59235 |
| air_temperature | 18.482203 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 18.0 |
| month | 11.0 |
| outdoor_humidity | 96.25 |
| outdoor_temperature | 10.229322 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 608880.75 |
| wind_direction | 265.0 |
| wind_speed | 3.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.4e+03 |
| n_updates | 21699 |
-----------------------------------------------
-----------------------------------------------******************************************************---------| 90%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 39.79686 |
| air_temperature | 18.229832 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 18.0 |
| month | 11.0 |
| outdoor_humidity | 71.0 |
| outdoor_temperature | 8.900488 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 262.5 |
| wind_speed | 5.05 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.45e+03 |
| n_updates | 21724 |
-----------------------------------------------
-----------------------------------------------*******************************************************--------| 91%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 30.653414 |
| air_temperature | 19.000013 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 19.0 |
| month | 11.0 |
| outdoor_humidity | 67.0 |
| outdoor_temperature | 4.4980135 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 272.5 |
| wind_speed | 5.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.38e+03 |
| n_updates | 21749 |
-----------------------------------------------
-----------------------------------------------*******************************************************--------| 91%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 179.40852 |
| air_humidity | 31.820286 |
| air_temperature | 19.999996 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 20.0 |
| month | 11.0 |
| outdoor_humidity | 93.75 |
| outdoor_temperature | 4.539938 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 161467.67 |
| wind_direction | 270.0 |
| wind_speed | 3.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.08e+03 |
| n_updates | 21774 |
-----------------------------------------------
-----------------------------------------------*******************************************************--------| 91%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 225.59595 |
| air_humidity | 32.762558 |
| air_temperature | 19.0 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 19.0 |
| month | 12.0 |
| outdoor_humidity | 82.0 |
| outdoor_temperature | 7.7978168 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 203036.36 |
| wind_direction | 312.5 |
| wind_speed | 10.525 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.51e+03 |
| n_updates | 21799 |
-----------------------------------------------
-----------------------------------------------********************************************************-------| 92%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 1103.6442 |
| air_humidity | 18.20577 |
| air_temperature | 18.0 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 18.0 |
| month | 12.0 |
| outdoor_humidity | 43.75 |
| outdoor_temperature | 1.2386879 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 993279.7 |
| wind_direction | 297.5 |
| wind_speed | 10.8 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 745 |
| n_updates | 21824 |
-----------------------------------------------
-----------------------------------------------********************************************************-------| 92%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 244.03078 |
| air_humidity | 19.739792 |
| air_temperature | 20.000017 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 6.0 |
| htg_setpoint | 20.0 |
| month | 12.0 |
| outdoor_humidity | 63.0 |
| outdoor_temperature | 2.0301712 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 219627.69 |
| wind_direction | 257.5 |
| wind_speed | 5.425 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.11e+03 |
| n_updates | 21849 |
-----------------------------------------------
-----------------------------------------------********************************************************-------| 92%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 244.03078 |
| air_humidity | 35.217537 |
| air_temperature | 20.274149 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 18.75 |
| direct_solar_radiation | 0.0 |
| hour | 7.0 |
| htg_setpoint | 22.0 |
| month | 12.0 |
| outdoor_humidity | 97.0 |
| outdoor_temperature | 6.9920654 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 219627.69 |
| wind_direction | 327.5 |
| wind_speed | 0.375 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.55e+03 |
| n_updates | 21874 |
-----------------------------------------------
-----------------------------------------------********************************************************-------| 92%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 32.84092 |
| air_temperature | 21.583551 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 68.5 |
| direct_solar_radiation | 3.0 |
| hour | 8.0 |
| htg_setpoint | 21.0 |
| month | 12.0 |
| outdoor_humidity | 76.75 |
| outdoor_temperature | 5.4908586 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 60.0 |
| wind_speed | 2.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.15e+03 |
| n_updates | 21899 |
-----------------------------------------------
-----------------------------------------------*********************************************************------| 93%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1209.0073 |
| air_humidity | 20.072664 |
| air_temperature | 22.122103 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 80.25 |
| direct_solar_radiation | 65.25 |
| hour | 9.0 |
| htg_setpoint | 22.0 |
| month | 12.0 |
| outdoor_humidity | 58.75 |
| outdoor_temperature | 0.2546476 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1088106.5 |
| wind_direction | 270.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.3e+03 |
| n_updates | 21924 |
-----------------------------------------------
-----------------------------------------------*********************************************************------| 93%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 968.7795 |
| air_humidity | 20.682884 |
| air_temperature | 18.82307 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 210.5 |
| direct_solar_radiation | 213.75 |
| hour | 10.0 |
| htg_setpoint | 16.0 |
| month | 12.0 |
| outdoor_humidity | 53.25 |
| outdoor_temperature | 1.9130111 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 871901.56 |
| wind_direction | 277.5 |
| wind_speed | 6.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.2e+03 |
| n_updates | 21949 |
-----------------------------------------------
-----------------------------------------------*********************************************************------| 93%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 18.628345 |
| air_temperature | 21.067177 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 92.25 |
| direct_solar_radiation | 492.0 |
| hour | 11.0 |
| htg_setpoint | 18.0 |
| month | 12.0 |
| outdoor_humidity | 46.5 |
| outdoor_temperature | 7.173874 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 302.5 |
| wind_speed | 7.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 8.75e+03 |
| n_updates | 21974 |
-----------------------------------------------
-----------------------------------------------*********************************************************------| 93%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 476.10425 |
| air_humidity | 22.734207 |
| air_temperature | 23.486908 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 167.25 |
| direct_solar_radiation | 121.75 |
| hour | 12.0 |
| htg_setpoint | 17.0 |
| month | 12.0 |
| outdoor_humidity | 66.0 |
| outdoor_temperature | 2.5671427 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 428493.84 |
| wind_direction | 327.5 |
| wind_speed | 0.375 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.74e+03 |
| n_updates | 21999 |
-----------------------------------------------
-----------------------------------------------**********************************************************-----| 94%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 755.6465 |
| air_humidity | 28.432045 |
| air_temperature | 23.13434 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 92.75 |
| direct_solar_radiation | 0.0 |
| hour | 13.0 |
| htg_setpoint | 22.0 |
| month | 12.0 |
| outdoor_humidity | 87.25 |
| outdoor_temperature | 3.7937093 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 680081.9 |
| wind_direction | 30.0 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 7.49e+03 |
| n_updates | 22024 |
-----------------------------------------------
-----------------------------------------------**********************************************************-----| 94%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 861.25696 |
| air_humidity | 26.538927 |
| air_temperature | 22.993273 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 53.25 |
| direct_solar_radiation | 0.0 |
| hour | 14.0 |
| htg_setpoint | 18.0 |
| month | 12.0 |
| outdoor_humidity | 73.0 |
| outdoor_temperature | 1.9933993 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 775131.25 |
| wind_direction | 35.0 |
| wind_speed | 3.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.18e+03 |
| n_updates | 22049 |
-----------------------------------------------
-----------------------------------------------**********************************************************-----| 94%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 30.87924 |
| air_temperature | 23.100765 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 36.0 |
| direct_solar_radiation | 9.0 |
| hour | 15.0 |
| htg_setpoint | 22.0 |
| month | 12.0 |
| outdoor_humidity | 79.75 |
| outdoor_temperature | 3.5068433 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 305.0 |
| wind_speed | 4.8 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.27e+03 |
| n_updates | 22074 |
-----------------------------------------------
-----------------------------------------------***********************************************************----| 95%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 22.573284 |
| air_temperature | 22.911606 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 16.0 |
| htg_setpoint | 21.0 |
| month | 12.0 |
| outdoor_humidity | 50.0 |
| outdoor_temperature | 3.334994 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 280.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.52e+03 |
| n_updates | 22099 |
-----------------------------------------------
-----------------------------------------------***********************************************************----| 95%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 17.63873 |
| air_temperature | 20.344404 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 15.0 |
| month | 12.0 |
| outdoor_humidity | 32.25 |
| outdoor_temperature | 3.4847171 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 310.0 |
| wind_speed | 8.925 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 572 |
| n_updates | 22124 |
-----------------------------------------------
-----------------------------------------------***********************************************************----| 95%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 78.55217 |
| air_humidity | 17.442886 |
| air_temperature | 20.012045 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 16.0 |
| month | 12.0 |
| outdoor_humidity | 62.25 |
| outdoor_temperature | 4.591076 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 67041.266 |
| wind_direction | 230.0 |
| wind_speed | 7.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.8e+03 |
| n_updates | 22149 |
-----------------------------------------------
-----------------------------------------------***********************************************************----| 95%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 538.88184 |
| air_humidity | 24.78695 |
| air_temperature | 21.017 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 21.0 |
| month | 12.0 |
| outdoor_humidity | 58.0 |
| outdoor_temperature | 5.038567 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 484993.62 |
| wind_direction | 220.0 |
| wind_speed | 3.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 9.34e+03 |
| n_updates | 22174 |
-----------------------------------------------
-----------------------------------------------************************************************************---| 96%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 443.30167 |
| air_humidity | 26.74962 |
| air_temperature | 20.506552 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 15.0 |
| month | 12.0 |
| outdoor_humidity | 52.0 |
| outdoor_temperature | 6.9844346 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 384110.12 |
| wind_direction | 337.5 |
| wind_speed | 4.25 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.71e+03 |
| n_updates | 22199 |
-----------------------------------------------
-----------------------------------------------************************************************************---| 96%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 798.4142 |
| air_humidity | 30.103796 |
| air_temperature | 20.000015 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 20.0 |
| month | 12.0 |
| outdoor_humidity | 80.25 |
| outdoor_temperature | 4.3912582 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 718572.75 |
| wind_direction | 230.0 |
| wind_speed | 5.05 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 824 |
| n_updates | 22224 |
-----------------------------------------------
-----------------------------------------------************************************************************---| 96%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 28.865433 |
| air_temperature | 18.52578 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 16.0 |
| month | 12.0 |
| outdoor_humidity | 76.25 |
| outdoor_temperature | 4.8629026 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 240.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.78e+03 |
| n_updates | 22249 |
-----------------------------------------------
-----------------------------------------------************************************************************---| 96%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 634.5351 |
| air_humidity | 24.009302 |
| air_temperature | 20.856209 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 21.0 |
| month | 12.0 |
| outdoor_humidity | 54.0 |
| outdoor_temperature | 5.6826453 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 577064.06 |
| wind_direction | 355.0 |
| wind_speed | 4.375 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 607 |
| n_updates | 22274 |
-----------------------------------------------
------------------------------------------------************************************************************--| 97%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1824.9485 |
| air_humidity | 13.21023 |
| air_temperature | 20.999922 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 21.0 |
| month | 12.0 |
| outdoor_humidity | 44.5 |
| outdoor_temperature | -2.3678339 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1642453.6 |
| wind_direction | 345.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 855 |
| n_updates | 22299 |
------------------------------------------------
-----------------------------------------------*************************************************************--| 97%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 15.420471 |
| air_temperature | 21.000063 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 21.0 |
| month | 12.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 3.1042883 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 80.0 |
| wind_speed | 8.4 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.4e+03 |
| n_updates | 22324 |
-----------------------------------------------
-----------------------------------------------**************************************************************-| 98%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 33.208645 |
| air_temperature | 19.72489 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 22.0 |
| month | 12.0 |
| outdoor_humidity | 72.25 |
| outdoor_temperature | 4.998941 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 297.5 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.88e+03 |
| n_updates | 22349 |
-----------------------------------------------
-----------------------------------------------**************************************************************-| 98%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 737.5208 |
| air_humidity | 35.81898 |
| air_temperature | 19.986662 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 21.0 |
| month | 12.0 |
| outdoor_humidity | 94.0 |
| outdoor_temperature | 8.87142 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 663768.75 |
| wind_direction | 95.0 |
| wind_speed | 8.075 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.74e+03 |
| n_updates | 22374 |
-----------------------------------------------
-----------------------------------------------**************************************************************-| 98%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 34.983475 |
| air_temperature | 20.000034 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 20.0 |
| month | 12.0 |
| outdoor_humidity | 89.75 |
| outdoor_temperature | 4.678801 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 247.5 |
| wind_speed | 3.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.38e+03 |
| n_updates | 22399 |
-----------------------------------------------
-----------------------------------------------**************************************************************-| 98%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 25.802359 |
| air_temperature | 17.999998 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 18.0 |
| month | 12.0 |
| outdoor_humidity | 55.5 |
| outdoor_temperature | 4.5781016 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 295.0 |
| wind_speed | 3.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.97e+03 |
| n_updates | 22424 |
-----------------------------------------------
-----------------------------------------------***************************************************************| 99%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 977.8774 |
| air_humidity | 30.994516 |
| air_temperature | 21.99999 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 6.0 |
| htg_setpoint | 22.0 |
| month | 12.0 |
| outdoor_humidity | 63.25 |
| outdoor_temperature | 1.8675216 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 880089.6 |
| wind_direction | 342.5 |
| wind_speed | 5.825 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.33e+03 |
| n_updates | 22449 |
-----------------------------------------------
------------------------------------------------**************************************************************| 99%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 1262.1515 |
| air_humidity | 19.378036 |
| air_temperature | 20.0 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 20.75 |
| direct_solar_radiation | 10.75 |
| hour | 7.0 |
| htg_setpoint | 20.0 |
| month | 12.0 |
| outdoor_humidity | 72.0 |
| outdoor_temperature | 0.44615075 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1135936.4 |
| wind_direction | 65.0 |
| wind_speed | 2.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.44e+03 |
| n_updates | 22474 |
------------------------------------------------
-----------------------------------------------***************************************************************| 99%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1012.9957 |
| air_humidity | 28.28078 |
| air_temperature | 21.999395 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 36.25 |
| direct_solar_radiation | 0.0 |
| hour | 8.0 |
| htg_setpoint | 22.0 |
| month | 12.0 |
| outdoor_humidity | 91.25 |
| outdoor_temperature | 1.2347054 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 911696.1 |
| wind_direction | 220.0 |
| wind_speed | 12.775 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.35e+03 |
| n_updates | 22499 |
-----------------------------------------------
------------------------------------------------**************************************************************| 99%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 1838.0356 |
| air_humidity | 15.019802 |
| air_temperature | 22.52058 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 90.75 |
| direct_solar_radiation | 430.75 |
| hour | 9.0 |
| htg_setpoint | 22.0 |
| month | 12.0 |
| outdoor_humidity | 41.0 |
| outdoor_temperature | -2.8313658 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1654232.1 |
| wind_direction | 265.0 |
| wind_speed | 10.175 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.62e+03 |
| n_updates | 22524 |
------------------------------------------------
[WRAPPER LoggerWrapper] (INFO) : End of episode, recording summary (progress.csv) if logger is active
[ENVIRONMENT] (INFO) : Environment closed. [SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43]
#----------------------------------------------------------------------------------------------#
[ENVIRONMENT] (INFO) : Starting a new episode... [SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43_EVALUATION] [Episode 2]
#----------------------------------------------------------------------------------------------#
[MODELING] (INFO) : Episode directory created [/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43_EVALUATION-res1/Eplus-env-sub_run2]
[MODELING] (INFO) : Weather file USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3.epw used.
[MODELING] (INFO) : Updated building model with whole Output:Variable available names
[MODELING] (INFO) : Updated building model with whole Output:Meter available names
[MODELING] (INFO) : Adapting weather to building model. [USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3.epw]
[ENVIRONMENT] (INFO) : Saving episode output path... [/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43_EVALUATION-res1/Eplus-env-sub_run2/output]
[SIMULATOR] (INFO) : Running EnergyPlus with args: ['-w', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43_EVALUATION-res1/Eplus-env-sub_run2/USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3_Random_1.0_0.0_0.001.epw', '-d', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43_EVALUATION-res1/Eplus-env-sub_run2/output', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43_EVALUATION-res1/Eplus-env-sub_run2/5ZoneAutoDXVAV.epJSON']
[ENVIRONMENT] (INFO) : Episode 2 started.
/usr/local/lib/python3.10/dist-packages/opyplus/weather_data/weather_data.py:493: FutureWarning: the 'line_terminator'' keyword is deprecated, use 'lineterminator' instead.
epw_content = self._headers_to_epw(use_datetimes=use_datetimes) + df.to_csv(
[SIMULATOR] (INFO) : handlers are ready.
[SIMULATOR] (INFO) : System is ready.
[WRAPPER LoggerWrapper] (INFO) : Creating monitor.csv for current episode (episode 2) if logger is active
Progress: |**-------------------------------------------------------------------------------------------------| 2%
/usr/local/lib/python3.10/dist-packages/gymnasium/spaces/box.py:240: UserWarning: WARN: Casting input x to numpy array.
gym.logger.warn("Casting input x to numpy array.")
Progress: |***************************************************************************************************| 99%
[WRAPPER LoggerWrapper] (INFO) : End of episode, recording summary (progress.csv) if logger is active
[ENVIRONMENT] (INFO) : Environment closed. [SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43_EVALUATION]
#----------------------------------------------------------------------------------------------#
[ENVIRONMENT] (INFO) : Starting a new episode... [SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43] [Episode 5]
#----------------------------------------------------------------------------------------------#
[MODELING] (INFO) : Episode directory created [/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43-res1/Eplus-env-sub_run5]
[MODELING] (INFO) : Weather file USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3.epw used.
[MODELING] (INFO) : Updated building model with whole Output:Variable available names
[MODELING] (INFO) : Updated building model with whole Output:Meter available names
[MODELING] (INFO) : Adapting weather to building model. [USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3.epw]
[ENVIRONMENT] (INFO) : Saving episode output path... [/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43-res1/Eplus-env-sub_run5/output]
[SIMULATOR] (INFO) : Running EnergyPlus with args: ['-w', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43-res1/Eplus-env-sub_run5/USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3_Random_1.0_0.0_0.001.epw', '-d', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43-res1/Eplus-env-sub_run5/output', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43-res1/Eplus-env-sub_run5/5ZoneAutoDXVAV.epJSON']
[ENVIRONMENT] (INFO) : Episode 5 started.
/usr/local/lib/python3.10/dist-packages/opyplus/weather_data/weather_data.py:493: FutureWarning: the 'line_terminator'' keyword is deprecated, use 'lineterminator' instead.
epw_content = self._headers_to_epw(use_datetimes=use_datetimes) + df.to_csv(
[WRAPPER LoggerWrapper] (INFO) : Creating monitor.csv for current episode (episode 5) if logger is active
Eval num_timesteps=140154, episode_reward=-19379.18 +/- 0.00
Episode length: 35040.00 +/- 0.00
----------------------------------------------------
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| eval/ | |
| comfort_violation(%) | 58.2 |
| cumulative_comfort_penalty | -1.77e+04 |
| cumulative_energy_penalty | -1.71e+03 |
| cumulative_power_consumption | 3.42e+07 |
| cumulative_reward | -1.94e+04 |
| cumulative_temperature_violation | 3.53e+04 |
| episode_length | 3.5e+04 |
| mean_comfort_penalty | -0.504 |
| mean_energy_penalty | -0.0488 |
| mean_power_consumption | 975 |
| mean_reward | -0.553 |
| mean_temperature_violation | 1.01 |
| std_cumulative_reward | 0 |
| std_reward | 0 |
| observation/ | |
| HVAC_electricity_demand_rate | 2817.9358 |
| air_humidity | 19.03184 |
| air_temperature | 17.655746 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 17.0 |
| month | 12.0 |
| outdoor_humidity | 38.0 |
| outdoor_temperature | -7.3327703 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2536142.2 |
| wind_direction | 312.5 |
| wind_speed | 8.925 |
| rollout/ | |
| exploration_rate | 0.05 |
| time/ | |
| total_timesteps | 140154 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 8.86e+03 |
| n_updates | 22538 |
----------------------------------------------------
[SIMULATOR] (INFO) : handlers are ready.
[SIMULATOR] (INFO) : System is ready.
------------------------------------------------
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1502.1025 |
| air_humidity | 14.293673 |
| air_temperature | 22.445791 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 189.5 |
| direct_solar_radiation | 229.5 |
| hour | 11.0 |
| htg_setpoint | 21.0 |
| month | 1.0 |
| outdoor_humidity | 34.5 |
| outdoor_temperature | -1.5963737 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 1351892.4 |
| wind_direction | 265.0 |
| wind_speed | 9.55 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 903 |
| n_updates | 22549 |
------------------------------------------------
/usr/local/lib/python3.10/dist-packages/gymnasium/core.py:311: UserWarning: WARN: env.observation_variables to get variables from other wrappers is deprecated and will be removed in v1.0, to get this variable you can do `env.unwrapped.observation_variables` for environment variables or `env.get_wrapper_attr('observation_variables')` that will search the reminding wrappers.
logger.warn(
/usr/local/lib/python3.10/dist-packages/gymnasium/core.py:311: UserWarning: WARN: env.action_variables to get variables from other wrappers is deprecated and will be removed in v1.0, to get this variable you can do `env.unwrapped.action_variables` for environment variables or `env.get_wrapper_attr('action_variables')` that will search the reminding wrappers.
logger.warn(
/usr/local/lib/python3.10/dist-packages/gymnasium/core.py:311: UserWarning: WARN: env.is_discrete to get variables from other wrappers is deprecated and will be removed in v1.0, to get this variable you can do `env.unwrapped.is_discrete` for environment variables or `env.get_wrapper_attr('is_discrete')` that will search the reminding wrappers.
logger.warn(
/usr/local/lib/python3.10/dist-packages/gymnasium/spaces/box.py:240: UserWarning: WARN: Casting input x to numpy array.
gym.logger.warn("Casting input x to numpy array.")
--------------------------------------------------------------------------------------------------------------| 1%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 2402.0833 |
| air_humidity | 11.437536 |
| air_temperature | 23.042078 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 194.0 |
| direct_solar_radiation | 340.5 |
| hour | 12.0 |
| htg_setpoint | 21.0 |
| month | 1.0 |
| outdoor_humidity | 36.5 |
| outdoor_temperature | -7.386407 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 2161875.0 |
| wind_direction | 275.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.41e+03 |
| n_updates | 22574 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 1%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 730.1309 |
| air_humidity | 48.870342 |
| air_temperature | 22.699852 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 66.5 |
| direct_solar_radiation | 0.0 |
| hour | 13.0 |
| htg_setpoint | 20.0 |
| month | 1.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 10.502956 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 657117.8 |
| wind_direction | 60.0 |
| wind_speed | 6.575 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 586 |
| n_updates | 22599 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 1%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 1743.8271 |
| air_humidity | 15.084388 |
| air_temperature | 23.225992 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 84.5 |
| direct_solar_radiation | 617.25 |
| hour | 14.0 |
| htg_setpoint | 18.0 |
| month | 1.0 |
| outdoor_humidity | 41.25 |
| outdoor_temperature | -2.2472076 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1569444.5 |
| wind_direction | 267.5 |
| wind_speed | 8.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.24e+03 |
| n_updates | 22624 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 1%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 2086.3428 |
| air_humidity | 11.047049 |
| air_temperature | 21.712967 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 61.25 |
| direct_solar_radiation | 446.25 |
| hour | 15.0 |
| htg_setpoint | 15.0 |
| month | 1.0 |
| outdoor_humidity | 31.25 |
| outdoor_temperature | -3.6748056 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1877708.5 |
| wind_direction | 312.5 |
| wind_speed | 4.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.9e+03 |
| n_updates | 22649 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 2%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 1514.7529 |
| air_humidity | 11.312537 |
| air_temperature | 18.06745 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 17.75 |
| direct_solar_radiation | 39.0 |
| hour | 16.0 |
| htg_setpoint | 15.0 |
| month | 1.0 |
| outdoor_humidity | 38.5 |
| outdoor_temperature | -0.81685615 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1363277.6 |
| wind_direction | 212.5 |
| wind_speed | 8.075 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.15e+03 |
| n_updates | 22674 |
-------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 2%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 1679.8973 |
| air_humidity | 14.889441 |
| air_temperature | 23.022127 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 16.0 |
| month | 1.0 |
| outdoor_humidity | 33.25 |
| outdoor_temperature | -2.0998027 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1511907.6 |
| wind_direction | 255.0 |
| wind_speed | 7.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.05e+04 |
| n_updates | 22699 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 2%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1181.1582 |
| air_humidity | 30.25977 |
| air_temperature | 22.538345 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 21.0 |
| month | 1.0 |
| outdoor_humidity | 95.75 |
| outdoor_temperature | 0.8511174 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 1063042.4 |
| wind_direction | 30.0 |
| wind_speed | 4.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.41e+03 |
| n_updates | 22724 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 2%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1137.0897 |
| air_humidity | 35.217064 |
| air_temperature | 22.56905 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 16.0 |
| month | 1.0 |
| outdoor_humidity | 53.25 |
| outdoor_temperature | 1.0714601 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 1023380.7 |
| wind_direction | 300.0 |
| wind_speed | 12.075 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.99e+03 |
| n_updates | 22749 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 3%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 2040.2118 |
| air_humidity | 19.544863 |
| air_temperature | 21.461933 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 15.0 |
| month | 1.0 |
| outdoor_humidity | 46.0 |
| outdoor_temperature | -3.4441502 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 1836190.6 |
| wind_direction | 220.0 |
| wind_speed | 6.075 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.27e+03 |
| n_updates | 22774 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 3%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 2529.7434 |
| air_humidity | 14.992049 |
| air_temperature | 22.000025 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 22.0 |
| month | 1.0 |
| outdoor_humidity | 39.5 |
| outdoor_temperature | -5.891808 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2276769.0 |
| wind_direction | 300.0 |
| wind_speed | 1.575 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.86e+03 |
| n_updates | 22799 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 3%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 88.66814 |
| air_humidity | 24.351942 |
| air_temperature | 20.999962 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 21.0 |
| month | 1.0 |
| outdoor_humidity | 76.75 |
| outdoor_temperature | 5.703776 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 79801.32 |
| wind_direction | 187.5 |
| wind_speed | 3.725 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.4e+04 |
| n_updates | 22824 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 4%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1896.4862 |
| air_humidity | 26.343338 |
| air_temperature | 20.999996 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 21.0 |
| month | 1.0 |
| outdoor_humidity | 80.25 |
| outdoor_temperature | -2.7255228 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1706837.6 |
| wind_direction | 15.0 |
| wind_speed | 7.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.23e+03 |
| n_updates | 22849 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 4%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1738.7863 |
| air_humidity | 23.685612 |
| air_temperature | 21.000185 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 21.0 |
| month | 1.0 |
| outdoor_humidity | 95.0 |
| outdoor_temperature | -1.9370227 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1564907.6 |
| wind_direction | 40.0 |
| wind_speed | 6.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.53e+03 |
| n_updates | 22874 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 4%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 1819.8478 |
| air_humidity | 36.205776 |
| air_temperature | 18.776604 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 16.0 |
| month | 1.0 |
| outdoor_humidity | 59.75 |
| outdoor_temperature | -2.3423305 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1637863.0 |
| wind_direction | 262.5 |
| wind_speed | 8.4 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 869 |
| n_updates | 22899 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 5%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 28.533558 |
| air_temperature | 20.0 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 20.0 |
| month | 1.0 |
| outdoor_humidity | 77.25 |
| outdoor_temperature | 3.254023 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 237.5 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.3e+03 |
| n_updates | 22924 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 5%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 775.37915 |
| air_humidity | 30.69621 |
| air_temperature | 20.610672 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 21.0 |
| month | 1.0 |
| outdoor_humidity | 95.0 |
| outdoor_temperature | 5.3951087 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 697841.25 |
| wind_direction | 110.0 |
| wind_speed | 4.75 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.26e+03 |
| n_updates | 22949 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 5%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1090.8883 |
| air_humidity | 34.197186 |
| air_temperature | 21.0 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 21.0 |
| month | 1.0 |
| outdoor_humidity | 94.25 |
| outdoor_temperature | 1.3024671 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 981799.44 |
| wind_direction | 230.0 |
| wind_speed | 3.725 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.22e+04 |
| n_updates | 22974 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 5%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 76.24103 |
| air_humidity | 15.340016 |
| air_temperature | 22.000084 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 22.0 |
| month | 1.0 |
| outdoor_humidity | 32.5 |
| outdoor_temperature | 5.6408863 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 68616.93 |
| wind_direction | 277.5 |
| wind_speed | 5.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.2e+03 |
| n_updates | 22999 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 6%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 653.5078 |
| air_humidity | 25.526716 |
| air_temperature | 19.085022 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 6.0 |
| htg_setpoint | 18.0 |
| month | 1.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | -0.04738527 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 588157.06 |
| wind_direction | 62.5 |
| wind_speed | 1.65 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.51e+03 |
| n_updates | 23024 |
-------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 6%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 244.03078 |
| air_humidity | 34.741325 |
| air_temperature | 21.45583 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 7.0 |
| htg_setpoint | 22.0 |
| month | 1.0 |
| outdoor_humidity | 99.0 |
| outdoor_temperature | 6.0234256 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 219627.69 |
| wind_direction | 40.0 |
| wind_speed | 5.425 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 955 |
| n_updates | 23049 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 6%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 34.4651 |
| air_temperature | 21.080013 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 24.75 |
| direct_solar_radiation | 0.0 |
| hour | 8.0 |
| htg_setpoint | 21.0 |
| month | 1.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 4.02629 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 50.0 |
| wind_speed | 3.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.65e+03 |
| n_updates | 23074 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 7%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 49.845024 |
| air_temperature | 22.139757 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 68.75 |
| direct_solar_radiation | 0.0 |
| hour | 9.0 |
| htg_setpoint | 22.0 |
| month | 1.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 9.3318205 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 190.0 |
| wind_speed | 8.8 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.57e+03 |
| n_updates | 23099 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 7%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 22.955624 |
| air_temperature | 22.493074 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 134.25 |
| direct_solar_radiation | 0.0 |
| hour | 10.0 |
| htg_setpoint | 22.0 |
| month | 1.0 |
| outdoor_humidity | 54.25 |
| outdoor_temperature | 3.3589754 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 305.0 |
| wind_speed | 5.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.66e+03 |
| n_updates | 23124 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 7%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 134.70111 |
| air_humidity | 25.452288 |
| air_temperature | 21.407345 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 147.75 |
| direct_solar_radiation | 702.0 |
| hour | 11.0 |
| htg_setpoint | 22.0 |
| month | 1.0 |
| outdoor_humidity | 49.25 |
| outdoor_temperature | 5.5939164 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 130938.66 |
| wind_direction | 310.0 |
| wind_speed | 3.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.19e+03 |
| n_updates | 23149 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 7%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 94.756516 |
| air_humidity | 20.744356 |
| air_temperature | 21.000023 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 198.5 |
| direct_solar_radiation | 13.5 |
| hour | 12.0 |
| htg_setpoint | 21.0 |
| month | 1.0 |
| outdoor_humidity | 54.25 |
| outdoor_temperature | 4.2581863 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 85280.87 |
| wind_direction | 170.0 |
| wind_speed | 2.725 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 953 |
| n_updates | 23174 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 8%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 755.6465 |
| air_humidity | 31.130491 |
| air_temperature | 22.85354 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 204.5 |
| direct_solar_radiation | 107.25 |
| hour | 13.0 |
| htg_setpoint | 20.0 |
| month | 1.0 |
| outdoor_humidity | 82.75 |
| outdoor_temperature | 4.7462764 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 680081.9 |
| wind_direction | 65.0 |
| wind_speed | 2.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.49e+03 |
| n_updates | 23199 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 8%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 894.3857 |
| air_humidity | 21.35435 |
| air_temperature | 23.739147 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 101.5 |
| direct_solar_radiation | 669.5 |
| hour | 14.0 |
| htg_setpoint | 19.0 |
| month | 1.0 |
| outdoor_humidity | 47.75 |
| outdoor_temperature | 4.232125 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 804947.06 |
| wind_direction | 357.5 |
| wind_speed | 6.075 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.99e+03 |
| n_updates | 23224 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 8%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 909.95856 |
| air_humidity | 20.03153 |
| air_temperature | 23.830122 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 117.0 |
| direct_solar_radiation | 40.0 |
| hour | 15.0 |
| htg_setpoint | 22.0 |
| month | 1.0 |
| outdoor_humidity | 50.0 |
| outdoor_temperature | 1.9822812 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 818962.7 |
| wind_direction | 315.0 |
| wind_speed | 5.825 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.24e+03 |
| n_updates | 23249 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 8%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 1441.3219 |
| air_humidity | 13.395819 |
| air_temperature | 23.927961 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 35.25 |
| direct_solar_radiation | 430.5 |
| hour | 16.0 |
| htg_setpoint | 18.0 |
| month | 1.0 |
| outdoor_humidity | 20.0 |
| outdoor_temperature | -0.7346813 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1297189.8 |
| wind_direction | 0.0 |
| wind_speed | 0.525 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.38e+03 |
| n_updates | 23274 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 9%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 843.04126 |
| air_humidity | 27.11858 |
| air_temperature | 23.23063 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 19.0 |
| month | 2.0 |
| outdoor_humidity | 52.75 |
| outdoor_temperature | 6.9470654 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 758737.1 |
| wind_direction | 265.0 |
| wind_speed | 6.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.07e+03 |
| n_updates | 23299 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 9%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 10.769078 |
| air_temperature | 21.700409 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 20.0 |
| month | 2.0 |
| outdoor_humidity | 17.75 |
| outdoor_temperature | 1.4319363 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 340.0 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.7e+03 |
| n_updates | 23324 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 9%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1513.7151 |
| air_humidity | 8.712755 |
| air_temperature | 21.936546 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 22.0 |
| month | 2.0 |
| outdoor_humidity | 57.0 |
| outdoor_temperature | -0.8116667 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1362343.5 |
| wind_direction | 142.5 |
| wind_speed | 3.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.83e+03 |
| n_updates | 23349 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 10%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 1265.1232 |
| air_humidity | 30.580465 |
| air_temperature | 21.114868 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 16.0 |
| month | 2.0 |
| outdoor_humidity | 64.5 |
| outdoor_temperature | 0.4312929 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 1138610.8 |
| wind_direction | 320.0 |
| wind_speed | 7.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.54e+03 |
| n_updates | 23374 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 10%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 3361.0725 |
| air_humidity | 16.331541 |
| air_temperature | 20.00343 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 20.0 |
| month | 2.0 |
| outdoor_humidity | 41.0 |
| outdoor_temperature | -10.048454 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 3024965.2 |
| wind_direction | 305.0 |
| wind_speed | 7.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.35e+04 |
| n_updates | 23399 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 10%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 2836.715 |
| air_humidity | 16.323826 |
| air_temperature | 20.999985 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 21.0 |
| month | 2.0 |
| outdoor_humidity | 46.5 |
| outdoor_temperature | -7.4266663 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2553043.5 |
| wind_direction | 310.0 |
| wind_speed | 8.4 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4e+03 |
| n_updates | 23424 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 10%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 2352.4175 |
| air_humidity | 17.25093 |
| air_temperature | 21.999996 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 22.0 |
| month | 2.0 |
| outdoor_humidity | 45.0 |
| outdoor_temperature | -5.0051785 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2117175.8 |
| wind_direction | 295.0 |
| wind_speed | 3.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.19e+03 |
| n_updates | 23449 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 11%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 2825.996 |
| air_humidity | 16.099987 |
| air_temperature | 20.839624 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 22.0 |
| month | 2.0 |
| outdoor_humidity | 45.5 |
| outdoor_temperature | -7.373072 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2543396.5 |
| wind_direction | 317.5 |
| wind_speed | 7.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.46e+03 |
| n_updates | 23474 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 11%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 1623.5151 |
| air_humidity | 13.141766 |
| air_temperature | 18.081106 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 18.0 |
| month | 2.0 |
| outdoor_humidity | 45.0 |
| outdoor_temperature | -1.360667 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1461163.6 |
| wind_direction | 272.5 |
| wind_speed | 6.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.75e+03 |
| n_updates | 23499 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 11%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 965.93756 |
| air_humidity | 17.687538 |
| air_temperature | 17.630848 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 15.0 |
| month | 2.0 |
| outdoor_humidity | 73.75 |
| outdoor_temperature | 1.9272208 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 869343.75 |
| wind_direction | 250.0 |
| wind_speed | 3.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 823 |
| n_updates | 23524 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 12%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1933.3208 |
| air_humidity | 15.414612 |
| air_temperature | 21.75106 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 22.0 |
| month | 2.0 |
| outdoor_humidity | 30.75 |
| outdoor_temperature | -2.9096956 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1739988.8 |
| wind_direction | 300.0 |
| wind_speed | 5.825 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.87e+03 |
| n_updates | 23549 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 12%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 3003.5579 |
| air_humidity | 14.476208 |
| air_temperature | 18.999998 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 19.0 |
| month | 2.0 |
| outdoor_humidity | 41.5 |
| outdoor_temperature | -8.260881 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2703202.2 |
| wind_direction | 300.0 |
| wind_speed | 5.425 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.39e+03 |
| n_updates | 23574 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 12%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 2032.1776 |
| air_humidity | 15.796214 |
| air_temperature | 21.991514 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 22.0 |
| month | 2.0 |
| outdoor_humidity | 48.5 |
| outdoor_temperature | -3.4039795 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1828959.9 |
| wind_direction | 272.5 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.41e+03 |
| n_updates | 23599 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 13%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1802.0966 |
| air_humidity | 18.37355 |
| air_temperature | 21.000368 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 6.0 |
| htg_setpoint | 21.0 |
| month | 2.0 |
| outdoor_humidity | 70.25 |
| outdoor_temperature | -5.790329 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1621886.9 |
| wind_direction | 77.5 |
| wind_speed | 3.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.84e+03 |
| n_updates | 23624 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 13%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 1535.6776 |
| air_humidity | 38.64689 |
| air_temperature | 18.027721 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 32.25 |
| direct_solar_radiation | 71.25 |
| hour | 7.0 |
| htg_setpoint | 18.0 |
| month | 2.0 |
| outdoor_humidity | 92.0 |
| outdoor_temperature | -0.92147976 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1382109.9 |
| wind_direction | 257.5 |
| wind_speed | 3.725 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 878 |
| n_updates | 23649 |
-------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 13%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1070.4492 |
| air_humidity | 22.329798 |
| air_temperature | 19.0 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 57.5 |
| direct_solar_radiation | 387.0 |
| hour | 8.0 |
| htg_setpoint | 19.0 |
| month | 2.0 |
| outdoor_humidity | 52.75 |
| outdoor_temperature | 1.4046625 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 963404.25 |
| wind_direction | 2.5 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.47e+03 |
| n_updates | 23674 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 13%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 871.7625 |
| air_humidity | 22.375334 |
| air_temperature | 22.626062 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 161.75 |
| direct_solar_radiation | 270.5 |
| hour | 9.0 |
| htg_setpoint | 22.0 |
| month | 2.0 |
| outdoor_humidity | 54.75 |
| outdoor_temperature | 5.4629197 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 784586.25 |
| wind_direction | 290.0 |
| wind_speed | 1.775 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.77e+03 |
| n_updates | 23699 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 14%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 871.7625 |
| air_humidity | 31.798983 |
| air_temperature | 22.695002 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 161.75 |
| direct_solar_radiation | 561.5 |
| hour | 10.0 |
| htg_setpoint | 20.0 |
| month | 2.0 |
| outdoor_humidity | 64.75 |
| outdoor_temperature | 8.719495 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 784586.25 |
| wind_direction | 292.5 |
| wind_speed | 1.775 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.1e+03 |
| n_updates | 23724 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 14%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 755.6465 |
| air_humidity | 21.862947 |
| air_temperature | 23.217703 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 175.75 |
| direct_solar_radiation | 266.5 |
| hour | 11.0 |
| htg_setpoint | 16.0 |
| month | 2.0 |
| outdoor_humidity | 39.5 |
| outdoor_temperature | 7.437775 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 680081.9 |
| wind_direction | 82.5 |
| wind_speed | 3.725 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 9.13e+03 |
| n_updates | 23749 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 14%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 476.10425 |
| air_humidity | 32.07955 |
| air_temperature | 22.814928 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 163.5 |
| direct_solar_radiation | 0.0 |
| hour | 12.0 |
| htg_setpoint | 20.0 |
| month | 2.0 |
| outdoor_humidity | 85.0 |
| outdoor_temperature | 4.6540294 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 428493.84 |
| wind_direction | 2.5 |
| wind_speed | 3.725 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.33e+03 |
| n_updates | 23774 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 14%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 852.2755 |
| air_humidity | 15.134862 |
| air_temperature | 23.507696 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 120.25 |
| direct_solar_radiation | 763.5 |
| hour | 13.0 |
| htg_setpoint | 21.0 |
| month | 2.0 |
| outdoor_humidity | 28.5 |
| outdoor_temperature | 3.5265477 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 767048.0 |
| wind_direction | 310.0 |
| wind_speed | 5.05 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.14e+03 |
| n_updates | 23799 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 15%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 80.00732 |
| air_humidity | 23.061901 |
| air_temperature | 20.99999 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 143.0 |
| direct_solar_radiation | 0.0 |
| hour | 14.0 |
| htg_setpoint | 21.0 |
| month | 2.0 |
| outdoor_humidity | 71.5 |
| outdoor_temperature | 4.6219144 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 72006.586 |
| wind_direction | 145.0 |
| wind_speed | 2.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.98e+03 |
| n_updates | 23824 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 15%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 119.331 |
| air_humidity | 20.039072 |
| air_temperature | 21.185757 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 82.5 |
| direct_solar_radiation | 674.5 |
| hour | 15.0 |
| htg_setpoint | 21.0 |
| month | 2.0 |
| outdoor_humidity | 29.75 |
| outdoor_temperature | 9.234543 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 108005.73 |
| wind_direction | 290.0 |
| wind_speed | 9.3 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.77e+03 |
| n_updates | 23849 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 15%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 17.926476 |
| air_temperature | 23.740973 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 70.75 |
| direct_solar_radiation | 468.5 |
| hour | 16.0 |
| htg_setpoint | 17.0 |
| month | 2.0 |
| outdoor_humidity | 36.0 |
| outdoor_temperature | 3.8430476 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 260.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 890 |
| n_updates | 23874 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 16%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1566.8773 |
| air_humidity | 14.682913 |
| air_temperature | 22.498217 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 18.75 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 21.0 |
| month | 2.0 |
| outdoor_humidity | 27.25 |
| outdoor_temperature | -1.5347023 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1410189.5 |
| wind_direction | 62.5 |
| wind_speed | 3.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.1e+04 |
| n_updates | 23899 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 16%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1056.3701 |
| air_humidity | 33.320927 |
| air_temperature | 22.997578 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 21.0 |
| month | 2.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 1.4750577 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 950733.1 |
| wind_direction | 32.5 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.84e+03 |
| n_updates | 23924 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 16%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 567.25543 |
| air_humidity | 37.674805 |
| air_temperature | 22.00009 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 22.0 |
| month | 2.0 |
| outdoor_humidity | 94.25 |
| outdoor_temperature | 4.278501 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 510529.88 |
| wind_direction | 27.5 |
| wind_speed | 3.725 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.62e+03 |
| n_updates | 23949 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 16%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 361.7099 |
| air_humidity | 28.397816 |
| air_temperature | 21.395718 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 16.0 |
| month | 3.0 |
| outdoor_humidity | 70.0 |
| outdoor_temperature | 6.3793273 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 315658.1 |
| wind_direction | 150.0 |
| wind_speed | 3.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.31e+03 |
| n_updates | 23974 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 17%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 38.01982 |
| air_temperature | 20.494572 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 16.0 |
| month | 3.0 |
| outdoor_humidity | 46.75 |
| outdoor_temperature | 13.6984215 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 292.5 |
| wind_speed | 8.775 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.67e+03 |
| n_updates | 23999 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 17%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 27.335354 |
| air_temperature | 20.544844 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 15.0 |
| month | 3.0 |
| outdoor_humidity | 54.5 |
| outdoor_temperature | 10.299374 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 347.5 |
| wind_speed | 2.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 979 |
| n_updates | 24024 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 17%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 498.51852 |
| air_humidity | 41.83481 |
| air_temperature | 21.420488 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 22.0 |
| month | 3.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 6.471261 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 448666.66 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.87e+03 |
| n_updates | 24049 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 18%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 857.4525 |
| air_humidity | 46.919167 |
| air_temperature | 19.564985 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 21.0 |
| month | 3.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 7.447118 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 819357.75 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.68e+03 |
| n_updates | 24074 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 18%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 34.682133 |
| air_temperature | 18.215752 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 16.0 |
| month | 3.0 |
| outdoor_humidity | 52.75 |
| outdoor_temperature | 7.4204226 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 300.0 |
| wind_speed | 9.675 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 584 |
| n_updates | 24099 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 18%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 39.790886 |
| air_temperature | 17.81009 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 17.0 |
| month | 3.0 |
| outdoor_humidity | 94.25 |
| outdoor_temperature | 4.7941985 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 90.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.16e+03 |
| n_updates | 24124 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 19%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 989.4895 |
| air_humidity | 35.68059 |
| air_temperature | 21.999998 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 22.0 |
| month | 3.0 |
| outdoor_humidity | 99.0 |
| outdoor_temperature | 1.8094609 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 890540.56 |
| wind_direction | 145.0 |
| wind_speed | 2.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1e+03 |
| n_updates | 24149 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 19%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 31.470379 |
| air_temperature | 21.0 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 21.0 |
| month | 3.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 2.564283 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 50.0 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.31e+03 |
| n_updates | 24174 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 19%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 1292.4681 |
| air_humidity | 20.69247 |
| air_temperature | 20.999994 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 21.0 |
| month | 3.0 |
| outdoor_humidity | 59.5 |
| outdoor_temperature | 0.29456785 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1163221.2 |
| wind_direction | 342.5 |
| wind_speed | 8.075 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.95e+03 |
| n_updates | 24199 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 19%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 244.03078 |
| air_humidity | 26.87485 |
| air_temperature | 20.670357 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 6.0 |
| htg_setpoint | 21.0 |
| month | 3.0 |
| outdoor_humidity | 80.0 |
| outdoor_temperature | 2.8225725 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 219627.69 |
| wind_direction | 240.0 |
| wind_speed | 5.125 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.48e+04 |
| n_updates | 24224 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 20%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 537.9154 |
| air_humidity | 17.599335 |
| air_temperature | 18.355534 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 76.5 |
| direct_solar_radiation | 340.5 |
| hour | 7.0 |
| htg_setpoint | 18.0 |
| month | 3.0 |
| outdoor_humidity | 45.0 |
| outdoor_temperature | 0.5305769 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 484123.84 |
| wind_direction | 312.5 |
| wind_speed | 8.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 660 |
| n_updates | 24249 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 20%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1382.9108 |
| air_humidity | 14.891599 |
| air_temperature | 21.999945 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 118.0 |
| direct_solar_radiation | 615.0 |
| hour | 8.0 |
| htg_setpoint | 22.0 |
| month | 3.0 |
| outdoor_humidity | 37.75 |
| outdoor_temperature | -0.5557412 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1244619.6 |
| wind_direction | 50.0 |
| wind_speed | 2.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.38e+03 |
| n_updates | 24274 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 20%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 803.8625 |
| air_humidity | 29.357159 |
| air_temperature | 22.554504 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 130.5 |
| direct_solar_radiation | 737.75 |
| hour | 9.0 |
| htg_setpoint | 20.0 |
| month | 3.0 |
| outdoor_humidity | 49.0 |
| outdoor_temperature | 10.128186 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 739491.4 |
| wind_direction | 270.0 |
| wind_speed | 7.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.57e+03 |
| n_updates | 24299 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 20%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 1323.8452 |
| air_humidity | 16.495691 |
| air_temperature | 17.75022 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 116.25 |
| direct_solar_radiation | 0.0 |
| hour | 10.0 |
| htg_setpoint | 15.0 |
| month | 3.0 |
| outdoor_humidity | 78.5 |
| outdoor_temperature | 0.13768224 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1191460.8 |
| wind_direction | 65.0 |
| wind_speed | 8.525 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.36e+03 |
| n_updates | 24324 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 21%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 1658.1755 |
| air_humidity | 17.395845 |
| air_temperature | 20.233568 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 262.25 |
| direct_solar_radiation | 31.5 |
| hour | 11.0 |
| htg_setpoint | 20.0 |
| month | 3.0 |
| outdoor_humidity | 80.0 |
| outdoor_temperature | -1.5339695 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1492358.0 |
| wind_direction | 32.5 |
| wind_speed | 5.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.94e+03 |
| n_updates | 24349 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 21%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 476.10425 |
| air_humidity | 27.279238 |
| air_temperature | 23.898874 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 263.25 |
| direct_solar_radiation | 613.0 |
| hour | 12.0 |
| htg_setpoint | 16.0 |
| month | 3.0 |
| outdoor_humidity | 79.25 |
| outdoor_temperature | 2.688903 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 428493.84 |
| wind_direction | 195.0 |
| wind_speed | 2.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 886 |
| n_updates | 24374 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 21%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 755.6465 |
| air_humidity | 26.631628 |
| air_temperature | 23.065317 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 161.5 |
| direct_solar_radiation | 9.0 |
| hour | 13.0 |
| htg_setpoint | 17.0 |
| month | 3.0 |
| outdoor_humidity | 76.0 |
| outdoor_temperature | 2.6888297 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 680081.9 |
| wind_direction | 15.0 |
| wind_speed | 7.825 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 497 |
| n_updates | 24399 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 22%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 31.569489 |
| air_temperature | 23.826828 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 131.5 |
| direct_solar_radiation | 803.75 |
| hour | 14.0 |
| htg_setpoint | 22.0 |
| month | 3.0 |
| outdoor_humidity | 71.5 |
| outdoor_temperature | 8.234565 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 180.0 |
| wind_speed | 10.425 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.46e+03 |
| n_updates | 24424 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 22%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 658.0206 |
| air_humidity | 21.673584 |
| air_temperature | 23.873396 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 210.5 |
| direct_solar_radiation | 360.75 |
| hour | 15.0 |
| htg_setpoint | 16.0 |
| month | 3.0 |
| outdoor_humidity | 34.25 |
| outdoor_temperature | 8.975745 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 594968.7 |
| wind_direction | 290.0 |
| wind_speed | 10.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 815 |
| n_updates | 24449 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 22%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1073.464 |
| air_humidity | 13.284251 |
| air_temperature | 23.545315 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 91.5 |
| direct_solar_radiation | 613.0 |
| hour | 16.0 |
| htg_setpoint | 22.0 |
| month | 3.0 |
| outdoor_humidity | 19.5 |
| outdoor_temperature | 1.1647538 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 966117.6 |
| wind_direction | 315.0 |
| wind_speed | 6.575 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 7.58e+03 |
| n_updates | 24474 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 22%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 143.16599 |
| air_humidity | 12.985385 |
| air_temperature | 22.0 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 49.0 |
| direct_solar_radiation | 350.75 |
| hour | 17.0 |
| htg_setpoint | 22.0 |
| month | 3.0 |
| outdoor_humidity | 34.5 |
| outdoor_temperature | 5.93227 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 127358.41 |
| wind_direction | 237.5 |
| wind_speed | 8.65 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.2e+03 |
| n_updates | 24499 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 23%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 22.004717 |
| air_temperature | 21.936619 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 16.0 |
| month | 3.0 |
| outdoor_humidity | 81.25 |
| outdoor_temperature | 5.1236157 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 180.0 |
| wind_speed | 9.3 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.91e+03 |
| n_updates | 24524 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 23%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 370.9882 |
| air_humidity | 43.365337 |
| air_temperature | 22.001156 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 22.0 |
| month | 3.0 |
| outdoor_humidity | 97.0 |
| outdoor_temperature | 6.6044636 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 333889.38 |
| wind_direction | 170.0 |
| wind_speed | 4.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.63e+03 |
| n_updates | 24549 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 23%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 205.3318 |
| air_humidity | 48.651054 |
| air_temperature | 21.7617 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 19.0 |
| month | 3.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 10.614986 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 181480.81 |
| wind_direction | 192.5 |
| wind_speed | 5.175 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 789 |
| n_updates | 24574 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 23%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 52.010227 |
| air_temperature | 23.79372 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 21.0 |
| month | 3.0 |
| outdoor_humidity | 67.5 |
| outdoor_temperature | 16.146664 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 0.0 |
| wind_speed | 8.075 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.17e+03 |
| n_updates | 24599 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 24%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 633.59576 |
| air_humidity | 36.390213 |
| air_temperature | 18.894945 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 16.0 |
| month | 3.0 |
| outdoor_humidity | 67.25 |
| outdoor_temperature | 7.8684926 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 570236.2 |
| wind_direction | 57.5 |
| wind_speed | 7.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.25e+03 |
| n_updates | 24624 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 24%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 40.3879 |
| air_temperature | 20.59343 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 20.0 |
| month | 3.0 |
| outdoor_humidity | 86.0 |
| outdoor_temperature | 3.5267246 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 100.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 924 |
| n_updates | 24649 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 25%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 104.15375 |
| air_humidity | 31.20326 |
| air_temperature | 20.999985 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 21.0 |
| month | 3.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 4.188778 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 93738.375 |
| wind_direction | 32.5 |
| wind_speed | 3.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.16e+03 |
| n_updates | 24674 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 25%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 39.983723 |
| air_temperature | 20.003113 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 22.0 |
| month | 4.0 |
| outdoor_humidity | 70.25 |
| outdoor_temperature | 3.8033586 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 35.0 |
| wind_speed | 3.65 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.05e+03 |
| n_updates | 24699 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 25%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 69.221664 |
| air_temperature | 19.668886 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 19.0 |
| month | 4.0 |
| outdoor_humidity | 85.0 |
| outdoor_temperature | 4.3165517 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 272.5 |
| wind_speed | 5.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.23e+03 |
| n_updates | 24724 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 25%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 54.650764 |
| air_temperature | 19.165148 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 22.0 |
| month | 4.0 |
| outdoor_humidity | 55.5 |
| outdoor_temperature | 2.5306246 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 322.5 |
| wind_speed | 4.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 8.58e+03 |
| n_updates | 24749 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 26%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 54.53965 |
| air_temperature | 19.157383 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 19.0 |
| month | 4.0 |
| outdoor_humidity | 71.25 |
| outdoor_temperature | 4.457339 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 237.5 |
| wind_speed | 4.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.91e+03 |
| n_updates | 24774 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 26%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 59.95734 |
| air_temperature | 19.35088 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 16.0 |
| month | 4.0 |
| outdoor_humidity | 61.0 |
| outdoor_temperature | 6.4295363 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 220.0 |
| wind_speed | 3.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.42e+03 |
| n_updates | 24799 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 26%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 82.47406 |
| air_temperature | 20.338148 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 29.0 |
| direct_solar_radiation | 369.25 |
| hour | 6.0 |
| htg_setpoint | 15.0 |
| month | 4.0 |
| outdoor_humidity | 95.0 |
| outdoor_temperature | 7.9402747 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 275.0 |
| wind_speed | 2.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.86e+03 |
| n_updates | 24824 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 26%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 79.3464 |
| air_temperature | 20.925404 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 121.0 |
| direct_solar_radiation | 356.75 |
| hour | 7.0 |
| htg_setpoint | 15.0 |
| month | 4.0 |
| outdoor_humidity | 61.5 |
| outdoor_temperature | 14.273043 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 225.0 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.2e+03 |
| n_updates | 24849 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 27%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 2664.3604 |
| air_humidity | 63.509575 |
| air_temperature | 21.99787 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 154.75 |
| direct_solar_radiation | 452.0 |
| hour | 8.0 |
| htg_setpoint | 22.0 |
| month | 4.0 |
| outdoor_humidity | 57.0 |
| outdoor_temperature | 20.487545 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 2375066.5 |
| wind_direction | 225.0 |
| wind_speed | 2.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 8.68e+03 |
| n_updates | 24874 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 27%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 3005.8865 |
| air_humidity | 62.8656 |
| air_temperature | 22.20208 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 218.5 |
| direct_solar_radiation | 174.75 |
| hour | 9.0 |
| htg_setpoint | 15.0 |
| month | 4.0 |
| outdoor_humidity | 71.25 |
| outdoor_temperature | 20.12474 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 2684761.2 |
| wind_direction | 192.5 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.64e+04 |
| n_updates | 24899 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 27%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 3164.7615 |
| air_humidity | 54.197342 |
| air_temperature | 23.086617 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 263.25 |
| direct_solar_radiation | 404.75 |
| hour | 10.0 |
| htg_setpoint | 22.0 |
| month | 4.0 |
| outdoor_humidity | 44.25 |
| outdoor_temperature | 20.261744 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 2821500.5 |
| wind_direction | 270.0 |
| wind_speed | 9.675 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.54e+03 |
| n_updates | 24924 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 28%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 17.615269 |
| air_temperature | 23.11328 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 177.25 |
| direct_solar_radiation | 827.75 |
| hour | 11.0 |
| htg_setpoint | 22.0 |
| month | 4.0 |
| outdoor_humidity | 21.5 |
| outdoor_temperature | 10.584083 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 315.0 |
| wind_speed | 10.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.75e+03 |
| n_updates | 24949 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 28%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 438.20398 |
| air_humidity | 14.780738 |
| air_temperature | 23.99957 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 110.0 |
| direct_solar_radiation | 941.5 |
| hour | 12.0 |
| htg_setpoint | 21.0 |
| month | 4.0 |
| outdoor_humidity | 17.25 |
| outdoor_temperature | 9.602093 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 397929.97 |
| wind_direction | 352.5 |
| wind_speed | 6.075 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.03e+03 |
| n_updates | 24974 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 28%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 33.816364 |
| air_temperature | 21.155039 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 229.75 |
| direct_solar_radiation | 0.0 |
| hour | 13.0 |
| htg_setpoint | 17.0 |
| month | 4.0 |
| outdoor_humidity | 66.25 |
| outdoor_temperature | 8.980941 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 175.0 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.66e+03 |
| n_updates | 24999 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 28%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 31.091959 |
| air_temperature | 22.514444 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 190.75 |
| direct_solar_radiation | 620.25 |
| hour | 14.0 |
| htg_setpoint | 15.0 |
| month | 4.0 |
| outdoor_humidity | 50.0 |
| outdoor_temperature | 10.923158 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 137.5 |
| wind_speed | 6.325 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.03e+03 |
| n_updates | 25024 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 29%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 456.93237 |
| air_humidity | 48.886036 |
| air_temperature | 23.283798 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 110.5 |
| direct_solar_radiation | 0.0 |
| hour | 15.0 |
| htg_setpoint | 20.0 |
| month | 4.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 9.940544 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 411239.16 |
| wind_direction | 107.5 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.84e+03 |
| n_updates | 25049 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 29%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 4053.9346 |
| air_humidity | 47.16852 |
| air_temperature | 23.773457 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 144.25 |
| direct_solar_radiation | 458.75 |
| hour | 16.0 |
| htg_setpoint | 22.0 |
| month | 4.0 |
| outdoor_humidity | 42.25 |
| outdoor_temperature | 21.774252 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 3648541.2 |
| wind_direction | 255.0 |
| wind_speed | 7.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.2e+03 |
| n_updates | 25074 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 29%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 44.031296 |
| air_temperature | 25.765486 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 47.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 19.0 |
| month | 4.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 9.873235 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 87.5 |
| wind_speed | 5.575 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.75e+04 |
| n_updates | 25099 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 29%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 35.823162 |
| air_temperature | 27.340607 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 15.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 18.0 |
| month | 4.0 |
| outdoor_humidity | 67.5 |
| outdoor_temperature | 8.295609 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 52.5 |
| wind_speed | 4.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.5e+03 |
| n_updates | 25124 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 30%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 38.977745 |
| air_temperature | 27.899288 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 16.0 |
| month | 4.0 |
| outdoor_humidity | 57.75 |
| outdoor_temperature | 7.2945585 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 157.5 |
| wind_speed | 3.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.95e+03 |
| n_updates | 25149 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 30%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 63.1766 |
| air_temperature | 20.729017 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 17.0 |
| month | 4.0 |
| outdoor_humidity | 76.25 |
| outdoor_temperature | 10.415078 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 70.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 952 |
| n_updates | 25174 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 30%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 67.9957 |
| air_temperature | 19.192297 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 22.0 |
| month | 4.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 7.950842 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 257.5 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.93e+03 |
| n_updates | 25199 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 31%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 55.464287 |
| air_temperature | 22.169394 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 18.0 |
| month | 4.0 |
| outdoor_humidity | 66.0 |
| outdoor_temperature | 8.823215 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 237.5 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 492 |
| n_updates | 25224 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 31%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 58.59591 |
| air_temperature | 22.65728 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 20.0 |
| month | 4.0 |
| outdoor_humidity | 80.75 |
| outdoor_temperature | 10.0935545 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 230.0 |
| wind_speed | 2.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.77e+03 |
| n_updates | 25249 |
------------------------------------------------
--------------------------------------------------------------------------------------------------------------| 31%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 80.50592 |
| air_temperature | 21.325115 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 19.0 |
| month | 4.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 8.709449 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 102.5 |
| wind_speed | 1.9 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.82e+03 |
| n_updates | 25274 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 32%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 67.27389 |
| air_temperature | 21.735031 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 16.0 |
| month | 4.0 |
| outdoor_humidity | 83.0 |
| outdoor_temperature | 9.947844 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 70.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.33e+03 |
| n_updates | 25299 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 32%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 66.82053 |
| air_temperature | 21.900738 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 21.0 |
| month | 4.0 |
| outdoor_humidity | 83.0 |
| outdoor_temperature | 13.363319 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 235.0 |
| wind_speed | 3.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.59e+03 |
| n_updates | 25324 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 32%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 68.47042 |
| air_temperature | 21.461578 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 22.0 |
| month | 4.0 |
| outdoor_humidity | 79.5 |
| outdoor_temperature | 14.763615 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 47.5 |
| wind_speed | 5.325 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.85e+03 |
| n_updates | 25349 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 32%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 76.52933 |
| air_temperature | 19.80769 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 21.0 |
| month | 4.0 |
| outdoor_humidity | 76.25 |
| outdoor_temperature | 10.0389 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 102.5 |
| wind_speed | 4.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.23e+03 |
| n_updates | 25374 |
----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 33%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 77.56004 |
| air_temperature | 19.754734 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 12.75 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 22.0 |
| month | 4.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 12.546379 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 110.0 |
| wind_speed | 2.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.05e+03 |
| n_updates | 25399 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 33%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 100.0 |
| air_temperature | 19.873129 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 99.75 |
| direct_solar_radiation | 283.5 |
| hour | 6.0 |
| htg_setpoint | 16.0 |
| month | 5.0 |
| outdoor_humidity | 68.0 |
| outdoor_temperature | 8.822796 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 30.0 |
| wind_speed | 4.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.38e+03 |
| n_updates | 25424 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 33%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 250.6906 |
| air_humidity | 60.83376 |
| air_temperature | 21.001799 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 151.5 |
| direct_solar_radiation | 495.25 |
| hour | 7.0 |
| htg_setpoint | 21.0 |
| month | 5.0 |
| outdoor_humidity | 42.75 |
| outdoor_temperature | 13.935489 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 226307.55 |
| wind_direction | 35.0 |
| wind_speed | 6.325 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.92e+03 |
| n_updates | 25449 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 34%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 352.57874 |
| air_humidity | 51.372105 |
| air_temperature | 21.999958 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 189.75 |
| direct_solar_radiation | 4.5 |
| hour | 8.0 |
| htg_setpoint | 22.0 |
| month | 5.0 |
| outdoor_humidity | 77.0 |
| outdoor_temperature | 12.262045 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 326594.3 |
| wind_direction | 40.0 |
| wind_speed | 10.55 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.52e+03 |
| n_updates | 25474 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 34%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 70.50618 |
| air_temperature | 20.628372 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 129.5 |
| direct_solar_radiation | 0.0 |
| hour | 9.0 |
| htg_setpoint | 20.0 |
| month | 5.0 |
| outdoor_humidity | 96.25 |
| outdoor_temperature | 13.166679 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 15.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.95e+03 |
| n_updates | 25499 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 34%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 66.167046 |
| air_temperature | 21.691072 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 309.25 |
| direct_solar_radiation | 31.5 |
| hour | 10.0 |
| htg_setpoint | 18.0 |
| month | 5.0 |
| outdoor_humidity | 87.75 |
| outdoor_temperature | 16.63086 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 200.0 |
| wind_speed | 3.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 676 |
| n_updates | 25524 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 34%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 410.55798 |
| air_humidity | 54.012146 |
| air_temperature | 25.636631 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 346.75 |
| direct_solar_radiation | 16.5 |
| hour | 11.0 |
| htg_setpoint | 18.0 |
| month | 5.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | 15.807955 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 369502.8 |
| wind_direction | 140.0 |
| wind_speed | 3.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.45e+03 |
| n_updates | 25549 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 35%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 651.70325 |
| air_humidity | 58.94949 |
| air_temperature | 22.544811 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 143.5 |
| direct_solar_radiation | 0.0 |
| hour | 12.0 |
| htg_setpoint | 22.0 |
| month | 5.0 |
| outdoor_humidity | 99.25 |
| outdoor_temperature | 13.337481 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 586532.94 |
| wind_direction | 77.5 |
| wind_speed | 5.325 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.48e+03 |
| n_updates | 25574 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 35%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 1041.8875 |
| air_humidity | 57.804943 |
| air_temperature | 24.998793 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 263.75 |
| direct_solar_radiation | 10.5 |
| hour | 13.0 |
| htg_setpoint | 20.0 |
| month | 5.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 16.276424 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 937698.75 |
| wind_direction | 122.5 |
| wind_speed | 4.8 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.19e+03 |
| n_updates | 25599 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 35%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 3808.2505 |
| air_humidity | 51.37692 |
| air_temperature | 23.888063 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 365.75 |
| direct_solar_radiation | 196.25 |
| hour | 14.0 |
| htg_setpoint | 17.0 |
| month | 5.0 |
| outdoor_humidity | 46.5 |
| outdoor_temperature | 23.000093 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 3427425.5 |
| wind_direction | 232.5 |
| wind_speed | 7.175 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.26e+03 |
| n_updates | 25624 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 35%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 4303.7583 |
| air_humidity | 42.214954 |
| air_temperature | 24.2613 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 168.5 |
| direct_solar_radiation | 652.5 |
| hour | 15.0 |
| htg_setpoint | 17.0 |
| month | 5.0 |
| outdoor_humidity | 34.25 |
| outdoor_temperature | 23.414637 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 3870570.8 |
| wind_direction | 337.5 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 950 |
| n_updates | 25649 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 36%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 55.714012 |
| air_temperature | 26.159508 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 129.5 |
| direct_solar_radiation | 608.75 |
| hour | 16.0 |
| htg_setpoint | 19.0 |
| month | 5.0 |
| outdoor_humidity | 61.75 |
| outdoor_temperature | 13.151306 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 180.0 |
| wind_speed | 7.325 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.61e+03 |
| n_updates | 25674 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 36%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 51.74679 |
| air_temperature | 27.251833 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 106.25 |
| direct_solar_radiation | 385.25 |
| hour | 17.0 |
| htg_setpoint | 17.0 |
| month | 5.0 |
| outdoor_humidity | 49.0 |
| outdoor_temperature | 20.827276 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 140.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.05e+03 |
| n_updates | 25699 |
-----------------------------------------------
--------------------------------------------------------------------------------------------------------------| 36%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 30.98147 |
| air_temperature | 28.937086 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 46.0 |
| direct_solar_radiation | 185.5 |
| hour | 18.0 |
| htg_setpoint | 19.0 |
| month | 5.0 |
| outdoor_humidity | 51.5 |
| outdoor_temperature | 13.358186 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 130.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.12e+03 |
| n_updates | 25724 |
-----------------------------------------------
-----------------------------------------------*--------------------------------------------------------------| 37%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 41.291485 |
| air_temperature | 29.252293 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 21.0 |
| month | 5.0 |
| outdoor_humidity | 59.5 |
| outdoor_temperature | 9.602841 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 142.5 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.03e+03 |
| n_updates | 25749 |
-----------------------------------------------
-----------------------------------------------*--------------------------------------------------------------| 37%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 45.470722 |
| air_temperature | 26.394222 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 22.0 |
| month | 5.0 |
| outdoor_humidity | 54.5 |
| outdoor_temperature | 10.277687 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 77.5 |
| wind_speed | 1.65 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.65e+03 |
| n_updates | 25774 |
-----------------------------------------------
-----------------------------------------------*--------------------------------------------------------------| 37%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 50.822987 |
| air_temperature | 25.489048 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 15.0 |
| month | 5.0 |
| outdoor_humidity | 74.75 |
| outdoor_temperature | 12.156735 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 80.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.18e+04 |
| n_updates | 25799 |
-----------------------------------------------
-----------------------------------------------*--------------------------------------------------------------| 37%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 63.22203 |
| air_temperature | 24.64449 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 18.0 |
| month | 5.0 |
| outdoor_humidity | 84.0 |
| outdoor_temperature | 13.052027 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 72.5 |
| wind_speed | 2.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 8.12e+03 |
| n_updates | 25824 |
-----------------------------------------------
-----------------------------------------------**-------------------------------------------------------------| 38%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 72.95142 |
| air_temperature | 22.187042 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 20.0 |
| month | 5.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 15.178462 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 100.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.91e+03 |
| n_updates | 25849 |
-----------------------------------------------
-----------------------------------------------**-------------------------------------------------------------| 38%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 75.1022 |
| air_temperature | 21.650509 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 18.0 |
| month | 5.0 |
| outdoor_humidity | 95.25 |
| outdoor_temperature | 15.624398 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 332.5 |
| wind_speed | 5.575 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.52e+03 |
| n_updates | 25874 |
-----------------------------------------------
-----------------------------------------------**-------------------------------------------------------------| 38%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 61.087856 |
| air_temperature | 22.81011 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 21.0 |
| month | 5.0 |
| outdoor_humidity | 45.25 |
| outdoor_temperature | 13.491618 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 340.0 |
| wind_speed | 4.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.78e+03 |
| n_updates | 25899 |
-----------------------------------------------
-----------------------------------------------***------------------------------------------------------------| 39%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 73.322044 |
| air_temperature | 21.77929 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 16.0 |
| month | 5.0 |
| outdoor_humidity | 78.75 |
| outdoor_temperature | 14.339748 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 45.0 |
| wind_speed | 1.125 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.21e+03 |
| n_updates | 25924 |
-----------------------------------------------
-----------------------------------------------***------------------------------------------------------------| 39%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 73.95429 |
| air_temperature | 21.417715 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 21.0 |
| month | 5.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 15.883621 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 92.5 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 998 |
| n_updates | 25949 |
-----------------------------------------------
-----------------------------------------------***------------------------------------------------------------| 39%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 100.0 |
| air_temperature | 21.256157 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 20.0 |
| month | 5.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 15.279231 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 97.5 |
| wind_speed | 2.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.46e+03 |
| n_updates | 25974 |
-----------------------------------------------
-----------------------------------------------****-----------------------------------------------------------| 40%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 100.0 |
| air_temperature | 21.208662 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 63.75 |
| direct_solar_radiation | 114.75 |
| hour | 5.0 |
| htg_setpoint | 22.0 |
| month | 5.0 |
| outdoor_humidity | 76.5 |
| outdoor_temperature | 12.422109 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 225.0 |
| wind_speed | 5.325 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.5e+03 |
| n_updates | 25999 |
-----------------------------------------------
-----------------------------------------------****-----------------------------------------------------------| 40%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 96.97581 |
| air_temperature | 21.713926 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 127.0 |
| direct_solar_radiation | 287.75 |
| hour | 6.0 |
| htg_setpoint | 22.0 |
| month | 5.0 |
| outdoor_humidity | 59.5 |
| outdoor_temperature | 16.465273 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 220.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.34e+03 |
| n_updates | 26024 |
-----------------------------------------------
-----------------------------------------------****-----------------------------------------------------------| 40%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 187.26651 |
| air_humidity | 92.392784 |
| air_temperature | 20.754955 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 165.25 |
| direct_solar_radiation | 485.0 |
| hour | 7.0 |
| htg_setpoint | 15.0 |
| month | 5.0 |
| outdoor_humidity | 64.75 |
| outdoor_temperature | 15.890859 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 168539.34 |
| wind_direction | 315.0 |
| wind_speed | 4.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.71e+03 |
| n_updates | 26049 |
-----------------------------------------------
-----------------------------------------------****-----------------------------------------------------------| 40%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 2839.5784 |
| air_humidity | 52.345562 |
| air_temperature | 21.531103 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 176.75 |
| direct_solar_radiation | 652.25 |
| hour | 8.0 |
| htg_setpoint | 17.0 |
| month | 5.0 |
| outdoor_humidity | 43.0 |
| outdoor_temperature | 19.804117 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 2523309.0 |
| wind_direction | 317.5 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.98e+03 |
| n_updates | 26074 |
-----------------------------------------------
-----------------------------------------------*****----------------------------------------------------------| 41%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 3925.5715 |
| air_humidity | 57.477875 |
| air_temperature | 22.937943 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 187.25 |
| direct_solar_radiation | 717.0 |
| hour | 9.0 |
| htg_setpoint | 21.0 |
| month | 5.0 |
| outdoor_humidity | 38.75 |
| outdoor_temperature | 24.464203 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 3504417.8 |
| wind_direction | 237.5 |
| wind_speed | 3.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.22e+03 |
| n_updates | 26099 |
-----------------------------------------------
-----------------------------------------------*****----------------------------------------------------------| 41%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 4254.275 |
| air_humidity | 52.1896 |
| air_temperature | 23.768286 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 245.25 |
| direct_solar_radiation | 677.0 |
| hour | 10.0 |
| htg_setpoint | 17.0 |
| month | 5.0 |
| outdoor_humidity | 35.25 |
| outdoor_temperature | 26.724548 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 3828847.2 |
| wind_direction | 327.5 |
| wind_speed | 2.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.93e+03 |
| n_updates | 26124 |
-----------------------------------------------
-----------------------------------------------*****----------------------------------------------------------| 41%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 4908.532 |
| air_humidity | 58.187576 |
| air_temperature | 24.125984 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 272.25 |
| direct_solar_radiation | 681.0 |
| hour | 11.0 |
| htg_setpoint | 19.0 |
| month | 5.0 |
| outdoor_humidity | 59.5 |
| outdoor_temperature | 25.087038 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 4400032.0 |
| wind_direction | 147.5 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.15e+03 |
| n_updates | 26149 |
-----------------------------------------------
-----------------------------------------------*****----------------------------------------------------------| 41%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 70.08793 |
| air_temperature | 25.162493 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 214.5 |
| direct_solar_radiation | 742.5 |
| hour | 12.0 |
| htg_setpoint | 22.0 |
| month | 6.0 |
| outdoor_humidity | 49.75 |
| outdoor_temperature | 27.204876 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 250.0 |
| wind_speed | 6.825 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.46e+03 |
| n_updates | 26174 |
-----------------------------------------------
-----------------------------------------------******---------------------------------------------------------| 42%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 69.17672 |
| air_temperature | 25.449238 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 120.25 |
| direct_solar_radiation | 868.75 |
| hour | 13.0 |
| htg_setpoint | 22.0 |
| month | 6.0 |
| outdoor_humidity | 31.75 |
| outdoor_temperature | 19.77673 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 297.5 |
| wind_speed | 5.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.82e+03 |
| n_updates | 26199 |
-----------------------------------------------
-----------------------------------------------******---------------------------------------------------------| 42%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 4341.2114 |
| air_humidity | 47.581272 |
| air_temperature | 24.12907 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 164.25 |
| direct_solar_radiation | 792.0 |
| hour | 14.0 |
| htg_setpoint | 20.0 |
| month | 6.0 |
| outdoor_humidity | 44.0 |
| outdoor_temperature | 22.831139 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 3903533.5 |
| wind_direction | 217.5 |
| wind_speed | 6.075 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.91e+03 |
| n_updates | 26224 |
-----------------------------------------------
-----------------------------------------------******---------------------------------------------------------| 42%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 4636.2915 |
| air_humidity | 40.08801 |
| air_temperature | 24.42213 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 249.5 |
| direct_solar_radiation | 492.25 |
| hour | 15.0 |
| htg_setpoint | 19.0 |
| month | 6.0 |
| outdoor_humidity | 32.5 |
| outdoor_temperature | 24.683224 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 4171513.5 |
| wind_direction | 192.5 |
| wind_speed | 5.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.36e+03 |
| n_updates | 26249 |
-----------------------------------------------
-----------------------------------------------*******--------------------------------------------------------| 43%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 3491.4077 |
| air_humidity | 55.89672 |
| air_temperature | 23.679293 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 170.25 |
| direct_solar_radiation | 0.0 |
| hour | 16.0 |
| htg_setpoint | 21.0 |
| month | 6.0 |
| outdoor_humidity | 59.0 |
| outdoor_temperature | 19.496178 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 3150081.5 |
| wind_direction | 177.5 |
| wind_speed | 6.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 9.49e+03 |
| n_updates | 26274 |
-----------------------------------------------
-----------------------------------------------*******--------------------------------------------------------| 43%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 55.47146 |
| air_temperature | 25.53944 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 94.25 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 22.0 |
| month | 6.0 |
| outdoor_humidity | 88.5 |
| outdoor_temperature | 18.309011 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 8.8 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.43e+03 |
| n_updates | 26299 |
-----------------------------------------------
-----------------------------------------------*******--------------------------------------------------------| 43%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 47.804707 |
| air_temperature | 30.097784 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 70.0 |
| direct_solar_radiation | 78.0 |
| hour | 18.0 |
| htg_setpoint | 21.0 |
| month | 6.0 |
| outdoor_humidity | 43.5 |
| outdoor_temperature | 27.872309 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 0.0 |
| wind_speed | 6.575 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.37e+03 |
| n_updates | 26324 |
-----------------------------------------------
----------------------------------------------********--------------------------------------------------------| 43%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 67.28057 |
| air_temperature | 26.83315 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 12.25 |
| direct_solar_radiation | 6.5 |
| hour | 19.0 |
| htg_setpoint | 15.0 |
| month | 6.0 |
| outdoor_humidity | 48.75 |
| outdoor_temperature | 20.32014 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 50.0 |
| wind_speed | 3.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.02e+04 |
| n_updates | 26349 |
----------------------------------------------
-----------------------------------------------********-------------------------------------------------------| 44%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 69.60585 |
| air_temperature | 26.294132 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 21.0 |
| month | 6.0 |
| outdoor_humidity | 70.25 |
| outdoor_temperature | 17.06836 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 200.0 |
| wind_speed | 3.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.91e+03 |
| n_updates | 26374 |
-----------------------------------------------
-----------------------------------------------********-------------------------------------------------------| 44%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 62.328117 |
| air_temperature | 26.36409 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 15.0 |
| month | 6.0 |
| outdoor_humidity | 80.0 |
| outdoor_temperature | 17.44078 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 170.0 |
| wind_speed | 3.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 7.16e+03 |
| n_updates | 26399 |
-----------------------------------------------
-----------------------------------------------********-------------------------------------------------------| 44%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 78.79806 |
| air_temperature | 24.133389 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 21.0 |
| month | 6.0 |
| outdoor_humidity | 90.0 |
| outdoor_temperature | 19.43842 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 162.5 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.15e+03 |
| n_updates | 26424 |
-----------------------------------------------
-----------------------------------------------********-------------------------------------------------------| 44%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 80.323395 |
| air_temperature | 23.736319 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 20.0 |
| month | 6.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 19.34564 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 200.0 |
| wind_speed | 5.575 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.8e+03 |
| n_updates | 26449 |
-----------------------------------------------
-----------------------------------------------*********------------------------------------------------------| 45%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 81.02745 |
| air_temperature | 23.606394 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 21.0 |
| month | 6.0 |
| outdoor_humidity | 99.0 |
| outdoor_temperature | 19.023228 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 200.0 |
| wind_speed | 6.075 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 658 |
| n_updates | 26474 |
-----------------------------------------------
-----------------------------------------------*********------------------------------------------------------| 45%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 82.58435 |
| air_temperature | 23.921928 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 21.0 |
| month | 6.0 |
| outdoor_humidity | 87.0 |
| outdoor_temperature | 21.423105 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 60.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.94e+03 |
| n_updates | 26499 |
-----------------------------------------------
-----------------------------------------------**********-----------------------------------------------------| 46%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 80.42138 |
| air_temperature | 24.429708 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 21.0 |
| month | 6.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 21.647804 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 92.5 |
| wind_speed | 3.725 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.49e+03 |
| n_updates | 26524 |
-----------------------------------------------
-----------------------------------------------**********-----------------------------------------------------| 46%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 90.55395 |
| air_temperature | 22.446527 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 20.0 |
| month | 6.0 |
| outdoor_humidity | 98.25 |
| outdoor_temperature | 19.192326 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 142.5 |
| wind_speed | 1.5 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.04e+03 |
| n_updates | 26549 |
-----------------------------------------------
-----------------------------------------------**********-----------------------------------------------------| 46%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 86.08581 |
| air_temperature | 22.69578 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 18.0 |
| month | 6.0 |
| outdoor_humidity | 97.0 |
| outdoor_temperature | 22.023277 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 230.0 |
| wind_speed | 2.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.15e+03 |
| n_updates | 26574 |
-----------------------------------------------
-----------------------------------------------**********-----------------------------------------------------| 46%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 87.03985 |
| air_temperature | 22.502487 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 48.5 |
| direct_solar_radiation | 192.75 |
| hour | 5.0 |
| htg_setpoint | 17.0 |
| month | 6.0 |
| outdoor_humidity | 84.0 |
| outdoor_temperature | 22.397675 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 272.5 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.58e+03 |
| n_updates | 26599 |
-----------------------------------------------
-----------------------------------------------***********----------------------------------------------------| 47%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 87.01041 |
| air_temperature | 22.92337 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 115.0 |
| direct_solar_radiation | 271.0 |
| hour | 6.0 |
| htg_setpoint | 18.0 |
| month | 6.0 |
| outdoor_humidity | 75.25 |
| outdoor_temperature | 20.942945 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 50.0 |
| wind_speed | 4.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.78e+03 |
| n_updates | 26624 |
-----------------------------------------------
-----------------------------------------------***********----------------------------------------------------| 47%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 1697.0332 |
| air_humidity | 83.39393 |
| air_temperature | 20.575014 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 98.5 |
| direct_solar_radiation | 0.0 |
| hour | 7.0 |
| htg_setpoint | 15.0 |
| month | 6.0 |
| outdoor_humidity | 90.0 |
| outdoor_temperature | 20.522898 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1457054.8 |
| wind_direction | 212.5 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.29e+03 |
| n_updates | 26649 |
-----------------------------------------------
-----------------------------------------------***********----------------------------------------------------| 47%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 74.909515 |
| air_temperature | 24.961433 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 124.5 |
| direct_solar_radiation | 684.25 |
| hour | 8.0 |
| htg_setpoint | 20.0 |
| month | 6.0 |
| outdoor_humidity | 42.0 |
| outdoor_temperature | 24.839106 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 347.5 |
| wind_speed | 5.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.31e+03 |
| n_updates | 26674 |
-----------------------------------------------
-----------------------------------------------***********----------------------------------------------------| 47%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 73.118645 |
| air_temperature | 25.401157 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 310.5 |
| direct_solar_radiation | 442.0 |
| hour | 9.0 |
| htg_setpoint | 22.0 |
| month | 6.0 |
| outdoor_humidity | 43.25 |
| outdoor_temperature | 27.892473 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 282.5 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 468 |
| n_updates | 26699 |
-----------------------------------------------
-----------------------------------------------************---------------------------------------------------| 48%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 7243.911 |
| air_humidity | 60.207127 |
| air_temperature | 23.405018 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 436.0 |
| direct_solar_radiation | 309.5 |
| hour | 10.0 |
| htg_setpoint | 19.0 |
| month | 6.0 |
| outdoor_humidity | 76.25 |
| outdoor_temperature | 26.610846 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 6482271.0 |
| wind_direction | 142.5 |
| wind_speed | 5.05 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.21e+03 |
| n_updates | 26724 |
-----------------------------------------------
-----------------------------------------------************---------------------------------------------------| 48%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 6050.424 |
| air_humidity | 58.90546 |
| air_temperature | 23.9165 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 290.5 |
| direct_solar_radiation | 425.25 |
| hour | 11.0 |
| htg_setpoint | 19.0 |
| month | 6.0 |
| outdoor_humidity | 80.75 |
| outdoor_temperature | 24.506327 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 5445381.5 |
| wind_direction | 197.5 |
| wind_speed | 5.325 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 635 |
| n_updates | 26749 |
-----------------------------------------------
-----------------------------------------------************---------------------------------------------------| 48%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 5802.945 |
| air_humidity | 56.61357 |
| air_temperature | 24.26556 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 195.0 |
| direct_solar_radiation | 756.5 |
| hour | 12.0 |
| htg_setpoint | 20.0 |
| month | 6.0 |
| outdoor_humidity | 57.0 |
| outdoor_temperature | 27.554426 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 5239022.0 |
| wind_direction | 190.0 |
| wind_speed | 5.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.57e+03 |
| n_updates | 26774 |
-----------------------------------------------
-----------------------------------------------*************--------------------------------------------------| 49%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 5660.5815 |
| air_humidity | 57.0777 |
| air_temperature | 24.114191 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 274.75 |
| direct_solar_radiation | 610.0 |
| hour | 13.0 |
| htg_setpoint | 15.0 |
| month | 6.0 |
| outdoor_humidity | 68.25 |
| outdoor_temperature | 25.911041 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 5093937.0 |
| wind_direction | 187.5 |
| wind_speed | 9.925 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.18e+03 |
| n_updates | 26799 |
-----------------------------------------------
-----------------------------------------------*************--------------------------------------------------| 49%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 5907.6895 |
| air_humidity | 57.608673 |
| air_temperature | 24.191023 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 319.25 |
| direct_solar_radiation | 279.5 |
| hour | 14.0 |
| htg_setpoint | 15.0 |
| month | 6.0 |
| outdoor_humidity | 79.75 |
| outdoor_temperature | 23.198372 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 5316920.5 |
| wind_direction | 215.0 |
| wind_speed | 6.575 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.77e+03 |
| n_updates | 26824 |
-----------------------------------------------
-----------------------------------------------*************--------------------------------------------------| 49%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 79.9303 |
| air_temperature | 24.091045 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 244.75 |
| direct_solar_radiation | 0.0 |
| hour | 15.0 |
| htg_setpoint | 18.0 |
| month | 6.0 |
| outdoor_humidity | 80.5 |
| outdoor_temperature | 21.664352 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 117.5 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 7.95e+03 |
| n_updates | 26849 |
-----------------------------------------------
-----------------------------------------------*************--------------------------------------------------| 49%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 69.5355 |
| air_temperature | 26.462065 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 293.25 |
| direct_solar_radiation | 91.5 |
| hour | 16.0 |
| htg_setpoint | 22.0 |
| month | 6.0 |
| outdoor_humidity | 81.75 |
| outdoor_temperature | 21.446198 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 192.5 |
| wind_speed | 8.4 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.9e+03 |
| n_updates | 26874 |
-----------------------------------------------
-----------------------------------------------**************-------------------------------------------------| 50%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 52.676174 |
| air_temperature | 26.86975 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 88.75 |
| direct_solar_radiation | 543.5 |
| hour | 17.0 |
| htg_setpoint | 19.0 |
| month | 7.0 |
| outdoor_humidity | 79.0 |
| outdoor_temperature | 25.234293 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.91e+03 |
| n_updates | 26899 |
-----------------------------------------------
-----------------------------------------------**************-------------------------------------------------| 50%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 49.196945 |
| air_temperature | 29.72702 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 57.0 |
| direct_solar_radiation | 78.75 |
| hour | 18.0 |
| htg_setpoint | 18.0 |
| month | 7.0 |
| outdoor_humidity | 81.75 |
| outdoor_temperature | 21.81109 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 195.0 |
| wind_speed | 7.575 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3e+03 |
| n_updates | 26924 |
-----------------------------------------------
-----------------------------------------------**************-------------------------------------------------| 50%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 59.365803 |
| air_temperature | 28.91152 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 24.75 |
| direct_solar_radiation | 5.25 |
| hour | 19.0 |
| htg_setpoint | 18.0 |
| month | 7.0 |
| outdoor_humidity | 73.0 |
| outdoor_temperature | 23.71026 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 275.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.94e+03 |
| n_updates | 26949 |
-----------------------------------------------
-----------------------------------------------**************-------------------------------------------------| 50%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 60.808823 |
| air_temperature | 28.34431 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 22.0 |
| month | 7.0 |
| outdoor_humidity | 67.75 |
| outdoor_temperature | 22.228575 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 102.5 |
| wind_speed | 2.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.39e+03 |
| n_updates | 26974 |
-----------------------------------------------
-----------------------------------------------***************------------------------------------------------| 51%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 66.46324 |
| air_temperature | 27.034254 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 17.0 |
| month | 7.0 |
| outdoor_humidity | 93.75 |
| outdoor_temperature | 21.445435 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 197.5 |
| wind_speed | 6.05 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.23e+03 |
| n_updates | 26999 |
-----------------------------------------------
-----------------------------------------------***************------------------------------------------------| 51%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 68.088394 |
| air_temperature | 26.545113 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 19.0 |
| month | 7.0 |
| outdoor_humidity | 83.5 |
| outdoor_temperature | 24.056477 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.63e+03 |
| n_updates | 27024 |
-----------------------------------------------
-----------------------------------------------***************------------------------------------------------| 51%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 68.48272 |
| air_temperature | 26.513992 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 18.0 |
| month | 7.0 |
| outdoor_humidity | 70.25 |
| outdoor_temperature | 26.597227 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 67.5 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.66e+03 |
| n_updates | 27049 |
-----------------------------------------------
-----------------------------------------------****************-----------------------------------------------| 52%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 81.68627 |
| air_temperature | 24.137533 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 20.0 |
| month | 7.0 |
| outdoor_humidity | 94.0 |
| outdoor_temperature | 23.70093 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 17.5 |
| wind_speed | 0.375 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 564 |
| n_updates | 27074 |
-----------------------------------------------
-----------------------------------------------****************-----------------------------------------------| 52%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 80.33599 |
| air_temperature | 24.662937 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 22.0 |
| month | 7.0 |
| outdoor_humidity | 61.0 |
| outdoor_temperature | 27.363422 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 292.5 |
| wind_speed | 5.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.54e+03 |
| n_updates | 27099 |
-----------------------------------------------
-----------------------------------------------****************-----------------------------------------------| 52%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 77.69531 |
| air_temperature | 23.924374 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 22.0 |
| month | 7.0 |
| outdoor_humidity | 55.5 |
| outdoor_temperature | 20.73869 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 350.0 |
| wind_speed | 4.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.1e+03 |
| n_updates | 27124 |
-----------------------------------------------
-----------------------------------------------*****************----------------------------------------------| 53%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 78.839935 |
| air_temperature | 22.857756 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 18.0 |
| month | 7.0 |
| outdoor_humidity | 78.25 |
| outdoor_temperature | 20.073912 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 272.5 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.31e+03 |
| n_updates | 27149 |
-----------------------------------------------
-----------------------------------------------*****************----------------------------------------------| 53%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 75.361885 |
| air_temperature | 24.164515 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 18.0 |
| month | 7.0 |
| outdoor_humidity | 91.5 |
| outdoor_temperature | 23.011673 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 232.5 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.83e+03 |
| n_updates | 27174 |
-----------------------------------------------
-----------------------------------------------*****************----------------------------------------------| 53%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 73.6474 |
| air_temperature | 24.565113 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 27.5 |
| direct_solar_radiation | 219.5 |
| hour | 5.0 |
| htg_setpoint | 15.0 |
| month | 7.0 |
| outdoor_humidity | 80.0 |
| outdoor_temperature | 24.377394 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 35.0 |
| wind_speed | 2.725 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.65e+03 |
| n_updates | 27199 |
-----------------------------------------------
-----------------------------------------------*****************----------------------------------------------| 53%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 83.95512 |
| air_temperature | 22.372908 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 41.5 |
| direct_solar_radiation | 0.0 |
| hour | 6.0 |
| htg_setpoint | 22.0 |
| month | 7.0 |
| outdoor_humidity | 97.75 |
| outdoor_temperature | 20.871769 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 22.5 |
| wind_speed | 3.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.23e+03 |
| n_updates | 27224 |
-----------------------------------------------
-----------------------------------------------******************---------------------------------------------| 54%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 2666.0518 |
| air_humidity | 83.77003 |
| air_temperature | 21.471449 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 173.5 |
| direct_solar_radiation | 193.5 |
| hour | 7.0 |
| htg_setpoint | 17.0 |
| month | 7.0 |
| outdoor_humidity | 81.25 |
| outdoor_temperature | 24.982664 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2182675.2 |
| wind_direction | 357.5 |
| wind_speed | 0.375 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.35e+03 |
| n_updates | 27249 |
-----------------------------------------------
-----------------------------------------------******************---------------------------------------------| 54%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 6300.4297 |
| air_humidity | 69.49313 |
| air_temperature | 22.120296 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 112.75 |
| direct_solar_radiation | 736.75 |
| hour | 8.0 |
| htg_setpoint | 19.0 |
| month | 7.0 |
| outdoor_humidity | 60.5 |
| outdoor_temperature | 26.808458 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 5725117.5 |
| wind_direction | 75.0 |
| wind_speed | 2.725 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.65e+03 |
| n_updates | 27274 |
-----------------------------------------------
-----------------------------------------------******************---------------------------------------------| 54%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 8811.83 |
| air_humidity | 65.531456 |
| air_temperature | 22.561466 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 152.0 |
| direct_solar_radiation | 0.0 |
| hour | 9.0 |
| htg_setpoint | 22.0 |
| month | 7.0 |
| outdoor_humidity | 94.0 |
| outdoor_temperature | 25.571522 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 7934286.0 |
| wind_direction | 115.0 |
| wind_speed | 3.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.52e+03 |
| n_updates | 27299 |
-----------------------------------------------
-----------------------------------------------******************---------------------------------------------| 54%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 6695.759 |
| air_humidity | 60.31789 |
| air_temperature | 23.617308 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 319.75 |
| direct_solar_radiation | 525.25 |
| hour | 10.0 |
| htg_setpoint | 17.0 |
| month | 7.0 |
| outdoor_humidity | 74.0 |
| outdoor_temperature | 26.11162 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 5988546.0 |
| wind_direction | 197.5 |
| wind_speed | 4.375 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.74e+03 |
| n_updates | 27324 |
-----------------------------------------------
-----------------------------------------------*******************--------------------------------------------| 55%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 67.9954 |
| air_temperature | 26.786385 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 386.5 |
| direct_solar_radiation | 388.5 |
| hour | 11.0 |
| htg_setpoint | 22.0 |
| month | 7.0 |
| outdoor_humidity | 74.5 |
| outdoor_temperature | 30.048075 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 192.5 |
| wind_speed | 3.725 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.49e+03 |
| n_updates | 27349 |
-----------------------------------------------
-----------------------------------------------*******************--------------------------------------------| 55%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 68.56357 |
| air_temperature | 26.611694 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 372.0 |
| direct_solar_radiation | 480.0 |
| hour | 12.0 |
| htg_setpoint | 22.0 |
| month | 7.0 |
| outdoor_humidity | 71.0 |
| outdoor_temperature | 27.463623 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 197.5 |
| wind_speed | 5.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.03e+03 |
| n_updates | 27374 |
-----------------------------------------------
-----------------------------------------------*******************--------------------------------------------| 55%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 7725.646 |
| air_humidity | 57.66061 |
| air_temperature | 24.009495 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 362.25 |
| direct_solar_radiation | 482.0 |
| hour | 13.0 |
| htg_setpoint | 19.0 |
| month | 7.0 |
| outdoor_humidity | 75.5 |
| outdoor_temperature | 27.697567 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 6953081.0 |
| wind_direction | 200.0 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.38e+03 |
| n_updates | 27399 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 56%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 7112.092 |
| air_humidity | 59.154137 |
| air_temperature | 23.774574 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 233.25 |
| direct_solar_radiation | 0.0 |
| hour | 14.0 |
| htg_setpoint | 20.0 |
| month | 7.0 |
| outdoor_humidity | 92.5 |
| outdoor_temperature | 24.108007 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 6400882.5 |
| wind_direction | 242.5 |
| wind_speed | 5.5 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 9.33e+03 |
| n_updates | 27424 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 56%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 7589.05 |
| air_humidity | 58.069283 |
| air_temperature | 24.212975 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 168.75 |
| direct_solar_radiation | 636.25 |
| hour | 15.0 |
| htg_setpoint | 17.0 |
| month | 7.0 |
| outdoor_humidity | 65.75 |
| outdoor_temperature | 27.823973 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 6806540.0 |
| wind_direction | 192.5 |
| wind_speed | 4.75 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 539 |
| n_updates | 27449 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 56%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 7284.086 |
| air_humidity | 57.75603 |
| air_temperature | 24.36963 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 224.0 |
| direct_solar_radiation | 46.5 |
| hour | 16.0 |
| htg_setpoint | 15.0 |
| month | 7.0 |
| outdoor_humidity | 65.25 |
| outdoor_temperature | 27.25723 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 6567345.5 |
| wind_direction | 200.0 |
| wind_speed | 5.675 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.68e+03 |
| n_updates | 27474 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 56%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 54.34198 |
| air_temperature | 26.128633 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 81.5 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 16.0 |
| month | 7.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 21.060984 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 252.5 |
| wind_speed | 3.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.5e+03 |
| n_updates | 27499 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 57%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 66.87071 |
| air_temperature | 27.23084 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 41.5 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 16.0 |
| month | 7.0 |
| outdoor_humidity | 78.25 |
| outdoor_temperature | 22.799652 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 190.0 |
| wind_speed | 2.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 652 |
| n_updates | 27524 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 57%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 70.91234 |
| air_temperature | 26.266905 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 16.0 |
| month | 7.0 |
| outdoor_humidity | 75.25 |
| outdoor_temperature | 22.761063 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 227.5 |
| wind_speed | 5.3 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.84e+03 |
| n_updates | 27549 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 57%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 60.622814 |
| air_temperature | 27.924835 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 21.0 |
| month | 7.0 |
| outdoor_humidity | 84.0 |
| outdoor_temperature | 23.308342 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 182.5 |
| wind_speed | 4.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.28e+03 |
| n_updates | 27574 |
-----------------------------------------------
-----------------------------------------------********************-------------------------------------------| 57%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 68.77873 |
| air_temperature | 26.76857 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 21.0 |
| month | 7.0 |
| outdoor_humidity | 90.75 |
| outdoor_temperature | 23.624556 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 210.0 |
| wind_speed | 4.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.2e+03 |
| n_updates | 27599 |
-----------------------------------------------
-----------------------------------------------*********************------------------------------------------| 58%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 74.077736 |
| air_temperature | 25.452297 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 21.0 |
| month | 7.0 |
| outdoor_humidity | 82.0 |
| outdoor_temperature | 23.497612 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 195.0 |
| wind_speed | 3.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.44e+03 |
| n_updates | 27624 |
-----------------------------------------------
-----------------------------------------------*********************------------------------------------------| 58%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 72.40393 |
| air_temperature | 25.641663 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 66.25 |
| outdoor_temperature | 24.917315 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 232.5 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 7.44e+03 |
| n_updates | 27649 |
-----------------------------------------------
-----------------------------------------------***********************----------------------------------------| 59%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 75.30557 |
| air_temperature | 25.393188 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 17.0 |
| month | 8.0 |
| outdoor_humidity | 76.75 |
| outdoor_temperature | 28.25013 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 200.0 |
| wind_speed | 3.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.4e+03 |
| n_updates | 27674 |
-----------------------------------------------
----------------------------------------------************************----------------------------------------| 59%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 74.47385 |
| air_temperature | 25.55956 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 20.0 |
| month | 8.0 |
| outdoor_humidity | 94.0 |
| outdoor_temperature | 25.52394 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 187.5 |
| wind_speed | 2.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.64e+03 |
| n_updates | 27699 |
----------------------------------------------
-----------------------------------------------***********************----------------------------------------| 59%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 76.14162 |
| air_temperature | 25.054893 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 18.0 |
| month | 8.0 |
| outdoor_humidity | 82.75 |
| outdoor_temperature | 25.166603 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 260.0 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 8.39e+03 |
| n_updates | 27724 |
-----------------------------------------------
-----------------------------------------------***********************----------------------------------------| 59%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 85.48113 |
| air_temperature | 23.279541 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 19.0 |
| month | 8.0 |
| outdoor_humidity | 89.5 |
| outdoor_temperature | 23.949242 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 0.0 |
| wind_speed | 2.725 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.23e+03 |
| n_updates | 27749 |
-----------------------------------------------
-----------------------------------------------************************---------------------------------------| 60%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 90.318306 |
| air_temperature | 21.868973 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 86.5 |
| outdoor_temperature | 18.62419 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 42.5 |
| wind_speed | 2.725 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.65e+03 |
| n_updates | 27774 |
-----------------------------------------------
-----------------------------------------------************************---------------------------------------| 60%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 91.097755 |
| air_temperature | 21.851496 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 21.0 |
| direct_solar_radiation | 57.75 |
| hour | 5.0 |
| htg_setpoint | 19.0 |
| month | 8.0 |
| outdoor_humidity | 72.5 |
| outdoor_temperature | 15.950931 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 30.0 |
| wind_speed | 3.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.83e+03 |
| n_updates | 27799 |
-----------------------------------------------
-----------------------------------------------************************---------------------------------------| 60%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 86.87412 |
| air_temperature | 21.786936 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 98.75 |
| direct_solar_radiation | 102.0 |
| hour | 6.0 |
| htg_setpoint | 19.0 |
| month | 8.0 |
| outdoor_humidity | 73.5 |
| outdoor_temperature | 19.933619 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 35.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.68e+03 |
| n_updates | 27824 |
-----------------------------------------------
-----------------------------------------------************************---------------------------------------| 60%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 78.27451 |
| air_temperature | 23.58274 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 71.0 |
| direct_solar_radiation | 629.0 |
| hour | 7.0 |
| htg_setpoint | 18.0 |
| month | 8.0 |
| outdoor_humidity | 74.75 |
| outdoor_temperature | 22.477741 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 275.0 |
| wind_speed | 2.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.16e+03 |
| n_updates | 27849 |
-----------------------------------------------
-----------------------------------------------*************************--------------------------------------| 61%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 75.46448 |
| air_temperature | 24.158018 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 259.0 |
| direct_solar_radiation | 266.5 |
| hour | 8.0 |
| htg_setpoint | 15.0 |
| month | 8.0 |
| outdoor_humidity | 79.0 |
| outdoor_temperature | 25.413868 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 205.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.28e+03 |
| n_updates | 27874 |
-----------------------------------------------
-----------------------------------------------*************************--------------------------------------| 61%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 7313.54 |
| air_humidity | 62.38672 |
| air_temperature | 22.854069 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 337.5 |
| direct_solar_radiation | 108.5 |
| hour | 9.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 78.5 |
| outdoor_temperature | 26.157618 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 6531380.0 |
| wind_direction | 230.0 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.41e+03 |
| n_updates | 27899 |
-----------------------------------------------
-----------------------------------------------*************************--------------------------------------| 61%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 4564.3667 |
| air_humidity | 58.276726 |
| air_temperature | 23.902065 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 279.75 |
| direct_solar_radiation | 594.75 |
| hour | 10.0 |
| htg_setpoint | 18.0 |
| month | 8.0 |
| outdoor_humidity | 39.75 |
| outdoor_temperature | 27.919645 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 4107929.8 |
| wind_direction | 335.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.33e+03 |
| n_updates | 27924 |
-----------------------------------------------
-----------------------------------------------**************************-------------------------------------| 62%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 7623.4326 |
| air_humidity | 58.009117 |
| air_temperature | 24.158352 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 354.75 |
| direct_solar_radiation | 483.5 |
| hour | 11.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 66.5 |
| outdoor_temperature | 27.180256 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 6847386.5 |
| wind_direction | 197.5 |
| wind_speed | 5.05 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.55e+03 |
| n_updates | 27949 |
-----------------------------------------------
-----------------------------------------------**************************-------------------------------------| 62%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 6947.407 |
| air_humidity | 58.06217 |
| air_temperature | 23.78853 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 186.5 |
| direct_solar_radiation | 707.25 |
| hour | 12.0 |
| htg_setpoint | 22.0 |
| month | 8.0 |
| outdoor_humidity | 52.25 |
| outdoor_temperature | 29.24378 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 6253876.0 |
| wind_direction | 165.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.43e+03 |
| n_updates | 27974 |
-----------------------------------------------
-----------------------------------------------**************************-------------------------------------| 62%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 9098.813 |
| air_humidity | 56.64776 |
| air_temperature | 24.771917 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 305.0 |
| direct_solar_radiation | 491.5 |
| hour | 13.0 |
| htg_setpoint | 15.0 |
| month | 8.0 |
| outdoor_humidity | 58.0 |
| outdoor_temperature | 33.54648 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 8188946.0 |
| wind_direction | 100.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.43e+04 |
| n_updates | 27999 |
-----------------------------------------------
-----------------------------------------------**************************-------------------------------------| 62%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 76.09069 |
| air_temperature | 27.673058 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 353.25 |
| direct_solar_radiation | 251.75 |
| hour | 14.0 |
| htg_setpoint | 20.0 |
| month | 8.0 |
| outdoor_humidity | 60.0 |
| outdoor_temperature | 31.510412 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 37.5 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.23e+03 |
| n_updates | 28024 |
-----------------------------------------------
-----------------------------------------------***************************------------------------------------| 63%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 71.35179 |
| air_temperature | 28.799736 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 138.5 |
| direct_solar_radiation | 643.5 |
| hour | 15.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 33.0 |
| outdoor_temperature | 32.46637 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 57.5 |
| wind_speed | 6.325 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.98e+03 |
| n_updates | 28049 |
-----------------------------------------------
-----------------------------------------------***************************------------------------------------| 63%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 4839.138 |
| air_humidity | 53.21796 |
| air_temperature | 24.422802 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 147.5 |
| direct_solar_radiation | 458.5 |
| hour | 16.0 |
| htg_setpoint | 16.0 |
| month | 8.0 |
| outdoor_humidity | 43.75 |
| outdoor_temperature | 27.520885 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 4355224.5 |
| wind_direction | 117.5 |
| wind_speed | 4.875 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.24e+03 |
| n_updates | 28074 |
-----------------------------------------------
-----------------------------------------------***************************------------------------------------| 63%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 49.3193 |
| air_temperature | 26.766872 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 98.0 |
| direct_solar_radiation | 229.0 |
| hour | 17.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 53.5 |
| outdoor_temperature | 25.16245 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 167.5 |
| wind_speed | 3.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.46e+03 |
| n_updates | 28099 |
-----------------------------------------------
-----------------------------------------------***************************------------------------------------| 63%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 50.959476 |
| air_temperature | 30.62267 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 23.25 |
| direct_solar_radiation | 160.5 |
| hour | 18.0 |
| htg_setpoint | 19.0 |
| month | 8.0 |
| outdoor_humidity | 66.75 |
| outdoor_temperature | 29.26822 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 202.5 |
| wind_speed | 3.725 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.14e+03 |
| n_updates | 28124 |
-----------------------------------------------
-----------------------------------------------****************************-----------------------------------| 64%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 43.667793 |
| air_temperature | 29.918974 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 31.25 |
| outdoor_temperature | 26.58964 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 320.0 |
| wind_speed | 5.575 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.25e+03 |
| n_updates | 28149 |
-----------------------------------------------
-----------------------------------------------****************************-----------------------------------| 64%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 61.34158 |
| air_temperature | 28.237514 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 15.0 |
| month | 8.0 |
| outdoor_humidity | 68.75 |
| outdoor_temperature | 23.070307 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 207.5 |
| wind_speed | 7.075 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.49e+03 |
| n_updates | 28174 |
-----------------------------------------------
-----------------------------------------------****************************-----------------------------------| 64%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 67.39148 |
| air_temperature | 26.905378 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 32.5 |
| outdoor_temperature | 24.062317 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 0.0 |
| wind_speed | 8.075 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.4e+03 |
| n_updates | 28199 |
-----------------------------------------------
-----------------------------------------------*****************************----------------------------------| 65%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 71.6153 |
| air_temperature | 25.987005 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 50.0 |
| outdoor_temperature | 21.171911 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 57.5 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.58e+03 |
| n_updates | 28224 |
-----------------------------------------------
-----------------------------------------------*****************************----------------------------------| 65%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 79.07577 |
| air_temperature | 24.398651 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 68.75 |
| outdoor_temperature | 19.837196 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 225.0 |
| wind_speed | 4.75 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.27e+03 |
| n_updates | 28249 |
-----------------------------------------------
-----------------------------------------------*****************************----------------------------------| 65%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 79.98378 |
| air_temperature | 24.30729 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 22.0 |
| month | 8.0 |
| outdoor_humidity | 68.5 |
| outdoor_temperature | 23.006378 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 42.5 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.3e+03 |
| n_updates | 28274 |
-----------------------------------------------
-----------------------------------------------******************************---------------------------------| 66%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 77.59511 |
| air_temperature | 23.637218 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 17.0 |
| month | 8.0 |
| outdoor_humidity | 65.5 |
| outdoor_temperature | 19.68392 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 47.5 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.93e+03 |
| n_updates | 28299 |
-----------------------------------------------
-----------------------------------------------******************************---------------------------------| 66%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 78.53599 |
| air_temperature | 23.408203 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 21.0 |
| month | 8.0 |
| outdoor_humidity | 57.5 |
| outdoor_temperature | 24.29011 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 355.0 |
| wind_speed | 0.65 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.19e+03 |
| n_updates | 28324 |
-----------------------------------------------
-----------------------------------------------******************************---------------------------------| 66%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 61.36071 |
| air_temperature | 23.787981 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 22.0 |
| month | 8.0 |
| outdoor_humidity | 72.25 |
| outdoor_temperature | 18.836372 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 257.5 |
| wind_speed | 1.65 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.11e+03 |
| n_updates | 28349 |
-----------------------------------------------
-----------------------------------------------******************************---------------------------------| 66%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 61.465584 |
| air_temperature | 23.595346 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 22.0 |
| month | 9.0 |
| outdoor_humidity | 86.25 |
| outdoor_temperature | 21.46531 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 295.0 |
| wind_speed | 2.725 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.91e+03 |
| n_updates | 28374 |
-----------------------------------------------
-----------------------------------------------*******************************--------------------------------| 67%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 62.35134 |
| air_temperature | 23.423868 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 22.0 |
| month | 9.0 |
| outdoor_humidity | 55.5 |
| outdoor_temperature | 23.927923 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 0.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.98e+03 |
| n_updates | 28399 |
-----------------------------------------------
-----------------------------------------------*******************************--------------------------------| 67%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 93.466934 |
| air_temperature | 21.542606 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 50.75 |
| direct_solar_radiation | 189.25 |
| hour | 6.0 |
| htg_setpoint | 22.0 |
| month | 9.0 |
| outdoor_humidity | 62.5 |
| outdoor_temperature | 15.690955 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 45.0 |
| wind_speed | 3.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.02e+03 |
| n_updates | 28424 |
-----------------------------------------------
-----------------------------------------------*******************************--------------------------------| 67%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 2477.6624 |
| air_humidity | 77.14731 |
| air_temperature | 22.00025 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 83.0 |
| direct_solar_radiation | 502.75 |
| hour | 7.0 |
| htg_setpoint | 22.0 |
| month | 9.0 |
| outdoor_humidity | 73.25 |
| outdoor_temperature | 20.781527 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1977187.8 |
| wind_direction | 270.0 |
| wind_speed | 1.125 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.29e+03 |
| n_updates | 28449 |
-----------------------------------------------
-----------------------------------------------********************************-------------------------------| 68%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 4321.831 |
| air_humidity | 70.94759 |
| air_temperature | 21.340313 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 116.75 |
| direct_solar_radiation | 631.0 |
| hour | 8.0 |
| htg_setpoint | 16.0 |
| month | 9.0 |
| outdoor_humidity | 67.5 |
| outdoor_temperature | 22.644999 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 3888217.8 |
| wind_direction | 240.0 |
| wind_speed | 4.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.62e+03 |
| n_updates | 28474 |
-----------------------------------------------
-----------------------------------------------********************************-------------------------------| 68%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 4505.541 |
| air_humidity | 62.703907 |
| air_temperature | 22.807577 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 98.25 |
| direct_solar_radiation | 812.75 |
| hour | 9.0 |
| htg_setpoint | 21.0 |
| month | 9.0 |
| outdoor_humidity | 53.5 |
| outdoor_temperature | 24.97025 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 4071010.2 |
| wind_direction | 22.5 |
| wind_speed | 3.725 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.55e+03 |
| n_updates | 28499 |
-----------------------------------------------
-----------------------------------------------********************************-------------------------------| 68%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 75.33011 |
| air_temperature | 24.624273 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 154.75 |
| direct_solar_radiation | 478.5 |
| hour | 10.0 |
| htg_setpoint | 22.0 |
| month | 9.0 |
| outdoor_humidity | 74.5 |
| outdoor_temperature | 25.0148 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 197.5 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.3e+03 |
| n_updates | 28524 |
-----------------------------------------------
-----------------------------------------------********************************-------------------------------| 68%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 72.02536 |
| air_temperature | 25.36438 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 400.5 |
| direct_solar_radiation | 304.0 |
| hour | 11.0 |
| htg_setpoint | 17.0 |
| month | 9.0 |
| outdoor_humidity | 65.0 |
| outdoor_temperature | 22.886509 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 50.0 |
| wind_speed | 6.575 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.89e+03 |
| n_updates | 28549 |
-----------------------------------------------
-----------------------------------------------*********************************------------------------------| 69%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 3543.0786 |
| air_humidity | 58.36832 |
| air_temperature | 23.563951 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 261.25 |
| direct_solar_radiation | 207.0 |
| hour | 12.0 |
| htg_setpoint | 21.0 |
| month | 9.0 |
| outdoor_humidity | 67.75 |
| outdoor_temperature | 21.862566 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 3202745.5 |
| wind_direction | 175.0 |
| wind_speed | 3.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 7.82e+03 |
| n_updates | 28574 |
-----------------------------------------------
-----------------------------------------------*********************************------------------------------| 69%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 4519.3745 |
| air_humidity | 41.45838 |
| air_temperature | 23.592949 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 98.25 |
| direct_solar_radiation | 822.75 |
| hour | 13.0 |
| htg_setpoint | 22.0 |
| month | 9.0 |
| outdoor_humidity | 31.75 |
| outdoor_temperature | 24.748531 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 4067437.0 |
| wind_direction | 7.5 |
| wind_speed | 6.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.52e+03 |
| n_updates | 28599 |
-----------------------------------------------
-----------------------------------------------*********************************------------------------------| 69%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 3872.587 |
| air_humidity | 35.28053 |
| air_temperature | 24.146233 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 174.0 |
| direct_solar_radiation | 672.0 |
| hour | 14.0 |
| htg_setpoint | 19.0 |
| month | 9.0 |
| outdoor_humidity | 36.25 |
| outdoor_temperature | 20.738441 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 3485328.2 |
| wind_direction | 210.0 |
| wind_speed | 4.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.6e+03 |
| n_updates | 28624 |
-----------------------------------------------
-----------------------------------------------*********************************------------------------------| 69%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 4094.6316 |
| air_humidity | 54.37934 |
| air_temperature | 24.257887 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 184.5 |
| direct_solar_radiation | 314.25 |
| hour | 15.0 |
| htg_setpoint | 19.0 |
| month | 9.0 |
| outdoor_humidity | 47.0 |
| outdoor_temperature | 24.230354 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 3685168.5 |
| wind_direction | 172.5 |
| wind_speed | 5.825 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.75e+03 |
| n_updates | 28649 |
-----------------------------------------------
-----------------------------------------------**********************************-----------------------------| 70%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 6661.5596 |
| air_humidity | 59.606304 |
| air_temperature | 23.828178 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 115.5 |
| direct_solar_radiation | 171.75 |
| hour | 16.0 |
| htg_setpoint | 18.0 |
| month | 9.0 |
| outdoor_humidity | 80.5 |
| outdoor_temperature | 24.708069 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 5995403.5 |
| wind_direction | 200.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.82e+03 |
| n_updates | 28674 |
-----------------------------------------------
-----------------------------------------------**********************************-----------------------------| 70%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 61.85174 |
| air_temperature | 27.639334 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 44.75 |
| direct_solar_radiation | 168.0 |
| hour | 17.0 |
| htg_setpoint | 19.0 |
| month | 9.0 |
| outdoor_humidity | 52.25 |
| outdoor_temperature | 25.771671 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 272.5 |
| wind_speed | 4.5 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.35e+03 |
| n_updates | 28699 |
-----------------------------------------------
-----------------------------------------------**********************************-----------------------------| 70%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 66.16045 |
| air_temperature | 26.708534 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 22.0 |
| month | 9.0 |
| outdoor_humidity | 59.5 |
| outdoor_temperature | 19.23912 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 152.5 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.08e+03 |
| n_updates | 28724 |
-----------------------------------------------
-----------------------------------------------***********************************----------------------------| 71%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 58.647655 |
| air_temperature | 28.036564 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 19.0 |
| month | 9.0 |
| outdoor_humidity | 69.25 |
| outdoor_temperature | 19.43089 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 135.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.57e+03 |
| n_updates | 28749 |
-----------------------------------------------
-----------------------------------------------***********************************----------------------------| 71%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 67.57566 |
| air_temperature | 26.250349 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 15.0 |
| month | 9.0 |
| outdoor_humidity | 76.75 |
| outdoor_temperature | 21.130976 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 350.0 |
| wind_speed | 4.8 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.15e+03 |
| n_updates | 28774 |
-----------------------------------------------
-----------------------------------------------***********************************----------------------------| 71%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 63.49098 |
| air_temperature | 25.85888 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 22.0 |
| month | 9.0 |
| outdoor_humidity | 47.0 |
| outdoor_temperature | 18.392647 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 25.0 |
| wind_speed | 3.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.9e+03 |
| n_updates | 28799 |
-----------------------------------------------
-----------------------------------------------***********************************----------------------------| 71%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 75.80126 |
| air_temperature | 24.471773 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 22.0 |
| month | 9.0 |
| outdoor_humidity | 78.0 |
| outdoor_temperature | 15.646113 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 40.0 |
| wind_speed | 2.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.9e+03 |
| n_updates | 28824 |
-----------------------------------------------
-----------------------------------------------************************************---------------------------| 72%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 80.124214 |
| air_temperature | 23.765324 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 19.0 |
| month | 9.0 |
| outdoor_humidity | 79.5 |
| outdoor_temperature | 19.500229 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 197.5 |
| wind_speed | 2.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.5e+03 |
| n_updates | 28849 |
-----------------------------------------------
-----------------------------------------------************************************---------------------------| 72%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 80.977394 |
| air_temperature | 23.556833 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 21.0 |
| month | 9.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 20.698845 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 210.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.04e+03 |
| n_updates | 28874 |
-----------------------------------------------
-----------------------------------------------************************************---------------------------| 72%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 91.42441 |
| air_temperature | 21.563036 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 19.0 |
| month | 9.0 |
| outdoor_humidity | 69.5 |
| outdoor_temperature | 13.609982 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 340.0 |
| wind_speed | 4.625 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.97e+03 |
| n_updates | 28899 |
-----------------------------------------------
-----------------------------------------------*************************************--------------------------| 73%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 61.102802 |
| air_temperature | 21.2258 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 16.0 |
| month | 9.0 |
| outdoor_humidity | 65.0 |
| outdoor_temperature | 12.835937 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 20.0 |
| wind_speed | 2.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.69e+03 |
| n_updates | 28924 |
-----------------------------------------------
-----------------------------------------------*************************************--------------------------| 73%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 79.69949 |
| air_temperature | 21.181385 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 16.0 |
| month | 9.0 |
| outdoor_humidity | 75.25 |
| outdoor_temperature | 13.469681 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 60.0 |
| wind_speed | 3.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.07e+03 |
| n_updates | 28949 |
-----------------------------------------------
-----------------------------------------------*************************************--------------------------| 73%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 96.59502 |
| air_temperature | 20.909647 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 22.0 |
| month | 9.0 |
| outdoor_humidity | 93.75 |
| outdoor_temperature | 14.101468 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 12.5 |
| wind_speed | 4.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.52e+04 |
| n_updates | 28974 |
-----------------------------------------------
-----------------------------------------------**************************************-------------------------| 74%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 83.66151 |
| air_temperature | 20.910679 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 22.0 |
| month | 9.0 |
| outdoor_humidity | 93.0 |
| outdoor_temperature | 14.273347 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 335.0 |
| wind_speed | 0.775 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 9.57e+03 |
| n_updates | 28999 |
-----------------------------------------------
-----------------------------------------------**************************************-------------------------| 74%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 86.35091 |
| air_temperature | 22.401176 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 28.5 |
| direct_solar_radiation | 148.0 |
| hour | 6.0 |
| htg_setpoint | 15.0 |
| month | 9.0 |
| outdoor_humidity | 66.5 |
| outdoor_temperature | 17.324581 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 305.0 |
| wind_speed | 2.725 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.63e+03 |
| n_updates | 29024 |
-----------------------------------------------
------------------------------------------------*************************************-------------------------| 74%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 0.0 |
| air_humidity | 91.71592 |
| air_temperature | 21.573292 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 61.5 |
| direct_solar_radiation | 462.0 |
| hour | 7.0 |
| htg_setpoint | 18.0 |
| month | 9.0 |
| outdoor_humidity | 62.5 |
| outdoor_temperature | 15.8933735 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 0.0 |
| wind_direction | 55.0 |
| wind_speed | 3.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 951 |
| n_updates | 29049 |
------------------------------------------------
-----------------------------------------------**************************************-------------------------| 74%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 251.89574 |
| air_humidity | 70.314674 |
| air_temperature | 21.169209 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 77.5 |
| direct_solar_radiation | 664.75 |
| hour | 8.0 |
| htg_setpoint | 19.0 |
| month | 9.0 |
| outdoor_humidity | 71.5 |
| outdoor_temperature | 14.55806 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 226868.83 |
| wind_direction | 40.0 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.4e+03 |
| n_updates | 29074 |
-----------------------------------------------
-----------------------------------------------***************************************------------------------| 75%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 850.58246 |
| air_humidity | 35.990826 |
| air_temperature | 22.583698 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 119.5 |
| direct_solar_radiation | 0.0 |
| hour | 9.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 66.0 |
| outdoor_temperature | 9.76581 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 769733.7 |
| wind_direction | 5.0 |
| wind_speed | 6.075 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.25e+04 |
| n_updates | 29099 |
-----------------------------------------------
-----------------------------------------------***************************************------------------------| 75%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 42.975693 |
| air_temperature | 26.315203 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 204.5 |
| direct_solar_radiation | 600.75 |
| hour | 10.0 |
| htg_setpoint | 21.0 |
| month | 10.0 |
| outdoor_humidity | 59.0 |
| outdoor_temperature | 19.211603 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 280.0 |
| wind_speed | 3.725 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 636 |
| n_updates | 29124 |
-----------------------------------------------
-----------------------------------------------***************************************------------------------| 75%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 51.393436 |
| air_temperature | 28.684353 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 178.5 |
| direct_solar_radiation | 708.25 |
| hour | 11.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 68.0 |
| outdoor_temperature | 21.962957 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 182.5 |
| wind_speed | 4.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.99e+03 |
| n_updates | 29149 |
-----------------------------------------------
-----------------------------------------------***************************************------------------------| 75%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 48.845577 |
| air_temperature | 30.227089 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 179.5 |
| direct_solar_radiation | 708.25 |
| hour | 12.0 |
| htg_setpoint | 18.0 |
| month | 10.0 |
| outdoor_humidity | 57.0 |
| outdoor_temperature | 24.785164 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 200.0 |
| wind_speed | 4.375 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.71e+03 |
| n_updates | 29174 |
-----------------------------------------------
-----------------------------------------------****************************************-----------------------| 76%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 49.81533 |
| air_temperature | 26.78495 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 206.75 |
| direct_solar_radiation | 562.75 |
| hour | 13.0 |
| htg_setpoint | 16.0 |
| month | 10.0 |
| outdoor_humidity | 69.75 |
| outdoor_temperature | 24.165697 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 172.5 |
| wind_speed | 4.375 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.19e+03 |
| n_updates | 29199 |
-----------------------------------------------
-----------------------------------------------****************************************-----------------------| 76%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 51.039814 |
| air_temperature | 22.37903 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 163.0 |
| direct_solar_radiation | 601.5 |
| hour | 14.0 |
| htg_setpoint | 15.0 |
| month | 10.0 |
| outdoor_humidity | 47.0 |
| outdoor_temperature | 16.668146 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 302.5 |
| wind_speed | 9.4 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.64e+03 |
| n_updates | 29224 |
-----------------------------------------------
-----------------------------------------------****************************************-----------------------| 76%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 755.82086 |
| air_humidity | 21.041191 |
| air_temperature | 24.998777 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 144.75 |
| direct_solar_radiation | 395.75 |
| hour | 15.0 |
| htg_setpoint | 20.0 |
| month | 10.0 |
| outdoor_humidity | 30.75 |
| outdoor_temperature | 12.887494 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 680238.8 |
| wind_direction | 300.0 |
| wind_speed | 9.3 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.18e+04 |
| n_updates | 29249 |
-----------------------------------------------
------------------------------------------------****************************************----------------------| 77%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 589.74524 |
| air_humidity | 23.250929 |
| air_temperature | 25.870352 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 62.25 |
| direct_solar_radiation | 407.75 |
| hour | 16.0 |
| htg_setpoint | 19.0 |
| month | 10.0 |
| outdoor_humidity | 35.5 |
| outdoor_temperature | 11.0501375 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 530770.75 |
| wind_direction | 327.5 |
| wind_speed | 6.325 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3e+03 |
| n_updates | 29274 |
------------------------------------------------
-----------------------------------------------*****************************************----------------------| 77%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 354.78235 |
| air_humidity | 32.41525 |
| air_temperature | 25.343817 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 13.5 |
| direct_solar_radiation | 74.25 |
| hour | 17.0 |
| htg_setpoint | 16.0 |
| month | 10.0 |
| outdoor_humidity | 59.25 |
| outdoor_temperature | 12.575379 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 320056.66 |
| wind_direction | 197.5 |
| wind_speed | 4.675 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.08e+03 |
| n_updates | 29299 |
-----------------------------------------------
-----------------------------------------------*****************************************----------------------| 77%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 171.28177 |
| air_humidity | 52.437138 |
| air_temperature | 25.750761 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 16.0 |
| month | 10.0 |
| outdoor_humidity | 95.25 |
| outdoor_temperature | 13.752086 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 153119.23 |
| wind_direction | 190.0 |
| wind_speed | 3.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 992 |
| n_updates | 29324 |
-----------------------------------------------
-----------------------------------------------*****************************************----------------------| 77%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 847.4101 |
| air_humidity | 64.57593 |
| air_temperature | 23.547272 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 89.25 |
| outdoor_temperature | 17.499285 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 803713.75 |
| wind_direction | 195.0 |
| wind_speed | 6.175 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.11e+03 |
| n_updates | 29349 |
-----------------------------------------------
-----------------------------------------------******************************************---------------------| 78%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 61.545914 |
| air_temperature | 22.444693 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 21.0 |
| month | 10.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 14.527241 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 195.0 |
| wind_speed | 2.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.8e+03 |
| n_updates | 29374 |
-----------------------------------------------
-----------------------------------------------******************************************---------------------| 78%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 65.661545 |
| air_temperature | 22.53084 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 21.0 |
| month | 10.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 15.061643 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 85.0 |
| wind_speed | 2.325 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.06e+03 |
| n_updates | 29399 |
-----------------------------------------------
-----------------------------------------------******************************************---------------------| 78%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 75.10691 |
| air_temperature | 21.282064 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 21.0 |
| month | 10.0 |
| outdoor_humidity | 97.75 |
| outdoor_temperature | 17.111017 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 120.0 |
| wind_speed | 7.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.96e+03 |
| n_updates | 29424 |
-----------------------------------------------
-----------------------------------------------******************************************---------------------| 78%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 163.51776 |
| air_humidity | 38.679943 |
| air_temperature | 20.623661 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 19.0 |
| month | 10.0 |
| outdoor_humidity | 64.0 |
| outdoor_temperature | 11.948649 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 144203.34 |
| wind_direction | 322.5 |
| wind_speed | 1.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.08e+03 |
| n_updates | 29449 |
-----------------------------------------------
-----------------------------------------------*******************************************--------------------| 79%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 195.99529 |
| air_humidity | 59.502026 |
| air_temperature | 20.113121 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 16.0 |
| month | 10.0 |
| outdoor_humidity | 79.75 |
| outdoor_temperature | 11.68936 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 176395.77 |
| wind_direction | 285.0 |
| wind_speed | 6.3 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.14e+03 |
| n_updates | 29474 |
-----------------------------------------------
-----------------------------------------------*******************************************--------------------| 79%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 395.82913 |
| air_humidity | 18.008144 |
| air_temperature | 21.827543 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 40.5 |
| outdoor_temperature | 7.9139585 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 356246.22 |
| wind_direction | 287.5 |
| wind_speed | 9.15 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.37e+03 |
| n_updates | 29499 |
-----------------------------------------------
-----------------------------------------------********************************************-------------------| 80%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 28.179922 |
| air_temperature | 20.999998 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 21.0 |
| month | 10.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 3.8165884 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.79e+03 |
| n_updates | 29524 |
-----------------------------------------------
-----------------------------------------------********************************************-------------------| 80%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 51.240658 |
| air_temperature | 20.216024 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 19.0 |
| month | 10.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 11.049884 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.96e+03 |
| n_updates | 29549 |
-----------------------------------------------
-----------------------------------------------********************************************-------------------| 80%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 269.9406 |
| air_humidity | 44.607895 |
| air_temperature | 21.994785 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 94.75 |
| outdoor_temperature | 10.3867 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 242946.55 |
| wind_direction | 0.0 |
| wind_speed | 0.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.46e+03 |
| n_updates | 29574 |
-----------------------------------------------
-----------------------------------------------********************************************-------------------| 80%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 265.15726 |
| air_humidity | 67.06001 |
| air_temperature | 19.49031 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 15.0 |
| month | 10.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 12.626621 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 247664.86 |
| wind_direction | 320.0 |
| wind_speed | 0.65 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.8e+03 |
| n_updates | 29599 |
-----------------------------------------------
-----------------------------------------------*********************************************------------------| 81%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 173.63905 |
| air_humidity | 48.56933 |
| air_temperature | 20.999338 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 6.0 |
| htg_setpoint | 21.0 |
| month | 10.0 |
| outdoor_humidity | 85.5 |
| outdoor_temperature | 13.303662 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 155237.77 |
| wind_direction | 65.0 |
| wind_speed | 2.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.25e+03 |
| n_updates | 29624 |
-----------------------------------------------
-----------------------------------------------*********************************************------------------| 81%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 118.98283 |
| air_humidity | 65.69601 |
| air_temperature | 21.996305 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 69.5 |
| direct_solar_radiation | 315.75 |
| hour | 7.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 15.818181 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 103831.44 |
| wind_direction | 197.5 |
| wind_speed | 3.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.04e+03 |
| n_updates | 29649 |
-----------------------------------------------
-----------------------------------------------*********************************************------------------| 81%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 247.43365 |
| air_humidity | 72.01116 |
| air_temperature | 21.810081 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 104.0 |
| direct_solar_radiation | 539.5 |
| hour | 8.0 |
| htg_setpoint | 17.0 |
| month | 10.0 |
| outdoor_humidity | 90.0 |
| outdoor_temperature | 18.61902 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 213251.53 |
| wind_direction | 200.0 |
| wind_speed | 4.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.45e+03 |
| n_updates | 29674 |
-----------------------------------------------
-----------------------------------------------*********************************************------------------| 81%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 17.864481 |
| air_temperature | 22.0 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 163.75 |
| direct_solar_radiation | 544.0 |
| hour | 9.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 31.25 |
| outdoor_temperature | 12.616375 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 250.0 |
| wind_speed | 10.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.55e+03 |
| n_updates | 29699 |
-----------------------------------------------
-----------------------------------------------**********************************************-----------------| 82%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 67.14059 |
| air_humidity | 20.561531 |
| air_temperature | 21.291023 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 129.75 |
| direct_solar_radiation | 744.25 |
| hour | 10.0 |
| htg_setpoint | 21.0 |
| month | 10.0 |
| outdoor_humidity | 48.75 |
| outdoor_temperature | 9.290013 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60426.527 |
| wind_direction | 287.5 |
| wind_speed | 8.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.14e+03 |
| n_updates | 29724 |
-----------------------------------------------
-----------------------------------------------**********************************************-----------------| 82%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 21.577415 |
| air_temperature | 23.974966 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 214.5 |
| direct_solar_radiation | 489.25 |
| hour | 11.0 |
| htg_setpoint | 21.0 |
| month | 10.0 |
| outdoor_humidity | 34.75 |
| outdoor_temperature | 9.945387 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 342.5 |
| wind_speed | 7.325 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.33e+03 |
| n_updates | 29749 |
-----------------------------------------------
-----------------------------------------------**********************************************-----------------| 82%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 25.4303 |
| air_temperature | 24.09662 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 193.75 |
| direct_solar_radiation | 532.75 |
| hour | 12.0 |
| htg_setpoint | 22.0 |
| month | 10.0 |
| outdoor_humidity | 45.5 |
| outdoor_temperature | 12.126259 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 220.0 |
| wind_speed | 4.875 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.56e+03 |
| n_updates | 29774 |
-----------------------------------------------
-----------------------------------------------***********************************************----------------| 83%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 443.75012 |
| air_humidity | 30.129744 |
| air_temperature | 26.99909 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 112.5 |
| direct_solar_radiation | 750.0 |
| hour | 13.0 |
| htg_setpoint | 18.0 |
| month | 10.0 |
| outdoor_humidity | 45.0 |
| outdoor_temperature | 16.957376 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 398741.12 |
| wind_direction | 345.0 |
| wind_speed | 3.075 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 9.75e+03 |
| n_updates | 29799 |
-----------------------------------------------
-----------------------------------------------***********************************************----------------| 83%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 43.644516 |
| air_temperature | 23.742807 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 67.5 |
| direct_solar_radiation | 0.0 |
| hour | 14.0 |
| htg_setpoint | 21.0 |
| month | 10.0 |
| outdoor_humidity | 74.25 |
| outdoor_temperature | 14.38035 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 170.0 |
| wind_speed | 5.425 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 872 |
| n_updates | 29824 |
-----------------------------------------------
-----------------------------------------------***********************************************----------------| 83%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 948.68085 |
| air_humidity | 25.795471 |
| air_temperature | 24.864655 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 87.75 |
| direct_solar_radiation | 362.0 |
| hour | 15.0 |
| htg_setpoint | 21.0 |
| month | 11.0 |
| outdoor_humidity | 38.0 |
| outdoor_temperature | 14.421692 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 853812.75 |
| wind_direction | 347.5 |
| wind_speed | 8.65 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 8.91e+03 |
| n_updates | 29849 |
-----------------------------------------------
-----------------------------------------------***********************************************----------------| 83%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 21.444937 |
| air_temperature | 23.155224 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 31.5 |
| direct_solar_radiation | 51.0 |
| hour | 16.0 |
| htg_setpoint | 19.0 |
| month | 11.0 |
| outdoor_humidity | 33.25 |
| outdoor_temperature | 15.639802 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 352.5 |
| wind_speed | 6.7 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.42e+03 |
| n_updates | 29874 |
-----------------------------------------------
-----------------------------------------------************************************************---------------| 84%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 31.62606 |
| air_temperature | 22.500097 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 66.5 |
| outdoor_temperature | 12.85239 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 255.0 |
| wind_speed | 2.325 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.69e+03 |
| n_updates | 29899 |
-----------------------------------------------
-----------------------------------------------************************************************---------------| 84%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 405.7212 |
| air_humidity | 36.554108 |
| air_temperature | 23.08769 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 55.25 |
| outdoor_temperature | 15.108369 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 374291.9 |
| wind_direction | 267.5 |
| wind_speed | 6.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.02e+03 |
| n_updates | 29924 |
-----------------------------------------------
-----------------------------------------------************************************************---------------| 84%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 305.68054 |
| air_humidity | 31.438751 |
| air_temperature | 21.759789 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 5.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 20.0 |
| month | 11.0 |
| outdoor_humidity | 59.25 |
| outdoor_temperature | 8.8017235 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 269702.0 |
| wind_direction | 312.5 |
| wind_speed | 9.4 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.6e+03 |
| n_updates | 29949 |
-----------------------------------------------
-----------------------------------------------************************************************---------------| 84%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 261.02954 |
| air_humidity | 34.213444 |
| air_temperature | 21.81333 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 16.0 |
| month | 11.0 |
| outdoor_humidity | 68.0 |
| outdoor_temperature | 8.8243685 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 229651.97 |
| wind_direction | 347.5 |
| wind_speed | 2.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.25e+03 |
| n_updates | 29974 |
-----------------------------------------------
-----------------------------------------------*************************************************--------------| 85%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 267.00937 |
| air_humidity | 41.375805 |
| air_temperature | 20.47146 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 20.0 |
| month | 11.0 |
| outdoor_humidity | 78.5 |
| outdoor_temperature | 9.48292 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 240308.42 |
| wind_direction | 357.5 |
| wind_speed | 3.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 844 |
| n_updates | 29999 |
-----------------------------------------------
-----------------------------------------------*************************************************--------------| 85%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 245.04773 |
| air_humidity | 56.78811 |
| air_temperature | 20.049967 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 17.0 |
| month | 11.0 |
| outdoor_humidity | 93.5 |
| outdoor_temperature | 10.860969 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 220542.95 |
| wind_direction | 135.0 |
| wind_speed | 1.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 9.55e+03 |
| n_updates | 30024 |
-----------------------------------------------
-----------------------------------------------*************************************************--------------| 85%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 66.95168 |
| air_humidity | 56.25504 |
| air_temperature | 21.000023 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 21.0 |
| month | 11.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 11.277805 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 60256.516 |
| wind_direction | 127.5 |
| wind_speed | 8.525 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.81e+03 |
| n_updates | 30049 |
-----------------------------------------------
-----------------------------------------------**************************************************-------------| 86%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 236.33292 |
| air_humidity | 55.955597 |
| air_temperature | 19.760807 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 16.0 |
| month | 11.0 |
| outdoor_humidity | 87.75 |
| outdoor_temperature | 13.201731 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 213139.3 |
| wind_direction | 310.0 |
| wind_speed | 7.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.57e+03 |
| n_updates | 30074 |
-----------------------------------------------
-----------------------------------------------**************************************************-------------| 86%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 709.60114 |
| air_humidity | 40.48715 |
| air_temperature | 19.001703 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 19.0 |
| month | 11.0 |
| outdoor_humidity | 77.75 |
| outdoor_temperature | 8.46865 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 635589.6 |
| wind_direction | 352.5 |
| wind_speed | 7.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.16e+03 |
| n_updates | 30099 |
-----------------------------------------------
-----------------------------------------------**************************************************-------------| 86%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 41.221107 |
| air_temperature | 18.109674 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 16.0 |
| month | 11.0 |
| outdoor_humidity | 87.25 |
| outdoor_temperature | 6.9412384 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 345.0 |
| wind_speed | 2.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.16e+03 |
| n_updates | 30124 |
-----------------------------------------------
-----------------------------------------------***************************************************------------| 87%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 263.63318 |
| air_humidity | 50.26123 |
| air_temperature | 21.0 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 21.0 |
| month | 11.0 |
| outdoor_humidity | 94.0 |
| outdoor_temperature | 11.222464 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 237269.86 |
| wind_direction | 162.5 |
| wind_speed | 1.775 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 914 |
| n_updates | 30149 |
-----------------------------------------------
-----------------------------------------------***************************************************------------| 87%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 34.58445 |
| air_temperature | 18.038744 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 17.0 |
| month | 11.0 |
| outdoor_humidity | 65.75 |
| outdoor_temperature | 2.9729078 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 285.0 |
| wind_speed | 6.075 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 892 |
| n_updates | 30174 |
-----------------------------------------------
-----------------------------------------------***************************************************------------| 87%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 30.037195 |
| air_temperature | 18.692776 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 18.0 |
| month | 11.0 |
| outdoor_humidity | 73.75 |
| outdoor_temperature | 3.3160286 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 267.5 |
| wind_speed | 5.325 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.12e+04 |
| n_updates | 30199 |
-----------------------------------------------
-----------------------------------------------***************************************************------------| 87%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 97.770256 |
| air_humidity | 39.53176 |
| air_temperature | 19.99999 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 6.0 |
| htg_setpoint | 20.0 |
| month | 11.0 |
| outdoor_humidity | 94.0 |
| outdoor_temperature | 8.763005 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 87993.23 |
| wind_direction | 240.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.51e+03 |
| n_updates | 30224 |
-----------------------------------------------
-----------------------------------------------****************************************************-----------| 88%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 244.03078 |
| air_humidity | 30.103163 |
| air_temperature | 18.441555 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 45.0 |
| direct_solar_radiation | 18.0 |
| hour | 7.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 84.25 |
| outdoor_temperature | 2.4654706 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 219627.69 |
| wind_direction | 265.0 |
| wind_speed | 5.825 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.28e+03 |
| n_updates | 30249 |
-----------------------------------------------
-----------------------------------------------****************************************************-----------| 88%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 24.286907 |
| air_temperature | 19.844337 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 91.0 |
| direct_solar_radiation | 154.5 |
| hour | 8.0 |
| htg_setpoint | 17.0 |
| month | 11.0 |
| outdoor_humidity | 65.0 |
| outdoor_temperature | 3.499041 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 275.0 |
| wind_speed | 5.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.68e+03 |
| n_updates | 30274 |
-----------------------------------------------
-----------------------------------------------****************************************************-----------| 88%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 925.3575 |
| air_humidity | 25.10244 |
| air_temperature | 21.518553 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 95.75 |
| direct_solar_radiation | 622.0 |
| hour | 9.0 |
| htg_setpoint | 18.0 |
| month | 11.0 |
| outdoor_humidity | 70.5 |
| outdoor_temperature | 1.7320251 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 827500.2 |
| wind_direction | 235.0 |
| wind_speed | 3.625 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.56e+03 |
| n_updates | 30299 |
-----------------------------------------------
-----------------------------------------------*****************************************************----------| 89%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1231.8073 |
| air_humidity | 23.295015 |
| air_temperature | 23.016277 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 160.5 |
| direct_solar_radiation | 503.0 |
| hour | 10.0 |
| htg_setpoint | 21.0 |
| month | 11.0 |
| outdoor_humidity | 72.5 |
| outdoor_temperature | 0.3730378 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 1108626.5 |
| wind_direction | 272.5 |
| wind_speed | 6.825 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.13e+03 |
| n_updates | 30324 |
-----------------------------------------------
-----------------------------------------------*****************************************************----------| 89%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1072.4786 |
| air_humidity | 19.873655 |
| air_temperature | 23.144833 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 208.75 |
| direct_solar_radiation | 347.0 |
| hour | 11.0 |
| htg_setpoint | 21.0 |
| month | 11.0 |
| outdoor_humidity | 51.0 |
| outdoor_temperature | 0.8989847 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 965230.75 |
| wind_direction | 292.5 |
| wind_speed | 9.775 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.24e+03 |
| n_updates | 30349 |
-----------------------------------------------
-----------------------------------------------*****************************************************----------| 89%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 17.420418 |
| air_temperature | 21.237041 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 117.25 |
| direct_solar_radiation | 708.5 |
| hour | 12.0 |
| htg_setpoint | 16.0 |
| month | 11.0 |
| outdoor_humidity | 47.0 |
| outdoor_temperature | 3.577261 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 317.5 |
| wind_speed | 4.875 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 8.84e+03 |
| n_updates | 30374 |
-----------------------------------------------
-----------------------------------------------*****************************************************----------| 89%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 969.2309 |
| air_humidity | 13.720751 |
| air_temperature | 21.337305 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 174.75 |
| direct_solar_radiation | 322.5 |
| hour | 13.0 |
| htg_setpoint | 19.0 |
| month | 11.0 |
| outdoor_humidity | 46.0 |
| outdoor_temperature | 1.910754 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 872307.8 |
| wind_direction | 285.0 |
| wind_speed | 5.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.51e+03 |
| n_updates | 30399 |
-----------------------------------------------
-----------------------------------------------******************************************************---------| 90%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 26.28127 |
| air_temperature | 23.197556 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 107.25 |
| direct_solar_radiation | 16.25 |
| hour | 14.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 80.75 |
| outdoor_temperature | 3.4707968 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 90.0 |
| wind_speed | 4.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.18e+03 |
| n_updates | 30424 |
-----------------------------------------------
-----------------------------------------------******************************************************---------| 90%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 384.50226 |
| air_humidity | 57.287544 |
| air_temperature | 24.413916 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 36.5 |
| direct_solar_radiation | 0.0 |
| hour | 15.0 |
| htg_setpoint | 20.0 |
| month | 11.0 |
| outdoor_humidity | 100.0 |
| outdoor_temperature | 13.534519 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 346052.03 |
| wind_direction | 165.0 |
| wind_speed | 4.375 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.43e+03 |
| n_updates | 30449 |
-----------------------------------------------
-----------------------------------------------******************************************************---------| 90%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 749.6212 |
| air_humidity | 32.984917 |
| air_temperature | 23.419529 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 13.0 |
| direct_solar_radiation | 12.0 |
| hour | 16.0 |
| htg_setpoint | 22.0 |
| month | 11.0 |
| outdoor_humidity | 66.0 |
| outdoor_temperature | 8.94522 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 674659.1 |
| wind_direction | 250.0 |
| wind_speed | 6.2 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.28e+03 |
| n_updates | 30474 |
-----------------------------------------------
-----------------------------------------------******************************************************---------| 90%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 32.733772 |
| air_temperature | 23.07528 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 20.0 |
| month | 11.0 |
| outdoor_humidity | 66.5 |
| outdoor_temperature | 7.035179 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 227.5 |
| wind_speed | 2.85 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.62e+03 |
| n_updates | 30499 |
-----------------------------------------------
-----------------------------------------------*******************************************************--------| 91%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 709.4852 |
| air_humidity | 30.311705 |
| air_temperature | 22.56456 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 21.0 |
| month | 11.0 |
| outdoor_humidity | 70.0 |
| outdoor_temperature | 5.236861 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 631967.6 |
| wind_direction | 165.0 |
| wind_speed | 2.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 733 |
| n_updates | 30524 |
-----------------------------------------------
-----------------------------------------------*******************************************************--------| 91%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 386.22852 |
| air_humidity | 26.344952 |
| air_temperature | 18.58754 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 30.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 18.0 |
| month | 11.0 |
| outdoor_humidity | 49.75 |
| outdoor_temperature | 5.560407 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 347605.66 |
| wind_direction | 310.0 |
| wind_speed | 8.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.12e+03 |
| n_updates | 30549 |
-----------------------------------------------
-----------------------------------------------*******************************************************--------| 91%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 1243.507 |
| air_humidity | 16.326004 |
| air_temperature | 20.84595 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 20.0 |
| month | 12.0 |
| outdoor_humidity | 48.0 |
| outdoor_temperature | 0.5393739 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1119156.2 |
| wind_direction | 307.5 |
| wind_speed | 12.325 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.52e+03 |
| n_updates | 30574 |
-----------------------------------------------
-----------------------------------------------********************************************************-------| 92%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 770.26825 |
| air_humidity | 20.772131 |
| air_temperature | 19.714325 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 2.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 15.0 |
| month | 12.0 |
| outdoor_humidity | 49.0 |
| outdoor_temperature | 4.096941 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 648078.06 |
| wind_direction | 297.5 |
| wind_speed | 6.925 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.1e+03 |
| n_updates | 30599 |
-----------------------------------------------
-----------------------------------------------********************************************************-------| 92%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 29.780336 |
| air_temperature | 18.484047 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 3.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 18.0 |
| month | 12.0 |
| outdoor_humidity | 57.75 |
| outdoor_temperature | 6.4706626 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 222.5 |
| wind_speed | 5.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.53e+03 |
| n_updates | 30624 |
-----------------------------------------------
-----------------------------------------------********************************************************-------| 92%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 413.7795 |
| air_humidity | 38.355236 |
| air_temperature | 21.587442 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 4.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 22.0 |
| month | 12.0 |
| outdoor_humidity | 91.5 |
| outdoor_temperature | 7.7593412 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 372401.56 |
| wind_direction | 245.0 |
| wind_speed | 2.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.32e+03 |
| n_updates | 30649 |
-----------------------------------------------
-----------------------------------------------*********************************************************------| 93%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 1199.713 |
| air_humidity | 35.19888 |
| air_temperature | 18.850016 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 6.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 18.0 |
| month | 12.0 |
| outdoor_humidity | 59.5 |
| outdoor_temperature | 0.7583431 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1079741.8 |
| wind_direction | 270.0 |
| wind_speed | 6.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.84e+03 |
| n_updates | 30674 |
-----------------------------------------------
------------------------------------------------********************************************************------| 93%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 1826.2998 |
| air_humidity | 24.840548 |
| air_temperature | 18.298168 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 7.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 1.0 |
| htg_setpoint | 15.0 |
| month | 12.0 |
| outdoor_humidity | 66.0 |
| outdoor_temperature | -2.3745906 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1643669.9 |
| wind_direction | 260.0 |
| wind_speed | 8.925 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 7.26e+03 |
| n_updates | 30699 |
------------------------------------------------
-----------------------------------------------*********************************************************------| 93%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 20.057957 |
| air_temperature | 18.570192 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 8.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 2.0 |
| htg_setpoint | 16.0 |
| month | 12.0 |
| outdoor_humidity | 54.0 |
| outdoor_temperature | 3.9157972 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 280.0 |
| wind_speed | 6.075 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.14e+03 |
| n_updates | 30724 |
-----------------------------------------------
-----------------------------------------------*********************************************************------| 93%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 1083.1313 |
| air_humidity | 22.157179 |
| air_temperature | 20.578194 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 9.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 3.0 |
| htg_setpoint | 17.0 |
| month | 12.0 |
| outdoor_humidity | 76.75 |
| outdoor_temperature | 1.341252 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 974818.2 |
| wind_direction | 0.0 |
| wind_speed | 0.525 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 7.08e+03 |
| n_updates | 30749 |
-----------------------------------------------
-----------------------------------------------**********************************************************-----| 94%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 36.529976 |
| air_temperature | 18.427694 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 10.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 4.0 |
| htg_setpoint | 16.0 |
| month | 12.0 |
| outdoor_humidity | 67.5 |
| outdoor_temperature | 2.9428682 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 37.5 |
| wind_speed | 1.125 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.56e+03 |
| n_updates | 30774 |
-----------------------------------------------
-----------------------------------------------**********************************************************-----| 94%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 43.5748 |
| air_temperature | 18.313513 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 11.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 5.0 |
| htg_setpoint | 18.0 |
| month | 12.0 |
| outdoor_humidity | 92.0 |
| outdoor_temperature | 2.2255049 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 27.5 |
| wind_speed | 3.975 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.09e+03 |
| n_updates | 30799 |
-----------------------------------------------
-----------------------------------------------**********************************************************-----| 94%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 244.03078 |
| air_humidity | 28.005653 |
| air_temperature | 21.000095 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 12.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 6.0 |
| htg_setpoint | 21.0 |
| month | 12.0 |
| outdoor_humidity | 92.0 |
| outdoor_temperature | 2.1828644 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 219627.69 |
| wind_direction | 27.5 |
| wind_speed | 4.35 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.07e+03 |
| n_updates | 30824 |
-----------------------------------------------
------------------------------------------------**********************************************************----| 95%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 551.1428 |
| air_humidity | 27.979132 |
| air_temperature | 18.311874 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 13.0 |
| diffuse_solar_radiation | 11.25 |
| direct_solar_radiation | 39.75 |
| hour | 7.0 |
| htg_setpoint | 15.0 |
| month | 12.0 |
| outdoor_humidity | 59.5 |
| outdoor_temperature | 0.46443972 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 496028.53 |
| wind_direction | 265.0 |
| wind_speed | 4.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.57e+03 |
| n_updates | 30849 |
------------------------------------------------
-------------------------------------------------*********************************************************----| 95%
| action_network/ | |
| index | 3 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 27 |
| Heating_Setpoint_RL | 18 |
| observation/ | |
| HVAC_electricity_demand_rate | 1375.0525 |
| air_humidity | 25.567287 |
| air_temperature | 18.014524 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 14.0 |
| diffuse_solar_radiation | 35.5 |
| direct_solar_radiation | 417.0 |
| hour | 8.0 |
| htg_setpoint | 18.0 |
| month | 12.0 |
| outdoor_humidity | 59.75 |
| outdoor_temperature | -0.11835387 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1237547.2 |
| wind_direction | 280.0 |
| wind_speed | 6.95 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.01e+03 |
| n_updates | 30874 |
-------------------------------------------------
------------------------------------------------**********************************************************----| 95%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 1464.2988 |
| air_humidity | 15.480985 |
| air_temperature | 17.915373 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 15.0 |
| diffuse_solar_radiation | 70.0 |
| direct_solar_radiation | 568.25 |
| hour | 9.0 |
| htg_setpoint | 15.0 |
| month | 12.0 |
| outdoor_humidity | 48.5 |
| outdoor_temperature | -0.5645857 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1317869.0 |
| wind_direction | 0.0 |
| wind_speed | 1.575 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 964 |
| n_updates | 30899 |
------------------------------------------------
-----------------------------------------------***********************************************************----| 95%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 906.4148 |
| air_humidity | 23.380638 |
| air_temperature | 23.220278 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 16.0 |
| diffuse_solar_radiation | 108.0 |
| direct_solar_radiation | 526.5 |
| hour | 10.0 |
| htg_setpoint | 22.0 |
| month | 12.0 |
| outdoor_humidity | 54.75 |
| outdoor_temperature | 4.6327314 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 815773.3 |
| wind_direction | 230.0 |
| wind_speed | 4.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.32e+03 |
| n_updates | 30924 |
-----------------------------------------------
-----------------------------------------------************************************************************---| 96%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 640.8608 |
| air_humidity | 23.445868 |
| air_temperature | 23.922201 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 17.0 |
| diffuse_solar_radiation | 53.0 |
| direct_solar_radiation | 789.0 |
| hour | 11.0 |
| htg_setpoint | 19.0 |
| month | 12.0 |
| outdoor_humidity | 30.75 |
| outdoor_temperature | 10.970597 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 560345.6 |
| wind_direction | 280.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 3.5e+03 |
| n_updates | 30949 |
-----------------------------------------------
-----------------------------------------------************************************************************---| 96%
| action_network/ | |
| index | 2 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 28 |
| Heating_Setpoint_RL | 17 |
| observation/ | |
| HVAC_electricity_demand_rate | 454.61838 |
| air_humidity | 22.946701 |
| air_temperature | 23.933338 |
| clg_setpoint | 28.0 |
| co2_emission | 0.0 |
| day_of_month | 18.0 |
| diffuse_solar_radiation | 130.25 |
| direct_solar_radiation | 570.25 |
| hour | 12.0 |
| htg_setpoint | 17.0 |
| month | 12.0 |
| outdoor_humidity | 43.75 |
| outdoor_temperature | 7.093073 |
| people_occupant | 8.0 |
| total_electricity_HVAC | 410771.34 |
| wind_direction | 0.0 |
| wind_speed | 3.1 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.21e+03 |
| n_updates | 30974 |
-----------------------------------------------
-----------------------------------------------************************************************************---| 96%
| action_network/ | |
| index | 0 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 30 |
| Heating_Setpoint_RL | 15 |
| observation/ | |
| HVAC_electricity_demand_rate | 560.646 |
| air_humidity | 24.925459 |
| air_temperature | 23.773348 |
| clg_setpoint | 30.0 |
| co2_emission | 0.0 |
| day_of_month | 19.0 |
| diffuse_solar_radiation | 180.75 |
| direct_solar_radiation | 201.5 |
| hour | 13.0 |
| htg_setpoint | 15.0 |
| month | 12.0 |
| outdoor_humidity | 43.75 |
| outdoor_temperature | 10.055126 |
| people_occupant | 16.0 |
| total_electricity_HVAC | 504581.38 |
| wind_direction | 237.5 |
| wind_speed | 6.325 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.05e+03 |
| n_updates | 30999 |
-----------------------------------------------
-----------------------------------------------************************************************************---| 96%
| action_network/ | |
| index | 9 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 28.871876 |
| air_temperature | 23.722929 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 20.0 |
| diffuse_solar_radiation | 100.0 |
| direct_solar_radiation | 30.25 |
| hour | 14.0 |
| htg_setpoint | 21.0 |
| month | 12.0 |
| outdoor_humidity | 55.5 |
| outdoor_temperature | 10.191752 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 340.0 |
| wind_speed | 5.05 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.4e+03 |
| n_updates | 31024 |
-----------------------------------------------
-----------------------------------------------*************************************************************--| 97%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 16.536386 |
| air_temperature | 23.418673 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 21.0 |
| diffuse_solar_radiation | 40.25 |
| direct_solar_radiation | 492.75 |
| hour | 15.0 |
| htg_setpoint | 22.0 |
| month | 12.0 |
| outdoor_humidity | 29.0 |
| outdoor_temperature | 3.5819852 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 345.0 |
| wind_speed | 5.3 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 622 |
| n_updates | 31049 |
-----------------------------------------------
-----------------------------------------------*************************************************************--| 97%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 1063.0789 |
| air_humidity | 13.744133 |
| air_temperature | 21.999746 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 22.0 |
| diffuse_solar_radiation | 19.25 |
| direct_solar_radiation | 46.75 |
| hour | 16.0 |
| htg_setpoint | 22.0 |
| month | 12.0 |
| outdoor_humidity | 56.75 |
| outdoor_temperature | 1.4415144 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 956770.94 |
| wind_direction | 57.5 |
| wind_speed | 2.725 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.55e+04 |
| n_updates | 31074 |
-----------------------------------------------
-----------------------------------------------*************************************************************--| 97%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 859.9368 |
| air_humidity | 31.90969 |
| air_temperature | 22.74368 |
| clg_setpoint | 22.5 |
| co2_emission | 0.0 |
| day_of_month | 23.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 17.0 |
| htg_setpoint | 22.0 |
| month | 12.0 |
| outdoor_humidity | 81.25 |
| outdoor_temperature | 3.8199086 |
| people_occupant | 20.0 |
| total_electricity_HVAC | 773943.1 |
| wind_direction | 335.0 |
| wind_speed | 4.0 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.4e+03 |
| n_updates | 31099 |
-----------------------------------------------
-----------------------------------------------**************************************************************-| 98%
| action_network/ | |
| index | 5 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 25 |
| Heating_Setpoint_RL | 20 |
| observation/ | |
| HVAC_electricity_demand_rate | 1086.9948 |
| air_humidity | 25.50109 |
| air_temperature | 23.585283 |
| clg_setpoint | 25.0 |
| co2_emission | 0.0 |
| day_of_month | 24.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 18.0 |
| htg_setpoint | 20.0 |
| month | 12.0 |
| outdoor_humidity | 69.5 |
| outdoor_temperature | 1.3219347 |
| people_occupant | 10.0 |
| total_electricity_HVAC | 978295.25 |
| wind_direction | 105.0 |
| wind_speed | 2.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.51e+03 |
| n_updates | 31124 |
-----------------------------------------------
-----------------------------------------------**************************************************************-| 98%
| action_network/ | |
| index | 8 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 22.5 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 362.91885 |
| air_humidity | 38.318344 |
| air_temperature | 21.535563 |
| clg_setpoint | 27.0 |
| co2_emission | 0.0 |
| day_of_month | 25.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 19.0 |
| htg_setpoint | 18.0 |
| month | 12.0 |
| outdoor_humidity | 74.5 |
| outdoor_temperature | 8.044543 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 319316.4 |
| wind_direction | 260.0 |
| wind_speed | 4.75 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.68e+03 |
| n_updates | 31149 |
-----------------------------------------------
-----------------------------------------------**************************************************************-| 98%
| action_network/ | |
| index | 1 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 29 |
| Heating_Setpoint_RL | 16 |
| observation/ | |
| HVAC_electricity_demand_rate | 692.0048 |
| air_humidity | 28.121815 |
| air_temperature | 19.953701 |
| clg_setpoint | 29.0 |
| co2_emission | 0.0 |
| day_of_month | 26.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 20.0 |
| htg_setpoint | 16.0 |
| month | 12.0 |
| outdoor_humidity | 52.0 |
| outdoor_temperature | 5.671339 |
| people_occupant | 2.0 |
| total_electricity_HVAC | 596618.9 |
| wind_direction | 262.5 |
| wind_speed | 7.45 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 6.86e+03 |
| n_updates | 31174 |
-----------------------------------------------
-----------------------------------------------**************************************************************-| 98%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1085.7454 |
| air_humidity | 37.932785 |
| air_temperature | 20.481953 |
| clg_setpoint | 26.0 |
| co2_emission | 0.0 |
| day_of_month | 27.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 21.0 |
| htg_setpoint | 19.0 |
| month | 12.0 |
| outdoor_humidity | 96.0 |
| outdoor_temperature | 1.3281816 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 977170.8 |
| wind_direction | 7.5 |
| wind_speed | 7.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 5.9e+03 |
| n_updates | 31199 |
-----------------------------------------------
------------------------------------------------**************************************************************| 99%
| action_network/ | |
| index | 7 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 23 |
| Heating_Setpoint_RL | 22 |
| observation/ | |
| HVAC_electricity_demand_rate | 1320.7164 |
| air_humidity | 19.061243 |
| air_temperature | 22.000002 |
| clg_setpoint | 23.0 |
| co2_emission | 0.0 |
| day_of_month | 28.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 22.0 |
| htg_setpoint | 22.0 |
| month | 12.0 |
| outdoor_humidity | 54.5 |
| outdoor_temperature | 0.15332632 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1188644.8 |
| wind_direction | 40.0 |
| wind_speed | 2.225 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 2.23e+03 |
| n_updates | 31224 |
------------------------------------------------
-----------------------------------------------***************************************************************| 99%
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 951.3817 |
| air_humidity | 29.622356 |
| air_temperature | 18.660505 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 29.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 23.0 |
| htg_setpoint | 21.0 |
| month | 12.0 |
| outdoor_humidity | 96.75 |
| outdoor_temperature | 3.5307813 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 856243.5 |
| wind_direction | 27.5 |
| wind_speed | 12.475 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.66e+03 |
| n_updates | 31249 |
-----------------------------------------------
-----------------------------------------------***************************************************************| 99%
| action_network/ | |
| index | 6 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 24 |
| Heating_Setpoint_RL | 21 |
| observation/ | |
| HVAC_electricity_demand_rate | 1123.5736 |
| air_humidity | 28.958847 |
| air_temperature | 21.000471 |
| clg_setpoint | 24.0 |
| co2_emission | 0.0 |
| day_of_month | 31.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 21.0 |
| month | 12.0 |
| outdoor_humidity | 56.0 |
| outdoor_temperature | 1.1390406 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 1011216.2 |
| wind_direction | 252.5 |
| wind_speed | 11.05 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 4.89e+03 |
| n_updates | 31274 |
-----------------------------------------------
[WRAPPER LoggerWrapper] (INFO) : End of episode detected, recording summary (progress.csv) if logger is active
#----------------------------------------------------------------------------------------------#
[ENVIRONMENT] (INFO) : Starting a new episode... [SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43] [Episode 6]
#----------------------------------------------------------------------------------------------#
[MODELING] (INFO) : Episode directory created [/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43-res1/Eplus-env-sub_run6]
[MODELING] (INFO) : Weather file USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3.epw used.
[MODELING] (INFO) : Updated building model with whole Output:Variable available names
[MODELING] (INFO) : Updated building model with whole Output:Meter available names
[MODELING] (INFO) : Adapting weather to building model. [USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3.epw]
[ENVIRONMENT] (INFO) : Saving episode output path... [/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43-res1/Eplus-env-sub_run6/output]
[SIMULATOR] (INFO) : Running EnergyPlus with args: ['-w', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43-res1/Eplus-env-sub_run6/USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3_Random_1.0_0.0_0.001.epw', '-d', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43-res1/Eplus-env-sub_run6/output', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43-res1/Eplus-env-sub_run6/5ZoneAutoDXVAV.epJSON']
[ENVIRONMENT] (INFO) : Episode 6 started.
/usr/local/lib/python3.10/dist-packages/opyplus/weather_data/weather_data.py:493: FutureWarning: the 'line_terminator'' keyword is deprecated, use 'lineterminator' instead.
epw_content = self._headers_to_epw(use_datetimes=use_datetimes) + df.to_csv(
[SIMULATOR] (INFO) : handlers are ready.
[SIMULATOR] (INFO) : System is ready.
[WRAPPER LoggerWrapper] (INFO) : Creating monitor.csv for current episode (episode 6) if logger is active
-----------------------------------------------------
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| episode/ | |
| comfort_violation_time(%) | 43.7 |
| cumulative_comfort_penalty | -2.27e+04 |
| cumulative_energy_penalty | -3.56e+03 |
| cumulative_power | 7.11e+07 |
| cumulative_reward | -26294.172 |
| cumulative_temperature_violation | 4.55e+04 |
| episode_length | 70077 |
| mean_comfort_penalty | -0.324 |
| mean_energy_penalty | -0.0507 |
| mean_power | 1.01e+03 |
| mean_reward | -0.37521827 |
| mean_temperature_violation | 0.649 |
| observation/ | |
| HVAC_electricity_demand_rate | 3207.3267 |
| air_humidity | 17.21404 |
| air_temperature | 16.906685 |
| clg_setpoint | 40.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 12.8 |
| month | 1.0 |
| outdoor_humidity | 43.25 |
| outdoor_temperature | -9.279724 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2886594.0 |
| wind_direction | 305.0 |
| wind_speed | 8.4 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.33e+04 |
| n_updates | 31298 |
-----------------------------------------------------
-----------------------------------
| rollout/ | |
| ep_len_mean | 3.5e+04 |
| ep_rew_mean | -1.25e+04 |
| exploration_rate | 0.05 |
| time/ | |
| episodes | 3 |
| fps | 815 |
| time_elapsed | 214 |
| total_timesteps | 175194 |
-----------------------------------
------------------------------------------------
| action_network/ | |
| index | 4 |
| action_simulation/ | |
| Cooling_Setpoint_RL | 26 |
| Heating_Setpoint_RL | 19 |
| observation/ | |
| HVAC_electricity_demand_rate | 2556.6965 |
| air_humidity | 17.018936 |
| air_temperature | 16.829346 |
| clg_setpoint | 40.0 |
| co2_emission | 0.0 |
| day_of_month | 1.0 |
| diffuse_solar_radiation | 0.0 |
| direct_solar_radiation | 0.0 |
| hour | 0.0 |
| htg_setpoint | 12.8 |
| month | 1.0 |
| outdoor_humidity | 57.75 |
| outdoor_temperature | -6.0265746 |
| people_occupant | 0.0 |
| total_electricity_HVAC | 2301027.0 |
| wind_direction | 275.0 |
| wind_speed | 7.6 |
| rollout/ | |
| exploration_rate | 0.05 |
| train/ | |
| learning_rate | 0.0001 |
| loss | 1.6e+04 |
| n_updates | 31299 |
------------------------------------------------
/usr/local/lib/python3.10/dist-packages/gymnasium/core.py:311: UserWarning: WARN: env.observation_variables to get variables from other wrappers is deprecated and will be removed in v1.0, to get this variable you can do `env.unwrapped.observation_variables` for environment variables or `env.get_wrapper_attr('observation_variables')` that will search the reminding wrappers.
logger.warn(
/usr/local/lib/python3.10/dist-packages/gymnasium/core.py:311: UserWarning: WARN: env.action_variables to get variables from other wrappers is deprecated and will be removed in v1.0, to get this variable you can do `env.unwrapped.action_variables` for environment variables or `env.get_wrapper_attr('action_variables')` that will search the reminding wrappers.
logger.warn(
/usr/local/lib/python3.10/dist-packages/gymnasium/core.py:311: UserWarning: WARN: env.is_discrete to get variables from other wrappers is deprecated and will be removed in v1.0, to get this variable you can do `env.unwrapped.is_discrete` for environment variables or `env.get_wrapper_attr('is_discrete')` that will search the reminding wrappers.
logger.warn(
/usr/local/lib/python3.10/dist-packages/gymnasium/spaces/box.py:240: UserWarning: WARN: Casting input x to numpy array.
gym.logger.warn("Casting input x to numpy array.")
[11]:
<stable_baselines3.dqn.dqn.DQN at 0x7f6d49d5f670>
wandb: WARNING (User provided step: 175194 is less than current step: 175195. Dropping entry: {'rollout/ep_len_mean': 35040.0, '_timestamp': 1700214442.738834}).
wandb: WARNING (User provided step: 175194 is less than current step: 175195. Dropping entry: {'rollout/ep_rew_mean': -12505.215683, '_timestamp': 1700214442.7389145}).
wandb: WARNING (User provided step: 175194 is less than current step: 175195. Dropping entry: {'rollout/exploration_rate': 0.05, '_timestamp': 1700214442.738949}).
wandb: WARNING (User provided step: 175194 is less than current step: 175195. Dropping entry: {'time/episodes': 3, '_timestamp': 1700214442.7389746}).
wandb: WARNING (User provided step: 175194 is less than current step: 175195. Dropping entry: {'time/fps': 815, '_timestamp': 1700214442.7390523}).
wandb: WARNING (User provided step: 175194 is less than current step: 175195. Dropping entry: {'time/time_elapsed': 214, '_timestamp': 1700214442.739078}).
wandb: WARNING (User provided step: 175194 is less than current step: 175195. Dropping entry: {'time/total_timesteps': 175194, '_timestamp': 1700214442.7391007}).
Now, we save the current model (model version when training has finished).
[12]:
model.save(str(env.get_wrapper_attr('timestep_per_episode'))+ '/' + experiment_name)
/usr/local/lib/python3.10/dist-packages/stable_baselines3/common/save_util.py:278: UserWarning: Path '35040' does not exist. Will create it.
warnings.warn(f"Path '{path.parent}' does not exist. Will create it.")
And as always, remember to close the environment.
[13]:
env.close()
[WRAPPER LoggerWrapper] (INFO) : End of episode, recording summary (progress.csv) if logger is active
Progress: |***************************************************************************************************| 99%
[ENVIRONMENT] (INFO) : Environment closed. [SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43]
We have to upload all Sinergym output as wandb artifact. This output include all sinergym_output (and LoggerWrapper CSV files) and models generated in training and evaluation episodes.
[14]:
artifact = wandb.Artifact(
name="experiment1",
type="training")
artifact.add_dir(
env.get_wrapper_attr('workspace_path'),
name='training_output/')
artifact.add_dir(
eval_env.get_wrapper_attr('workspace_path'),
name='evaluation_output/')
run.log_artifact(artifact)
# wandb has finished
run.finish()
wandb: Adding directory to artifact (/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43-res1)... Done. 0.1s
wandb: Adding directory to artifact (/workspaces/sinergym/examples/Eplus-env-SB3_DQN-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:43_EVALUATION-res1)... Done. 0.0s
wandb: WARNING Source type is set to 'repo' but some required information is missing from the environment. A job will not be created from this run. See https://docs.wandb.ai/guides/launch/create-job
Run history:
action_network/index | ▃▃█▆▁▆▅▆▆▃▃▇▁▃█▅▅▇▂▂▃▄▁▅▇▂▇▁▅▃█▃▇▄▅▇▄▇▃▁ |
action_simulation/Cooling_Setpoint_RL | ▆▅▁▁█▁▃▂▂▅▆▁█▆▁▃▃▁▇▇▅▄█▃▁▇▁█▃▅▁▅▁▄▃▁▄▁▅█ |
action_simulation/Heating_Setpoint_RL | ▃▄▇█▁█▆▇▇▄▃█▁▃▇▆▆█▂▂▄▅▁▆█▂█▁▆▄▇▄█▅▆█▅█▄▁ |
episode/comfort_violation_time(%) | ▁▁█ |
episode/cumulative_comfort_penalty | █▂▁ |
episode/cumulative_energy_penalty | █▁▁ |
episode/cumulative_power | ▁██ |
episode/cumulative_reward | █▂▁ |
episode/cumulative_temperature_violation | ▁▇█ |
episode/episode_length | ▁██ |
episode/mean_comfort_penalty | █▅▁ |
episode/mean_energy_penalty | ▁█▄ |
episode/mean_power | █▁▅ |
episode/mean_reward | █▅▁ |
episode/mean_temperature_violation | ▁▄█ |
eval/comfort_violation(%) | ▁█ |
eval/cumulative_comfort_penalty | █▁ |
eval/cumulative_energy_penalty | ▁█ |
eval/cumulative_power_consumption | █▁ |
eval/cumulative_reward | █▁ |
eval/cumulative_temperature_violation | ▁█ |
eval/episode_length | ▁▁ |
eval/mean_comfort_penalty | █▁ |
eval/mean_energy_penalty | ▁█ |
eval/mean_power_consumption | █▁ |
eval/mean_reward | █▁ |
eval/mean_temperature_violation | ▁█ |
eval/std_cumulative_reward | ▁▁ |
eval/std_reward | ▁▁ |
observation/HVAC_electricity_demand_rate | ▂▂▁▁▁▄▁▁▁▂▁▆▁▁▁▁▂▂▁▁▁▁▂▂▁▁▂▁▁▁▁▂▁▂▁▁█▅▁▂ |
observation/air_humidity | ▂▂▅▆▇▆▆▃▃▂▁▅▅▆▆▂▄▃▅▇██▄▂▂▂▄▆▆▆▂▁▁▂▇▆▅▃▂▂ |
observation/air_temperature | ▆▃▄▅▅▄▂▂▂▅▆▅█▇▃▂▁▂▂▄▅▃▇▂▄▃▄▆▇▆▃▁▄▃▂▆▅▅▇▅ |
observation/clg_setpoint | ▁▁▄█▅▁█▄▃▆▇▅█▁▁▃▇▄▂▅▁▄█▃▁▇▁█▃▅▁▅▁▁▁▁▄▁▅█ |
observation/co2_emission | ▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁ |
observation/day_of_month | ▅▅▃█▅▆▃█▅▅▃█▅▃▃█▅▃▄▁▆▃▄▁▅▃█▁▆▃█▁▅▃█▁▆▃█▅ |
observation/diffuse_solar_radiation | ▂▁▁▁▁▃▄▁▁▃▄▆▇▄▁▁▁▁▂▃▂▂▄▂▃▇▅▁▁▁▁▁▁▁▁▃█▃▃▄ |
observation/direct_solar_radiation | ▄▁▁▁▁▇▄▁▁▆▇▆▄▁▁▁▁▁▅▅▁▁▅▃▆▄▁▁▁▁▁▁▁▁▁█▅█▇▃ |
observation/hour | ▅████▂▂▂▁▅▅▅▅▅████▁▁▁▁▅▅▄▄▄▇▇▇▇▁▁▁▁▄▄▄▄▄ |
observation/htg_setpoint | █▇▅▁▄█▁▅▆▃▂▄▁█▇▆▂▅▇▄█▅▁▆█▂█▁▆▄▇▄█▇██▅█▄▁ |
observation/month | ▁▂▃▄▅▅▇▇▁▂▃▄▅▆▇▇▁▂▃▄▅▆▇█▁▂▃▄▅▆▇█▁▂▃▄▅▆▇█ |
observation/outdoor_humidity | ▃▅▃▆█▄█▆█▄▁▄▆▆▇▄▇▇▃▇▇▇▆▃▃▄▆▄▇▇▄▃▃▅█▂▆▂▄▃ |
observation/outdoor_temperature | ▄▂▃▆██▅▃▃▃▅██▆▅▂▂▂▂▆█▆▆▂▃▂▄▆▇▆▄▁▂▁▄▆█▇▅▃ |
observation/people_occupant | █▁▁▁▁▁▁▁▁███▁▁▁▁▁▁▁▁▁▁▁▁▁▁▇▁▁▁▁▁▁▁▁▁▇▇▇▇ |
observation/total_electricity_HVAC | ▂▂▁▁▁▄▁▁▁▂▁▆▁▁▁▁▂▂▁▁▁▁▂▂▁▁▂▁▁▁▁▂▁▂▁▁█▅▁▂ |
observation/wind_direction | ▅▅▇▁▅█▆▇▃▃▇▄▅▂▁▇▆▃█▅▆▂▅▇▆▂▃█▅▂▆▇▇█▃▇▅▁█▆ |
observation/wind_speed | ▂▃▆▁▃▄▃▅▅▃█▅▄▃▁▆▅▄▅▃▃▂▃█▆▆▄▅▅▂▃▇▄▅▃▄▅▅▃▄ |
rollout/exploration_rate | █▇▄▂▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁ |
time/total_timesteps | ▁█ |
train/learning_rate | ▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁▁ |
train/loss | ▇▁▃▂▃▂▁▂▁▂▂▁▂▃▃▁▂▁▁▂▂▁▃▃▂▂█▂▃▂▂▂▁▂▂▁▂▁▁▂ |
train/n_updates | ▁▁▁▁▂▂▂▂▂▃▃▃▃▃▃▄▄▄▄▄▅▅▅▅▅▅▆▆▆▆▆▇▇▇▇▇▇███ |
Run summary:
action_network/index | 4 |
action_simulation/Cooling_Setpoint_RL | 26 |
action_simulation/Heating_Setpoint_RL | 19 |
episode/comfort_violation_time(%) | 43.69622 |
episode/cumulative_comfort_penalty | -22738.12343 |
episode/cumulative_energy_penalty | -3556.04736 |
episode/cumulative_power | 71120947.22879 |
episode/cumulative_reward | -26294.17188 |
episode/cumulative_temperature_violation | 45476.24685 |
episode/episode_length | 70077 |
episode/mean_comfort_penalty | -0.32447 |
episode/mean_energy_penalty | -0.05074 |
episode/mean_power | 1014.89714 |
episode/mean_reward | -0.37522 |
episode/mean_temperature_violation | 0.64895 |
eval/comfort_violation(%) | 58.24201 |
eval/cumulative_comfort_penalty | -17670.69841 |
eval/cumulative_energy_penalty | -1708.48282 |
eval/cumulative_power_consumption | 34169656.38755 |
eval/cumulative_reward | -19379.18123 |
eval/cumulative_temperature_violation | 35341.39683 |
eval/episode_length | 35040.0 |
eval/mean_comfort_penalty | -0.5043 |
eval/mean_energy_penalty | -0.04876 |
eval/mean_power_consumption | 975.16143 |
eval/mean_reward | -0.55306 |
eval/mean_temperature_violation | 1.0086 |
eval/std_cumulative_reward | 0.0 |
eval/std_reward | 0.0 |
observation/HVAC_electricity_demand_rate | 2556.69653 |
observation/air_humidity | 17.01894 |
observation/air_temperature | 16.82935 |
observation/clg_setpoint | 40.0 |
observation/co2_emission | 0.0 |
observation/day_of_month | 1.0 |
observation/diffuse_solar_radiation | 0.0 |
observation/direct_solar_radiation | 0.0 |
observation/hour | 0.0 |
observation/htg_setpoint | 12.8 |
observation/month | 1.0 |
observation/outdoor_humidity | 57.75 |
observation/outdoor_temperature | -6.02657 |
observation/people_occupant | 0.0 |
observation/total_electricity_HVAC | 2301027.0 |
observation/wind_direction | 275.0 |
observation/wind_speed | 7.6 |
rollout/exploration_rate | 0.05 |
time/total_timesteps | 140154 |
train/learning_rate | 0.0001 |
train/loss | 16037.58984 |
train/n_updates | 31299 |
Synced 5 W&B file(s), 0 media file(s), 150 artifact file(s) and 0 other file(s)
./wandb/run-20231117_094304-pf27dlou/logs
We have all the experiments results in our local computer, but we can see the execution in wandb too:
If we check our projects, we can see the execution allocated:
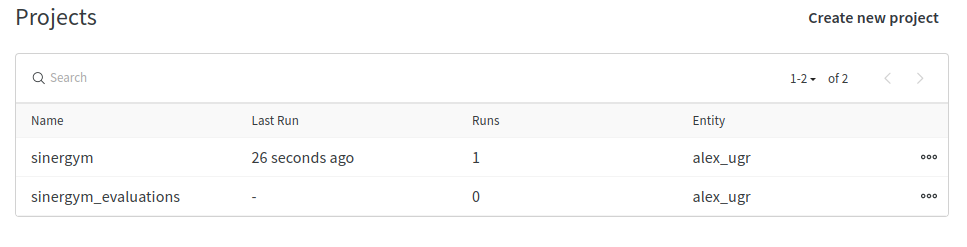
Hyperparameters tracked in the training experiment:
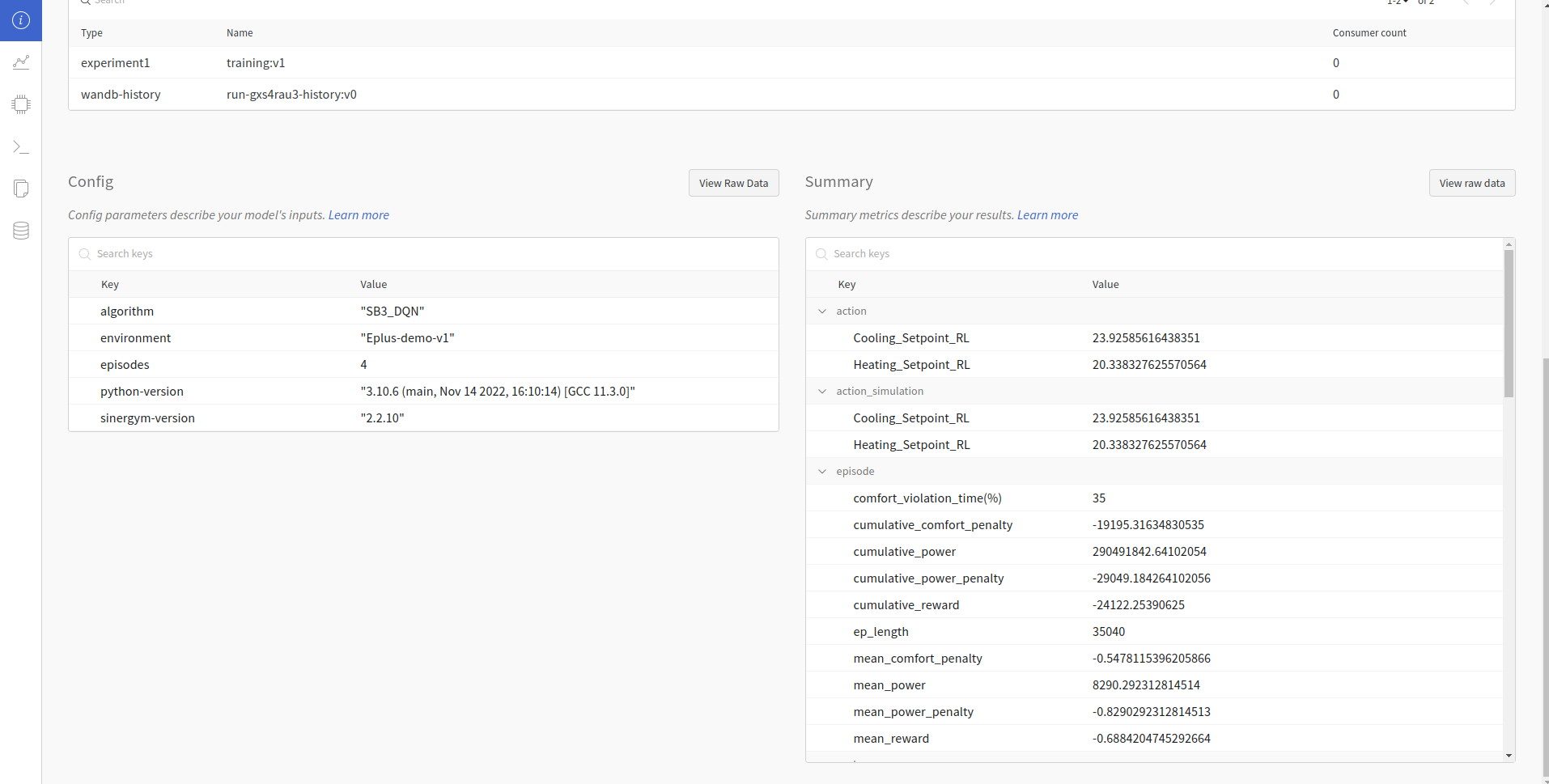
Artifacts registered (if evaluation is enabled, best model is registered too):

Visualization of metrics in real time:
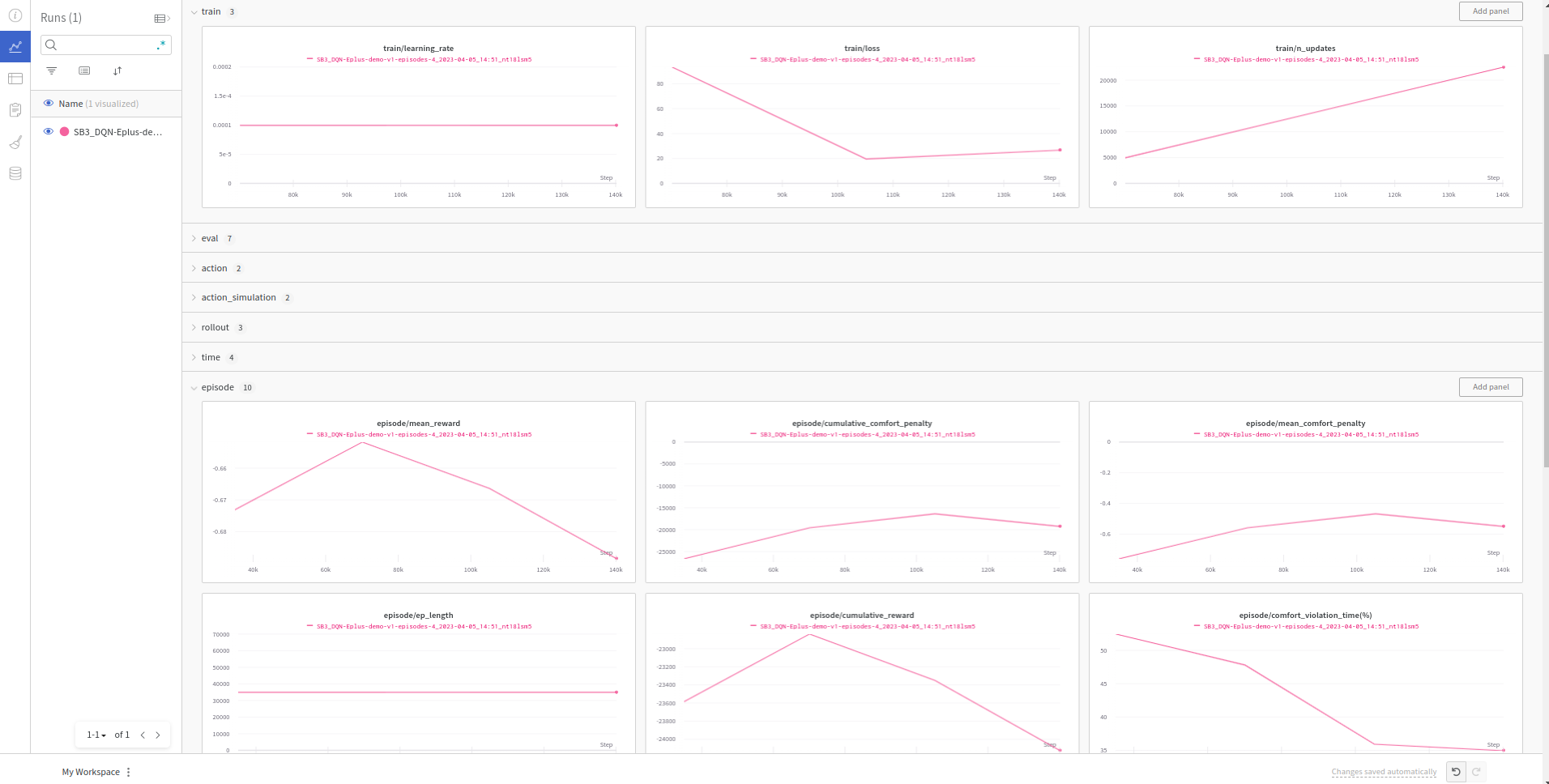
23.2. Loading a model
We are going to rely on the script available in the repository root called load_agent.py
. This script applies all the possibilities that Sinergym has to work with deep reinforcement learning models loaded and set parameters to everything, so that we can define the load options from the execution of the script easily by a JSON file.
For more information about how run load_agent.py
, please, see Load a trained model.
First, we define the Sinergym environment ID where we want to check the loaded agent and the name of the evaluation experiment.
[15]:
# Environment ID
environment = "Eplus-5zone-mixed-discrete-stochastic-v1"
# Episodes
episodes=5
# Evaluation name
evaluation_date = datetime.today().strftime('%Y-%m-%d_%H:%M')
evaluation_name = 'SB3_DQN-EVAL-' + environment + \
'-episodes-' + str(episodes)
evaluation_name += '_' + evaluation_date
We can also use wandb here. We can allocate this evaluation of a loaded model in other project in order to not merge experiments.
[16]:
# Create wandb.config object in order to log all experiment params
experiment_params = {
'sinergym-version': sinergym.__version__,
'python-version': sys.version
}
experiment_params.update({'environment':environment,
'episodes':episodes,
'algorithm':'SB3_DQN'})
# Get wandb init params (you have to specify your own project and entity)
wandb_params = {"project": 'sinergym_evaluations',
"entity": 'alex_ugr'}
# Init wandb entry
run = wandb.init(
name=experiment_name + '_' + wandb.util.generate_id(),
config=experiment_params,
** wandb_params
)
/workspaces/sinergym/examples/wandb/run-20231117_094859-15owsnpb
We make the gymnasium environment, it is important to wrap the environment with the same wrappers as used in training. We can use the evaluation experiment name to rename the environment.
[17]:
env = gym.make(environment, env_name=evaluation_name)
env = LoggerWrapper(env)
#==============================================================================================#
[ENVIRONMENT] (INFO) : Creating Gymnasium environment... [SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:48]
#==============================================================================================#
[MODELING] (INFO) : Experiment working directory created [/workspaces/sinergym/examples/Eplus-env-SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:48-res35041]
[MODELING] (INFO) : runperiod established: {'start_day': 1, 'start_month': 1, 'start_year': 1991, 'end_day': 31, 'end_month': 12, 'end_year': 1991, 'start_weekday': 1, 'n_steps_per_hour': 4}
[MODELING] (INFO) : Episode length (seconds): 31536000.0
[MODELING] (INFO) : timestep size (seconds): 900.0
[MODELING] (INFO) : timesteps per episode: 35040
[MODELING] (INFO) : Model Config is correct.
[REWARD] (INFO) : Reward function initialized.
[ENVIRONMENT] (INFO) : Environment SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:48 created successfully.
[WRAPPER DiscretizeEnv] (INFO) : New Discrete Space and mapping: Discrete(10)
[WRAPPER DiscretizeEnv] (INFO) : Make sure that the action space is compatible and contained in the original environment.
[WRAPPER DiscretizeEnv] (INFO) : Wrapper initialized
[WRAPPER LoggerWrapper] (INFO) : Wrapper initialized.
We load the Stable Baselines 3 DQN model using the model allocated in our local computer, although we can use a remote model allocated in wandb from other training experiment.
[18]:
# get wandb artifact path (to load model)
load_artifact_entity = 'alex_ugr'
load_artifact_project = 'sinergym'
load_artifact_name = 'experiment1'
load_artifact_tag = 'latest'
load_artifact_model_path = 'evaluation_output/best_model/model.zip'
wandb_path = load_artifact_entity + '/' + load_artifact_project + \
'/' + load_artifact_name + ':' + load_artifact_tag
# Download artifact
artifact = run.use_artifact(wandb_path)
artifact.get_path(load_artifact_model_path).download('.')
# Set model path to local wandb file downloaded
model_path = './' + load_artifact_model_path
model = DQN.load(model_path)
As we can see, The wandb model we want to load can come from an artifact of an different entity or project from the one we are using to register the evaluation of the loaded model, as long as it is accessible. The next step is use the model to predict actions and interact with the environment in order to collect data to evaluate the model.
[19]:
for i in range(episodes):
obs, info = env.reset()
rewards = []
terminated = False
current_month = 0
while not terminated:
a, _ = model.predict(obs)
obs, reward, terminated, truncated, info = env.step(a)
rewards.append(reward)
if info['month'] != current_month:
current_month = info['month']
print(info['month'], sum(rewards))
print(
'Episode ',
i,
'Mean reward: ',
np.mean(rewards),
'Cumulative reward: ',
sum(rewards))
env.close()
#----------------------------------------------------------------------------------------------#
[ENVIRONMENT] (INFO) : Starting a new episode... [SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:48] [Episode 1]
#----------------------------------------------------------------------------------------------#
[MODELING] (INFO) : Episode directory created [/workspaces/sinergym/examples/Eplus-env-SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:48-res35041/Eplus-env-sub_run1]
[MODELING] (INFO) : Weather file USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3.epw used.
[MODELING] (INFO) : Updated building model with whole Output:Variable available names
[MODELING] (INFO) : Updated building model with whole Output:Meter available names
[MODELING] (INFO) : Adapting weather to building model. [USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3.epw]
[ENVIRONMENT] (INFO) : Saving episode output path... [/workspaces/sinergym/examples/Eplus-env-SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:48-res35041/Eplus-env-sub_run1/output]
[SIMULATOR] (INFO) : Running EnergyPlus with args: ['-w', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:48-res35041/Eplus-env-sub_run1/USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3_Random_1.0_0.0_0.001.epw', '-d', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:48-res35041/Eplus-env-sub_run1/output', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:48-res35041/Eplus-env-sub_run1/5ZoneAutoDXVAV.epJSON']
[ENVIRONMENT] (INFO) : Episode 1 started.
/usr/local/lib/python3.10/dist-packages/opyplus/weather_data/weather_data.py:493: FutureWarning: the 'line_terminator'' keyword is deprecated, use 'lineterminator' instead.
epw_content = self._headers_to_epw(use_datetimes=use_datetimes) + df.to_csv(
[SIMULATOR] (INFO) : handlers initialized.
[SIMULATOR] (INFO) : handlers are ready.
[SIMULATOR] (INFO) : System is ready.
[WRAPPER LoggerWrapper] (INFO) : Creating monitor.csv for current episode (episode 1) if logger is active
1 -1.7954676783357755
Progress: |*--------------------------------------------------------------------------------------------------| 1%
/usr/local/lib/python3.10/dist-packages/gymnasium/spaces/box.py:240: UserWarning: WARN: Casting input x to numpy array.
gym.logger.warn("Casting input x to numpy array.")
2 -963.9496999955164------------------------------------------------------------------------------------------| 9%
3 -1817.582571129998*******-----------------------------------------------------------------------------------| 16%
4 -2841.5036276598917***************--------------------------------------------------------------------------| 25%
5 -4340.565445997684************************------------------------------------------------------------------| 33%
6 -6108.581378312066********************************----------------------------------------------------------| 41%
7 -7223.767956524665*****************************************-------------------------------------------------| 50%
8 -8558.958125000907************************************************------------------------------------------| 58%
9 -9950.644160496227*********************************************************---------------------------------| 66%
10 -11044.144766497926****************************************************************------------------------| 75%
11 -13176.534777951341************************************************************************----------------| 83%
12 -14049.395840884017********************************************************************************--------| 91%
Progress: |***************************************************************************************************| 99%
Episode 0 Mean reward: -0.4263319241570109 Cumulative reward: -14938.670622460524
[WRAPPER LoggerWrapper] (INFO) : End of episode detected, recording summary (progress.csv) if logger is active
#----------------------------------------------------------------------------------------------#
[ENVIRONMENT] (INFO) : Starting a new episode... [SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:48] [Episode 2]
#----------------------------------------------------------------------------------------------#
[MODELING] (INFO) : Episode directory created [/workspaces/sinergym/examples/Eplus-env-SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:48-res35041/Eplus-env-sub_run2]
[MODELING] (INFO) : Weather file USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3.epw used.
[MODELING] (INFO) : Updated building model with whole Output:Variable available names
[MODELING] (INFO) : Updated building model with whole Output:Meter available names
[MODELING] (INFO) : Adapting weather to building model. [USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3.epw]
[ENVIRONMENT] (INFO) : Saving episode output path... [/workspaces/sinergym/examples/Eplus-env-SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:48-res35041/Eplus-env-sub_run2/output]
[SIMULATOR] (INFO) : Running EnergyPlus with args: ['-w', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:48-res35041/Eplus-env-sub_run2/USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3_Random_1.0_0.0_0.001.epw', '-d', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:48-res35041/Eplus-env-sub_run2/output', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:48-res35041/Eplus-env-sub_run2/5ZoneAutoDXVAV.epJSON']
[ENVIRONMENT] (INFO) : Episode 2 started.
/usr/local/lib/python3.10/dist-packages/opyplus/weather_data/weather_data.py:493: FutureWarning: the 'line_terminator'' keyword is deprecated, use 'lineterminator' instead.
epw_content = self._headers_to_epw(use_datetimes=use_datetimes) + df.to_csv(
[SIMULATOR] (INFO) : handlers are ready.
[SIMULATOR] (INFO) : System is ready.
[WRAPPER LoggerWrapper] (INFO) : Creating monitor.csv for current episode (episode 2) if logger is active
1 -1.7052782846459695
Progress: |**-------------------------------------------------------------------------------------------------| 2%
/usr/local/lib/python3.10/dist-packages/gymnasium/spaces/box.py:240: UserWarning: WARN: Casting input x to numpy array.
gym.logger.warn("Casting input x to numpy array.")
2 -936.5481180476335------------------------------------------------------------------------------------------| 9%
3 -1798.784133735751*******-----------------------------------------------------------------------------------| 16%
4 -2827.2387312028445***************--------------------------------------------------------------------------| 25%
5 -4366.328889075081************************------------------------------------------------------------------| 33%
6 -6183.997471720583********************************----------------------------------------------------------| 41%
7 -7325.563685767946*****************************************-------------------------------------------------| 50%
8 -8681.46846670104*************************************************------------------------------------------| 58%
9 -10086.073464526888********************************************************---------------------------------| 66%
10 -11172.340415472303****************************************************************------------------------| 75%
11 -13219.748208010345************************************************************************----------------| 83%
12 -14115.750843779568********************************************************************************--------| 91%
Progress: |***************************************************************************************************| 99%
Episode 1 Mean reward: -0.4283177306652533 Cumulative reward: -15008.253282509391
[WRAPPER LoggerWrapper] (INFO) : End of episode detected, recording summary (progress.csv) if logger is active
#----------------------------------------------------------------------------------------------#
[ENVIRONMENT] (INFO) : Starting a new episode... [SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:48] [Episode 3]
#----------------------------------------------------------------------------------------------#
[MODELING] (INFO) : Episode directory created [/workspaces/sinergym/examples/Eplus-env-SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:48-res35041/Eplus-env-sub_run3]
[MODELING] (INFO) : Weather file USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3.epw used.
[MODELING] (INFO) : Updated building model with whole Output:Variable available names
[MODELING] (INFO) : Updated building model with whole Output:Meter available names
[MODELING] (INFO) : Adapting weather to building model. [USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3.epw]
[ENVIRONMENT] (INFO) : Saving episode output path... [/workspaces/sinergym/examples/Eplus-env-SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:48-res35041/Eplus-env-sub_run3/output]
[SIMULATOR] (INFO) : Running EnergyPlus with args: ['-w', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:48-res35041/Eplus-env-sub_run3/USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3_Random_1.0_0.0_0.001.epw', '-d', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:48-res35041/Eplus-env-sub_run3/output', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:48-res35041/Eplus-env-sub_run3/5ZoneAutoDXVAV.epJSON']
[ENVIRONMENT] (INFO) : Episode 3 started.
/usr/local/lib/python3.10/dist-packages/opyplus/weather_data/weather_data.py:493: FutureWarning: the 'line_terminator'' keyword is deprecated, use 'lineterminator' instead.
epw_content = self._headers_to_epw(use_datetimes=use_datetimes) + df.to_csv(
[SIMULATOR] (INFO) : handlers are ready.
[SIMULATOR] (INFO) : System is ready.
[WRAPPER LoggerWrapper] (INFO) : Creating monitor.csv for current episode (episode 3) if logger is active
1 -1.7042527324647196
Progress: |**-------------------------------------------------------------------------------------------------| 2%
/usr/local/lib/python3.10/dist-packages/gymnasium/spaces/box.py:240: UserWarning: WARN: Casting input x to numpy array.
gym.logger.warn("Casting input x to numpy array.")
2 -934.5236861805773------------------------------------------------------------------------------------------| 9%
3 -1799.7806890630795******-----------------------------------------------------------------------------------| 16%
4 -2820.081000039381****************--------------------------------------------------------------------------| 25%
5 -4295.9802117699255***********************------------------------------------------------------------------| 33%
6 -6066.508686603184********************************----------------------------------------------------------| 41%
7 -7176.935226174976*****************************************-------------------------------------------------| 50%
8 -8529.98270733462*************************************************------------------------------------------| 58%
9 -9927.566541371296*********************************************************---------------------------------| 66%
10 -10989.840486913932****************************************************************------------------------| 75%
11 -12997.533912090104************************************************************************----------------| 83%
12 -13880.172965873764********************************************************************************--------| 91%
Progress: |***************************************************************************************************| 99%
Episode 2 Mean reward: -0.42214784762863855 Cumulative reward: -14792.06058090633
[WRAPPER LoggerWrapper] (INFO) : End of episode detected, recording summary (progress.csv) if logger is active
#----------------------------------------------------------------------------------------------#
[ENVIRONMENT] (INFO) : Starting a new episode... [SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:48] [Episode 4]
#----------------------------------------------------------------------------------------------#
[MODELING] (INFO) : Episode directory created [/workspaces/sinergym/examples/Eplus-env-SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:48-res35041/Eplus-env-sub_run4]
[MODELING] (INFO) : Weather file USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3.epw used.
[MODELING] (INFO) : Updated building model with whole Output:Variable available names
[MODELING] (INFO) : Updated building model with whole Output:Meter available names
[MODELING] (INFO) : Adapting weather to building model. [USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3.epw]
[ENVIRONMENT] (INFO) : Saving episode output path... [/workspaces/sinergym/examples/Eplus-env-SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:48-res35041/Eplus-env-sub_run4/output]
[SIMULATOR] (INFO) : Running EnergyPlus with args: ['-w', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:48-res35041/Eplus-env-sub_run4/USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3_Random_1.0_0.0_0.001.epw', '-d', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:48-res35041/Eplus-env-sub_run4/output', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:48-res35041/Eplus-env-sub_run4/5ZoneAutoDXVAV.epJSON']
[ENVIRONMENT] (INFO) : Episode 4 started.
/usr/local/lib/python3.10/dist-packages/opyplus/weather_data/weather_data.py:493: FutureWarning: the 'line_terminator'' keyword is deprecated, use 'lineterminator' instead.
epw_content = self._headers_to_epw(use_datetimes=use_datetimes) + df.to_csv(
[SIMULATOR] (INFO) : handlers are ready.
[SIMULATOR] (INFO) : System is ready.
[WRAPPER LoggerWrapper] (INFO) : Creating monitor.csv for current episode (episode 4) if logger is active
1 -1.7502089967989307
Progress: |**-------------------------------------------------------------------------------------------------| 2%
/usr/local/lib/python3.10/dist-packages/gymnasium/spaces/box.py:240: UserWarning: WARN: Casting input x to numpy array.
gym.logger.warn("Casting input x to numpy array.")
2 -942.9367504242642------------------------------------------------------------------------------------------| 9%
3 -1800.656177587228*******-----------------------------------------------------------------------------------| 16%
4 -2794.655237212276****************--------------------------------------------------------------------------| 25%
5 -4333.22916802518*************************------------------------------------------------------------------| 33%
6 -6090.34169685729*********************************----------------------------------------------------------| 41%
7 -7224.1494897446855****************************************-------------------------------------------------| 50%
8 -8598.938588370329************************************************------------------------------------------| 58%
9 -9997.264926241101*********************************************************---------------------------------| 66%
10 -11077.148069449044****************************************************************------------------------| 75%
11 -13197.575884449961************************************************************************----------------| 83%
12 -14086.931630958963********************************************************************************--------| 91%
Progress: |***************************************************************************************************| 99%
Episode 3 Mean reward: -0.4275640288043432 Cumulative reward: -14981.8435693031
[WRAPPER LoggerWrapper] (INFO) : End of episode detected, recording summary (progress.csv) if logger is active
#----------------------------------------------------------------------------------------------#
[ENVIRONMENT] (INFO) : Starting a new episode... [SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:48] [Episode 5]
#----------------------------------------------------------------------------------------------#
[MODELING] (INFO) : Episode directory created [/workspaces/sinergym/examples/Eplus-env-SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:48-res35041/Eplus-env-sub_run5]
[MODELING] (INFO) : Weather file USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3.epw used.
[MODELING] (INFO) : Updated building model with whole Output:Variable available names
[MODELING] (INFO) : Updated building model with whole Output:Meter available names
[MODELING] (INFO) : Adapting weather to building model. [USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3.epw]
[ENVIRONMENT] (INFO) : Saving episode output path... [/workspaces/sinergym/examples/Eplus-env-SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:48-res35041/Eplus-env-sub_run5/output]
[SIMULATOR] (INFO) : Running EnergyPlus with args: ['-w', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:48-res35041/Eplus-env-sub_run5/USA_NY_New.York-J.F.Kennedy.Intl.AP.744860_TMY3_Random_1.0_0.0_0.001.epw', '-d', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:48-res35041/Eplus-env-sub_run5/output', '/workspaces/sinergym/examples/Eplus-env-SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:48-res35041/Eplus-env-sub_run5/5ZoneAutoDXVAV.epJSON']
[ENVIRONMENT] (INFO) : Episode 5 started.
/usr/local/lib/python3.10/dist-packages/opyplus/weather_data/weather_data.py:493: FutureWarning: the 'line_terminator'' keyword is deprecated, use 'lineterminator' instead.
epw_content = self._headers_to_epw(use_datetimes=use_datetimes) + df.to_csv(
[SIMULATOR] (INFO) : handlers are ready.
[SIMULATOR] (INFO) : System is ready.
[WRAPPER LoggerWrapper] (INFO) : Creating monitor.csv for current episode (episode 5) if logger is active
1 -1.795342248728848
Progress: |**-------------------------------------------------------------------------------------------------| 2%
/usr/local/lib/python3.10/dist-packages/gymnasium/spaces/box.py:240: UserWarning: WARN: Casting input x to numpy array.
gym.logger.warn("Casting input x to numpy array.")
2 -938.7996243556881------------------------------------------------------------------------------------------| 9%
3 -1819.0145801281317******-----------------------------------------------------------------------------------| 16%
4 -2877.741132595393****************--------------------------------------------------------------------------| 25%
5 -4407.196686348535************************------------------------------------------------------------------| 33%
6 -6174.90714284908*********************************----------------------------------------------------------| 41%
7 -7316.297986671081*****************************************-------------------------------------------------| 50%
8 -8651.146489725308************************************************------------------------------------------| 58%
9 -10070.592382785619********************************************************---------------------------------| 66%
10 -11131.844874744675****************************************************************------------------------| 75%
11 -13342.353151567342************************************************************************----------------| 83%
12 -14226.469403206707********************************************************************************--------| 91%
Progress: |***************************************************************************************************| 99%
Episode 4 Mean reward: -0.43131719567353316 Cumulative reward: -15113.354536399509
[WRAPPER LoggerWrapper] (INFO) : End of episode, recording summary (progress.csv) if logger is active
[ENVIRONMENT] (INFO) : Environment closed. [SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-11-17_09:48]
Finally, we register the evaluation data in wandb as an artifact to save it.
[ ]:
artifact = wandb.Artifact(
name="evaluation1",
type="evaluating")
artifact.add_dir(
env.experiment_path,
name='evaluation_output/')
run.log_artifact(artifact)
# wandb has finished
run.finish()
wandb: Adding directory to artifact (/workspaces/sinergym/examples/Eplus-env-SB3_DQN-EVAL-Eplus-5zone-mixed-discrete-stochastic-v1-episodes-5_2023-08-03_13:36-res1)... Done. 0.1s
Synced 5 W&B file(s), 0 media file(s), 92 artifact file(s) and 0 other file(s)
./wandb/run-20230803_133654-empzk2on/logs
We have the loaded model results in our local computer, but we can see the execution in wandb too:
If we check the wandb project list, we can see that sinergym_evaluations project has a new run:
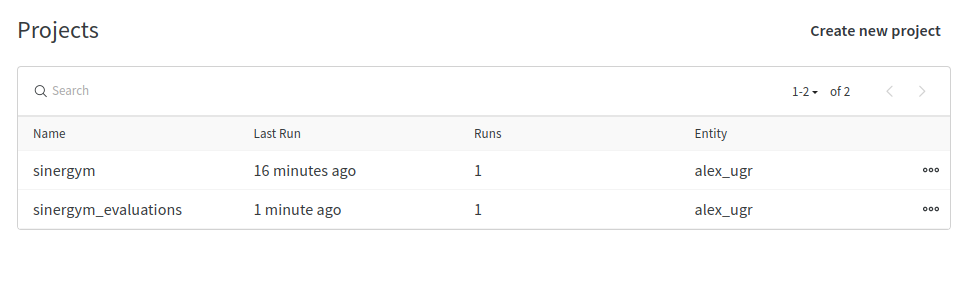
Hyperparameters tracked in the evaluation experiment and we can see the previous training artifact used to load the model:
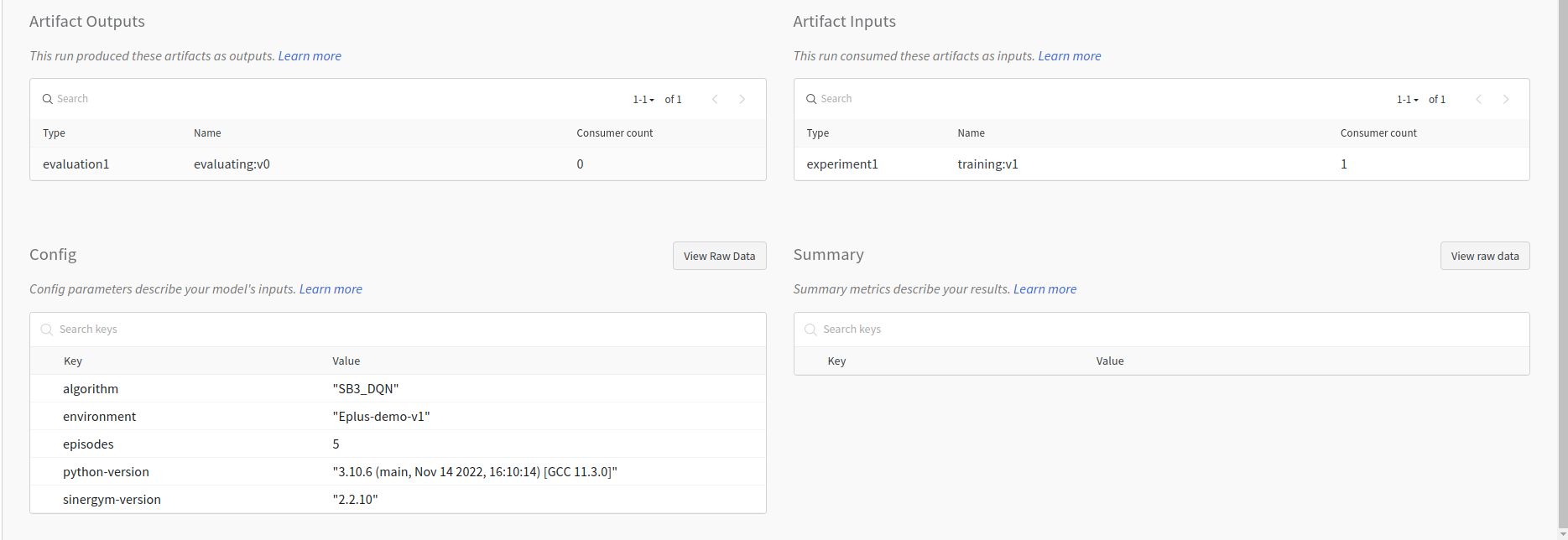
Artifact registered with Sinergym Output (and CSV files generated with the Logger Wrapper):
