Environments
Sinergym generates multiple environments for each building, each defined by a unique configuration that specifies the control problem to be addressed. To view the list of available environment IDs, it is recommended to use the provided method:
# This script is available in scripts/consult_environments.py
import sinergym
import gymnasium as gym
print(sinergym.__version__)
print(sinergym.ids())
# Make and consult environment
env = gym.make('Eplus-5zone-hot-continuous-stochastic-v1')
print(env.get_wrapper_attr('to_str')())
Environment names follow the format Eplus-<building-id>-<weather-id>-<control_type>-<stochastic (optional)>-v1
.
These identifiers provide a general summary of the environment’s characteristics. For more detailed information about a specific environment, use the to_str method as shown in the example code.
Important
Environments are automatically generated using YAML configuration files for each building. This eliminates the need to manually register each environment ID or set parameters directly in the environment constructor. For more information, see Environments configuration and registration.
Note
Additionally, Sinergym supports full serialization of environments and wrappers, enabling easy saving, modification, and restoration of experiments. This ensures reproducibility and simplifies configuration sharing. For more details, see Serialization and Configuration Management.
Note
Discrete environments are fully customizable. By default, these environments use a basic control scheme. However, you can opt for a continuous environment and apply custom discretization using our dedicated wrapper. For further details, refer to DiscretizeEnv.
Note
For additional details on buildings (epJSON) and weather (EPW) configuration, see Buildings and Weathers sections, respectively.
Available parameters
The environment constructor allows you to fully configure the context of an environment for experimentation. You can either start with a predefined setup provided by Sinergym or create a completely new one.
Sinergym initially supplies non-configured buildings and weather files. Based on the arguments provided, these files are automatically updated by Sinergym to accommodate the specified features. For example:
Selecting a different weather file updates the building’s location and simulated days.
Adding new observation variables modifies the
Output:Variable
andOutput:Meter
fields.If weather variability is enabled, a weather file with episodic random noise will be used.
These updated versions of the building and weather files are saved in the Sinergym output folder, while the original files remain untouched.
The following subsections will detail the parameters available and their respective functions.
Building file
The building_file
parameter refers to the epJSON file, an adaptation of the IDF (Intermediate Data Format) used to define EnergyPlus building models.
Before starting the simulation, Sinergym performs a preparatory step to adapt the building model. For more details, refer to the Modeling component in the Sinergym backend diagram.
This parameter can be set to either a single building file name or a full path, if an external building file is being used.
Weather files
The weather_file
parameter specifies the EPW (EnergyPlus Weather) file, which defines the climate conditions for a full year.
This parameter can be provided as a single weather file name (str
) or as a list of multiple weather files (List[str]
). When multiple files are specified, Sinergym will randomly select one EPW file for each episode and automatically adapt the building model accordingly. This feature adds complexity to the environment, if desired.
The weather file used in each episode is saved in the Sinergym episode output folder. If variability (see section Weather variability) is enabled, the stored EPW file will include the corresponding noise adjustments.
Alternatively, the weather_file parameter can also be set to a full path, which is useful when using custom or external weather files not located in the default weather directory. This allows greater flexibility in testing specific climate scenarios or regional data.
Weather variability
Weather variability can be added to an environment using the weather_variability
parameter.
This feature utilizes an Ornstein-Uhlenbeck process to introduce random noise into the weather data on an episode-by-episode basis. This noise is specified as a Python dictionary, where each key is the name of an EPW column, and the corresponding value is a tuple of three variables (\(\sigma\), \(\mu\), and \(\tau\)) that define the characteristics of the noise. This enables to apply different noise configurations to different variables of the weather data.
Starting with Sinergym v3.6.2, the weather data column names (or variable names) are generated using the Weather
class from the epw module. The list of available variable names is as follows:
Year
,Month
,Day
,Hour
,Minute
,Data Source and Uncertainty Flags
,Dry Bulb Temperature
,Dew Point Temperature
,Relative Humidity
,Atmospheric Station Pressure
,Extraterrestrial Horizontal Radiation
,Extraterrestrial Direct Normal Radiation
,Horizontal Infrared Radiation Intensity
,Global Horizontal Radiation
,Direct Normal Radiation
,Diffuse Horizontal Radiation
,Global Horizontal Illuminance
,Direct Normal Illuminance
,Diffuse Horizontal Illuminance
,Zenith Luminance
,Wind Direction
,Wind Speed
,Total Sky Cover
,Opaque Sky Cover (used if Horizontal IR Intensity missing)
,Visibility
,Ceiling Height
,Present Weather Observation
,Present Weather Codes
,Precipitable Water
,Aerosol Optical Depth
,Snow Depth
,Days Since Last Snowfall
,Albedo
,Liquid Precipitation Depth
,Liquid Precipitation Quantity
Note
If you are using an older version of Sinergym, the weather data columns or variables names is
generated with the opyplus WeatherData
class, for more information about the available variable
names with opyplus, visit opyplus documentation.
\(\sigma\) represents the standard deviation of the noise and determines the amplitude of variability. In a climate context, \(\sigma\) can reflect daily or annual fluctuations in temperature (ºC). High values of \(\sigma\) indicate a more unpredictable climate with larger temperature swings, while low values suggest a more stable climate.
\(\mu\) is the mean of the noise, representing the value toward which the process naturally tends to return (ºC). When the random variable in the process deviates from \(\mu\), a restoring force pushes it back toward the mean. If \(\mu\) is set to 0, the process is centered relative to the original climate conditions.
Finally, \(\tau\) is the time constant (in hours), controlling how quickly the process returns to the mean \(\mu\) after a disturbance. A small \(\tau\) indicates a rapid return to the mean, resulting in a less persistent and more rigid system. Conversely, a large \(\tau\) means the process takes longer to stabilize, allowing deviations to persist for a longer time before normalizing.
In climate systems, a large \(\tau\) could model scenarios where extreme events, such as heatwaves or cold spells, last longer before the system reverts to its average state. The next figure illustrates the effect of different hyperparameters on the Ornstein-Uhlenbeck process noise in a mixed weather.
Starting from version 3.7.2 of Sinergym, these hyperparameters (:math:sigma, :math:mu, and :math:tau) can also be defined as ranges of values rather than fixed constants. To configure this, a tuple of two values (minimum and maximum) is used instead of a single float. For each episode, a random value is sampled from the specified range, enabling more dynamic and varied simulations that better capture the inherent unpredictability of climate systems. A CSV file is saved with the chosen parameters in each episode subfolder.
Note
Starting from Sinergym v3.7.1, \(\tau\) is represented in hours instead of as a percentage of the climate file, making its use more intuitive.
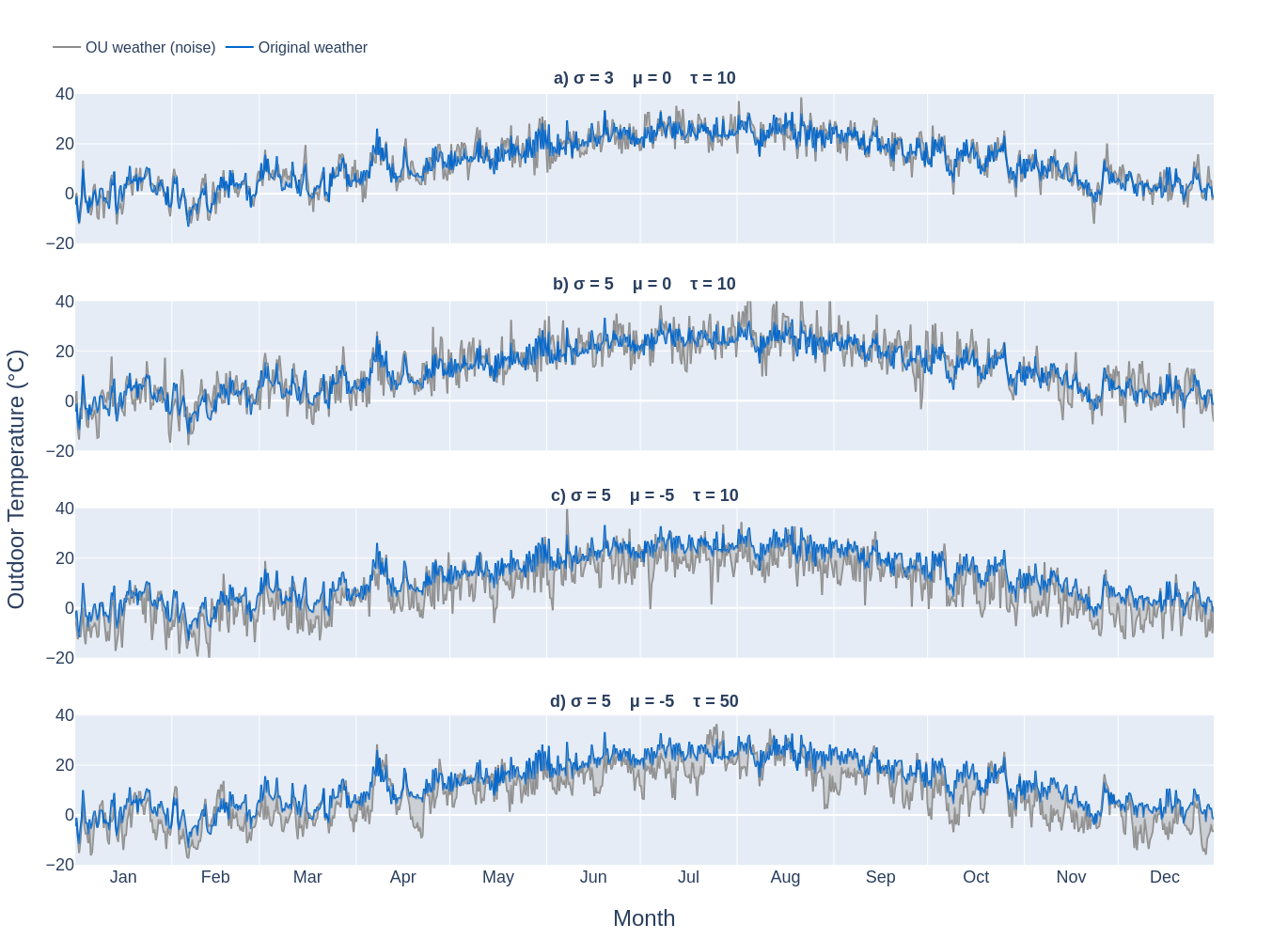
Reward
The reward parameter specifies the reward class (refer to section Rewards) that the environment will use to compute and return scalar reward values at each timestep.
Reward kwargs
The reward_kwargs
parameter is a Python dictionary used to define all the arguments required by the reward class specified for the environment.
The arguments may vary depending on the type of reward class chosen. Additionally, if a user creates a custom reward class, this parameter can include any new arguments needed for that implementation.
Furthermore, these arguments may need to be adjusted based on the building used in the environment. For instance, parameters like the comfort range or the energy and temperature variables used to compute the reward might differ between buildings.
For more details about rewards, refer to section Rewards.
Maximum episode data stored in Sinergym output
Sinergym stores all experiment outputs in a folder, which is organized into sub-folders for each episode (see section Sinergym output for further details). The env_name
parameter is utilized to generate the working directory name, facilitating differentiation between multiple experiments within the same environment.
The parameter max_ep_store
controls the number of episodes’ output data that will be retained. Specifically, the experiment will store the output of the last n
episodes, where n
is defined by this parameter.
If Sinergym’s CSV storage feature is enabled (refer to section CSVLogger), a progress.csv
file will be generated. This file contains summary data for each episode.
Time variables
The EnergyPlus Python API offers several methods to extract information about the ongoing simulation time. The time_variables
argument is a list where you can specify the names of the
API methods with the values to be included in the observations.
By default, Sinergym environments include the time variables month
, day_of_month
and hour
.
Variables
The variables
argument is a dictionary in which it is specified the Output:Variable
entries to be included in the environment’s observation. The format for each element, so that Sinergym can process it correctly, is as follows:
variables = {
# <custom_variable_name> : (<"Output:Variable" original name>,<variable_key>),
# ...
}
Note
For more information about the available variables in an environment, execute a default simulation with EnergyPlus and check the RDD file generated in the output.
Meters
In a similar way, the argument meters
is a dictionary in which we can specify the Output:Meter
’s we want to include in the environment observation.
The format of each element must be the following:
meters = {
# <custom_meter_name> : <"Output:Meter" original name>,
# ...
}
Note
For more information about the available meters in an environment, execute a default simulation with EnergyPlus and see the MDD and MTD files generated in the output.
Actuators
The argument called actuators
is a dictionary in which we specify the actuators to be controlled. The format must be the following:
actuators = {
# <custom_actuator_name> : (<actuator_type>,<actuator_value>,<actuator_original_name>),
# ...
}
Important
Actuators that have not been specified will be controlled by the building’s default schedulers.
Note
For more information about the available actuators in an environment, execute a default control with
Sinergym directly (i.e., with an empty action space) and check the file data_available.txt
generated.
Context
The argument called context
is a dictionary where actuators are also specified in the same way as in Actuators. However, the internal processing differs for the actuators defined here.
These values will not be changed by the actions sent from the agent to the environment using step(a)
method, as happens in Gymnasium’s interaction flow. Instead, these actuators can be modified at any time, outside the control flow, using the update_context(List[float])
method whenever needed.
This allows internal variables within the building to be configured in real time. For example, if we want to enforce either specific occupancy levels or lighting conditions or setpoints values that are not part of the building’s control optimization process.
contexts = {
# <custom_actuator_name> : (<actuator_type>,<actuator_value>,<actuator_original_name>),
# ...
}
Initial context
It is a list containing the initial values of the context variables defined in the previous section. If not specified, it is initialized with the default values provided by the building’s definition.
This list must include the initial values in the same order as defined in the context dictionary and, of course, with the same number of elements.
Action space
In Sinergym, the environment’s observation and action spaces are defined through the arguments time_variables
, variables
, meters
, and actuators
. While the observation space (composed of time_variables
, variables
, and meters
) is automatically generated, the action space (defined by the actuators
) requires explicit definition to establish the range of values supported by the Gymnasium interface or the number of discrete values in a discrete environment.
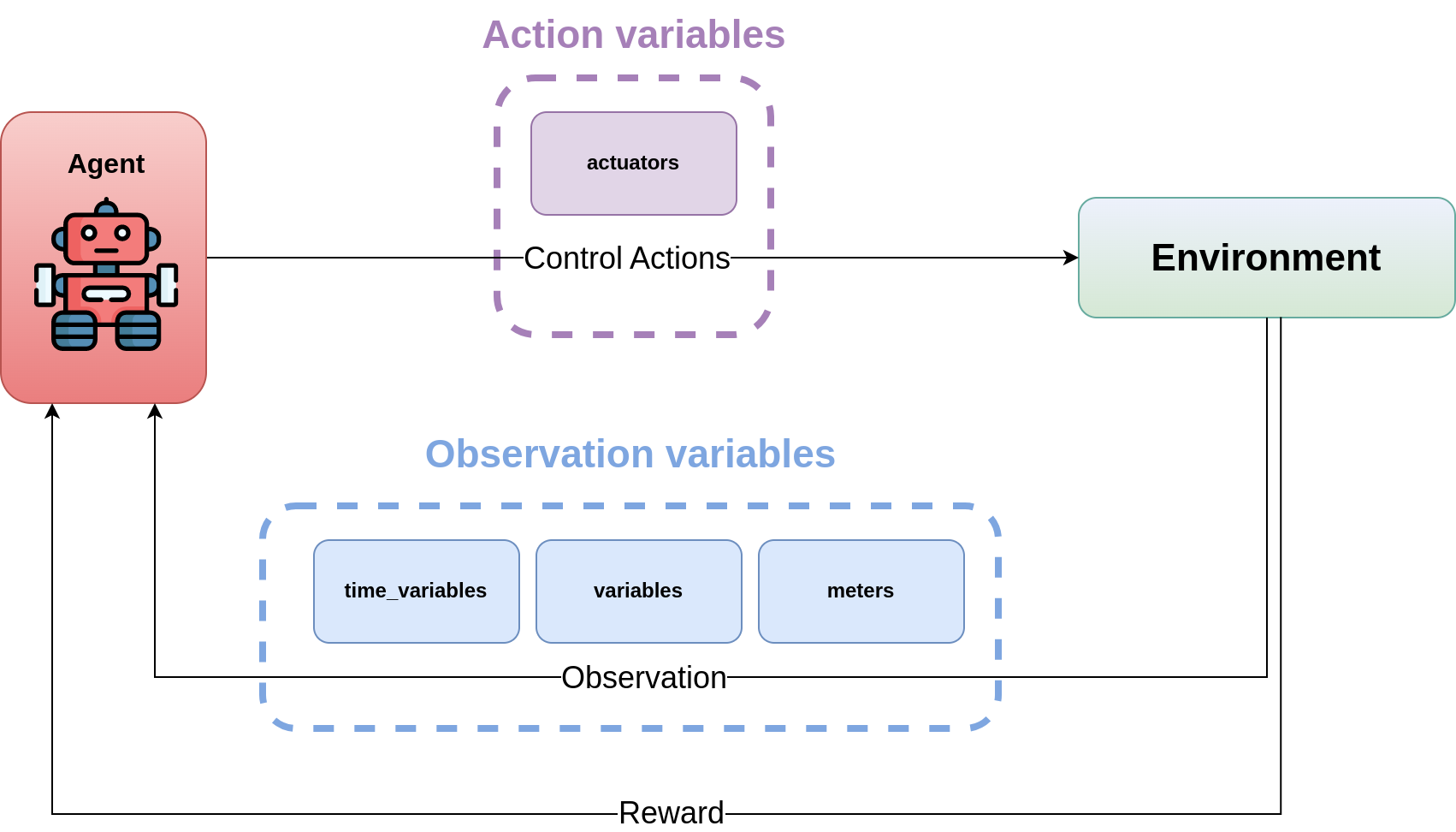
The action_space
argument adheres to the Gymnasium standard and must be a continuous space (gym.spaces.Box
) due to the EnergyPlus simulator’s continuous values requirements. It’s crucial that this definition aligns with the previously defined actuators. In any case, Sinergym will highlight any inconsistencies.
Note
To adapt an environment to Gymnasium’s Discrete
, MultiDiscrete
, or MultiBinary
spaces,
similar to our predefined discrete environments, see section DiscretizeEnv and the
example in Action discretization wrapper.
Important
While Sinergym’s environments come with predefined observation and action variables ( details available in default_configuration), users are encouraged to explore and experiment with these spaces. For guidance, refer to Changing observation and action spaces.
Sinergym also offers the option to create empty action interfaces. In this case, control is managed by the default building model schedulers. For more information, see the usage example in Default building control using an empty action space.
Building extra configuration
Parameters related to the building model and simulation, such as people occupant
, timesteps per simulation hour
, and runperiod
, can be set as extra configurations. These parameters are specified in the building_config
argument, a Python Dictionary. For additional information on extra configurations in Sinergym, refer to Extra configuration in Sinergym simulations.
Seed
The seed
parameter is used to set the random seed for the global environment. This ensures reproducibility in the simulation results, including weather noise and/or dynamic normalization. If not specified, the seed is randomly generated.
Warning
If you define this global seed, the seed feature for reset will be disabled.
Adding new weathers
Sinergym provides a variety of weather files of diverse global climates to enhance experimental diversity.
To incorporate a new weather:
Download an EPW and its corresponding DDY file from the EnergyPlus page. The DDY file provides location and design day details.
Ensure both files share the same name, differing only in their extensions, and place them in the weathers folder.
Sinergym will automatically modify the SizingPeriod:DesignDays
and Site:Location
fields in the building model file using the DDY file.
Adding new buildings
Users can either modify existing environments or create new ones, incorporating new climates, actions, and observation spaces. It is also possible to incorporate new building models (epJSON file) apart from those currently supported.
To add new buildings to Sinergym, follow these steps:
Add your building file (epJSON) to the buildings directory. Ensure it’s compatible with the EnergyPlus version used by Sinergym. If you’re using an IDF file from an older version, update it with IDFVersionUpdater and convert it to epJSON format using ConvertInputFormat. Both tools are available in the EnergyPlus installation folder.
Adjust building objects like
RunPeriod
andSimulationControl
to suit your needs in Sinergym. We recommend settingrun_simulation_for_sizing_periods
toNo
inSimulationControl
.RunPeriod
sets the episode length, which can be configured in the building file or Sinergym settings (see runperiod). Make these modifications in the IDF before step 1 or directly in the epJSON file.Identify the components of the building that you want to observe and control. This is the most challenging part of the process. Typically, users are already familiar with the building and know the name and key of the elements in advance. If not, follow the process below:
Run a preliminary simulation with EnergyPlus directly, without any control, to check the different
OutputVariables
andMeters
. Consult the output files, specifically the RDD extension file, to identify possible observable variables.The challenge is knowing the names but not the possible Keys (EnergyPlus doesn’t initially provide this information). Use these names to define the environment (see step 4). If the Key is incorrect, Sinergym will notify you of the error and provide a
data_available.txt
file in the output, as it has already connected with the EnergyPlus API. This file contains all the controllable schedulers for the actions and all the observable variables, now with their respective Keys, enabling the correct definition of the environment.
With this information, the next step is defining the environment using the building model. You can:
Use the Sinergym environment constructor directly. The arguments for building observation and control are explained within the class and should be specified in the same format as the EnergyPlus API.
Set up the configuration to register environment IDs directly. For more information, refer to Environments configuration and registration. Sinergym will verify that the established configuration is correct and notify about any potential errors.
If you used Sinergym’s registry, you will have access to environment IDs associated with your building. Use them with
gym.make(<environment_id>)
as usual. Besides, if you created an environment instance directly, use that instance to start interacting with the building.
Note
To obtain information about the environment instance with the new building model, refer to Getting information about Sinergym environments.